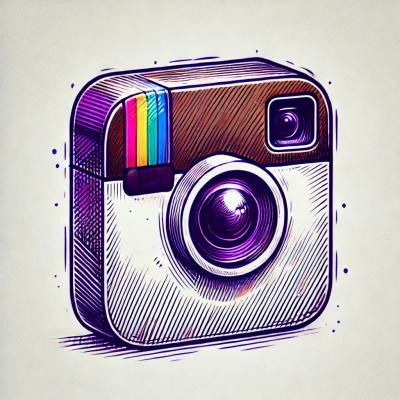
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
extra-array
Advanced tools
An array is a collection of values, stored contiguously.
š¦ Node.js,
š Web,
š Files,
š° Docs,
š Wiki.
This package includes comprehensive set of array functions with which you can generate an array, clone it, query about it, get non-negative indices, manage its length, get/set elements, fully or partially sort it, obtain minimum(s)/maximum(s), compare it with another array, get a part of it, search a value, obtain all possible arrangements or random arrangements, find an element, take/drop elements or scan from its beginning or its end, search the index of a part of it, perform functional operations, flatten multi-level arrays, obtain prefix sum, manipulate it in various ways, count/partition elements, split it, concatenate/join multiple arrays, rearrange elements within it, or performing set operations upon it.
We use a consistent naming scheme that helps you quickly identify the functions
you need. All functions except from*()
take array as 1st parameter. Some
functions operate on a specified range in the array and are called ranged*()
,
such as rangedPartialSort()
. Functions like swap()
are pure and do not
modify the array itself, while functions like swap$()
do modify (update) the
array itself. Some functions accept a map function for faster comparison, such
as unique()
. Further, functions which return an iterable instead of an array
are prefixed with i
, such as isubsequences()
. We borrow some names from
other programming languages such as Haskell, Python, Java, and
Processing.
With this package, you can simplify the implementation of complex algorithms,
and be able to achieve your goals faster, regardless of your level of expertise.
Try it out today and discover how it can transform your development experience!
This package is available in Node.js and Web formats. To use it on the web,
simply use the extra_array
global variable after loading with a <script>
tag
from the jsDelivr CDN.
Stability: Experimental.
NOTE: The use of negative indices in certain functions such as
slice()
is provided as a convenience for access elements from the end of the array. However, negative indices can be thought of as referring to a virtual mirrored version of the original array, which can be counter-intuitive and make it harder to reason about the behavior of functions that use them. We are working on a solution to this problem. Any suggestions are welcome.
const xarray = require('extra-array');
// import * as xarray from "extra-array";
// import * as xarray from "https://unpkg.com/extra-array/index.mjs"; (deno)
var x = [1, 2, 3];
xarray.get(x, -1);
// ā 3
var x = [1, 2, 3, 4];
xarray.swap(x, 0, 1);
// ā [ 2, 1, 3, 4 ]
var x = [1, 2, 3, 4];
xarray.rotate(x, 1);
// ā [ 2, 3, 4, 1 ]
xarray.permutations([1, 2, 3]);
// ā [
// [], [ 1 ],
// [ 2 ], [ 3 ],
// [ 1, 2 ], [ 1, 3 ],
// [ 2, 1 ], [ 2, 3 ],
// [ 3, 1 ], [ 3, 2 ],
// [ 1, 2, 3 ], [ 1, 3, 2 ],
// [ 2, 1, 3 ], [ 2, 3, 1 ],
// [ 3, 1, 2 ], [ 3, 2, 1 ]
// ]
Property | Description |
---|---|
fromRange | Generate array from given number range. |
fromInvocation | Generate array from repeated function invocation. |
fromApplication | Generate array from repeated function application. |
fromIterable | Convert an iterable to array. |
fromIterable$ | Convert an iterable to array! |
shallowClone | Shallow clone an array. |
deepClone | Deep clone an array. |
is | Check if value is an array. |
keys | Obtain all indices. |
values | Get all values. |
entries | Obtain all index-value pairs. |
index | Get zero-based index for an element in array. |
indexRange | Get zero-based index range for part of array. |
isEmpty | Check if an array is empty. |
length | Find the length of an array. |
resize$ | Resize an array to given length! |
clear$ | Remove all elements from an array! |
get | Get value at index. |
getAll | Get values at indices. |
getPath | Get value at path in a nested array. |
hasPath | Check if nested array has a path. |
set | Set value at index. |
set$ | Set value at index! |
setPath$ | Set value at path in a nested array! |
swap | Exchange two values. |
swap$ | Exchange two values! |
swapRanges | Exchange two ranges of values. |
swapRanges$ | Exchange two ranges of values! |
remove | Remove value at index. |
remove$ | Remove value at index! |
removePath$ | Remove value at path in a nested array! |
isSorted | Examine if array is sorted. |
hasUnsortedValue | Examine if array has an unsorted value. |
searchUnsortedValue | Find first index of an unsorted value. |
sort | Arrange values in order. |
sort$ | Arrange values in order! |
partialSort | Partially arrange values in order. |
partialSort$ | Partially arrange values in order! |
minimum | Find first smallest value. |
minimumEntry | Find first smallest entry. |
maximum | Find first largest value. |
maximumEntry | Find first largest entry. |
range | Find smallest and largest values. |
rangeEntries | Find smallest and largest entries. |
minimums | Find smallest values. |
minimumEntries | Find smallest entries. |
maximums | Find largest values. |
maximumEntries | Find largest entries. |
searchMinimumValue | Find first index of minimum value. |
searchMaximumValue | Find first index of maximum value. |
searchMinimumValues | Find indices of minimum values. |
searchMaximumValues | Find indices of maximum values. |
isEqual | Examine if two arrays are equal. |
compare | Compare two arrays (lexicographically). |
head | Get first value. |
tail | Get values except first. |
init | Get values except last. |
last | Get last value. |
middle | Get values from middle. |
slice | Get part of an array. |
slice$ | Get part of an array! |
includes | Check if array has a value. |
hasValue | Examine if array has a value. |
searchValue | Find first index of a value. |
searchValueRight | Find last index of a value. |
searchValueAll | Find indices of value. |
searchAdjacentDuplicateValue | Find first index of an adjacent duplicate value. |
searchMismatchedValue | Find first index where two arrays differ. |
hasPrefix | Examine if array starts with a prefix. |
hasSuffix | Examine if array ends with a suffix. |
hasInfix | Examine if array contains an infix. |
hasSubsequence | Examine if array has a subsequence. |
hasPermutation | Examine if array has a permutation. |
prefixes | Obtain all possible prefixes. |
suffixes | Obtain all possible suffixes. |
infixes | Obtain all possible infixes. |
subsequences | Obtain all possible subsequences. |
permutations | Obtain all possible permutations. |
searchInfix | Find first index of an infix. |
searchInfixRight | Find last index of an infix. |
searchInfixAll | Find indices of an infix. |
searchSubsequence | Find first index of a subsequence. |
randomValue | Pick an arbitrary value. |
randomPrefix | Pick an arbitrary prefix. |
randomSuffix | Pick an arbitrary suffix. |
randomInfix | Pick an arbitrary infix. |
randomSubsequence | Pick an arbitrary subsequence. |
randomPermutation | Pick an arbitrary permutation. |
randomPermutation$ | Pick an arbitrary permutation! |
find | Find first value passing a test. |
findRight | Find last value passing a test. |
take | Keep first n values only. |
takeRight | Keep last n values only. |
takeWhile | Keep values from left, while a test passes. |
takeWhileRight | Keep values from right, while a test passes. |
drop | Discard first n values only. |
dropRight | Discard last n values only. |
dropWhile | Discard values from left, while a test passes. |
dropWhileRight | Discard values from right, while a test passes. |
scanWhile | Scan from left, while a test passes. |
scanWhileRight | Scan from right, while a test passes. |
scanUntil | Scan from left, until a test passes. |
scanUntilRight | Scan from right, until a test passes. |
indexOf | Find first index of a value. |
lastIndexOf | Find last index of a value. |
search | Find index of first value passing a test. |
searchRight | Find index of last value passing a test. |
searchAll | Find indices of values passing a test. |
forEach | Call a function for each value. |
some | Examine if any value satisfies a test. |
every | Examine if all values satisfy a test. |
map | Transform values of an array. |
map$ | Transform values of an array! |
reduce | Reduce values of array to a single value. |
reduceRight | Reduce values from right, to a single value. |
filter | Keep values which pass a test. |
filter$ | Keep values which pass a test! |
filterAt | Keep values at given indices. |
reject | Discard values which pass a test. |
reject$ | Discard values which pass a test! |
rejectAt | Discard values at given indices. |
flat | Flatten nested array to given depth. |
flatMap | Flatten nested array, based on map function. |
exclusiveScan | Perform exclusive prefix scan from left to right. |
exclusiveScan$ | Perform exclusive prefix scan from left to right! |
inclusiveScan | Perform inclusive prefix scan from left to right. |
inclusiveScan$ | Perform inclusive prefix scan from left to right! |
adjacentCombine | Combine adjacent values of an array. |
adjacentCombine$ | Combine adjacent values of an array! |
intersperse | Place a separator between every value. |
interpolate | Estimate new values between existing ones. |
intermix | Place values of an array between another. |
interleave | Place values from iterables alternately. |
zip | Combine values from arrays. |
fill | Fill with given value. |
fill$ | Fill with given value! |
push | Add value to the end. |
push$ | Add values to the end! |
pop | Remove last value. |
pop$ | Remove last value! |
shift | Remove first value. |
shift$ | Remove first value! |
unshift | Add values to the start. |
unshift$ | Add values to the start! |
copy | Copy part of array to another. |
copy$ | Copy part of array to another! |
copyWithin | Copy part of array within. |
copyWithin$ | Copy part of array within! |
moveWithin | Move part of array within. |
moveWithin$ | Move part of array within! |
splice | Remove or replace existing values. |
splice$ | Remove or replace existing values! |
count | Count values which satisfy a test. |
countEach | Count occurrences of each distinct value. |
partition | Segregate values by test result. |
partitionEach | Segregate each distinct value. |
split | Break array considering test as separator. |
splitAt | Break array considering indices as separator. |
cut | Break array when test passes. |
cutRight | Break array after test passes. |
cutAt | Break array at given indices. |
cutAtRight | Break array after given indices. |
group | Keep similar values together and in order. |
chunk | Break array into chunks of given size. |
concat | Append values from arrays. |
concat$ | Append values from arrays! |
join | Join values together into a string. |
cycle | Obtain values that cycle through array. |
repeat | Repeat an array given times. |
reverse | Reverse the values. |
reverse$ | Reverse the values! |
rotate | Rotate values in array. |
rotate$ | Rotate values in array! |
isUnique | Examine if there are no duplicate values. |
isDisjoint | Examine if arrays have no value in common. |
unique | Remove duplicate values. |
union | Obtain values present in any array. |
union$ | Obtain values present in any array! |
intersection | Obtain values present in both arrays. |
difference | Obtain values not present in another array. |
symmetricDifference | Obtain values not present in both arrays. |
cartesianProduct | Obtain cartesian product of arrays. |
FAQs
An array is a collection of values, stored contiguously.
The npm package extra-array receives a total of 1,037 weekly downloads. As such, extra-array popularity was classified as popular.
We found that extra-array demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Ā It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.