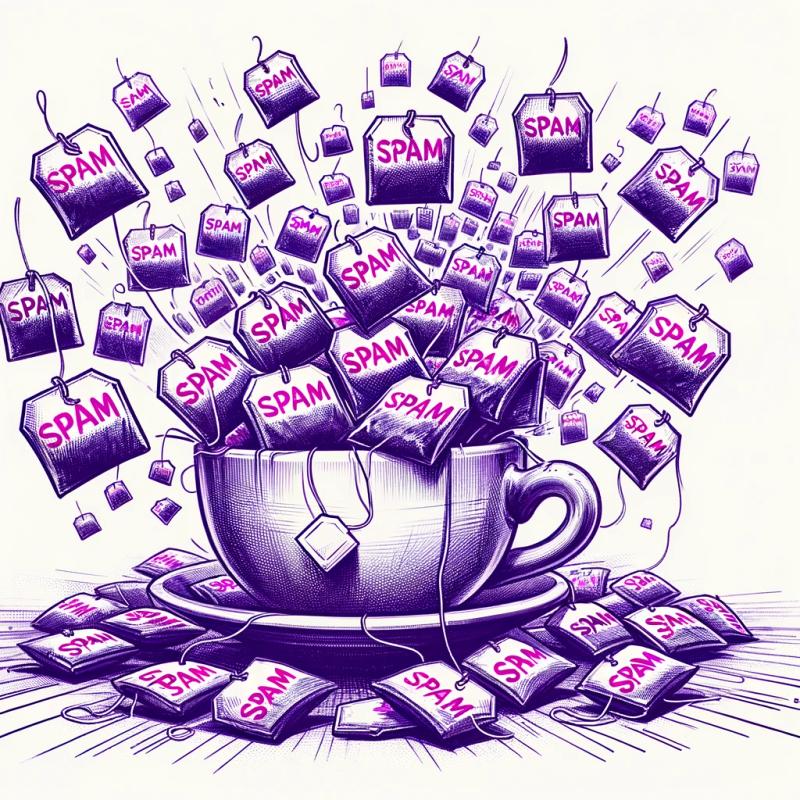
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
extra-lazy
Advanced tools
Readme
Yet another lazy evaluation library.
npm install --save extra-lazy
# or
yarn add extra-lazy
import { lazy } from 'extra-lazy'
const getValue = lazy(() => {
// ...
return value
})
const value = getValue()
function lazy<T>(getter: () => T): () => T
Create a value lazily.
which implicitly has memoization, because the evaluation will only be performed once.
function weakLazy<T extends object>(getter: () => T): () => T
function lazyFunction<Result, Args extends any[]>(
getter: () => (...args: Args) => Result
): (...args: Args) => Result
Create a function lazily.
function lazyAsyncFunction<Result, Args extends any[]>(
getter: () => PromiseLike<(...args: Args) => Result>
): (...args: Args) => Promise<Result>
Create a async function lazily.
function lazyStatic<T>(
getter: () => T
, deps?: unknown[] = []
): T
/**
* @param fn
* The function must satisfy the following conditions, it's like React hooks very much:
* - The function should not be an async function,
* it is impossible to ensure that `lazyStatic` works correctly in asynchronous flows.
* - `lazyStatic` calls should not be in loops or branches.
*/
function withLazyStatic<Result, Args extends any[]>(
fn: (...args: Args) => Result
): (...args: Args) => Result
Example:
const fn = withLazyStatic((text: string) => lazyStatic(() => text))
fn('hello') // 'hello'
fn('world') // 'hello'
// bad
withLazyStatic(() => {
while (condition) {
const value = lazyStatic(() => {
// ...
})
// ...
}
})
// good
withLazyStatic(() => {
const value = lazyStatic(() => {
// ...
})
while (condition) {
// ...
}
})
// bad
withLazyStatic(() => {
if (condition) {
const value = lazyStatic(() => {
// ...
})
// ...
} else {
// ...
}
})
// good
withLazyStatic(() => {
const value = lazyStatic(() => {
// ...
})
if (condition) {
// ...
} else {
// ...
}
})
// bad
withLazyStatic((form: IForm) => {
if (validate(form)) {
const value = lazyStatic(() => {
// ...
})
// ...
} else {
const value = lazyStatic(() => {
// ...
})
return null // or throw an error
}
})
// okay
withLazyStatic((form: IForm) => {
if (validate(form)) {
const value = lazyStatic(() => {
// ...
})
// ...
} else {
return null // or throw an error
}
})
// good, `lazyStatic` can always be called after guards
withLazyStatic((form: IForm) => {
if (!validate(form)) return null // or throw an error
const value = lazyStatic(() => {
// ...
})
// ...
})
FAQs
Yet another lazy evaluation library.
The npm package extra-lazy receives a total of 883 weekly downloads. As such, extra-lazy popularity was classified as not popular.
We found that extra-lazy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.