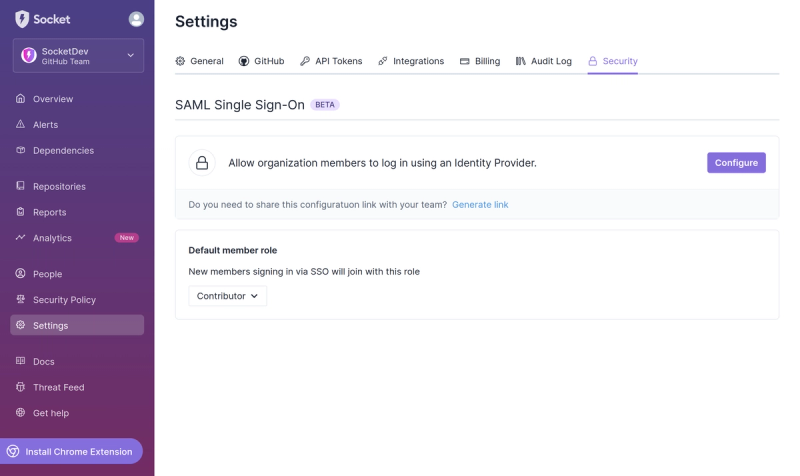
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
fast-insta-api
Advanced tools
Changelog
Readme
Simple, easy implementation of the Instagram private web API.
Some API reference from jlobos/instagram-web-api
Send DM using client from dilame/instagram-private-api
npm install fast-insta-api
//send dm by username
const Insta = require('node-insta-web-api')
const InstaClient = new Insta();
(async () => {
//required username & password for login
const username = '';
const password = '';
const usernameReceiver = ['username target'];
const message = 'text message';
const result = await InstaClient.sendDmByUsername(username, password, usernameReceiver, message);
console.log(result)
})()
//get profile data
const Insta = require('node-insta-web-api')
const InstaClient = new Insta();
(async () => {
await InstaClient.login('username','password');
const profileData = await InstaClient.getProfileData();
console.log(profileData)
})()
//get image by username
const Insta = require('node-insta-web-api')
const InstaClient = new Insta();
(async () => {
await Insta.getCookie()
const photos = await Insta.getImageByUser('username');
console.log(photos)
})()
//update bio using existing cookies
const Insta = require('node-insta-web-api')
const InstaClient = new Insta();
(async () => {
await InstaClient.useExistingCookie()
const payload = {
biography: 'test update bio 1'
}
const result = await InstaClient.updateProfile(payload)
console.log(result)
})()
//get following with pagination using existing cookie
await InstaClient.useExistingCookie();
const dataUser = await InstaClient.getProfileByUsername('amin_udin69');
let following;
let hasNextPage;
let endCursor = '';
const resultAllFollowing = [];
do{
following = await InstaClient.getFollowingByDataUser(dataUser, 12, endCursor);
hasNextPage = following.page_info.has_next_page;
endCursor = following.page_info.end_cursor;
for (let index = 0; index < following.edges.length; index++) {
const element = following.edges[index];
resultAllFollowing.push(element.node)
}
}while(hasNextPage);
console.log(resultAllFollowing)
await client.getCookie()
getting guest cookie
await client._getMediaId('https://www.instagram.com/p/CDFIAxxxxx/')
getting media id by url
url
: A String
await client.useExistingCookie()
u can use existing cookies, if you don't want to log in repeatedly
await client.login('username', 'password')
Login.
username
: A String
password
: A String
//login required
await InstaClient.login('username','password');
const profileData = await InstaClient.getProfileData();
console.log(profileData)
Getting profile data.
//login required
//using a url is under development
//by url
await InstaClient.login('username','password');
await InstaClient.changeProfileImage('url')
//by path
await InstaClient.login('username','password');
const photo = path.join(__dirname, 'blackhat.png');
await InstaClient.changeProfileImage(photo)
Change Profile Image.
image
: A String
url / image path const payload = {
biography: 'test update bio 1',
email: 'update@email.com'
}
const a = await InstaClient.updateProfile(payload)
console.log(a)
update profile. for now you can only update your bio.
params
biography
: A String
email
: A String
await client.getImageByUser('username')
Gets user photos.
username
: A String
const data = await InstaClient.getVideoByShortCode('CDDs8unBjXX');
fs.writeFileSync('./test.mp4', data.base64, 'base64')
console.log(data)
Get video base64 and buffer by short code.
shortCode
: A String
//login required
await InstaClient.useExistingCookie()
const data = await InstaClient.getLoginActivity();
console.log(data)
get login activity.
//login required
await InstaClient.useExistingCookie()
const data = await InstaClient.getRecentNotification();
console.log(data)
get recent notification.
//login required
await InstaClient.useExistingCookie()
const data = await InstaClient.getDirectMessage();
console.log(data)
get direct message.
await InstaClient.getCookie()
const data = await InstaClient.getProfileByUsername('username');
console.log(data)
get profile user.
username
: A String
//login required
await InstaClient.useExistingCookie()
const data = await InstaClient.followByUsername('username');
console.log(data)
follow user by username.
username
: A String
//login required
await InstaClient.useExistingCookie()
const data = await InstaClient.unfollowByUsername('username');
console.log(data)
unfollow user by username.
username
: A String
await InstaClient.useExistingCookie()
const data = await InstaClient.getStoriesByUsername('username');
console.log(data)
get stories by username.
username
: A String
await InstaClient.useExistingCookie()
const data = await InstaClient.likeMediaById(00000);
console.log(data)
like media by media id
mediaId
: A Number
await InstaClient.useExistingCookie()
const data = await InstaClient.likeMediaByShortCode('CDFIAQtHUxxxx');
console.log(data)
like media by shortcode
shortCode
: A String
await InstaClient.useExistingCookie()
const data = await InstaClient.unlikeMediaByShortCode('CDFIAQtHUxxxx');
console.log(data)
unlike media by shortcode
shortCode
: A String
await InstaClient.useExistingCookie()
const data = await InstaClient.deleteMediaByShortCode('CDFIAQtHUxxxx');
console.log(data)
delete media by shortcode
shortCode
: A String
await InstaClient.useExistingCookie()
const data = await InstaClient.saveImageByShortCode('CDFIAQtHUxxxx');
console.log(data)
save media by shortcode
shortCode
: A String
await InstaClient.useExistingCookie()
const data = await InstaClient.unsaveImageByShortCode('CDFIAQtHUxxxx');
console.log(data)
save media by shortcode
shortCode
: A String
await InstaClient.useExistingCookie()
const payload = {
mediaId: 100000,
commentText: 'Your Text Comment'
}
const data = await InstaClient.commentToMediaByMediaId(payload);
console.log(data)
add comment to a media by shortcode
params
mediaId
: A Number
commentText
: A String
await InstaClient.useExistingCookie()
const payload = {
shortCode:'CDFIAQxxxx',
commentText: 'Your Text Comment'
}
const data = await InstaClient.commentToMediaByShortCode(payload);
console.log(data)
add comment to a media by shortcode
params
shortCode
: A String
commentText
: A String
await InstaClient.useExistingCookie()
const payload = {
shortCode:'CDFIAQtxxxx',
commentText: '%40username reply comment',
commentId: '17870873200867xxx'
}
const data = await InstaClient.replyCommentByShortCode(payload);
console.log(data)
reply comment in media by shortcode
params
shortCode
: A String
commentText
: A String
commentId
: A String
await InstaClient.useExistingCookie()
const data = await InstaClient.getEmbedMediaByShortCode('CDFIAQtHUiw');
console.log(data)
get embed media by shortCode
shortCode
: A String
await InstaClient.useExistingCookie()
const data = await InstaClient.getMediaFeedByHashtag('berita');
console.log(data)
get post by hastag
name
: A String
await InstaClient.useExistingCookie()
const data = await InstaClient.getUserPostById(00000);
console.log(data)
get post by user id
userId
: A Number
await InstaClient.useExistingCookie()
const data = await InstaClient.findPeopleByUserId(00000);
console.log(data)
find people by userid
userid
: A Number
await InstaClient.useExistingCookie()
const data = await InstaClient.findPeopleByUsername('menjadi');
console.log(data)
find people by username
username
: A String
const photo = path.join(__dirname, '3.jpeg');
await InstaClient.useExistingCookie();
const resultAddPost = await InstaClient.addPost(photo, 'this is caption');
console.log(resultAddPost)
add post to feed
image
: A String
path of imagecaption
: A String
const photo = path.join(__dirname, '3.jpeg');
await InstaClient.useExistingCookie();
const resultAddStory = await InstaClient.addStory(photo);
console.log(resultAddStory)
add story
image
: A String
path of image await InstaClient.useExistingCookie();
const dataUser = await InstaClient.getProfileByUsername('amin_udin69');
let following;
let hasNextPage;
let endCursor = '';
const resultAllFollowing = [];
do{
following = await InstaClient.getFollowingByDataUser(dataUser, 12, endCursor);
hasNextPage = following.page_info.has_next_page;
endCursor = following.page_info.end_cursor;
for (let index = 0; index < following.edges.length; index++) {
const element = following.edges[index];
resultAllFollowing.push(element.node)
}
}while(hasNextPage);
console.log(resultAllFollowing)
get following by data user
dataUser
: A Object
data usersize
: A Number
size per pagecursor
: A String
end cursor//login required
const username = ''; //required
const password = ''; //required
const usernameReceiver = ['username target'];
const message = 'text message';
const result = await InstaClient.sendDmByUsername(username, password, usernameReceiver, message);
console.log(result)
send dm
username
: A String
username for loginpassword
: A String
password for loginusernameReceiver
: A Array
list username receiver message/dmmessage
: A String
text message await InstaClient.useExistingCookie();
const sendConfirmationEmailResult = await InstaClient.sendConfirmationEmail();
console.log(sendConfirmationEmailResult)
MIT © Archv Id
FAQs
Simple, easy implementation of the Instagram private web API.
The npm package fast-insta-api receives a total of 10 weekly downloads. As such, fast-insta-api popularity was classified as not popular.
We found that fast-insta-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.