fast-json-patch
Advanced tools
Comparing version 2.0.7 to 2.1.0
@@ -1,2 +0,2 @@ | ||
/*! fast-json-patch, version: 2.0.7 */ | ||
/*! fast-json-patch, version: 2.1.0 */ | ||
var jsonpatch = | ||
@@ -68,3 +68,3 @@ /******/ (function(modules) { // webpackBootstrap | ||
/******/ // Load entry module and return exports | ||
/******/ return __webpack_require__(__webpack_require__.s = 3); | ||
/******/ return __webpack_require__(__webpack_require__.s = 2); | ||
/******/ }) | ||
@@ -76,2 +76,7 @@ /************************************************************************/ | ||
/*! | ||
* https://github.com/Starcounter-Jack/JSON-Patch | ||
* (c) 2017 Joachim Wester | ||
* MIT license | ||
*/ | ||
var __extends = (this && this.__extends) || function (d, b) { | ||
@@ -82,7 +87,2 @@ for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; | ||
}; | ||
/*! | ||
* https://github.com/Starcounter-Jack/JSON-Patch | ||
* (c) 2017 Joachim Wester | ||
* MIT license | ||
*/ | ||
var _hasOwnProperty = Object.prototype.hasOwnProperty; | ||
@@ -224,7 +224,16 @@ function hasOwnProperty(obj, key) { | ||
exports.hasUndefined = hasUndefined; | ||
function patchErrorMessageFormatter(message, args) { | ||
var messageParts = [message]; | ||
for (var key in args) { | ||
var value = typeof args[key] === 'object' ? JSON.stringify(args[key], null, 2) : args[key]; // pretty print | ||
if (typeof value !== 'undefined') { | ||
messageParts.push(key + ": " + value); | ||
} | ||
} | ||
return messageParts.join('\n'); | ||
} | ||
var PatchError = (function (_super) { | ||
__extends(PatchError, _super); | ||
function PatchError(message, name, index, operation, tree) { | ||
_super.call(this, message); | ||
this.message = message; | ||
_super.call(this, patchErrorMessageFormatter(message, { name: name, index: index, operation: operation, tree: tree })); | ||
this.name = name; | ||
@@ -234,2 +243,3 @@ this.index = index; | ||
this.tree = tree; | ||
this.message = patchErrorMessageFormatter(message, { name: name, index: index, operation: operation, tree: tree }); | ||
} | ||
@@ -246,3 +256,3 @@ return PatchError; | ||
var equalsOptions = { strict: true }; | ||
var _equals = __webpack_require__(2); | ||
var _equals = __webpack_require__(3); | ||
var areEquals = function (a, b) { | ||
@@ -356,7 +366,10 @@ return _equals(a, b, equalsOptions); | ||
* @param mutateDocument Whether to mutate the original document or clone it before applying | ||
* @param banPrototypeModifications Whether to ban modifications to `__proto__`, defaults to `true`. | ||
* @return `{newDocument, result}` after the operation | ||
*/ | ||
function applyOperation(document, operation, validateOperation, mutateDocument) { | ||
function applyOperation(document, operation, validateOperation, mutateDocument, banPrototypeModifications, index) { | ||
if (validateOperation === void 0) { validateOperation = false; } | ||
if (mutateDocument === void 0) { mutateDocument = true; } | ||
if (banPrototypeModifications === void 0) { banPrototypeModifications = true; } | ||
if (index === void 0) { index = 0; } | ||
if (validateOperation) { | ||
@@ -392,3 +405,3 @@ if (typeof validateOperation == 'function') { | ||
if (returnValue.test === false) { | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', 0, operation, document); | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', index, operation, document); | ||
} | ||
@@ -409,3 +422,3 @@ returnValue.newDocument = document; | ||
if (validateOperation) { | ||
throw new exports.JsonPatchError('Operation `op` property is not one of operations defined in RFC-6902', 'OPERATION_OP_INVALID', 0, operation, document); | ||
throw new exports.JsonPatchError('Operation `op` property is not one of operations defined in RFC-6902', 'OPERATION_OP_INVALID', index, operation, document); | ||
} | ||
@@ -437,2 +450,5 @@ else { | ||
key = keys[t]; | ||
if (banPrototypeModifications && key == '__proto__') { | ||
throw new TypeError('JSON-Patch: modifying `__proto__` prop is banned for security reasons, if this was on purpose, please set `banPrototypeModifications` flag false and pass it to this function. More info in fast-json-patch README'); | ||
} | ||
if (validateOperation) { | ||
@@ -458,3 +474,3 @@ if (existingPathFragment === undefined) { | ||
if (validateOperation && !helpers_1.isInteger(key)) { | ||
throw new exports.JsonPatchError("Expected an unsigned base-10 integer value, making the new referenced value the array element with the zero-based index", "OPERATION_PATH_ILLEGAL_ARRAY_INDEX", 0, operation.path, operation); | ||
throw new exports.JsonPatchError("Expected an unsigned base-10 integer value, making the new referenced value the array element with the zero-based index", "OPERATION_PATH_ILLEGAL_ARRAY_INDEX", index, operation, document); | ||
} // only parse key when it's an integer for `arr.prop` to work | ||
@@ -467,7 +483,7 @@ else if (helpers_1.isInteger(key)) { | ||
if (validateOperation && operation.op === "add" && key > obj.length) { | ||
throw new exports.JsonPatchError("The specified index MUST NOT be greater than the number of elements in the array", "OPERATION_VALUE_OUT_OF_BOUNDS", 0, operation.path, operation); | ||
throw new exports.JsonPatchError("The specified index MUST NOT be greater than the number of elements in the array", "OPERATION_VALUE_OUT_OF_BOUNDS", index, operation, document); | ||
} | ||
var returnValue = arrOps[operation.op].call(operation, obj, key, document); // Apply patch | ||
if (returnValue.test === false) { | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', 0, operation, document); | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', index, operation, document); | ||
} | ||
@@ -484,3 +500,3 @@ return returnValue; | ||
if (returnValue.test === false) { | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', 0, operation, document); | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', index, operation, document); | ||
} | ||
@@ -506,6 +522,8 @@ return returnValue; | ||
* @param mutateDocument Whether to mutate the original document or clone it before applying | ||
* @param banPrototypeModifications Whether to ban modifications to `__proto__`, defaults to `true`. | ||
* @return An array of `{newDocument, result}` after the patch | ||
*/ | ||
function applyPatch(document, patch, validateOperation, mutateDocument) { | ||
function applyPatch(document, patch, validateOperation, mutateDocument, banPrototypeModifications) { | ||
if (mutateDocument === void 0) { mutateDocument = true; } | ||
if (banPrototypeModifications === void 0) { banPrototypeModifications = true; } | ||
if (validateOperation) { | ||
@@ -521,3 +539,4 @@ if (!Array.isArray(patch)) { | ||
for (var i = 0, length_1 = patch.length; i < length_1; i++) { | ||
results[i] = applyOperation(document, patch[i], validateOperation); | ||
// we don't need to pass mutateDocument argument because if it was true, we already deep cloned the object, we'll just pass `true` | ||
results[i] = applyOperation(document, patch[i], validateOperation, true, banPrototypeModifications, i); | ||
document = results[i].newDocument; // in case root was replaced | ||
@@ -538,6 +557,6 @@ } | ||
*/ | ||
function applyReducer(document, operation) { | ||
function applyReducer(document, operation, index) { | ||
var operationResult = applyOperation(document, operation); | ||
if (operationResult.test === false) { | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', 0, operation, document); | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', index, operation, document); | ||
} | ||
@@ -639,107 +658,7 @@ return operationResult.newDocument; | ||
var pSlice = Array.prototype.slice; | ||
var objectKeys = __webpack_require__(5); | ||
var isArguments = __webpack_require__(4); | ||
var deepEqual = module.exports = function (actual, expected, opts) { | ||
if (!opts) opts = {}; | ||
// 7.1. All identical values are equivalent, as determined by ===. | ||
if (actual === expected) { | ||
return true; | ||
} else if (actual instanceof Date && expected instanceof Date) { | ||
return actual.getTime() === expected.getTime(); | ||
// 7.3. Other pairs that do not both pass typeof value == 'object', | ||
// equivalence is determined by ==. | ||
} else if (!actual || !expected || typeof actual != 'object' && typeof expected != 'object') { | ||
return opts.strict ? actual === expected : actual == expected; | ||
// 7.4. For all other Object pairs, including Array objects, equivalence is | ||
// determined by having the same number of owned properties (as verified | ||
// with Object.prototype.hasOwnProperty.call), the same set of keys | ||
// (although not necessarily the same order), equivalent values for every | ||
// corresponding key, and an identical 'prototype' property. Note: this | ||
// accounts for both named and indexed properties on Arrays. | ||
} else { | ||
return objEquiv(actual, expected, opts); | ||
} | ||
} | ||
function isUndefinedOrNull(value) { | ||
return value === null || value === undefined; | ||
} | ||
function isBuffer (x) { | ||
if (!x || typeof x !== 'object' || typeof x.length !== 'number') return false; | ||
if (typeof x.copy !== 'function' || typeof x.slice !== 'function') { | ||
return false; | ||
} | ||
if (x.length > 0 && typeof x[0] !== 'number') return false; | ||
return true; | ||
} | ||
function objEquiv(a, b, opts) { | ||
var i, key; | ||
if (isUndefinedOrNull(a) || isUndefinedOrNull(b)) | ||
return false; | ||
// an identical 'prototype' property. | ||
if (a.prototype !== b.prototype) return false; | ||
//~~~I've managed to break Object.keys through screwy arguments passing. | ||
// Converting to array solves the problem. | ||
if (isArguments(a)) { | ||
if (!isArguments(b)) { | ||
return false; | ||
} | ||
a = pSlice.call(a); | ||
b = pSlice.call(b); | ||
return deepEqual(a, b, opts); | ||
} | ||
if (isBuffer(a)) { | ||
if (!isBuffer(b)) { | ||
return false; | ||
} | ||
if (a.length !== b.length) return false; | ||
for (i = 0; i < a.length; i++) { | ||
if (a[i] !== b[i]) return false; | ||
} | ||
return true; | ||
} | ||
try { | ||
var ka = objectKeys(a), | ||
kb = objectKeys(b); | ||
} catch (e) {//happens when one is a string literal and the other isn't | ||
return false; | ||
} | ||
// having the same number of owned properties (keys incorporates | ||
// hasOwnProperty) | ||
if (ka.length != kb.length) | ||
return false; | ||
//the same set of keys (although not necessarily the same order), | ||
ka.sort(); | ||
kb.sort(); | ||
//~~~cheap key test | ||
for (i = ka.length - 1; i >= 0; i--) { | ||
if (ka[i] != kb[i]) | ||
return false; | ||
} | ||
//equivalent values for every corresponding key, and | ||
//~~~possibly expensive deep test | ||
for (i = ka.length - 1; i >= 0; i--) { | ||
key = ka[i]; | ||
if (!deepEqual(a[key], b[key], opts)) return false; | ||
} | ||
return typeof a === typeof b; | ||
} | ||
/***/ }), | ||
/* 3 */ | ||
/***/ (function(module, exports, __webpack_require__) { | ||
var equalsOptions = { strict: true }; | ||
var _equals = __webpack_require__(2); | ||
var areEquals = function (a, b) { | ||
return _equals(a, b, equalsOptions); | ||
}; | ||
/*! | ||
* https://github.com/Starcounter-Jack/JSON-Patch | ||
* (c) 2017 Joachim Wester | ||
* MIT license | ||
*/ | ||
var helpers_1 = __webpack_require__(0); | ||
@@ -911,6 +830,10 @@ var core_1 = __webpack_require__(1); | ||
} | ||
else { | ||
else if (Array.isArray(mirror) === Array.isArray(obj)) { | ||
patches.push({ op: "remove", path: path + "/" + helpers_1.escapePathComponent(key) }); | ||
deleted = true; // property has been deleted | ||
} | ||
else { | ||
patches.push({ op: "replace", path: path, value: obj }); | ||
changed = true; | ||
} | ||
} | ||
@@ -939,2 +862,102 @@ if (!deleted && newKeys.length == oldKeys.length) { | ||
/***/ }), | ||
/* 3 */ | ||
/***/ (function(module, exports, __webpack_require__) { | ||
var pSlice = Array.prototype.slice; | ||
var objectKeys = __webpack_require__(5); | ||
var isArguments = __webpack_require__(4); | ||
var deepEqual = module.exports = function (actual, expected, opts) { | ||
if (!opts) opts = {}; | ||
// 7.1. All identical values are equivalent, as determined by ===. | ||
if (actual === expected) { | ||
return true; | ||
} else if (actual instanceof Date && expected instanceof Date) { | ||
return actual.getTime() === expected.getTime(); | ||
// 7.3. Other pairs that do not both pass typeof value == 'object', | ||
// equivalence is determined by ==. | ||
} else if (!actual || !expected || typeof actual != 'object' && typeof expected != 'object') { | ||
return opts.strict ? actual === expected : actual == expected; | ||
// 7.4. For all other Object pairs, including Array objects, equivalence is | ||
// determined by having the same number of owned properties (as verified | ||
// with Object.prototype.hasOwnProperty.call), the same set of keys | ||
// (although not necessarily the same order), equivalent values for every | ||
// corresponding key, and an identical 'prototype' property. Note: this | ||
// accounts for both named and indexed properties on Arrays. | ||
} else { | ||
return objEquiv(actual, expected, opts); | ||
} | ||
} | ||
function isUndefinedOrNull(value) { | ||
return value === null || value === undefined; | ||
} | ||
function isBuffer (x) { | ||
if (!x || typeof x !== 'object' || typeof x.length !== 'number') return false; | ||
if (typeof x.copy !== 'function' || typeof x.slice !== 'function') { | ||
return false; | ||
} | ||
if (x.length > 0 && typeof x[0] !== 'number') return false; | ||
return true; | ||
} | ||
function objEquiv(a, b, opts) { | ||
var i, key; | ||
if (isUndefinedOrNull(a) || isUndefinedOrNull(b)) | ||
return false; | ||
// an identical 'prototype' property. | ||
if (a.prototype !== b.prototype) return false; | ||
//~~~I've managed to break Object.keys through screwy arguments passing. | ||
// Converting to array solves the problem. | ||
if (isArguments(a)) { | ||
if (!isArguments(b)) { | ||
return false; | ||
} | ||
a = pSlice.call(a); | ||
b = pSlice.call(b); | ||
return deepEqual(a, b, opts); | ||
} | ||
if (isBuffer(a)) { | ||
if (!isBuffer(b)) { | ||
return false; | ||
} | ||
if (a.length !== b.length) return false; | ||
for (i = 0; i < a.length; i++) { | ||
if (a[i] !== b[i]) return false; | ||
} | ||
return true; | ||
} | ||
try { | ||
var ka = objectKeys(a), | ||
kb = objectKeys(b); | ||
} catch (e) {//happens when one is a string literal and the other isn't | ||
return false; | ||
} | ||
// having the same number of owned properties (keys incorporates | ||
// hasOwnProperty) | ||
if (ka.length != kb.length) | ||
return false; | ||
//the same set of keys (although not necessarily the same order), | ||
ka.sort(); | ||
kb.sort(); | ||
//~~~cheap key test | ||
for (i = ka.length - 1; i >= 0; i--) { | ||
if (ka[i] != kb[i]) | ||
return false; | ||
} | ||
//equivalent values for every corresponding key, and | ||
//~~~possibly expensive deep test | ||
for (i = ka.length - 1; i >= 0; i--) { | ||
key = ka[i]; | ||
if (!deepEqual(a[key], b[key], opts)) return false; | ||
} | ||
return typeof a === typeof b; | ||
} | ||
/***/ }), | ||
/* 4 */ | ||
@@ -941,0 +964,0 @@ /***/ (function(module, exports) { |
@@ -1,2 +0,2 @@ | ||
/*! fast-json-patch, version: 2.0.7 */ | ||
var jsonpatch=function(a){function b(d){if(c[d])return c[d].exports;var e=c[d]={i:d,l:!1,exports:{}};return a[d].call(e.exports,e,e.exports,b),e.l=!0,e.exports}var c={};return b.m=a,b.c=c,b.i=function(a){return a},b.d=function(a,c,d){b.o(a,c)||Object.defineProperty(a,c,{configurable:!1,enumerable:!0,get:d})},b.n=function(a){var c=a&&a.__esModule?function(){return a['default']}:function(){return a};return b.d(c,'a',c),c},b.o=function(a,b){return Object.prototype.hasOwnProperty.call(a,b)},b.p='',b(b.s=3)}([function(a,b){function c(a,b){return i.call(a,b)}function d(a){if(Array.isArray(a)){for(var b=Array(a.length),d=0;d<b.length;d++)b[d]=''+d;return b}if(Object.keys)return Object.keys(a);var b=[];for(var e in a)c(a,e)&&b.push(e);return b}function e(a){return-1===a.indexOf('/')&&-1===a.indexOf('~')?a:a.replace(/~/g,'~0').replace(/\//g,'~1')}function f(a,b){var d;for(var g in a)if(c(a,g)){if(a[g]===b)return e(g)+'/';if('object'==typeof a[g]&&(d=f(a[g],b),''!=d))return e(g)+'/'+d}return''}function g(a){if(a===void 0)return!0;if(a)if(Array.isArray(a)){for(var b=0,c=a.length;b<c;b++)if(g(a[b]))return!0;}else if('object'==typeof a)for(var e=d(a),f=e.length,b=0;b<f;b++)if(g(a[e[b]]))return!0;return!1}var h=this&&this.__extends||function(a,c){function b(){this.constructor=a}for(var d in c)c.hasOwnProperty(d)&&(a[d]=c[d]);a.prototype=null===c?Object.create(c):(b.prototype=c.prototype,new b)},i=Object.prototype.hasOwnProperty;b.hasOwnProperty=c,b._objectKeys=d;b._deepClone=function(a){switch(typeof a){case'object':return JSON.parse(JSON.stringify(a));case'undefined':return null;default:return a;}},b.isInteger=function(a){for(var b,c=0,d=a.length;c<d;){if(b=a.charCodeAt(c),48<=b&&57>=b){c++;continue}return!1}return!0},b.escapePathComponent=e,b.unescapePathComponent=function(a){return a.replace(/~1/g,'/').replace(/~0/g,'~')},b._getPathRecursive=f,b.getPath=function(a,b){if(a===b)return'/';var c=f(a,b);if(''===c)throw new Error('Object not found in root');return'/'+c},b.hasUndefined=g;var j=function(a){function b(b,c,d,e,f){a.call(this,b),this.message=b,this.name=c,this.index=d,this.operation=e,this.tree=f}return h(b,a),b}(Error);b.PatchError=j},function(a,b,c){function d(a,b){if(''==b)return a;var c={op:'_get',path:b};return e(a,c),c.value}function e(a,c,e,f){if(void 0===e&&(e=!1),void 0===f&&(f=!0),e&&('function'==typeof e?e(c,0,a,c.path):g(c,0)),''===c.path){var h={newDocument:a};if('add'===c.op)return h.newDocument=c.value,h;if('replace'===c.op)return h.newDocument=c.value,h.removed=a,h;if('move'===c.op||'copy'===c.op)return h.newDocument=d(a,c.from),'move'===c.op&&(h.removed=a),h;if('test'===c.op){if(h.test=k(a,c.value),!1===h.test)throw new b.JsonPatchError('Test operation failed','TEST_OPERATION_FAILED',0,c,a);return h.newDocument=a,h}if('remove'===c.op)return h.removed=a,h.newDocument=null,h;if('_get'===c.op)return c.value=a,h;if(e)throw new b.JsonPatchError('Operation `op` property is not one of operations defined in RFC-6902','OPERATION_OP_INVALID',0,c,a);else return h}else{f||(a=l._deepClone(a));var i,j,o,p=c.path||'',q=p.split('/'),r=a,s=1,t=q.length;for(o='function'==typeof e?e:g;;){if(j=q[s],e&&void 0==i&&(void 0===r[j]?i=q.slice(0,s).join('/'):s==t-1&&(i=c.path),void 0!==i&&o(c,0,a,i)),s++,Array.isArray(r)){if('-'===j)j=r.length;else if(e&&!l.isInteger(j))throw new b.JsonPatchError('Expected an unsigned base-10 integer value, making the new referenced value the array element with the zero-based index','OPERATION_PATH_ILLEGAL_ARRAY_INDEX',0,c.path,c);else l.isInteger(j)&&(j=~~j);if(s>=t){if(e&&'add'===c.op&&j>r.length)throw new b.JsonPatchError('The specified index MUST NOT be greater than the number of elements in the array','OPERATION_VALUE_OUT_OF_BOUNDS',0,c.path,c);var h=n[c.op].call(c,r,j,a);if(!1===h.test)throw new b.JsonPatchError('Test operation failed','TEST_OPERATION_FAILED',0,c,a);return h}}else if(j&&-1!=j.indexOf('~')&&(j=l.unescapePathComponent(j)),s>=t){var h=m[c.op].call(c,r,j,a);if(!1===h.test)throw new b.JsonPatchError('Test operation failed','TEST_OPERATION_FAILED',0,c,a);return h}r=r[j]}}}function f(a,c,d,f){if(void 0===f&&(f=!0),d&&!Array.isArray(c))throw new b.JsonPatchError('Patch sequence must be an array','SEQUENCE_NOT_AN_ARRAY');f||(a=l._deepClone(a));for(var g=Array(c.length),h=0,i=c.length;h<i;h++)g[h]=e(a,c[h],d),a=g[h].newDocument;return g.newDocument=a,g}function g(a,c,d,e){if('object'!=typeof a||null===a||Array.isArray(a))throw new b.JsonPatchError('Operation is not an object','OPERATION_NOT_AN_OBJECT',c,a,d);else if(!m[a.op])throw new b.JsonPatchError('Operation `op` property is not one of operations defined in RFC-6902','OPERATION_OP_INVALID',c,a,d);else if('string'!=typeof a.path)throw new b.JsonPatchError('Operation `path` property is not a string','OPERATION_PATH_INVALID',c,a,d);else if(0!==a.path.indexOf('/')&&0<a.path.length)throw new b.JsonPatchError('Operation `path` property must start with "/"','OPERATION_PATH_INVALID',c,a,d);else if(('move'===a.op||'copy'===a.op)&&'string'!=typeof a.from)throw new b.JsonPatchError('Operation `from` property is not present (applicable in `move` and `copy` operations)','OPERATION_FROM_REQUIRED',c,a,d);else if(('add'===a.op||'replace'===a.op||'test'===a.op)&&a.value===void 0)throw new b.JsonPatchError('Operation `value` property is not present (applicable in `add`, `replace` and `test` operations)','OPERATION_VALUE_REQUIRED',c,a,d);else if(('add'===a.op||'replace'===a.op||'test'===a.op)&&l.hasUndefined(a.value))throw new b.JsonPatchError('Operation `value` property is not present (applicable in `add`, `replace` and `test` operations)','OPERATION_VALUE_CANNOT_CONTAIN_UNDEFINED',c,a,d);else if(d)if('add'==a.op){var f=a.path.split('/').length,g=e.split('/').length;if(f!==g+1&&f!==g)throw new b.JsonPatchError('Cannot perform an `add` operation at the desired path','OPERATION_PATH_CANNOT_ADD',c,a,d)}else if('replace'===a.op||'remove'===a.op||'_get'===a.op){if(a.path!==e)throw new b.JsonPatchError('Cannot perform the operation at a path that does not exist','OPERATION_PATH_UNRESOLVABLE',c,a,d);}else if('move'===a.op||'copy'===a.op){var i={op:'_get',path:a.from,value:void 0},j=h([i],d);if(j&&'OPERATION_PATH_UNRESOLVABLE'===j.name)throw new b.JsonPatchError('Cannot perform the operation from a path that does not exist','OPERATION_FROM_UNRESOLVABLE',c,a,d)}}function h(a,c,d){try{if(!Array.isArray(a))throw new b.JsonPatchError('Patch sequence must be an array','SEQUENCE_NOT_AN_ARRAY');if(c)f(l._deepClone(c),l._deepClone(a),d||!0);else{d=d||g;for(var e=0;e<a.length;e++)d(a[e],e,c,void 0)}}catch(a){if(a instanceof b.JsonPatchError)return a;throw a}}var i={strict:!0},j=c(2),k=function(c,a){return j(c,a,i)},l=c(0);b.JsonPatchError=l.PatchError,b.deepClone=l._deepClone;var m={add:function(a,b,c){return a[b]=this.value,{newDocument:c}},remove:function(a,b,c){var d=a[b];return delete a[b],{newDocument:c,removed:d}},replace:function(a,b,c){var d=a[b];return a[b]=this.value,{newDocument:c,removed:d}},move:function(a,b,c){var f=d(c,this.path);f&&(f=l._deepClone(f));var g=e(c,{op:'remove',path:this.from}).removed;return e(c,{op:'add',path:this.path,value:g}),{newDocument:c,removed:f}},copy:function(a,b,c){var f=d(c,this.from);return e(c,{op:'add',path:this.path,value:l._deepClone(f)}),{newDocument:c}},test:function(a,b,c){return{newDocument:c,test:k(a[b],this.value)}},_get:function(a,b,c){return this.value=a[b],{newDocument:c}}},n={add:function(a,b,c){return l.isInteger(b)?a.splice(b,0,this.value):a[b]=this.value,{newDocument:c,index:b}},remove:function(a,b,c){var d=a.splice(b,1);return{newDocument:c,removed:d[0]}},replace:function(a,b,c){var d=a[b];return a[b]=this.value,{newDocument:c,removed:d}},move:m.move,copy:m.copy,test:m.test,_get:m._get};b.getValueByPointer=d,b.applyOperation=e,b.applyPatch=f,b.applyReducer=function(a,c){var d=e(a,c);if(!1===d.test)throw new b.JsonPatchError('Test operation failed','TEST_OPERATION_FAILED',0,c,a);return d.newDocument},b.validator=g,b.validate=h},function(a,b,c){function d(a){return null===a||a===void 0}function e(a){return a&&'object'==typeof a&&'number'==typeof a.length&&('function'!=typeof a.copy||'function'!=typeof a.slice?!1:0<a.length&&'number'!=typeof a[0]?!1:!0)}function f(c,f,l){var m,i;if(d(c)||d(f))return!1;if(c.prototype!==f.prototype)return!1;if(j(c))return!!j(f)&&(c=g.call(c),f=g.call(f),k(c,f,l));if(e(c)){if(!e(f))return!1;if(c.length!==f.length)return!1;for(m=0;m<c.length;m++)if(c[m]!==f[m])return!1;return!0}try{var n=h(c),o=h(f)}catch(a){return!1}if(n.length!=o.length)return!1;for(n.sort(),o.sort(),m=n.length-1;0<=m;m--)if(n[m]!=o[m])return!1;for(m=n.length-1;0<=m;m--)if(i=n[m],!k(c[i],f[i],l))return!1;return typeof c==typeof f}var g=Array.prototype.slice,h=c(5),j=c(4),k=a.exports=function(a,b,c){return c||(c={}),a===b||(a instanceof Date&&b instanceof Date?a.getTime()===b.getTime():a&&b&&('object'==typeof a||'object'==typeof b)?f(a,b,c):c.strict?a===b:a==b)}},function(a,b,c){function d(a){return o.get(a)}function e(a,b){return a.observers.get(b)}function f(a,b){a.observers.delete(b.callback)}function g(a){var b=o.get(a.object);h(b.value,a.object,a.patches,''),a.patches.length&&l.applyPatch(b.value,a.patches);var c=a.patches;return 0<c.length&&(a.patches=[],a.callback&&a.callback(c)),c}function h(a,b,c,d){if(b!==a){'function'==typeof b.toJSON&&(b=b.toJSON());for(var e=k._objectKeys(b),f=k._objectKeys(a),g=!1,i=!1,j=f.length-1;0<=j;j--){var l=f[j],m=a[l];if(k.hasOwnProperty(b,l)&&(void 0!==b[l]||void 0===m||!1!==Array.isArray(b))){var n=b[l];'object'==typeof m&&null!=m&&'object'==typeof n&&null!=n?h(m,n,c,d+'/'+k.escapePathComponent(l)):m!==n&&(g=!0,c.push({op:'replace',path:d+'/'+k.escapePathComponent(l),value:k._deepClone(n)}))}else c.push({op:'remove',path:d+'/'+k.escapePathComponent(l)}),i=!0}if(i||e.length!=f.length)for(var l,j=0;j<e.length;j++)l=e[j],k.hasOwnProperty(a,l)||void 0===b[l]||c.push({op:'add',path:d+'/'+k.escapePathComponent(l),value:k._deepClone(b[l])})}}var i={strict:!0},j=c(2),k=c(0),l=c(1),m=c(1);b.applyOperation=m.applyOperation,b.applyPatch=m.applyPatch,b.applyReducer=m.applyReducer,b.getValueByPointer=m.getValueByPointer,b.validate=m.validate,b.validator=m.validator;var n=c(0);b.JsonPatchError=n.PatchError,b.deepClone=n._deepClone,b.escapePathComponent=n.escapePathComponent,b.unescapePathComponent=n.unescapePathComponent;var o=new WeakMap,p=function(){return function(a){this.observers=new Map,this.obj=a}}(),q=function(){return function(a,b){this.callback=a,this.observer=b}}();b.unobserve=function(a,b){b.unobserve()},b.observe=function(a,b){var c,h=d(a);if(!h)h=new p(a),o.set(a,h);else{var i=e(h,b);c=i&&i.observer}if(c)return c;if(c={},h.value=k._deepClone(a),b){c.callback=b,c.next=null;var j=function(){g(c)},l=function(){clearTimeout(c.next),c.next=setTimeout(j)};'undefined'!=typeof window&&(window.addEventListener?(window.addEventListener('mouseup',l),window.addEventListener('keyup',l),window.addEventListener('mousedown',l),window.addEventListener('keydown',l),window.addEventListener('change',l)):(document.documentElement.attachEvent('onmouseup',l),document.documentElement.attachEvent('onkeyup',l),document.documentElement.attachEvent('onmousedown',l),document.documentElement.attachEvent('onkeydown',l),document.documentElement.attachEvent('onchange',l)))}return c.patches=[],c.object=a,c.unobserve=function(){g(c),clearTimeout(c.next),f(h,c),'undefined'!=typeof window&&(window.removeEventListener?(window.removeEventListener('mouseup',l),window.removeEventListener('keyup',l),window.removeEventListener('mousedown',l),window.removeEventListener('keydown',l)):(document.documentElement.detachEvent('onmouseup',l),document.documentElement.detachEvent('onkeyup',l),document.documentElement.detachEvent('onmousedown',l),document.documentElement.detachEvent('onkeydown',l)))},h.observers.set(b,new q(b,c)),c},b.generate=g,b.compare=function(a,b){var c=[];return h(a,b,c,''),c}},function(a,b){function c(a){return'[object Arguments]'==Object.prototype.toString.call(a)}function d(a){return a&&'object'==typeof a&&'number'==typeof a.length&&Object.prototype.hasOwnProperty.call(a,'callee')&&!Object.prototype.propertyIsEnumerable.call(a,'callee')||!1}var e='[object Arguments]'==function(){return Object.prototype.toString.call(arguments)}();b=a.exports=e?c:d,b.supported=c;b.unsupported=d},function(a,b){function c(a){var b=[];for(var c in a)b.push(c);return b}b=a.exports='function'==typeof Object.keys?Object.keys:c,b.shim=c}]); | ||
/*! fast-json-patch, version: 2.1.0 */ | ||
var jsonpatch=function(a){function b(d){if(c[d])return c[d].exports;var e=c[d]={i:d,l:!1,exports:{}};return a[d].call(e.exports,e,e.exports,b),e.l=!0,e.exports}var c={};return b.m=a,b.c=c,b.i=function(a){return a},b.d=function(a,c,d){b.o(a,c)||Object.defineProperty(a,c,{configurable:!1,enumerable:!0,get:d})},b.n=function(a){var c=a&&a.__esModule?function(){return a['default']}:function(){return a};return b.d(c,'a',c),c},b.o=function(a,b){return Object.prototype.hasOwnProperty.call(a,b)},b.p='',b(b.s=2)}([function(a,b){function c(a,b){return j.call(a,b)}function d(a){if(Array.isArray(a)){for(var b=Array(a.length),d=0;d<b.length;d++)b[d]=''+d;return b}if(Object.keys)return Object.keys(a);var b=[];for(var e in a)c(a,e)&&b.push(e);return b}function e(a){return-1===a.indexOf('/')&&-1===a.indexOf('~')?a:a.replace(/~/g,'~0').replace(/\//g,'~1')}function f(a,b){var d;for(var g in a)if(c(a,g)){if(a[g]===b)return e(g)+'/';if('object'==typeof a[g]&&(d=f(a[g],b),''!=d))return e(g)+'/'+d}return''}function g(a){if(a===void 0)return!0;if(a)if(Array.isArray(a)){for(var b=0,c=a.length;b<c;b++)if(g(a[b]))return!0;}else if('object'==typeof a)for(var e=d(a),f=e.length,b=0;b<f;b++)if(g(a[e[b]]))return!0;return!1}function h(a,b){var c=[a];for(var d in b){var e='object'==typeof b[d]?JSON.stringify(b[d],null,2):b[d];'undefined'!=typeof e&&c.push(d+': '+e)}return c.join('\n')}var i=this&&this.__extends||function(a,c){function b(){this.constructor=a}for(var d in c)c.hasOwnProperty(d)&&(a[d]=c[d]);a.prototype=null===c?Object.create(c):(b.prototype=c.prototype,new b)},j=Object.prototype.hasOwnProperty;b.hasOwnProperty=c,b._objectKeys=d;b._deepClone=function(a){switch(typeof a){case'object':return JSON.parse(JSON.stringify(a));case'undefined':return null;default:return a;}},b.isInteger=function(a){for(var b,c=0,d=a.length;c<d;){if(b=a.charCodeAt(c),48<=b&&57>=b){c++;continue}return!1}return!0},b.escapePathComponent=e,b.unescapePathComponent=function(a){return a.replace(/~1/g,'/').replace(/~0/g,'~')},b._getPathRecursive=f,b.getPath=function(a,b){if(a===b)return'/';var c=f(a,b);if(''===c)throw new Error('Object not found in root');return'/'+c},b.hasUndefined=g;var k=function(a){function b(b,c,d,e,f){a.call(this,h(b,{name:c,index:d,operation:e,tree:f})),this.name=c,this.index=d,this.operation=e,this.tree=f,this.message=h(b,{name:c,index:d,operation:e,tree:f})}return i(b,a),b}(Error);b.PatchError=k},function(a,b,c){function d(a,b){if(''==b)return a;var c={op:'_get',path:b};return e(a,c),c.value}function e(a,c,e,f,h,i){if(void 0===e&&(e=!1),void 0===f&&(f=!0),void 0===h&&(h=!0),void 0===i&&(i=0),e&&('function'==typeof e?e(c,0,a,c.path):g(c,0)),''===c.path){var j={newDocument:a};if('add'===c.op)return j.newDocument=c.value,j;if('replace'===c.op)return j.newDocument=c.value,j.removed=a,j;if('move'===c.op||'copy'===c.op)return j.newDocument=d(a,c.from),'move'===c.op&&(j.removed=a),j;if('test'===c.op){if(j.test=k(a,c.value),!1===j.test)throw new b.JsonPatchError('Test operation failed','TEST_OPERATION_FAILED',i,c,a);return j.newDocument=a,j}if('remove'===c.op)return j.removed=a,j.newDocument=null,j;if('_get'===c.op)return c.value=a,j;if(e)throw new b.JsonPatchError('Operation `op` property is not one of operations defined in RFC-6902','OPERATION_OP_INVALID',i,c,a);else return j}else{f||(a=l._deepClone(a));var o,p,q,r=c.path||'',s=r.split('/'),u=a,v=1,t=s.length;for(q='function'==typeof e?e:g;;){if(p=s[v],h&&'__proto__'==p)throw new TypeError('JSON-Patch: modifying `__proto__` prop is banned for security reasons, if this was on purpose, please set `banPrototypeModifications` flag false and pass it to this function. More info in fast-json-patch README');if(e&&void 0==o&&(void 0===u[p]?o=s.slice(0,v).join('/'):v==t-1&&(o=c.path),void 0!==o&&q(c,0,a,o)),v++,Array.isArray(u)){if('-'===p)p=u.length;else if(e&&!l.isInteger(p))throw new b.JsonPatchError('Expected an unsigned base-10 integer value, making the new referenced value the array element with the zero-based index','OPERATION_PATH_ILLEGAL_ARRAY_INDEX',i,c,a);else l.isInteger(p)&&(p=~~p);if(v>=t){if(e&&'add'===c.op&&p>u.length)throw new b.JsonPatchError('The specified index MUST NOT be greater than the number of elements in the array','OPERATION_VALUE_OUT_OF_BOUNDS',i,c,a);var j=n[c.op].call(c,u,p,a);if(!1===j.test)throw new b.JsonPatchError('Test operation failed','TEST_OPERATION_FAILED',i,c,a);return j}}else if(p&&-1!=p.indexOf('~')&&(p=l.unescapePathComponent(p)),v>=t){var j=m[c.op].call(c,u,p,a);if(!1===j.test)throw new b.JsonPatchError('Test operation failed','TEST_OPERATION_FAILED',i,c,a);return j}u=u[p]}}}function f(a,c,d,f,g){if(void 0===f&&(f=!0),void 0===g&&(g=!0),d&&!Array.isArray(c))throw new b.JsonPatchError('Patch sequence must be an array','SEQUENCE_NOT_AN_ARRAY');f||(a=l._deepClone(a));for(var h=Array(c.length),j=0,i=c.length;j<i;j++)h[j]=e(a,c[j],d,!0,g,j),a=h[j].newDocument;return h.newDocument=a,h}function g(a,c,d,e){if('object'!=typeof a||null===a||Array.isArray(a))throw new b.JsonPatchError('Operation is not an object','OPERATION_NOT_AN_OBJECT',c,a,d);else if(!m[a.op])throw new b.JsonPatchError('Operation `op` property is not one of operations defined in RFC-6902','OPERATION_OP_INVALID',c,a,d);else if('string'!=typeof a.path)throw new b.JsonPatchError('Operation `path` property is not a string','OPERATION_PATH_INVALID',c,a,d);else if(0!==a.path.indexOf('/')&&0<a.path.length)throw new b.JsonPatchError('Operation `path` property must start with "/"','OPERATION_PATH_INVALID',c,a,d);else if(('move'===a.op||'copy'===a.op)&&'string'!=typeof a.from)throw new b.JsonPatchError('Operation `from` property is not present (applicable in `move` and `copy` operations)','OPERATION_FROM_REQUIRED',c,a,d);else if(('add'===a.op||'replace'===a.op||'test'===a.op)&&a.value===void 0)throw new b.JsonPatchError('Operation `value` property is not present (applicable in `add`, `replace` and `test` operations)','OPERATION_VALUE_REQUIRED',c,a,d);else if(('add'===a.op||'replace'===a.op||'test'===a.op)&&l.hasUndefined(a.value))throw new b.JsonPatchError('Operation `value` property is not present (applicable in `add`, `replace` and `test` operations)','OPERATION_VALUE_CANNOT_CONTAIN_UNDEFINED',c,a,d);else if(d)if('add'==a.op){var f=a.path.split('/').length,g=e.split('/').length;if(f!==g+1&&f!==g)throw new b.JsonPatchError('Cannot perform an `add` operation at the desired path','OPERATION_PATH_CANNOT_ADD',c,a,d)}else if('replace'===a.op||'remove'===a.op||'_get'===a.op){if(a.path!==e)throw new b.JsonPatchError('Cannot perform the operation at a path that does not exist','OPERATION_PATH_UNRESOLVABLE',c,a,d);}else if('move'===a.op||'copy'===a.op){var i={op:'_get',path:a.from,value:void 0},j=h([i],d);if(j&&'OPERATION_PATH_UNRESOLVABLE'===j.name)throw new b.JsonPatchError('Cannot perform the operation from a path that does not exist','OPERATION_FROM_UNRESOLVABLE',c,a,d)}}function h(a,c,d){try{if(!Array.isArray(a))throw new b.JsonPatchError('Patch sequence must be an array','SEQUENCE_NOT_AN_ARRAY');if(c)f(l._deepClone(c),l._deepClone(a),d||!0);else{d=d||g;for(var e=0;e<a.length;e++)d(a[e],e,c,void 0)}}catch(a){if(a instanceof b.JsonPatchError)return a;throw a}}var i={strict:!0},j=c(3),k=function(c,a){return j(c,a,i)},l=c(0);b.JsonPatchError=l.PatchError,b.deepClone=l._deepClone;var m={add:function(a,b,c){return a[b]=this.value,{newDocument:c}},remove:function(a,b,c){var d=a[b];return delete a[b],{newDocument:c,removed:d}},replace:function(a,b,c){var d=a[b];return a[b]=this.value,{newDocument:c,removed:d}},move:function(a,b,c){var f=d(c,this.path);f&&(f=l._deepClone(f));var g=e(c,{op:'remove',path:this.from}).removed;return e(c,{op:'add',path:this.path,value:g}),{newDocument:c,removed:f}},copy:function(a,b,c){var f=d(c,this.from);return e(c,{op:'add',path:this.path,value:l._deepClone(f)}),{newDocument:c}},test:function(a,b,c){return{newDocument:c,test:k(a[b],this.value)}},_get:function(a,b,c){return this.value=a[b],{newDocument:c}}},n={add:function(a,b,c){return l.isInteger(b)?a.splice(b,0,this.value):a[b]=this.value,{newDocument:c,index:b}},remove:function(a,b,c){var d=a.splice(b,1);return{newDocument:c,removed:d[0]}},replace:function(a,b,c){var d=a[b];return a[b]=this.value,{newDocument:c,removed:d}},move:m.move,copy:m.copy,test:m.test,_get:m._get};b.getValueByPointer=d,b.applyOperation=e,b.applyPatch=f,b.applyReducer=function(a,c,d){var f=e(a,c);if(!1===f.test)throw new b.JsonPatchError('Test operation failed','TEST_OPERATION_FAILED',d,c,a);return f.newDocument},b.validator=g,b.validate=h},function(a,b,c){function d(a){return m.get(a)}function e(a,b){return a.observers.get(b)}function f(a,b){a.observers.delete(b.callback)}function g(a){var b=m.get(a.object);h(b.value,a.object,a.patches,''),a.patches.length&&j.applyPatch(b.value,a.patches);var c=a.patches;return 0<c.length&&(a.patches=[],a.callback&&a.callback(c)),c}function h(a,b,c,d){if(b!==a){'function'==typeof b.toJSON&&(b=b.toJSON());for(var e=i._objectKeys(b),f=i._objectKeys(a),g=!1,j=!1,k=f.length-1;0<=k;k--){var l=f[k],m=a[l];if(i.hasOwnProperty(b,l)&&(void 0!==b[l]||void 0===m||!1!==Array.isArray(b))){var n=b[l];'object'==typeof m&&null!=m&&'object'==typeof n&&null!=n?h(m,n,c,d+'/'+i.escapePathComponent(l)):m!==n&&(g=!0,c.push({op:'replace',path:d+'/'+i.escapePathComponent(l),value:i._deepClone(n)}))}else Array.isArray(a)===Array.isArray(b)?(c.push({op:'remove',path:d+'/'+i.escapePathComponent(l)}),j=!0):(c.push({op:'replace',path:d,value:b}),g=!0)}if(j||e.length!=f.length)for(var l,k=0;k<e.length;k++)l=e[k],i.hasOwnProperty(a,l)||void 0===b[l]||c.push({op:'add',path:d+'/'+i.escapePathComponent(l),value:i._deepClone(b[l])})}}var i=c(0),j=c(1),k=c(1);b.applyOperation=k.applyOperation,b.applyPatch=k.applyPatch,b.applyReducer=k.applyReducer,b.getValueByPointer=k.getValueByPointer,b.validate=k.validate,b.validator=k.validator;var l=c(0);b.JsonPatchError=l.PatchError,b.deepClone=l._deepClone,b.escapePathComponent=l.escapePathComponent,b.unescapePathComponent=l.unescapePathComponent;var m=new WeakMap,n=function(){return function(a){this.observers=new Map,this.obj=a}}(),o=function(){return function(a,b){this.callback=a,this.observer=b}}();b.unobserve=function(a,b){b.unobserve()},b.observe=function(a,b){var c,h=d(a);if(!h)h=new n(a),m.set(a,h);else{var j=e(h,b);c=j&&j.observer}if(c)return c;if(c={},h.value=i._deepClone(a),b){c.callback=b,c.next=null;var k=function(){g(c)},l=function(){clearTimeout(c.next),c.next=setTimeout(k)};'undefined'!=typeof window&&(window.addEventListener?(window.addEventListener('mouseup',l),window.addEventListener('keyup',l),window.addEventListener('mousedown',l),window.addEventListener('keydown',l),window.addEventListener('change',l)):(document.documentElement.attachEvent('onmouseup',l),document.documentElement.attachEvent('onkeyup',l),document.documentElement.attachEvent('onmousedown',l),document.documentElement.attachEvent('onkeydown',l),document.documentElement.attachEvent('onchange',l)))}return c.patches=[],c.object=a,c.unobserve=function(){g(c),clearTimeout(c.next),f(h,c),'undefined'!=typeof window&&(window.removeEventListener?(window.removeEventListener('mouseup',l),window.removeEventListener('keyup',l),window.removeEventListener('mousedown',l),window.removeEventListener('keydown',l)):(document.documentElement.detachEvent('onmouseup',l),document.documentElement.detachEvent('onkeyup',l),document.documentElement.detachEvent('onmousedown',l),document.documentElement.detachEvent('onkeydown',l)))},h.observers.set(b,new o(b,c)),c},b.generate=g,b.compare=function(a,b){var c=[];return h(a,b,c,''),c}},function(a,b,c){function d(a){return null===a||a===void 0}function e(a){return a&&'object'==typeof a&&'number'==typeof a.length&&('function'!=typeof a.copy||'function'!=typeof a.slice?!1:0<a.length&&'number'!=typeof a[0]?!1:!0)}function f(c,f,l){var m,i;if(d(c)||d(f))return!1;if(c.prototype!==f.prototype)return!1;if(j(c))return!!j(f)&&(c=g.call(c),f=g.call(f),k(c,f,l));if(e(c)){if(!e(f))return!1;if(c.length!==f.length)return!1;for(m=0;m<c.length;m++)if(c[m]!==f[m])return!1;return!0}try{var n=h(c),o=h(f)}catch(a){return!1}if(n.length!=o.length)return!1;for(n.sort(),o.sort(),m=n.length-1;0<=m;m--)if(n[m]!=o[m])return!1;for(m=n.length-1;0<=m;m--)if(i=n[m],!k(c[i],f[i],l))return!1;return typeof c==typeof f}var g=Array.prototype.slice,h=c(5),j=c(4),k=a.exports=function(a,b,c){return c||(c={}),a===b||(a instanceof Date&&b instanceof Date?a.getTime()===b.getTime():a&&b&&('object'==typeof a||'object'==typeof b)?f(a,b,c):c.strict?a===b:a==b)}},function(a,b){function c(a){return'[object Arguments]'==Object.prototype.toString.call(a)}function d(a){return a&&'object'==typeof a&&'number'==typeof a.length&&Object.prototype.hasOwnProperty.call(a,'callee')&&!Object.prototype.propertyIsEnumerable.call(a,'callee')||!1}var e='[object Arguments]'==function(){return Object.prototype.toString.call(arguments)}();b=a.exports=e?c:d,b.supported=c;b.unsupported=d},function(a,b){function c(a){var b=[];for(var c in a)b.push(c);return b}b=a.exports='function'==typeof Object.keys?Object.keys:c,b.shim=c}]); |
@@ -72,5 +72,6 @@ import { PatchError, _deepClone } from './helpers'; | ||
* @param mutateDocument Whether to mutate the original document or clone it before applying | ||
* @param banPrototypeModifications Whether to ban modifications to `__proto__`, defaults to `true`. | ||
* @return `{newDocument, result}` after the operation | ||
*/ | ||
export declare function applyOperation<T>(document: T, operation: Operation, validateOperation?: boolean | Validator<T>, mutateDocument?: boolean): OperationResult<T>; | ||
export declare function applyOperation<T>(document: T, operation: Operation, validateOperation?: boolean | Validator<T>, mutateDocument?: boolean, banPrototypeModifications?: boolean, index?: number): OperationResult<T>; | ||
/** | ||
@@ -87,5 +88,6 @@ * Apply a full JSON Patch array on a JSON document. | ||
* @param mutateDocument Whether to mutate the original document or clone it before applying | ||
* @param banPrototypeModifications Whether to ban modifications to `__proto__`, defaults to `true`. | ||
* @return An array of `{newDocument, result}` after the patch | ||
*/ | ||
export declare function applyPatch<T>(document: T, patch: Operation[], validateOperation?: boolean | Validator<T>, mutateDocument?: boolean): PatchResult<T>; | ||
export declare function applyPatch<T>(document: T, patch: Operation[], validateOperation?: boolean | Validator<T>, mutateDocument?: boolean, banPrototypeModifications?: boolean): PatchResult<T>; | ||
/** | ||
@@ -100,3 +102,3 @@ * Apply a single JSON Patch Operation on a JSON document. | ||
*/ | ||
export declare function applyReducer<T>(document: T, operation: Operation): T; | ||
export declare function applyReducer<T>(document: T, operation: Operation, index: number): T; | ||
/** | ||
@@ -103,0 +105,0 @@ * Validates a single operation. Called from `jsonpatch.validate`. Throws `JsonPatchError` in case of an error. |
@@ -111,7 +111,10 @@ var equalsOptions = { strict: true }; | ||
* @param mutateDocument Whether to mutate the original document or clone it before applying | ||
* @param banPrototypeModifications Whether to ban modifications to `__proto__`, defaults to `true`. | ||
* @return `{newDocument, result}` after the operation | ||
*/ | ||
function applyOperation(document, operation, validateOperation, mutateDocument) { | ||
function applyOperation(document, operation, validateOperation, mutateDocument, banPrototypeModifications, index) { | ||
if (validateOperation === void 0) { validateOperation = false; } | ||
if (mutateDocument === void 0) { mutateDocument = true; } | ||
if (banPrototypeModifications === void 0) { banPrototypeModifications = true; } | ||
if (index === void 0) { index = 0; } | ||
if (validateOperation) { | ||
@@ -147,3 +150,3 @@ if (typeof validateOperation == 'function') { | ||
if (returnValue.test === false) { | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', 0, operation, document); | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', index, operation, document); | ||
} | ||
@@ -164,3 +167,3 @@ returnValue.newDocument = document; | ||
if (validateOperation) { | ||
throw new exports.JsonPatchError('Operation `op` property is not one of operations defined in RFC-6902', 'OPERATION_OP_INVALID', 0, operation, document); | ||
throw new exports.JsonPatchError('Operation `op` property is not one of operations defined in RFC-6902', 'OPERATION_OP_INVALID', index, operation, document); | ||
} | ||
@@ -192,2 +195,5 @@ else { | ||
key = keys[t]; | ||
if (banPrototypeModifications && key == '__proto__') { | ||
throw new TypeError('JSON-Patch: modifying `__proto__` prop is banned for security reasons, if this was on purpose, please set `banPrototypeModifications` flag false and pass it to this function. More info in fast-json-patch README'); | ||
} | ||
if (validateOperation) { | ||
@@ -213,3 +219,3 @@ if (existingPathFragment === undefined) { | ||
if (validateOperation && !helpers_1.isInteger(key)) { | ||
throw new exports.JsonPatchError("Expected an unsigned base-10 integer value, making the new referenced value the array element with the zero-based index", "OPERATION_PATH_ILLEGAL_ARRAY_INDEX", 0, operation.path, operation); | ||
throw new exports.JsonPatchError("Expected an unsigned base-10 integer value, making the new referenced value the array element with the zero-based index", "OPERATION_PATH_ILLEGAL_ARRAY_INDEX", index, operation, document); | ||
} // only parse key when it's an integer for `arr.prop` to work | ||
@@ -222,7 +228,7 @@ else if (helpers_1.isInteger(key)) { | ||
if (validateOperation && operation.op === "add" && key > obj.length) { | ||
throw new exports.JsonPatchError("The specified index MUST NOT be greater than the number of elements in the array", "OPERATION_VALUE_OUT_OF_BOUNDS", 0, operation.path, operation); | ||
throw new exports.JsonPatchError("The specified index MUST NOT be greater than the number of elements in the array", "OPERATION_VALUE_OUT_OF_BOUNDS", index, operation, document); | ||
} | ||
var returnValue = arrOps[operation.op].call(operation, obj, key, document); // Apply patch | ||
if (returnValue.test === false) { | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', 0, operation, document); | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', index, operation, document); | ||
} | ||
@@ -239,3 +245,3 @@ return returnValue; | ||
if (returnValue.test === false) { | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', 0, operation, document); | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', index, operation, document); | ||
} | ||
@@ -261,6 +267,8 @@ return returnValue; | ||
* @param mutateDocument Whether to mutate the original document or clone it before applying | ||
* @param banPrototypeModifications Whether to ban modifications to `__proto__`, defaults to `true`. | ||
* @return An array of `{newDocument, result}` after the patch | ||
*/ | ||
function applyPatch(document, patch, validateOperation, mutateDocument) { | ||
function applyPatch(document, patch, validateOperation, mutateDocument, banPrototypeModifications) { | ||
if (mutateDocument === void 0) { mutateDocument = true; } | ||
if (banPrototypeModifications === void 0) { banPrototypeModifications = true; } | ||
if (validateOperation) { | ||
@@ -276,3 +284,4 @@ if (!Array.isArray(patch)) { | ||
for (var i = 0, length_1 = patch.length; i < length_1; i++) { | ||
results[i] = applyOperation(document, patch[i], validateOperation); | ||
// we don't need to pass mutateDocument argument because if it was true, we already deep cloned the object, we'll just pass `true` | ||
results[i] = applyOperation(document, patch[i], validateOperation, true, banPrototypeModifications, i); | ||
document = results[i].newDocument; // in case root was replaced | ||
@@ -293,6 +302,6 @@ } | ||
*/ | ||
function applyReducer(document, operation) { | ||
function applyReducer(document, operation, index) { | ||
var operationResult = applyOperation(document, operation); | ||
if (operationResult.test === false) { | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', 0, operation, document); | ||
throw new exports.JsonPatchError("Test operation failed", 'TEST_OPERATION_FAILED', index, operation, document); | ||
} | ||
@@ -299,0 +308,0 @@ return operationResult.newDocument; |
@@ -1,6 +0,6 @@ | ||
var equalsOptions = { strict: true }; | ||
var _equals = require('deep-equal'); | ||
var areEquals = function (a, b) { | ||
return _equals(a, b, equalsOptions); | ||
}; | ||
/*! | ||
* https://github.com/Starcounter-Jack/JSON-Patch | ||
* (c) 2017 Joachim Wester | ||
* MIT license | ||
*/ | ||
var helpers_1 = require('./helpers'); | ||
@@ -172,6 +172,10 @@ var core_1 = require('./core'); | ||
} | ||
else { | ||
else if (Array.isArray(mirror) === Array.isArray(obj)) { | ||
patches.push({ op: "remove", path: path + "/" + helpers_1.escapePathComponent(key) }); | ||
deleted = true; // property has been deleted | ||
} | ||
else { | ||
patches.push({ op: "replace", path: path, value: obj }); | ||
changed = true; | ||
} | ||
} | ||
@@ -178,0 +182,0 @@ if (!deleted && newKeys.length == oldKeys.length) { |
@@ -0,1 +1,6 @@ | ||
/*! | ||
* https://github.com/Starcounter-Jack/JSON-Patch | ||
* (c) 2017 Joachim Wester | ||
* MIT license | ||
*/ | ||
export declare function hasOwnProperty(obj: any, key: any): any; | ||
@@ -31,3 +36,2 @@ export declare function _objectKeys(obj: any): any[]; | ||
export declare class PatchError extends Error { | ||
message: string; | ||
name: JsonPatchErrorName; | ||
@@ -34,0 +38,0 @@ index: number; |
@@ -0,1 +1,6 @@ | ||
/*! | ||
* https://github.com/Starcounter-Jack/JSON-Patch | ||
* (c) 2017 Joachim Wester | ||
* MIT license | ||
*/ | ||
var __extends = (this && this.__extends) || function (d, b) { | ||
@@ -6,7 +11,2 @@ for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; | ||
}; | ||
/*! | ||
* https://github.com/Starcounter-Jack/JSON-Patch | ||
* (c) 2017 Joachim Wester | ||
* MIT license | ||
*/ | ||
var _hasOwnProperty = Object.prototype.hasOwnProperty; | ||
@@ -148,7 +148,16 @@ function hasOwnProperty(obj, key) { | ||
exports.hasUndefined = hasUndefined; | ||
function patchErrorMessageFormatter(message, args) { | ||
var messageParts = [message]; | ||
for (var key in args) { | ||
var value = typeof args[key] === 'object' ? JSON.stringify(args[key], null, 2) : args[key]; // pretty print | ||
if (typeof value !== 'undefined') { | ||
messageParts.push(key + ": " + value); | ||
} | ||
} | ||
return messageParts.join('\n'); | ||
} | ||
var PatchError = (function (_super) { | ||
__extends(PatchError, _super); | ||
function PatchError(message, name, index, operation, tree) { | ||
_super.call(this, message); | ||
this.message = message; | ||
_super.call(this, patchErrorMessageFormatter(message, { name: name, index: index, operation: operation, tree: tree })); | ||
this.name = name; | ||
@@ -158,2 +167,3 @@ this.index = index; | ||
this.tree = tree; | ||
this.message = patchErrorMessageFormatter(message, { name: name, index: index, operation: operation, tree: tree }); | ||
} | ||
@@ -160,0 +170,0 @@ return PatchError; |
{ | ||
"name": "fast-json-patch", | ||
"version": "2.0.7", | ||
"version": "2.1.0", | ||
"description": "Fast implementation of JSON-Patch (RFC-6902) with duplex (observe changes) capabilities", | ||
@@ -34,8 +34,12 @@ "homepage": "https://github.com/Starcounter-Jack/JSON-Patch", | ||
"chalk": "^1.1.3", | ||
"jasmine": "^2.5.1", | ||
"grunt": "^1.0.4", | ||
"grunt-contrib-connect": "^2.0.0", | ||
"grunt-saucelabs": "^9.0.1", | ||
"jasmine": "^2.99.0", | ||
"jsdom": "^9.5.0", | ||
"jsonfile": "^2.3.1", | ||
"request": "^2.88.0", | ||
"typescript": "~2.0.0", | ||
"underscore": "^1.8.3", | ||
"webpack": "^2.6.1" | ||
"underscore": "^1.9.1", | ||
"webpack": "^2.7.0" | ||
}, | ||
@@ -42,0 +46,0 @@ "scripts": { |
@@ -15,2 +15,6 @@ JSON-Patch | ||
[](https://travis-ci.org/Starcounter-Jack/JSON-Patch) | ||
## Why you should use JSON-Patch | ||
@@ -29,16 +33,17 @@ | ||
##### [`add` benchmark](https://run.perf.zone/view/JSON-Patch-Add-Operation-1500650502896) | ||
##### [`add` benchmark](https://run.perf.zone/view/JSON-Patch-Add-Operation-1535541298893) | ||
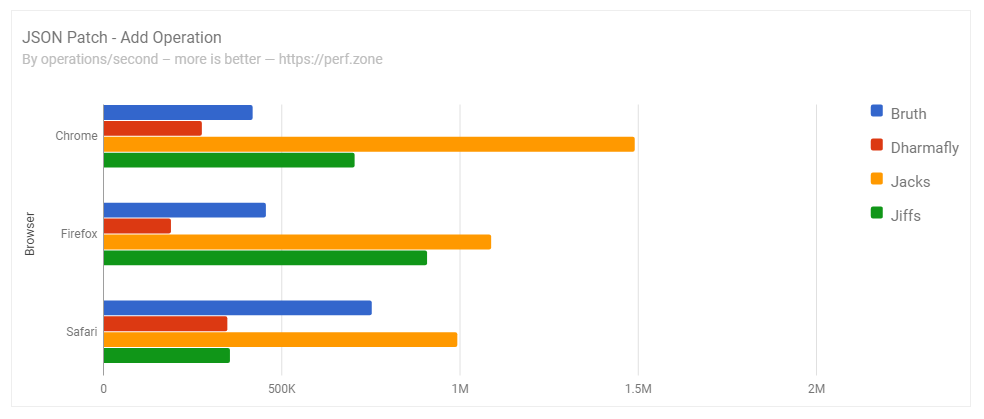 | ||
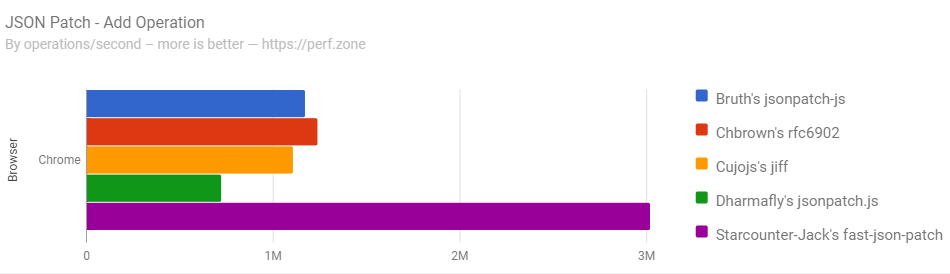 | ||
##### [`replace` benchmark](https://run.perf.zone/view/JSON-Patch-Replace-Operation-1500645548223) | ||
##### [`replace` benchmark](https://run.perf.zone/view/JSON-Patch-Replace-Operation-1535540952263) | ||
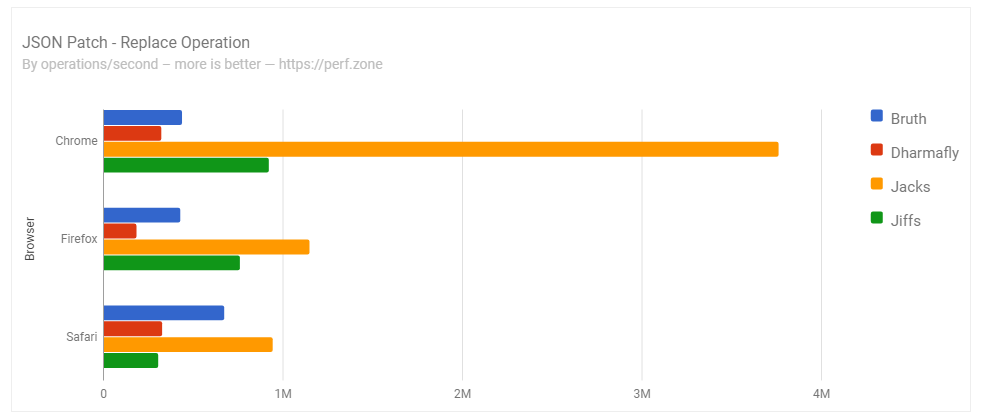 | ||
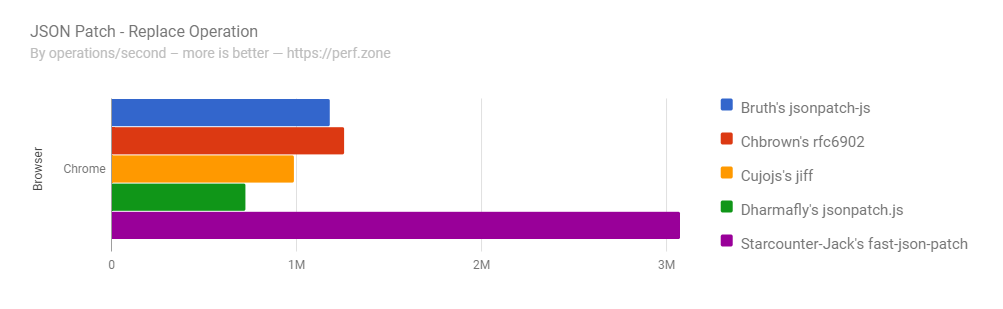 | ||
Tested on 24.07.2017. Compared libraries: | ||
Tested on 29.08.2018. Compared libraries: | ||
- [Starcounter-Jack/JSON-Patch](https://www.npmjs.com/package/fast-json-patch) 2.0.4 | ||
- [bruth/jsonpatch-js](https://www.npmjs.com/package/json-patch) 0.6.1 | ||
- [dharmafly/jsonpatch.js](https://www.npmjs.com/package/jsonpatch) 3.0.0 | ||
- [Starcounter-Jack/JSON-Patch](https://www.npmjs.com/package/fast-json-patch) 2.0.6 | ||
- [bruth/jsonpatch-js](https://www.npmjs.com/package/json-patch) 0.7.0 | ||
- [dharmafly/jsonpatch.js](https://www.npmjs.com/package/jsonpatch) 3.0.1 | ||
- [jiff](https://www.npmjs.com/package/jiff) 0.7.3 | ||
- [RFC6902](https://www.npmjs.com/package/rfc6902) 2.4.0 | ||
@@ -50,3 +55,3 @@ We aim the tests to be fair. Our library puts performance as the #1 priority, while other libraries can have different priorities. If you'd like to update the benchmarks or add a library, please fork the [perf.zone](https://perf.zone) benchmarks linked above and open an issue to include new results. | ||
* Allows you to freely manipulate object trees and then generate patches for outgoing traffic. | ||
* Tested in IE 8-11, Firefox, Chrome, Safari and Node.js | ||
* Tested in IE11, Firefox, Chrome, Safari and Node.js | ||
@@ -187,10 +192,16 @@ | ||
#### `jsonpatch.applyPatch<T>(document: any, patch: Operation[], validateOperation: Boolean | Function = false): OperationResult<T>[]` | ||
#### `function applyPatch<T>(document: T, patch: Operation[], validateOperation?: boolean | Validator<T>, mutateDocument: boolean = true, banPrototypeModifications: boolean = true): PatchResult<T>` | ||
Applies `patch` array on `obj`. | ||
- `document` The document to patch | ||
- `patch` a JSON-Patch array of operations to apply | ||
- `validateOperation` Boolean for whether to validate each operation with our default validator, or to pass a validator callback | ||
- `mutateDocument` Whether to mutate the original document or clone it before applying | ||
- `banPrototypeModifications` Whether to ban modifications to `__proto__`, defaults to `true`. | ||
An invalid patch results in throwing an error (see `jsonpatch.validate` for more information about the error object). | ||
It modifies the `document` object and `patch` - it gets the values by reference. | ||
If you would like to avoid touching your values, clone them: `jsonpatch.applyPatch(document, jsonpatch.deepClone(patch))`. | ||
If you would like to avoid touching your `patch` array values, clone them: `jsonpatch.applyPatch(document, jsonpatch.deepClone(patch))`. | ||
@@ -203,9 +214,9 @@ Returns an array of [`OperationResult`](#operationresult-type) objects - one item for each item in `patches`, each item is an object `{newDocument: any, test?: boolean, removed?: any}`. | ||
** Note: It throws `TEST_OPERATION_FAILED` error if `test` operation fails. ** | ||
- ** Note: It throws `TEST_OPERATION_FAILED` error if `test` operation fails. ** | ||
- ** Note II: the returned array has `newDocument` property that you can use as the final state of the patched document **. | ||
- ** Note III: By default, when `banPrototypeModifications` is `true`, this method throws a `TypeError` when you attempt to modify an object's prototype. | ||
** Note II: the returned array has `newDocument` property that you can use as the final state of the patched document **. | ||
- See [Validation notes](#validation-notes). | ||
#### `applyOperation<T>(document: any, operation: Operation, validateOperation: <Boolean | Function> = false, mutateDocument = true): OperationResult<T>` | ||
#### `function applyOperation<T>(document: T, operation: Operation, validateOperation: boolean | Validator<T> = false, mutateDocument: boolean = true, banPrototypeModifications: boolean = true, index: number = 0): OperationResult<T>` | ||
@@ -218,2 +229,4 @@ Applies single operation object `operation` on `document`. | ||
- `mutateDocument` Whether to mutate the original document or clone it before applying | ||
- `banPrototypeModifications` Whether to ban modifications to `__proto__`, defaults to `true`. | ||
- `index` The index of the operation in your patch array. Useful for better error reporting when that operation fails to apply. | ||
@@ -225,7 +238,8 @@ It modifies the `document` object and `operation` - it gets the values by reference. | ||
** Note: It throws `TEST_OPERATION_FAILED` error if `test` operation fails. ** | ||
- ** Note: It throws `TEST_OPERATION_FAILED` error if `test` operation fails. ** | ||
- ** Note II: By default, when `banPrototypeModifications` is `true`, this method throws a `TypeError` when you attempt to modify an object's prototype. | ||
- See [Validation notes](#validation-notes). | ||
#### `jsonpatch.applyReducer<T>(document: T, operation: Operation): T` | ||
#### `jsonpatch.applyReducer<T>(document: T, operation: Operation, index: number): T` | ||
@@ -232,0 +246,0 @@ **Ideal for `patch.reduce(jsonpatch.applyReducer, document)`**. |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
110657
1926
380
13