fastest-validator
Advanced tools
Comparing version 1.11.1 to 1.12.0
@@ -0,1 +1,11 @@ | ||
<a name="1.12.0"></a> | ||
# 1.12.0 (2021-10-17) | ||
## Changes | ||
- update dev dependencies. | ||
- add parameters to dynamic default value function. E.g: `age: (schema, field, parent, context) => { ... }` | ||
- fix typescript definitions. [#269](https://github.com/icebob/fastest-validator/pull/269), [#270](https://github.com/icebob/fastest-validator/pull/270), [#261](https://github.com/icebob/fastest-validator/pull/261) | ||
- fix multi validate with object strict remove. [#272](https://github.com/icebob/fastest-validator/pull/272) | ||
- add `normalize` method. [#275](https://github.com/icebob/fastest-validator/pull/275) E.g.: `validator.normalize({ a: "string[]|optional" })` | ||
<a name="1.11.1"></a> | ||
@@ -2,0 +12,0 @@ # 1.11.1 (2021-07-14) |
@@ -7,2 +7,3 @@ | ||
| "class" | ||
| "currency" | ||
| "custom" | ||
@@ -108,2 +109,37 @@ | "date" | ||
/** | ||
* Validation schema definition for "currency" built-in validator | ||
* @see https://github.com/icebob/fastest-validator#currency | ||
*/ | ||
export interface RuleCurrency extends RuleCustom { | ||
/** | ||
* Name of built-in validator | ||
*/ | ||
type: "currency"; | ||
/** | ||
* The currency symbol expected in string (as prefix) | ||
* @default null | ||
*/ | ||
currencySymbol?: string; | ||
/** | ||
* Toggle to make the currency symbol optional in string | ||
* @default false | ||
*/ | ||
symbolOptional?: boolean; | ||
/** | ||
* Thousand place separator character | ||
* @default ',' | ||
*/ | ||
thousandSeparator?: string; | ||
/** | ||
* Decimal place character | ||
* @default '.' | ||
*/ | ||
decimalSeparator?: string; | ||
/** | ||
* Custom regular expression to validate currency strings | ||
*/ | ||
customRegex?: RegExp | string; | ||
} | ||
/** | ||
* Validation schema definition for "date" built-in validator | ||
@@ -386,3 +422,3 @@ * @see https://github.com/icebob/fastest-validator#date | ||
*/ | ||
contains?: string[]; | ||
contains?: string; | ||
/** | ||
@@ -393,7 +429,7 @@ * The value must be an element of the enum array | ||
/** | ||
* The value must be an alphabetic string | ||
* The value must be a numeric string | ||
*/ | ||
numeric?: boolean; | ||
/** | ||
* The value must be a numeric string | ||
* The value must be an alphabetic string | ||
*/ | ||
@@ -761,2 +797,3 @@ alpha?: boolean; | ||
| RuleClass | ||
| RuleCurrency | ||
| RuleDate | ||
@@ -1055,2 +1092,15 @@ | RuleEmail | ||
}; | ||
/** | ||
* Normalize a schema, type or short hand definition by expanding it to a full form. The 'normalized' | ||
* form is the equivalent schema with any short hands undone. This ensure that each rule; always includes | ||
* a 'type' key, arrays always have an 'items' key, 'multi' always have a 'rules' key and objects always | ||
* have their properties defined in a 'props' key | ||
* | ||
* @param { ValidationSchema | string | any } value The value to normalize | ||
* @return {ValidationRule | ValidationSchema } The normalized form of the given rule or schema | ||
*/ | ||
normalize( | ||
value: ValidationSchema | string | any | ||
): ValidationRule | ValidationSchema | ||
} |
(function (global, factory) { | ||
typeof exports === 'object' && typeof module !== 'undefined' ? module.exports = factory() : | ||
typeof define === 'function' && define.amd ? define(factory) : | ||
(global = global || self, global.FastestValidator = factory()); | ||
}(this, (function () { 'use strict'; | ||
(global = typeof globalThis !== 'undefined' ? globalThis : global || self, global.FastestValidator = factory()); | ||
})(this, (function () { 'use strict'; | ||
@@ -120,2 +120,61 @@ function isObjectHasKeys(v) { | ||
}; | ||
messages.required; | ||
messages.string; | ||
messages.stringEmpty; | ||
messages.stringMin; | ||
messages.stringMax; | ||
messages.stringLength; | ||
messages.stringPattern; | ||
messages.stringContains; | ||
messages.stringEnum; | ||
messages.stringNumeric; | ||
messages.stringAlpha; | ||
messages.stringAlphanum; | ||
messages.stringAlphadash; | ||
messages.stringHex; | ||
messages.stringSingleLine; | ||
messages.stringBase64; | ||
messages.number; | ||
messages.numberMin; | ||
messages.numberMax; | ||
messages.numberEqual; | ||
messages.numberNotEqual; | ||
messages.numberInteger; | ||
messages.numberPositive; | ||
messages.numberNegative; | ||
messages.array; | ||
messages.arrayEmpty; | ||
messages.arrayMin; | ||
messages.arrayMax; | ||
messages.arrayLength; | ||
messages.arrayContains; | ||
messages.arrayUnique; | ||
messages.arrayEnum; | ||
messages.tuple; | ||
messages.tupleEmpty; | ||
messages.tupleLength; | ||
messages.currency; | ||
messages.date; | ||
messages.dateMin; | ||
messages.dateMax; | ||
messages.enumValue; | ||
messages.equalValue; | ||
messages.equalField; | ||
messages.forbidden; | ||
messages.email; | ||
messages.emailEmpty; | ||
messages.emailMin; | ||
messages.emailMax; | ||
messages.luhn; | ||
messages.mac; | ||
messages.object; | ||
messages.objectStrict; | ||
messages.objectMinProps; | ||
messages.objectMaxProps; | ||
messages.url; | ||
messages.urlEmpty; | ||
messages.uuid; | ||
messages.uuidVersion; | ||
messages.classInstanceOf; | ||
messages.objectID; | ||
@@ -415,3 +474,3 @@ /** Signature: function(value, field, parent, errors, context) | ||
var _function = function(ref, path, context) { | ||
var schema = ref.schema; | ||
ref.schema; | ||
var messages = ref.messages; | ||
@@ -428,17 +487,17 @@ | ||
var schema = ref.schema; | ||
var messages = ref.messages; | ||
ref.messages; | ||
var src = []; | ||
src.push("\n\t\tvar prevErrLen = errors.length;\n\t\tvar errBefore;\n\t\tvar hasValid = false;\n\t\tvar newVal = value;\n\t"); | ||
src.push("\n\t\tvar hasValid = false;\n\t\tvar newVal = value;\n\t\tvar checkErrors = [];\n\t"); | ||
for (var i = 0; i < schema.rules.length; i++) { | ||
src.push("\n\t\t\tif (!hasValid) {\n\t\t\t\terrBefore = errors.length;\n\t\t"); | ||
src.push("\n\t\t\tif (!hasValid) {\n\t\t\t\tvar _errors = [];\n\t\t"); | ||
var rule = this.getRuleFromSchema(schema.rules[i]); | ||
src.push(this.compileRule(rule, context, path, ("var tmpVal = " + (context.async ? "await " : "") + "context.fn[%%INDEX%%](value, field, parent, errors, context);"), "tmpVal")); | ||
src.push("\n\t\t\t\tif (errors.length == errBefore) {\n\t\t\t\t\thasValid = true;\n\t\t\t\t\tnewVal = tmpVal;\n\t\t\t\t}\n\t\t\t}\n\t\t"); | ||
src.push(this.compileRule(rule, context, path, ("var tmpVal = " + (context.async ? "await " : "") + "context.fn[%%INDEX%%](value, field, parent, _errors, context);"), "tmpVal")); | ||
src.push("\n\t\t\t\tif (_errors.length == 0) {\n\t\t\t\t\thasValid = true;\n\t\t\t\t\tnewVal = tmpVal;\n\t\t\t\t} else {\n\t\t\t\t\tArray.prototype.push.apply(checkErrors, _errors);\n\t\t\t\t}\n\t\t\t}\n\t\t"); | ||
} | ||
src.push("\n\t\tif (hasValid) {\n\t\t\terrors.length = prevErrLen;\n\t\t}\n\n\t\treturn newVal;\n\t"); | ||
src.push("\n\t\tif (!hasValid) {\n\t\t\tArray.prototype.push.apply(errors, checkErrors);\n\t\t}\n\n\t\treturn newVal;\n\t"); | ||
@@ -574,10 +633,12 @@ return { | ||
if (schema.strict) { | ||
sourceCode.push("\n\t\t\t\tif (errors.length === 0) {\n\t\t\t"); | ||
var allowedProps = Object.keys(subSchema); | ||
sourceCode.push(("\n\t\t\t\tfield = parentField;\n\t\t\t\tvar invalidProps = [];\n\t\t\t\tvar props = Object.keys(parentObj);\n\n\t\t\t\tfor (let i = 0; i < props.length; i++) {\n\t\t\t\t\tif (" + (JSON.stringify(allowedProps)) + ".indexOf(props[i]) === -1) {\n\t\t\t\t\t\tinvalidProps.push(props[i]);\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tif (invalidProps.length) {\n\t\t\t")); | ||
sourceCode.push(("\n\t\t\t\t\tfield = parentField;\n\t\t\t\t\tvar invalidProps = [];\n\t\t\t\t\tvar props = Object.keys(parentObj);\n\n\t\t\t\t\tfor (let i = 0; i < props.length; i++) {\n\t\t\t\t\t\tif (" + (JSON.stringify(allowedProps)) + ".indexOf(props[i]) === -1) {\n\t\t\t\t\t\t\tinvalidProps.push(props[i]);\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tif (invalidProps.length) {\n\t\t\t")); | ||
if (schema.strict == "remove") { | ||
sourceCode.push("\n\t\t\t\t\tinvalidProps.forEach(function(field) {\n\t\t\t\t\t\tdelete parentObj[field];\n\t\t\t\t\t});\n\t\t\t\t"); | ||
sourceCode.push("\n\t\t\t\t\t\tinvalidProps.forEach(function(field) {\n\t\t\t\t\t\t\tdelete parentObj[field];\n\t\t\t\t\t\t});\n\t\t\t\t"); | ||
} else { | ||
sourceCode.push(("\n\t\t\t\t\t" + (this.makeError({ type: "objectStrict", expected: "\"" + allowedProps.join(", ") + "\"", actual: "invalidProps.join(', ')", messages: messages })) + "\n\t\t\t\t")); | ||
} | ||
sourceCode.push("\n\t\t\t\t\t}\n\t\t\t"); | ||
sourceCode.push("\n\t\t\t\t}\n\t\t\t"); | ||
@@ -833,3 +894,3 @@ } | ||
var PATTERN = /^https?:\/\/\S+/; | ||
var PATTERN$2 = /^https?:\/\/\S+/; | ||
//const PATTERN = /^(?:(?:https?|ftp):\/\/)(?:\S+(?::\S*)?@)?(?:(?!(?:10|127)(?:\.\d{1,3}){3})(?!(?:169\.254|192\.168)(?:\.\d{1,3}){2})(?!172\.(?:1[6-9]|2\d|3[0-1])(?:\.\d{1,3}){2})(?:[1-9]\d?|1\d\d|2[01]\d|22[0-3])(?:\.(?:1?\d{1,2}|2[0-4]\d|25[0-5])){2}(?:\.(?:[1-9]\d?|1\d\d|2[0-4]\d|25[0-4]))|(?:(?:[a-z\u00a1-\uffff0-9]-*)*[a-z\u00a1-\uffff0-9]+)(?:\.(?:[a-z\u00a1-\uffff0-9]-*)*[a-z\u00a1-\uffff0-9]+)*(?:\.(?:[a-z\u00a1-\uffff]{2,}))\.?)(?::\d{2,5})?(?:[/?#]\S*)?$/i; | ||
@@ -854,3 +915,3 @@ //const PATTERN = /https?:\/\/(www\.)?[-a-zA-Z0-9@:%._\+~#=]{2,256}\.[a-z]{2,4}\b([-a-zA-Z0-9@:%_\+.~#?&//=]*)/g; | ||
src.push(("\n\t\tif (!" + (PATTERN.toString()) + ".test(value)) {\n\t\t\t" + (this.makeError({ type: "url", actual: "value", messages: messages })) + "\n\t\t}\n\n\t\treturn value;\n\t")); | ||
src.push(("\n\t\tif (!" + (PATTERN$2.toString()) + ".test(value)) {\n\t\t\t" + (this.makeError({ type: "url", actual: "value", messages: messages })) + "\n\t\t}\n\n\t\treturn value;\n\t")); | ||
@@ -884,3 +945,3 @@ return { | ||
var PATTERN$2 = /^((([a-f0-9][a-f0-9]+[-]){5}|([a-f0-9][a-f0-9]+[:]){5})([a-f0-9][a-f0-9])$)|(^([a-f0-9][a-f0-9][a-f0-9][a-f0-9]+[.]){2}([a-f0-9][a-f0-9][a-f0-9][a-f0-9]))$/i; | ||
var PATTERN = /^((([a-f0-9][a-f0-9]+[-]){5}|([a-f0-9][a-f0-9]+[:]){5})([a-f0-9][a-f0-9])$)|(^([a-f0-9][a-f0-9][a-f0-9][a-f0-9]+[.]){2}([a-f0-9][a-f0-9][a-f0-9][a-f0-9]))$/i; | ||
@@ -890,7 +951,7 @@ /** Signature: function(value, field, parent, errors, context) | ||
var mac = function(ref, path, context) { | ||
var schema = ref.schema; | ||
ref.schema; | ||
var messages = ref.messages; | ||
return { | ||
source: ("\n\t\t\tif (typeof value !== \"string\") {\n\t\t\t\t" + (this.makeError({ type: "string", actual: "value", messages: messages })) + "\n\t\t\t\treturn value;\n\t\t\t}\n\n\t\t\tvar v = value.toLowerCase();\n\t\t\tif (!" + (PATTERN$2.toString()) + ".test(v)) {\n\t\t\t\t" + (this.makeError({ type: "mac", actual: "value", messages: messages })) + "\n\t\t\t}\n\t\t\t\n\t\t\treturn value;\n\t\t") | ||
source: ("\n\t\t\tif (typeof value !== \"string\") {\n\t\t\t\t" + (this.makeError({ type: "string", actual: "value", messages: messages })) + "\n\t\t\t\treturn value;\n\t\t\t}\n\n\t\t\tvar v = value.toLowerCase();\n\t\t\tif (!" + (PATTERN.toString()) + ".test(v)) {\n\t\t\t\t" + (this.makeError({ type: "mac", actual: "value", messages: messages })) + "\n\t\t\t}\n\t\t\t\n\t\t\treturn value;\n\t\t") | ||
}; | ||
@@ -909,3 +970,3 @@ }; | ||
var luhn = function(ref, path, context) { | ||
var schema = ref.schema; | ||
ref.schema; | ||
var messages = ref.messages; | ||
@@ -1084,3 +1145,3 @@ | ||
context.customs[rule.index].defaultFn = rule.schema.default; | ||
defaultValue = "context.customs[" + (rule.index) + "].defaultFn()"; | ||
defaultValue = "context.customs[" + (rule.index) + "].defaultFn.call(this, context.rules[" + (rule.index) + "].schema, field, parent, context)"; | ||
} else { | ||
@@ -1235,37 +1296,4 @@ defaultValue = JSON.stringify(rule.schema.default); | ||
Validator.prototype.getRuleFromSchema = function getRuleFromSchema (schema) { | ||
var this$1 = this; | ||
schema = this.resolveType(schema); | ||
if (typeof schema === "string") { | ||
schema = this.parseShortHand(schema); | ||
} else if (Array.isArray(schema)) { | ||
if (schema.length == 0) | ||
{ throw new Error("Invalid schema."); } | ||
schema = { | ||
type: "multi", | ||
rules: schema | ||
}; | ||
// Check 'optional' flag | ||
var isOptional = schema.rules | ||
.map(function (s) { return this$1.getRuleFromSchema(s); }) | ||
.every(function (rule) { return rule.schema.optional == true; }); | ||
if (isOptional) | ||
{ schema.optional = true; } | ||
} | ||
if (schema.$$type) { | ||
var type = schema.$$type; | ||
var otherShorthandProps = this.getRuleFromSchema(type).schema; | ||
delete schema.$$type; | ||
var props = Object.assign({}, schema); | ||
for (var key in schema) { // clear object without changing reference | ||
delete schema[key]; | ||
} | ||
deepExtend_1(schema, otherShorthandProps, { skipIfExist: true }); | ||
schema.props = props; | ||
} | ||
var alias = this.aliases[schema.type]; | ||
@@ -1441,2 +1469,105 @@ if (alias) { | ||
/** | ||
* Resolve the schema 'type' by: | ||
* - parsing short hands into full type definitions | ||
* - expanding arrays into 'multi' types with a rules property | ||
* - objects which have a root $$type property into a schema which | ||
* explicitly has a 'type' property and a 'props' property. | ||
* | ||
* @param schema The schema to resolve the type of | ||
*/ | ||
Validator.prototype.resolveType = function resolveType (schema) { | ||
var this$1$1 = this; | ||
if (typeof schema === "string") { | ||
schema = this.parseShortHand(schema); | ||
} else if (Array.isArray(schema)) { | ||
if (schema.length === 0) | ||
{ throw new Error("Invalid schema."); } | ||
schema = { | ||
type: "multi", | ||
rules: schema | ||
}; | ||
// Check 'optional' flag | ||
var isOptional = schema.rules | ||
.map(function (s) { return this$1$1.getRuleFromSchema(s); }) | ||
.every(function (rule) { return rule.schema.optional === true; }); | ||
if (isOptional) | ||
{ schema.optional = true; } | ||
} | ||
if (schema.$$type) { | ||
var type = schema.$$type; | ||
var otherShorthandProps = this.getRuleFromSchema(type).schema; | ||
delete schema.$$type; | ||
var props = Object.assign({}, schema); | ||
for (var key in schema) { // clear object without changing reference | ||
delete schema[key]; | ||
} | ||
deepExtend_1(schema, otherShorthandProps, { skipIfExist: true }); | ||
schema.props = props; | ||
} | ||
return schema; | ||
}; | ||
/** | ||
* Normalize a schema, type or short hand definition by expanding it to a full form. The 'normalized' | ||
* form is the equivalent schema with any short hands undone. This ensure that each rule; always includes | ||
* a 'type' key, arrays always have an 'items' key, 'multi' always have a 'rules' key and objects always | ||
* have their properties defined in a 'props' key | ||
* | ||
* @param {Object|String} value The value to normalize | ||
* @returns {Object} The normalized form of the given rule or schema | ||
*/ | ||
Validator.prototype.normalize = function normalize (value) { | ||
var this$1$1 = this; | ||
var result = this.resolveType(value); | ||
if(this.aliases[result.type]) | ||
{ result = deepExtend_1(result, this.normalize(this.aliases[result.type]), { skipIfExists: true}); } | ||
result = deepExtend_1(result, this.defaults[result.type], { skipIfExist: true }); | ||
if(result.type === "multi") { | ||
result.rules = result.rules.map(function (r) { return this$1$1.normalize(r); }); | ||
result.optional = result.rules.every(function (r) { return r.optional === true; }); | ||
return result; | ||
} | ||
if(result.type === "array") { | ||
result.items = this.normalize(result.items); | ||
return result; | ||
} | ||
if(result.type === "object") { | ||
if(result.props) { | ||
Object.entries(result.props).forEach(function (ref) { | ||
var k = ref[0]; | ||
var v = ref[1]; | ||
return result.props[k] = this$1$1.normalize(v); | ||
}); | ||
} | ||
} | ||
if(typeof value === "object") { | ||
if(value.type) { | ||
var config = this.normalize(value.type); | ||
deepExtend_1(result, config, { skipIfExists: true }); | ||
} | ||
else { | ||
Object.entries(value).forEach(function (ref) { | ||
var k = ref[0]; | ||
var v = ref[1]; | ||
return result[k] = this$1$1.normalize(v); | ||
}); | ||
} | ||
} | ||
return result; | ||
}; | ||
var validator = Validator; | ||
@@ -1448,3 +1579,3 @@ | ||
}))); | ||
})); | ||
//# sourceMappingURL=index.js.map |
@@ -1,1 +0,88 @@ | ||
"use strict";function v(){function t(t){if(this.opts={},this.defaults={},this.messages=Object.assign({},F),this.rules={any:k,array:S,boolean:E,class:x,custom:b,currency:g,date:y,email:v,enum:d,equal:m,forbidden:h,function:p,multi:c,number:f,object:o,objectID:l,string:u,tuple:i,url:s,uuid:a,mac:r,luhn:n},this.aliases={},this.cache=new Map,t){if(j(this.opts,t),t.defaults&&j(this.defaults,t.defaults),t.messages)for(var O in t.messages)this.addMessage(O,t.messages[O]);if(t.aliases)for(var _ in t.aliases)this.alias(_,t.aliases[_]);if(t.customRules)for(var w in t.customRules)this.add(w,t.customRules[w]);if(t.plugins){if(t=t.plugins,!Array.isArray(t))throw Error("Plugins type must be array");t.forEach(this.plugin.bind(this))}this.opts.debug&&(t=function(t){return t},"undefined"==typeof window&&(t=e),this._formatter=t)}}function e(t){return T||(T=w(),A={parser:"babel",useTabs:!1,printWidth:120,trailingComma:"none",tabWidth:4,singleQuote:!1,semi:!0,bracketSpacing:!0},I=w(),N={language:"js",theme:I.fromJson({keyword:["white","bold"],built_in:"magenta",literal:"cyan",number:"magenta",regexp:"red",string:["yellow","bold"],symbol:"plain",class:"blue",attr:"plain",function:["white","bold"],title:"plain",params:"green",comment:"grey"})}),t=T.format(t,A),I.highlight(t,N)}function n(t){return t=t.messages,{source:'\n\t\t\tif (typeof value !== "string") {\n\t\t\t\t'+this.makeError({type:"string",actual:"value",messages:t})+'\n\t\t\t\treturn value;\n\t\t\t}\n\n\t\t\tif (typeof value !== "string")\n\t\t\t\tvalue = String(value);\n\n\t\t\tval = value.replace(/\\D+/g, "");\n\n\t\t\tvar array = [0, 2, 4, 6, 8, 1, 3, 5, 7, 9];\n\t\t\tvar len = val ? val.length : 0,\n\t\t\t\tbit = 1,\n\t\t\t\tsum = 0;\n\t\t\twhile (len--) {\n\t\t\t\tsum += !(bit ^= 1) ? parseInt(val[len], 10) : array[val[len]];\n\t\t\t}\n\n\t\t\tif (!(sum % 10 === 0 && sum > 0)) {\n\t\t\t\t'+this.makeError({type:"luhn",actual:"value",messages:t})+"\n\t\t\t}\n\n\t\t\treturn value;\n\t\t"}}function r(t){return t=t.messages,{source:'\n\t\t\tif (typeof value !== "string") {\n\t\t\t\t'+this.makeError({type:"string",actual:"value",messages:t})+"\n\t\t\t\treturn value;\n\t\t\t}\n\n\t\t\tvar v = value.toLowerCase();\n\t\t\tif (!"+J.toString()+".test(v)) {\n\t\t\t\t"+this.makeError({type:"mac",actual:"value",messages:t})+"\n\t\t\t}\n\t\t\t\n\t\t\treturn value;\n\t\t"}}function a(t){var e=t.schema;t=t.messages;var n=[];return n.push('\n\t\tif (typeof value !== "string") {\n\t\t\t'+this.makeError({type:"string",actual:"value",messages:t})+"\n\t\t\treturn value;\n\t\t}\n\n\t\tvar val = value.toLowerCase();\n\t\tif (!"+Y.toString()+".test(val)) {\n\t\t\t"+this.makeError({type:"uuid",actual:"value",messages:t})+"\n\t\t\treturn value;\n\t\t}\n\n\t\tconst version = val.charAt(14) | 0;\n\t"),7>parseInt(e.version)&&n.push("\n\t\t\tif ("+e.version+" !== version) {\n\t\t\t\t"+this.makeError({type:"uuidVersion",expected:e.version,actual:"version",messages:t})+"\n\t\t\t\treturn value;\n\t\t\t}\n\t\t"),n.push('\n\t\tswitch (version) {\n\t\tcase 0:\n\t\tcase 1:\n\t\tcase 2:\n\t\tcase 6:\n\t\t\tbreak;\n\t\tcase 3:\n\t\tcase 4:\n\t\tcase 5:\n\t\t\tif (["8", "9", "a", "b"].indexOf(val.charAt(19)) === -1) {\n\t\t\t\t'+this.makeError({type:"uuid",actual:"value",messages:t})+"\n\t\t\t}\n\t\t}\n\n\t\treturn value;\n\t"),{source:n.join("\n")}}function s(t){var e=t.schema;t=t.messages;var n=[];return n.push('\n\t\tif (typeof value !== "string") {\n\t\t\t'+this.makeError({type:"string",actual:"value",messages:t})+"\n\t\t\treturn value;\n\t\t}\n\t"),e.empty?n.push("\n\t\t\tif (value.length === 0) return value;\n\t\t"):n.push("\n\t\t\tif (value.length === 0) {\n\t\t\t\t"+this.makeError({type:"urlEmpty",actual:"value",messages:t})+"\n\t\t\t\treturn value;\n\t\t\t}\n\t\t"),n.push("\n\t\tif (!"+z.toString()+".test(value)) {\n\t\t\t"+this.makeError({type:"url",actual:"value",messages:t})+"\n\t\t}\n\n\t\treturn value;\n\t"),{source:n.join("\n")}}function i(t,e,n){var r=t.schema,a=t.messages;if(t=[],null!=r.items){if(!Array.isArray(r.items))throw Error("Invalid '"+r.type+"' schema. The 'items' field must be an array.");if(0===r.items.length)throw Error("Invalid '"+r.type+"' schema. The 'items' field must not be an empty array.")}if(t.push("\n\t\tif (!Array.isArray(value)) {\n\t\t\t"+this.makeError({type:"tuple",actual:"value",messages:a})+"\n\t\t\treturn value;\n\t\t}\n\n\t\tvar len = value.length;\n\t"),!1===r.empty&&t.push("\n\t\t\tif (len === 0) {\n\t\t\t\t"+this.makeError({type:"tupleEmpty",actual:"value",messages:a})+"\n\t\t\t\treturn value;\n\t\t\t}\n\t\t"),null!=r.items){for(t.push("\n\t\t\tif ("+r.empty+" !== false && len === 0) {\n\t\t\t\treturn value;\n\t\t\t}\n\n\t\t\tif (len !== "+r.items.length+") {\n\t\t\t\t"+this.makeError({type:"tupleLength",expected:r.items.length,actual:"len",messages:a})+"\n\t\t\t\treturn value;\n\t\t\t}\n\t\t"),t.push("\n\t\t\tvar arr = value;\n\t\t\tvar parentField = field;\n\t\t"),a=0;a<r.items.length;a++){t.push("\n\t\t\tvalue = arr["+a+"];\n\t\t");var s=e+"["+a+"]",i=this.getRuleFromSchema(r.items[a]);t.push(this.compileRule(i,n,s,"\n\t\t\tarr["+a+"] = "+(n.async?"await ":"")+"context.fn[%%INDEX%%](arr["+a+'], (parentField ? parentField : "") + "[" + '+a+' + "]", parent, errors, context);\n\t\t',"arr["+a+"]"))}t.push("\n\t\treturn arr;\n\t")}else t.push("\n\t\treturn value;\n\t");return{source:t.join("\n")}}function u(t){var e=t.schema;t=t.messages;var n=[],r=!1;if(!0===e.convert&&(r=!0,n.push('\n\t\t\tif (typeof value !== "string") {\n\t\t\t\tvalue = String(value);\n\t\t\t}\n\t\t')),n.push('\n\t\tif (typeof value !== "string") {\n\t\t\t'+this.makeError({type:"string",actual:"value",messages:t})+"\n\t\t\treturn value;\n\t\t}\n\n\t\tvar origValue = value;\n\t"),e.trim&&(r=!0,n.push("\n\t\t\tvalue = value.trim();\n\t\t")),e.trimLeft&&(r=!0,n.push("\n\t\t\tvalue = value.trimLeft();\n\t\t")),e.trimRight&&(r=!0,n.push("\n\t\t\tvalue = value.trimRight();\n\t\t")),e.padStart&&(r=!0,n.push("\n\t\t\tvalue = value.padStart("+e.padStart+", "+JSON.stringify(null!=e.padChar?e.padChar:" ")+");\n\t\t")),e.padEnd&&(r=!0,n.push("\n\t\t\tvalue = value.padEnd("+e.padEnd+", "+JSON.stringify(null!=e.padChar?e.padChar:" ")+");\n\t\t")),e.lowercase&&(r=!0,n.push("\n\t\t\tvalue = value.toLowerCase();\n\t\t")),e.uppercase&&(r=!0,n.push("\n\t\t\tvalue = value.toUpperCase();\n\t\t")),e.localeLowercase&&(r=!0,n.push("\n\t\t\tvalue = value.toLocaleLowerCase();\n\t\t")),e.localeUppercase&&(r=!0,n.push("\n\t\t\tvalue = value.toLocaleUpperCase();\n\t\t")),n.push("\n\t\t\tvar len = value.length;\n\t"),!1===e.empty&&n.push("\n\t\t\tif (len === 0) {\n\t\t\t\t"+this.makeError({type:"stringEmpty",actual:"value",messages:t})+"\n\t\t\t}\n\t\t"),null!=e.min&&n.push("\n\t\t\tif (len < "+e.min+") {\n\t\t\t\t"+this.makeError({type:"stringMin",expected:e.min,actual:"len",messages:t})+"\n\t\t\t}\n\t\t"),null!=e.max&&n.push("\n\t\t\tif (len > "+e.max+") {\n\t\t\t\t"+this.makeError({type:"stringMax",expected:e.max,actual:"len",messages:t})+"\n\t\t\t}\n\t\t"),null!=e.length&&n.push("\n\t\t\tif (len !== "+e.length+") {\n\t\t\t\t"+this.makeError({type:"stringLength",expected:e.length,actual:"len",messages:t})+"\n\t\t\t}\n\t\t"),null!=e.pattern){var a=e.pattern;"string"==typeof e.pattern&&(a=new RegExp(e.pattern,e.patternFlags)),a="\n\t\t\tif (!"+a.toString()+".test(value))\n\t\t\t\t"+this.makeError({type:"stringPattern",expected:'"'+a.toString().replace(/"/g,"\\$&")+'"',actual:"origValue",messages:t})+"\n\t\t",n.push("\n\t\t\tif ("+e.empty+" === true && len === 0) {\n\t\t\t\t// Do nothing\n\t\t\t} else {\n\t\t\t\t"+a+"\n\t\t\t}\n\t\t")}return null!=e.contains&&n.push('\n\t\t\tif (value.indexOf("'+e.contains+'") === -1) {\n\t\t\t\t'+this.makeError({type:"stringContains",expected:'"'+e.contains+'"',actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),null!=e.enum&&(a=JSON.stringify(e.enum),n.push("\n\t\t\tif ("+a+".indexOf(value) === -1) {\n\t\t\t\t"+this.makeError({type:"stringEnum",expected:'"'+e.enum.join(", ")+'"',actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t")),!0===e.numeric&&n.push("\n\t\t\tif (!"+V.toString()+".test(value) ) {\n\t\t\t\t"+this.makeError({type:"stringNumeric",actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),!0===e.alpha&&n.push("\n\t\t\tif(!"+R.toString()+".test(value)) {\n\t\t\t\t"+this.makeError({type:"stringAlpha",actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),!0===e.alphanum&&n.push("\n\t\t\tif(!"+$.toString()+".test(value)) {\n\t\t\t\t"+this.makeError({type:"stringAlphanum",actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),!0===e.alphadash&&n.push("\n\t\t\tif(!"+q.toString()+".test(value)) {\n\t\t\t\t"+this.makeError({type:"stringAlphadash",actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),!0===e.hex&&n.push("\n\t\t\tif(value.length % 2 !== 0 || !"+U.toString()+".test(value)) {\n\t\t\t\t"+this.makeError({type:"stringHex",actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),!0===e.singleLine&&n.push('\n\t\t\tif(value.includes("\\n")) {\n\t\t\t\t'+this.makeError({type:"stringSingleLine",messages:t})+"\n\t\t\t}\n\t\t"),!0===e.base64&&n.push("\n\t\t\tif(!"+D.toString()+".test(value)) {\n\t\t\t\t"+this.makeError({type:"stringBase64",actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),n.push("\n\t\treturn value;\n\t"),{sanitized:r,source:n.join("\n")}}function l(t,e,n){e=t.schema;var r=t.messages;t=t.index;var a=[];return n.customs[t]?n.customs[t].schema=e:n.customs[t]={schema:e},a.push("\n\t\tconst ObjectID = context.customs["+t+"].schema.ObjectID;\n\t\tif (!ObjectID.isValid(value)) {\n\t\t\t"+this.makeError({type:"objectID",actual:"value",messages:r})+"\n\t\t\treturn;\n\t\t}\n\t"),!0===e.convert?a.push("return new ObjectID(value)"):"hexString"===e.convert?a.push("return value.toString()"):a.push("return value"),{source:a.join("\n")}}function o(t,e,n){var r=t.schema;t=t.messages;var a=[];a.push('\n\t\tif (typeof value !== "object" || value === null || Array.isArray(value)) {\n\t\t\t'+this.makeError({type:"object",actual:"value",messages:t})+"\n\t\t\treturn value;\n\t\t}\n\t");var s=r.properties||r.props;if(s){a.push("var parentObj = value;"),a.push("var parentField = field;");for(var i=Object.keys(s),u=0;u<i.length;u++){var l=i[u],o=_(l),f=C.test(o)?"."+o:"['"+o+"']",c="parentObj"+f,p=(e?e+".":"")+l;a.push("\n// Field: "+_(p)),a.push('field = parentField ? parentField + "'+f+'" : "'+o+'";'),a.push("value = "+c+";"),l=this.getRuleFromSchema(s[l]),a.push(this.compileRule(l,n,p,"\n\t\t\t\t"+c+" = "+(n.async?"await ":"")+"context.fn[%%INDEX%%](value, field, parentObj, errors, context);\n\t\t\t",c))}r.strict&&(e=Object.keys(s),a.push("\n\t\t\t\tfield = parentField;\n\t\t\t\tvar invalidProps = [];\n\t\t\t\tvar props = Object.keys(parentObj);\n\n\t\t\t\tfor (let i = 0; i < props.length; i++) {\n\t\t\t\t\tif ("+JSON.stringify(e)+".indexOf(props[i]) === -1) {\n\t\t\t\t\t\tinvalidProps.push(props[i]);\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\tif (invalidProps.length) {\n\t\t\t"),"remove"==r.strict?a.push("\n\t\t\t\t\tinvalidProps.forEach(function(field) {\n\t\t\t\t\t\tdelete parentObj[field];\n\t\t\t\t\t});\n\t\t\t\t"):a.push("\n\t\t\t\t\t"+this.makeError({type:"objectStrict",expected:'"'+e.join(", ")+'"',actual:"invalidProps.join(', ')",messages:t})+"\n\t\t\t\t"),a.push("\n\t\t\t\t}\n\t\t\t"))}return null==r.minProps&&null==r.maxProps||(r.strict?a.push("\n\t\t\t\tprops = Object.keys("+(s?"parentObj":"value")+");\n\t\t\t"):a.push("\n\t\t\t\tvar props = Object.keys("+(s?"parentObj":"value")+");\n\t\t\t\t"+(s?"field = parentField;":"")+"\n\t\t\t")),null!=r.minProps&&a.push("\n\t\t\tif (props.length < "+r.minProps+") {\n\t\t\t\t"+this.makeError({type:"objectMinProps",expected:r.minProps,actual:"props.length",messages:t})+"\n\t\t\t}\n\t\t"),null!=r.maxProps&&a.push("\n\t\t\tif (props.length > "+r.maxProps+") {\n\t\t\t\t"+this.makeError({type:"objectMaxProps",expected:r.maxProps,actual:"props.length",messages:t})+"\n\t\t\t}\n\t\t"),s?a.push("\n\t\t\treturn parentObj;\n\t\t"):a.push("\n\t\t\treturn value;\n\t\t"),{source:a.join("\n")}}function f(t){var e=t.schema;t=t.messages;var n=[];n.push("\n\t\tvar origValue = value;\n\t");var r=!1;return!0===e.convert&&(r=!0,n.push('\n\t\t\tif (typeof value !== "number") {\n\t\t\t\tvalue = Number(value);\n\t\t\t}\n\t\t')),n.push('\n\t\tif (typeof value !== "number" || isNaN(value) || !isFinite(value)) {\n\t\t\t'+this.makeError({type:"number",actual:"origValue",messages:t})+"\n\t\t\treturn value;\n\t\t}\n\t"),null!=e.min&&n.push("\n\t\t\tif (value < "+e.min+") {\n\t\t\t\t"+this.makeError({type:"numberMin",expected:e.min,actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),null!=e.max&&n.push("\n\t\t\tif (value > "+e.max+") {\n\t\t\t\t"+this.makeError({type:"numberMax",expected:e.max,actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),null!=e.equal&&n.push("\n\t\t\tif (value !== "+e.equal+") {\n\t\t\t\t"+this.makeError({type:"numberEqual",expected:e.equal,actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),null!=e.notEqual&&n.push("\n\t\t\tif (value === "+e.notEqual+") {\n\t\t\t\t"+this.makeError({type:"numberNotEqual",expected:e.notEqual,actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),!0===e.integer&&n.push("\n\t\t\tif (value % 1 !== 0) {\n\t\t\t\t"+this.makeError({type:"numberInteger",actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),!0===e.positive&&n.push("\n\t\t\tif (value <= 0) {\n\t\t\t\t"+this.makeError({type:"numberPositive",actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),!0===e.negative&&n.push("\n\t\t\tif (value >= 0) {\n\t\t\t\t"+this.makeError({type:"numberNegative",actual:"origValue",messages:t})+"\n\t\t\t}\n\t\t"),n.push("\n\t\treturn value;\n\t"),{sanitized:r,source:n.join("\n")}}function c(t,e,n){t=t.schema;var r=[];r.push("\n\t\tvar prevErrLen = errors.length;\n\t\tvar errBefore;\n\t\tvar hasValid = false;\n\t\tvar newVal = value;\n\t");for(var a=0;a<t.rules.length;a++){r.push("\n\t\t\tif (!hasValid) {\n\t\t\t\terrBefore = errors.length;\n\t\t");var s=this.getRuleFromSchema(t.rules[a]);r.push(this.compileRule(s,n,e,"var tmpVal = "+(n.async?"await ":"")+"context.fn[%%INDEX%%](value, field, parent, errors, context);","tmpVal")),r.push("\n\t\t\t\tif (errors.length == errBefore) {\n\t\t\t\t\thasValid = true;\n\t\t\t\t\tnewVal = tmpVal;\n\t\t\t\t}\n\t\t\t}\n\t\t")}return r.push("\n\t\tif (hasValid) {\n\t\t\terrors.length = prevErrLen;\n\t\t}\n\n\t\treturn newVal;\n\t"),{source:r.join("\n")}}function p(t){return{source:'\n\t\t\tif (typeof value !== "function")\n\t\t\t\t'+this.makeError({type:"function",actual:"value",messages:t.messages})+"\n\n\t\t\treturn value;\n\t\t"}}function h(t){var e=t.schema;t=t.messages;var n=[];return n.push("\n\t\tif (value !== null && value !== undefined) {\n\t"),e.remove?n.push("\n\t\t\treturn undefined;\n\t\t"):n.push("\n\t\t\t"+this.makeError({type:"forbidden",actual:"value",messages:t})+"\n\t\t"),n.push("\n\t\t}\n\n\t\treturn value;\n\t"),{source:n.join("\n")}}function m(t){var e=t.schema;t=t.messages;var n=[];return e.field?(e.strict?n.push('\n\t\t\t\tif (value !== parent["'+e.field+'"])\n\t\t\t'):n.push('\n\t\t\t\tif (value != parent["'+e.field+'"])\n\t\t\t'),n.push("\n\t\t\t\t"+this.makeError({type:"equalField",actual:"value",expected:JSON.stringify(e.field),messages:t})+"\n\t\t")):(e.strict?n.push("\n\t\t\t\tif (value !== "+JSON.stringify(e.value)+")\n\t\t\t"):n.push("\n\t\t\t\tif (value != "+JSON.stringify(e.value)+")\n\t\t\t"),n.push("\n\t\t\t\t"+this.makeError({type:"equalValue",actual:"value",expected:JSON.stringify(e.value),messages:t})+"\n\t\t")),n.push("\n\t\treturn value;\n\t"),{source:n.join("\n")}}function d(t){var e=t.schema;return t=t.messages,{source:"\n\t\t\tif ("+JSON.stringify(e.values||[])+".indexOf(value) === -1)\n\t\t\t\t"+this.makeError({type:"enumValue",expected:'"'+e.values.join(", ")+'"',actual:"value",messages:t})+"\n\t\t\t\n\t\t\treturn value;\n\t\t"}}function v(t){var e=t.schema;t=t.messages;var n=[],r="precise"==e.mode?M:P,a=!1;return n.push('\n\t\tif (typeof value !== "string") {\n\t\t\t'+this.makeError({type:"string",actual:"value",messages:t})+"\n\t\t\treturn value;\n\t\t}\n\t"),e.empty?n.push("\n\t\t\tif (value.length === 0) return value;\n\t\t"):n.push("\n\t\t\tif (value.length === 0) {\n\t\t\t\t"+this.makeError({type:"emailEmpty",actual:"value",messages:t})+"\n\t\t\t\treturn value;\n\t\t\t}\n\t\t"),e.normalize&&(a=!0,n.push("\n\t\t\tvalue = value.trim().toLowerCase();\n\t\t")),null!=e.min&&n.push("\n\t\t\tif (value.length < "+e.min+") {\n\t\t\t\t"+this.makeError({type:"emailMin",expected:e.min,actual:"value.length",messages:t})+"\n\t\t\t}\n\t\t"),null!=e.max&&n.push("\n\t\t\tif (value.length > "+e.max+") {\n\t\t\t\t"+this.makeError({type:"emailMax",expected:e.max,actual:"value.length",messages:t})+"\n\t\t\t}\n\t\t"),n.push("\n\t\tif (!"+r.toString()+".test(value)) {\n\t\t\t"+this.makeError({type:"email",actual:"value",messages:t})+"\n\t\t}\n\n\t\treturn value;\n\t"),{sanitized:a,source:n.join("\n")}}function y(t){var e=t.schema;t=t.messages;var n=[],r=!1;return n.push("\n\t\tvar origValue = value;\n\t"),!0===e.convert&&(r=!0,n.push("\n\t\t\tif (!(value instanceof Date)) {\n\t\t\t\tvalue = new Date(value);\n\t\t\t}\n\t\t")),n.push("\n\t\tif (!(value instanceof Date) || isNaN(value.getTime()))\n\t\t\t"+this.makeError({type:"date",actual:"origValue",messages:t})+"\n\n\t\treturn value;\n\t"),{sanitized:r,source:n.join("\n")}}function g(t){var e=t.schema;t=t.messages;var n=e.currencySymbol||null,r=e.thousandSeparator||",",a=e.decimalSeparator||".",s=e.customRegex;return e=!e.symbolOptional,e="(?=.*\\d)^(-?~1|~1-?)(([0-9]\\d{0,2}(~2\\d{3})*)|0)?(\\~3\\d{1,2})?$".replace(/~1/g,n?"\\"+n+(e?"":"?"):"").replace("~2",r).replace("~3",a),(n=[]).push("\n\t\tif (!value.match("+(s||new RegExp(e))+")) {\n\t\t\t"+this.makeError({type:"currency",actual:"value",messages:t})+"\n\t\t\treturn value;\n\t\t}\n\n\t\treturn value;\n\t"),{source:n.join("\n")}}function b(t,e,n){var r=[];return r.push("\n\t\t"+this.makeCustomValidator({fnName:"check",path:e,schema:t.schema,messages:t.messages,context:n,ruleIndex:t.index})+"\n\t\treturn value;\n\t"),{source:r.join("\n")}}function x(t,e,n){e=t.schema;var r=t.messages;t=t.index;var a=[],s=e.instanceOf.name?e.instanceOf.name:"<UnknowClass>";return n.customs[t]?n.customs[t].schema=e:n.customs[t]={schema:e},a.push("\n\t\tif (!(value instanceof context.customs["+t+"].schema.instanceOf))\n\t\t\t"+this.makeError({type:"classInstanceOf",actual:"value",expected:"'"+s+"'",messages:r})+"\n\t"),a.push("\n\t\treturn value;\n\t"),{source:a.join("\n")}}function E(t){var e=t.schema;t=t.messages;var n=[],r=!1;return n.push("\n\t\tvar origValue = value;\n\t"),!0===e.convert&&(r=!0,n.push('\n\t\t\tif (typeof value !== "boolean") {\n\t\t\t\tif (\n\t\t\t\tvalue === 1\n\t\t\t\t|| value === "true"\n\t\t\t\t|| value === "1"\n\t\t\t\t|| value === "on"\n\t\t\t\t) {\n\t\t\t\t\tvalue = true;\n\t\t\t\t} else if (\n\t\t\t\tvalue === 0\n\t\t\t\t|| value === "false"\n\t\t\t\t|| value === "0"\n\t\t\t\t|| value === "off"\n\t\t\t\t) {\n\t\t\t\t\tvalue = false;\n\t\t\t\t}\n\t\t\t}\n\t\t')),n.push('\n\t\tif (typeof value !== "boolean") {\n\t\t\t'+this.makeError({type:"boolean",actual:"origValue",messages:t})+"\n\t\t}\n\t\t\n\t\treturn value;\n\t"),{sanitized:r,source:n.join("\n")}}function S(t,e,n){var r=t.schema,a=t.messages;if((t=[]).push("\n\t\tif (!Array.isArray(value)) {\n\t\t\t"+this.makeError({type:"array",actual:"value",messages:a})+"\n\t\t\treturn value;\n\t\t}\n\n\t\tvar len = value.length;\n\t"),!1===r.empty&&t.push("\n\t\t\tif (len === 0) {\n\t\t\t\t"+this.makeError({type:"arrayEmpty",actual:"value",messages:a})+"\n\t\t\t}\n\t\t"),null!=r.min&&t.push("\n\t\t\tif (len < "+r.min+") {\n\t\t\t\t"+this.makeError({type:"arrayMin",expected:r.min,actual:"len",messages:a})+"\n\t\t\t}\n\t\t"),null!=r.max&&t.push("\n\t\t\tif (len > "+r.max+") {\n\t\t\t\t"+this.makeError({type:"arrayMax",expected:r.max,actual:"len",messages:a})+"\n\t\t\t}\n\t\t"),null!=r.length&&t.push("\n\t\t\tif (len !== "+r.length+") {\n\t\t\t\t"+this.makeError({type:"arrayLength",expected:r.length,actual:"len",messages:a})+"\n\t\t\t}\n\t\t"),null!=r.contains&&t.push("\n\t\t\tif (value.indexOf("+JSON.stringify(r.contains)+") === -1) {\n\t\t\t\t"+this.makeError({type:"arrayContains",expected:JSON.stringify(r.contains),actual:"value",messages:a})+"\n\t\t\t}\n\t\t"),!0===r.unique&&t.push("\n\t\t\tif(len > (new Set(value)).size) {\n\t\t\t\t"+this.makeError({type:"arrayUnique",expected:"Array.from(new Set(value.filter((item, index) => value.indexOf(item) !== index)))",actual:"value",messages:a})+"\n\t\t\t}\n\t\t"),null!=r.enum){var s=JSON.stringify(r.enum);t.push("\n\t\t\tfor (var i = 0; i < value.length; i++) {\n\t\t\t\tif ("+s+".indexOf(value[i]) === -1) {\n\t\t\t\t\t"+this.makeError({type:"arrayEnum",expected:'"'+r.enum.join(", ")+'"',actual:"value[i]",messages:a})+"\n\t\t\t\t}\n\t\t\t}\n\t\t")}return null!=r.items?(t.push("\n\t\t\tvar arr = value;\n\t\t\tvar parentField = field;\n\t\t\tfor (var i = 0; i < arr.length; i++) {\n\t\t\t\tvalue = arr[i];\n\t\t"),e+="[]",r=this.getRuleFromSchema(r.items),t.push(this.compileRule(r,n,e,"arr[i] = "+(n.async?"await ":"")+'context.fn[%%INDEX%%](arr[i], (parentField ? parentField : "") + "[" + i + "]", parent, errors, context)',"arr[i]")),t.push("\n\t\t\t}\n\t\t"),t.push("\n\t\treturn arr;\n\t")):t.push("\n\t\treturn value;\n\t"),{source:t.join("\n")}}function k(){var t=[];return t.push("\n\t\treturn value;\n\t"),{source:t.join("\n")}}function O(t,e,n){return t.replace(e,void 0===n||null===n?"":"function"==typeof n.toString?n:typeof n)}function j(t,e,n){void 0===n&&(n={});for(var r in e){var a=e[r];(a="object"==typeof a&&!Array.isArray(a)&&null!=a&&0<Object.keys(a).length)?(t[r]=t[r]||{},j(t[r],e[r],n)):!0===n.skipIfExist&&void 0!==t[r]||(t[r]=e[r])}return t}function _(t){return t.replace(L,function(t){switch(t){case'"':case"'":case"\\":return"\\"+t;case"\n":return"\\n";case"\r":return"\\r";case"\u2028":return"\\u2028";case"\u2029":return"\\u2029"}})}function w(){throw Error("Dynamic requires are not currently supported by rollup-plugin-commonjs")}var T,A,I,N,F={required:"The '{field}' field is required.",string:"The '{field}' field must be a string.",stringEmpty:"The '{field}' field must not be empty.",stringMin:"The '{field}' field length must be greater than or equal to {expected} characters long.",stringMax:"The '{field}' field length must be less than or equal to {expected} characters long.",stringLength:"The '{field}' field length must be {expected} characters long.",stringPattern:"The '{field}' field fails to match the required pattern.",stringContains:"The '{field}' field must contain the '{expected}' text.",stringEnum:"The '{field}' field does not match any of the allowed values.",stringNumeric:"The '{field}' field must be a numeric string.",stringAlpha:"The '{field}' field must be an alphabetic string.",stringAlphanum:"The '{field}' field must be an alphanumeric string.",stringAlphadash:"The '{field}' field must be an alphadash string.",stringHex:"The '{field}' field must be a hex string.",stringSingleLine:"The '{field}' field must be a single line string.",stringBase64:"The '{field}' field must be a base64 string.",number:"The '{field}' field must be a number.",numberMin:"The '{field}' field must be greater than or equal to {expected}.",numberMax:"The '{field}' field must be less than or equal to {expected}.",numberEqual:"The '{field}' field must be equal to {expected}.",numberNotEqual:"The '{field}' field can't be equal to {expected}.",numberInteger:"The '{field}' field must be an integer.",numberPositive:"The '{field}' field must be a positive number.",numberNegative:"The '{field}' field must be a negative number.",array:"The '{field}' field must be an array.",arrayEmpty:"The '{field}' field must not be an empty array.",arrayMin:"The '{field}' field must contain at least {expected} items.",arrayMax:"The '{field}' field must contain less than or equal to {expected} items.",arrayLength:"The '{field}' field must contain {expected} items.",arrayContains:"The '{field}' field must contain the '{expected}' item.",arrayUnique:"The '{actual}' value in '{field}' field does not unique the '{expected}' values.",arrayEnum:"The '{actual}' value in '{field}' field does not match any of the '{expected}' values.",tuple:"The '{field}' field must be an array.",tupleEmpty:"The '{field}' field must not be an empty array.",tupleLength:"The '{field}' field must contain {expected} items.",boolean:"The '{field}' field must be a boolean.",currency:"The '{field}' must be a valid currency format",date:"The '{field}' field must be a Date.",dateMin:"The '{field}' field must be greater than or equal to {expected}.",dateMax:"The '{field}' field must be less than or equal to {expected}.",enumValue:"The '{field}' field value '{expected}' does not match any of the allowed values.",equalValue:"The '{field}' field value must be equal to '{expected}'.",equalField:"The '{field}' field value must be equal to '{expected}' field value.",forbidden:"The '{field}' field is forbidden.",function:"The '{field}' field must be a function.",email:"The '{field}' field must be a valid e-mail.",emailEmpty:"The '{field}' field must not be empty.",emailMin:"The '{field}' field length must be greater than or equal to {expected} characters long.",emailMax:"The '{field}' field length must be less than or equal to {expected} characters long.",luhn:"The '{field}' field must be a valid checksum luhn.",mac:"The '{field}' field must be a valid MAC address.",object:"The '{field}' must be an Object.",objectStrict:"The object '{field}' contains forbidden keys: '{actual}'.",objectMinProps:"The object '{field}' must contain at least {expected} properties.",objectMaxProps:"The object '{field}' must contain {expected} properties at most.",url:"The '{field}' field must be a valid URL.",urlEmpty:"The '{field}' field must not be empty.",uuid:"The '{field}' field must be a valid UUID.",uuidVersion:"The '{field}' field must be a valid UUID version provided.",classInstanceOf:"The '{field}' field must be an instance of the '{expected}' class.",objectID:"The '{field}' field must be an valid ObjectID"},M=/^(([^<>()[\]\\.,;:\s@"]+(\.[^<>()[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/,P=/^\S+@\S+\.\S+$/,C=/^[_$a-zA-Z][_$a-zA-Z0-9]*$/,L=/["'\\\n\r\u2028\u2029]/g,V=/^-?[0-9]\d*(\.\d+)?$/,R=/^[a-zA-Z]+$/,$=/^[a-zA-Z0-9]+$/,q=/^[a-zA-Z0-9_-]+$/,U=/^[0-9a-fA-F]+$/,D=/^(?:[A-Za-z0-9+\\/]{4})*(?:[A-Za-z0-9+\\/]{2}==|[A-Za-z0-9+/]{3}=)?$/,z=/^https?:\/\/\S+/,Y=/^([0-9a-f]{8}-[0-9a-f]{4}-[1-6][0-9a-f]{3}-[0-9a-f]{4}-[0-9a-f]{12}|[0]{8}-[0]{4}-[0]{4}-[0]{4}-[0]{12})$/i,J=/^((([a-f0-9][a-f0-9]+[-]){5}|([a-f0-9][a-f0-9]+[:]){5})([a-f0-9][a-f0-9])$)|(^([a-f0-9][a-f0-9][a-f0-9][a-f0-9]+[.]){2}([a-f0-9][a-f0-9][a-f0-9][a-f0-9]))$/i;try{var X=new Function("return Object.getPrototypeOf(async function(){}).constructor")()}catch(t){}return t.prototype.validate=function(t,e){return this.compile(e)(t)},t.prototype.wrapRequiredCheckSourceCode=function(t,e,n,r){var a=[],s=!0===t.schema.optional||"forbidden"===t.schema.type,i=!0===t.schema.optional||!0===t.schema.nullable||"forbidden"===t.schema.type;return null!=t.schema.default?(s=!1,!0!==t.schema.nullable&&(i=!1),"function"==typeof t.schema.default?(n.customs[t.index]||(n.customs[t.index]={}),n.customs[t.index].defaultFn=t.schema.default,t="context.customs["+t.index+"].defaultFn()"):t=JSON.stringify(t.schema.default),r="\n\t\t\t\tvalue = "+t+";\n\t\t\t\t"+r+" = value;\n\t\t\t"):r=this.makeError({type:"required",actual:"value",messages:t.messages}),a.push("\n\t\t\tif (value === undefined) { "+(s?"\n// allow undefined\n":r)+" }\n\t\t\telse if (value === null) { "+(i?"\n// allow null\n":r)+" }\n\t\t\t"+(e?"else { "+e+" }":"")+"\n\t\t"),a.join("\n")},t.prototype.compile=function(t){function e(t,e){return r.data=t,e&&e.meta&&(r.meta=e.meta),s.call(n,t,r)}if(null===t||"object"!=typeof t)throw Error("Invalid schema.");var n=this,r={index:0,async:!0===t.$$async,rules:[],fn:[],customs:{},utils:{replace:O}};if(this.cache.clear(),delete t.$$async,r.async&&!X)throw Error("Asynchronous mode is not supported.");if(!0!==t.$$root)if(Array.isArray(t))t=this.getRuleFromSchema(t).schema;else{var a=Object.assign({},t);t={type:"object",strict:a.$$strict,properties:a},delete a.$$strict}a=["var errors = [];","var field;","var parent = null;"],t=this.getRuleFromSchema(t),a.push(this.compileRule(t,r,null,(r.async?"await ":"")+"context.fn[%%INDEX%%](value, field, null, errors, context);","value")),a.push("if (errors.length) {"),a.push("\n\t\t\treturn errors.map(err => {\n\t\t\t\tif (err.message) {\n\t\t\t\t\terr.message = context.utils.replace(err.message, /\\{field\\}/g, err.field);\n\t\t\t\t\terr.message = context.utils.replace(err.message, /\\{expected\\}/g, err.expected);\n\t\t\t\t\terr.message = context.utils.replace(err.message, /\\{actual\\}/g, err.actual);\n\t\t\t\t}\n\n\t\t\t\treturn err;\n\t\t\t});\n\t\t"),a.push("}"),a.push("return true;"),t=a.join("\n");var s=new(r.async?X:Function)("value","context",t);return this.opts.debug&&console.log(this._formatter("// Main check function\n"+s.toString())),this.cache.clear(),e.async=r.async,e},t.prototype.compileRule=function(t,e,n,r,a){var s=[],i=this.cache.get(t.schema);return i?(t=i,t.cycle=!0,t.cycleStack=[],s.push(this.wrapRequiredCheckSourceCode(t,"\n\t\t\t\tvar rule = context.rules["+t.index+"];\n\t\t\t\tif (rule.cycleStack.indexOf(value) === -1) {\n\t\t\t\t\trule.cycleStack.push(value);\n\t\t\t\t\t"+r.replace(/%%INDEX%%/g,t.index)+"\n\t\t\t\t\trule.cycleStack.pop(value);\n\t\t\t\t}\n\t\t\t",e,a))):(this.cache.set(t.schema,t),t.index=e.index,e.rules[e.index]=t,i=null!=n?n:"$$root",e.index++,n=t.ruleFunction.call(this,t,n,e),n.source=n.source.replace(/%%INDEX%%/g,t.index),n=new(e.async?X:Function)("value","field","parent","errors","context",n.source),e.fn[t.index]=n.bind(this),s.push(this.wrapRequiredCheckSourceCode(t,r.replace(/%%INDEX%%/g,t.index),e,a)),s.push(this.makeCustomValidator({vName:a,path:i,schema:t.schema,context:e,messages:t.messages,ruleIndex:t.index})),this.opts.debug&&console.log(this._formatter("// Context.fn["+t.index+"]\n"+n.toString()))),s.join("\n")},t.prototype.getRuleFromSchema=function(t){var e=this;if("string"==typeof t)t=this.parseShortHand(t);else if(Array.isArray(t)){if(0==t.length)throw Error("Invalid schema.");(t={type:"multi",rules:t}).rules.map(function(t){return e.getRuleFromSchema(t)}).every(function(t){return 1==t.schema.optional})&&(t.optional=!0)}if(t.$$type){var n=this.getRuleFromSchema(t.$$type).schema;delete t.$$type;var r,a=Object.assign({},t);for(r in t)delete t[r];j(t,n,{skipIfExist:!0}),t.props=a}if((n=this.aliases[t.type])&&(delete t.type,t=j(t,n,{skipIfExist:!0})),!(n=this.rules[t.type]))throw Error("Invalid '"+t.type+"' type in validator schema.");return{messages:Object.assign({},this.messages,t.messages),schema:j(t,this.defaults[t.type],{skipIfExist:!0}),ruleFunction:n}},t.prototype.parseShortHand=function(t){var e=(t=t.split("|").map(function(t){return t.trim()}))[0],n=e.endsWith("[]")?this.getRuleFromSchema({type:"array",items:e.slice(0,-2)}).schema:{type:t[0]};return t.slice(1).map(function(t){var e=t.indexOf(":");if(-1!==e){var r=t.substr(0,e).trim();"true"===(t=t.substr(e+1).trim())||"false"===t?t="true"===t:Number.isNaN(Number(t))||(t=Number(t)),n[r]=t}else t.startsWith("no-")?n[t.slice(3)]=!1:n[t]=!0}),n},t.prototype.makeError=function(t){var e=t.type,n=t.field,r=t.expected,a=t.actual,s={type:'"'+e+'"',message:'"'+t.messages[e]+'"'};return s.field=n?'"'+n+'"':"field",null!=r&&(s.expected=r),null!=a&&(s.actual=a),"errors.push({ "+Object.keys(s).map(function(t){return t+": "+s[t]}).join(", ")+" });"},t.prototype.makeCustomValidator=function(t){var e=t.vName;void 0===e&&(e="value");var n=t.fnName;void 0===n&&(n="custom");var r=t.ruleIndex,a=t.path,s=t.schema,i=t.context,u=t.messages;t="rule"+r;var l="fnCustomErrors"+r;return"function"==typeof s[n]?(i.customs[r]?(i.customs[r].messages=u,i.customs[r].schema=s):i.customs[r]={messages:u,schema:s},this.opts.useNewCustomCheckerFunction?"\n \t\tconst "+t+" = context.customs["+r+"];\n\t\t\t\t\tconst "+l+" = [];\n\t\t\t\t\t"+e+" = "+(i.async?"await ":"")+t+".schema."+n+".call(this, "+e+", "+l+" , "+t+'.schema, "'+a+'", parent, context);\n\t\t\t\t\tif (Array.isArray('+l+" )) {\n \t\t"+l+" .forEach(err => errors.push(Object.assign({ message: "+t+".messages[err.type], field }, err)));\n\t\t\t\t\t}\n\t\t\t\t":(s="res_"+t,"\n\t\t\t\tconst "+t+" = context.customs["+r+"];\n\t\t\t\tconst "+s+" = "+(i.async?"await ":"")+t+".schema."+n+".call(this, "+e+", "+t+'.schema, "'+a+'", parent, context);\n\t\t\t\tif (Array.isArray('+s+")) {\n\t\t\t\t\t"+s+".forEach(err => errors.push(Object.assign({ message: "+t+".messages[err.type], field }, err)));\n\t\t\t\t}\n\t\t")):""},t.prototype.add=function(t,e){this.rules[t]=e},t.prototype.addMessage=function(t,e){this.messages[t]=e},t.prototype.alias=function(t,e){if(this.rules[t])throw Error("Alias name must not be a rule name");this.aliases[t]=e},t.prototype.plugin=function(t){if("function"!=typeof t)throw Error("Plugin fn type must be function");return t(this)},t}var f=f||{};f.scope={},f.arrayIteratorImpl=function(t){var e=0;return function(){return e<t.length?{done:!1,value:t[e++]}:{done:!0}}},f.arrayIterator=function(t){return{next:f.arrayIteratorImpl(t)}},f.ASSUME_ES5=!1,f.ASSUME_NO_NATIVE_MAP=!1,f.ASSUME_NO_NATIVE_SET=!1,f.SIMPLE_FROUND_POLYFILL=!1,f.ISOLATE_POLYFILLS=!1,f.defineProperty=f.ASSUME_ES5||"function"==typeof Object.defineProperties?Object.defineProperty:function(t,e,n){return t==Array.prototype||t==Object.prototype?t:(t[e]=n.value,t)},f.getGlobal=function(t){t=["object"==typeof globalThis&&globalThis,t,"object"==typeof window&&window,"object"==typeof self&&self,"object"==typeof global&&global];for(var e=0;e<t.length;++e){var n=t[e];if(n&&n.Math==Math)return n}throw Error("Cannot find global object")},f.global=f.getGlobal(this),f.IS_SYMBOL_NATIVE="function"==typeof Symbol&&"symbol"==typeof Symbol("x"),f.TRUST_ES6_POLYFILLS=!f.ISOLATE_POLYFILLS||f.IS_SYMBOL_NATIVE,f.polyfills={},f.propertyToPolyfillSymbol={},f.POLYFILL_PREFIX="$jscp$",f.polyfill=function(t,e,n,r){e&&(f.ISOLATE_POLYFILLS?f.polyfillIsolated(t,e,n,r):f.polyfillUnisolated(t,e,n,r))},f.polyfillUnisolated=function(t,e){var n=f.global;t=t.split(".");for(var r=0;r<t.length-1;r++){var a=t[r];a in n||(n[a]={}),n=n[a]}(e=e(r=n[t=t[t.length-1]]))!=r&&null!=e&&f.defineProperty(n,t,{configurable:!0,writable:!0,value:e})},f.polyfillIsolated=function(t,e,n){var r=t.split(".");t=1===r.length;var a=r[0];a=!t&&a in f.polyfills?f.polyfills:f.global;for(var s=0;s<r.length-1;s++){var i=r[s];i in a||(a[i]={}),a=a[i]}r=r[r.length-1],null!=(e=e(n=f.IS_SYMBOL_NATIVE&&"es6"===n?a[r]:null))&&(t?f.defineProperty(f.polyfills,r,{configurable:!0,writable:!0,value:e}):e!==n&&(f.propertyToPolyfillSymbol[r]=f.IS_SYMBOL_NATIVE?f.global.Symbol(r):f.POLYFILL_PREFIX+r,r=f.propertyToPolyfillSymbol[r],f.defineProperty(a,r,{configurable:!0,writable:!0,value:e})))},f.initSymbol=function(){},f.polyfill("Symbol",function(t){function e(t){if(this instanceof e)throw new TypeError("Symbol is not a constructor");return new n("jscomp_symbol_"+(t||"")+"_"+r++,t)}function n(t,e){this.$jscomp$symbol$id_=t,f.defineProperty(this,"description",{configurable:!0,writable:!0,value:e})}if(t)return t;n.prototype.toString=function(){return this.$jscomp$symbol$id_};var r=0;return e},"es6","es3"),f.initSymbolIterator=function(){},f.polyfill("Symbol.iterator",function(t){if(t)return t;t=Symbol("Symbol.iterator");for(var e="Array Int8Array Uint8Array Uint8ClampedArray Int16Array Uint16Array Int32Array Uint32Array Float32Array Float64Array".split(" "),n=0;n<e.length;n++){var r=f.global[e[n]];"function"==typeof r&&"function"!=typeof r.prototype[t]&&f.defineProperty(r.prototype,t,{configurable:!0,writable:!0,value:function(){return f.iteratorPrototype(f.arrayIteratorImpl(this))}})}return t},"es6","es3"),f.initSymbolAsyncIterator=function(){},f.iteratorPrototype=function(t){return t={next:t},t[Symbol.iterator]=function(){return this},t},f.iteratorFromArray=function(t,e){t instanceof String&&(t+="");var n=0,r={next:function(){if(n<t.length){var a=n++;return{value:e(a,t[a]),done:!1}}return r.next=function(){return{done:!0,value:void 0}},r.next()}};return r[Symbol.iterator]=function(){return r},r},f.polyfill("Array.prototype.keys",function(t){return t||function(){return f.iteratorFromArray(this,function(t){return t})}},"es6","es3"),f.polyfill("Array.prototype.values",function(t){return t||function(){return f.iteratorFromArray(this,function(t,e){return e})}},"es8","es3"),f.owns=function(t,e){return Object.prototype.hasOwnProperty.call(t,e)},f.assign=f.TRUST_ES6_POLYFILLS&&"function"==typeof Object.assign?Object.assign:function(t,e){for(var n=1;n<arguments.length;n++){var r=arguments[n];if(r)for(var a in r)f.owns(r,a)&&(t[a]=r[a])}return t},f.polyfill("Object.assign",function(t){return t||f.assign},"es6","es3"),f.checkEs6ConformanceViaProxy=function(){try{var t={},e=Object.create(new f.global.Proxy(t,{get:function(n,r,a){return n==t&&"q"==r&&a==e}}));return!0===e.q}catch(t){return!1}},f.USE_PROXY_FOR_ES6_CONFORMANCE_CHECKS=!1,f.ES6_CONFORMANCE=f.USE_PROXY_FOR_ES6_CONFORMANCE_CHECKS&&f.checkEs6ConformanceViaProxy(),f.makeIterator=function(t){var e="undefined"!=typeof Symbol&&Symbol.iterator&&t[Symbol.iterator];return e?e.call(t):f.arrayIterator(t)},f.polyfill("WeakMap",function(t){function e(t){if(this.id_=(u+=Math.random()+1).toString(),t){t=f.makeIterator(t);for(var e;!(e=t.next()).done;)e=e.value,this.set(e[0],e[1])}}function n(){}function r(t){var e=typeof t;return"object"===e&&null!==t||"function"===e}function a(t){if(!f.owns(t,i)){var e=new n;f.defineProperty(t,i,{value:e})}}function s(t){var e=Object[t];e&&(Object[t]=function(t){return t instanceof n?t:(a(t),e(t))})}if(f.USE_PROXY_FOR_ES6_CONFORMANCE_CHECKS){if(t&&f.ES6_CONFORMANCE)return t}else if(function(){if(!t||!Object.seal)return!1;try{var e=Object.seal({}),n=Object.seal({}),r=new t([[e,2],[n,3]]);return 2==r.get(e)&&3==r.get(n)&&(r.delete(e),r.set(n,4),!r.has(e)&&4==r.get(n))}catch(t){return!1}}())return t;var i="$jscomp_hidden_"+Math.random();s("freeze"),s("preventExtensions"),s("seal");var u=0;return e.prototype.set=function(t,e){if(!r(t))throw Error("Invalid WeakMap key");if(a(t),!f.owns(t,i))throw Error("WeakMap key fail: "+t);return t[i][this.id_]=e,this},e.prototype.get=function(t){return r(t)&&f.owns(t,i)?t[i][this.id_]:void 0},e.prototype.has=function(t){return r(t)&&f.owns(t,i)&&f.owns(t[i],this.id_)},e.prototype.delete=function(t){return!!(r(t)&&f.owns(t,i)&&f.owns(t[i],this.id_))&&delete t[i][this.id_]},e},"es6","es3"),f.MapEntry=function(){},f.polyfill("Map",function(t){function e(){var t={};return t.previous=t.next=t.head=t}function n(t,e){var n=t.head_;return f.iteratorPrototype(function(){if(n){for(;n.head!=t.head_;)n=n.previous;for(;n.next!=n.head;)return n=n.next,{done:!1,value:e(n)};n=null}return{done:!0,value:void 0}})}function r(t,e){var n=e&&typeof e;"object"==n||"function"==n?s.has(e)?n=s.get(e):(n=""+ ++i,s.set(e,n)):n="p_"+e;var r=t.data_[n];if(r&&f.owns(t.data_,n))for(t=0;t<r.length;t++){var a=r[t];if(e!==e&&a.key!==a.key||e===a.key)return{id:n,list:r,index:t,entry:a}}return{id:n,list:r,index:-1,entry:void 0}}function a(t){if(this.data_={},this.head_=e(),this.size=0,t){t=f.makeIterator(t);for(var n;!(n=t.next()).done;)n=n.value,this.set(n[0],n[1])}}if(f.USE_PROXY_FOR_ES6_CONFORMANCE_CHECKS){if(t&&f.ES6_CONFORMANCE)return t}else if(function(){if(f.ASSUME_NO_NATIVE_MAP||!t||"function"!=typeof t||!t.prototype.entries||"function"!=typeof Object.seal)return!1;try{var e=Object.seal({x:4}),n=new t(f.makeIterator([[e,"s"]]));if("s"!=n.get(e)||1!=n.size||n.get({x:4})||n.set({x:4},"t")!=n||2!=n.size)return!1;var r=n.entries(),a=r.next();return!a.done&&a.value[0]==e&&"s"==a.value[1]&&!((a=r.next()).done||4!=a.value[0].x||"t"!=a.value[1]||!r.next().done)}catch(t){return!1}}())return t;var s=new WeakMap;a.prototype.set=function(t,e){var n=r(this,t=0===t?0:t);return n.list||(n.list=this.data_[n.id]=[]),n.entry?n.entry.value=e:(n.entry={next:this.head_,previous:this.head_.previous,head:this.head_,key:t,value:e},n.list.push(n.entry),this.head_.previous.next=n.entry,this.head_.previous=n.entry,this.size++),this},a.prototype.delete=function(t){return!(!(t=r(this,t)).entry||!t.list)&&(t.list.splice(t.index,1),t.list.length||delete this.data_[t.id],t.entry.previous.next=t.entry.next,t.entry.next.previous=t.entry.previous,t.entry.head=null,this.size--,!0)},a.prototype.clear=function(){this.data_={},this.head_=this.head_.previous=e(),this.size=0},a.prototype.has=function(t){return!!r(this,t).entry},a.prototype.get=function(t){return(t=r(this,t).entry)&&t.value},a.prototype.entries=function(){return n(this,function(t){return[t.key,t.value]})},a.prototype.keys=function(){return n(this,function(t){return t.key})},a.prototype.values=function(){return n(this,function(t){return t.value})},a.prototype.forEach=function(t,e){for(var n,r=this.entries();!(n=r.next()).done;)n=n.value,t.call(e,n[1],n[0],this)},a.prototype[Symbol.iterator]=a.prototype.entries;var i=0;return a},"es6","es3"),f.checkStringArgs=function(t,e,n){if(null==t)throw new TypeError("The 'this' value for String.prototype."+n+" must not be null or undefined");if(e instanceof RegExp)throw new TypeError("First argument to String.prototype."+n+" must not be a regular expression");return t+""},f.polyfill("String.prototype.endsWith",function(t){return t||function(t,e){var n=f.checkStringArgs(this,t,"endsWith");t+="",void 0===e&&(e=n.length),e=Math.max(0,Math.min(0|e,n.length));for(var r=t.length;0<r&&0<e;)if(n[--e]!=t[--r])return!1;return 0>=r}},"es6","es3"),f.polyfill("Number.isNaN",function(t){return t||function(t){return"number"==typeof t&&isNaN(t)}},"es6","es3"),f.polyfill("String.prototype.startsWith",function(t){return t||function(t,e){var n=f.checkStringArgs(this,t,"startsWith");t+="";var r=n.length,a=t.length;e=Math.max(0,Math.min(0|e,n.length));for(var s=0;s<a&&e<r;)if(n[e++]!=t[s++])return!1;return s>=a}},"es6","es3");var u=this;"object"==typeof exports&&"undefined"!=typeof module?module.exports=v():"function"==typeof define&&define.amd?define(v):(u=u||self,u.FastestValidator=v()); | ||
'use strict';var g=g||{};g.scope={};g.arrayIteratorImpl=function(e){var h=0;return function(){return h<e.length?{done:!1,value:e[h++]}:{done:!0}}};g.arrayIterator=function(e){return{next:g.arrayIteratorImpl(e)}};g.ASSUME_ES5=!1;g.ASSUME_NO_NATIVE_MAP=!1;g.ASSUME_NO_NATIVE_SET=!1;g.SIMPLE_FROUND_POLYFILL=!1;g.ISOLATE_POLYFILLS=!1;g.FORCE_POLYFILL_PROMISE=!1;g.FORCE_POLYFILL_PROMISE_WHEN_NO_UNHANDLED_REJECTION=!1; | ||
g.defineProperty=g.ASSUME_ES5||"function"==typeof Object.defineProperties?Object.defineProperty:function(e,h,l){if(e==Array.prototype||e==Object.prototype)return e;e[h]=l.value;return e};g.getGlobal=function(e){e=["object"==typeof globalThis&&globalThis,e,"object"==typeof window&&window,"object"==typeof self&&self,"object"==typeof global&&global];for(var h=0;h<e.length;++h){var l=e[h];if(l&&l.Math==Math)return l}throw Error("Cannot find global object");};g.global=g.getGlobal(this); | ||
g.IS_SYMBOL_NATIVE="function"===typeof Symbol&&"symbol"===typeof Symbol("x");g.TRUST_ES6_POLYFILLS=!g.ISOLATE_POLYFILLS||g.IS_SYMBOL_NATIVE;g.polyfills={};g.propertyToPolyfillSymbol={};g.POLYFILL_PREFIX="$jscp$";g.polyfill=function(e,h,l,m){h&&(g.ISOLATE_POLYFILLS?g.polyfillIsolated(e,h,l,m):g.polyfillUnisolated(e,h,l,m))}; | ||
g.polyfillUnisolated=function(e,h){var l=g.global;e=e.split(".");for(var m=0;m<e.length-1;m++){var t=e[m];if(!(t in l))return;l=l[t]}e=e[e.length-1];m=l[e];h=h(m);h!=m&&null!=h&&g.defineProperty(l,e,{configurable:!0,writable:!0,value:h})}; | ||
g.polyfillIsolated=function(e,h,l){var m=e.split(".");e=1===m.length;var t=m[0];t=!e&&t in g.polyfills?g.polyfills:g.global;for(var w=0;w<m.length-1;w++){var x=m[w];if(!(x in t))return;t=t[x]}m=m[m.length-1];l=g.IS_SYMBOL_NATIVE&&"es6"===l?t[m]:null;h=h(l);null!=h&&(e?g.defineProperty(g.polyfills,m,{configurable:!0,writable:!0,value:h}):h!==l&&(void 0===g.propertyToPolyfillSymbol[m]&&(e=1E9*Math.random()>>>0,g.propertyToPolyfillSymbol[m]=g.IS_SYMBOL_NATIVE?g.global.Symbol(m):g.POLYFILL_PREFIX+e+"$"+ | ||
m),g.defineProperty(t,g.propertyToPolyfillSymbol[m],{configurable:!0,writable:!0,value:h})))};g.initSymbol=function(){}; | ||
g.polyfill("Symbol",function(e){function h(w){if(this instanceof h)throw new TypeError("Symbol is not a constructor");return new l(m+(w||"")+"_"+t++,w)}function l(w,x){this.$jscomp$symbol$id_=w;g.defineProperty(this,"description",{configurable:!0,writable:!0,value:x})}if(e)return e;l.prototype.toString=function(){return this.$jscomp$symbol$id_};var m="jscomp_symbol_"+(1E9*Math.random()>>>0)+"_",t=0;return h},"es6","es3"); | ||
g.polyfill("Symbol.iterator",function(e){if(e)return e;e=Symbol("Symbol.iterator");for(var h="Array Int8Array Uint8Array Uint8ClampedArray Int16Array Uint16Array Int32Array Uint32Array Float32Array Float64Array".split(" "),l=0;l<h.length;l++){var m=g.global[h[l]];"function"===typeof m&&"function"!=typeof m.prototype[e]&&g.defineProperty(m.prototype,e,{configurable:!0,writable:!0,value:function(){return g.iteratorPrototype(g.arrayIteratorImpl(this))}})}return e},"es6","es3"); | ||
g.iteratorPrototype=function(e){e={next:e};e[Symbol.iterator]=function(){return this};return e};g.iteratorFromArray=function(e,h){e instanceof String&&(e+="");var l=0,m=!1,t={next:function(){if(!m&&l<e.length){var w=l++;return{value:h(w,e[w]),done:!1}}m=!0;return{done:!0,value:void 0}}};t[Symbol.iterator]=function(){return t};return t};g.polyfill("Array.prototype.keys",function(e){return e?e:function(){return g.iteratorFromArray(this,function(h){return h})}},"es6","es3"); | ||
g.polyfill("Array.prototype.values",function(e){return e?e:function(){return g.iteratorFromArray(this,function(h,l){return l})}},"es8","es3");g.owns=function(e,h){return Object.prototype.hasOwnProperty.call(e,h)};g.assign=g.TRUST_ES6_POLYFILLS&&"function"==typeof Object.assign?Object.assign:function(e,h){for(var l=1;l<arguments.length;l++){var m=arguments[l];if(m)for(var t in m)g.owns(m,t)&&(e[t]=m[t])}return e};g.polyfill("Object.assign",function(e){return e||g.assign},"es6","es3"); | ||
g.checkEs6ConformanceViaProxy=function(){try{var e={},h=Object.create(new g.global.Proxy(e,{get:function(l,m,t){return l==e&&"q"==m&&t==h}}));return!0===h.q}catch(l){return!1}};g.USE_PROXY_FOR_ES6_CONFORMANCE_CHECKS=!1;g.ES6_CONFORMANCE=g.USE_PROXY_FOR_ES6_CONFORMANCE_CHECKS&&g.checkEs6ConformanceViaProxy();g.makeIterator=function(e){var h="undefined"!=typeof Symbol&&Symbol.iterator&&e[Symbol.iterator];return h?h.call(e):g.arrayIterator(e)}; | ||
g.polyfill("WeakMap",function(e){function h(k){this.id_=(n+=Math.random()+1).toString();if(k){k=g.makeIterator(k);for(var p;!(p=k.next()).done;)p=p.value,this.set(p[0],p[1])}}function l(){if(!e||!Object.seal)return!1;try{var k=Object.seal({}),p=Object.seal({}),u=new e([[k,2],[p,3]]);if(2!=u.get(k)||3!=u.get(p))return!1;u.delete(k);u.set(p,4);return!u.has(k)&&4==u.get(p)}catch(C){return!1}}function m(){}function t(k){var p=typeof k;return"object"===p&&null!==k||"function"===p}function w(k){if(!g.owns(k, | ||
y)){var p=new m;g.defineProperty(k,y,{value:p})}}function x(k){if(!g.ISOLATE_POLYFILLS){var p=Object[k];p&&(Object[k]=function(u){if(u instanceof m)return u;Object.isExtensible(u)&&w(u);return p(u)})}}if(g.USE_PROXY_FOR_ES6_CONFORMANCE_CHECKS){if(e&&g.ES6_CONFORMANCE)return e}else if(l())return e;var y="$jscomp_hidden_"+Math.random();x("freeze");x("preventExtensions");x("seal");var n=0;h.prototype.set=function(k,p){if(!t(k))throw Error("Invalid WeakMap key");w(k);if(!g.owns(k,y))throw Error("WeakMap key fail: "+ | ||
k);k[y][this.id_]=p;return this};h.prototype.get=function(k){return t(k)&&g.owns(k,y)?k[y][this.id_]:void 0};h.prototype.has=function(k){return t(k)&&g.owns(k,y)&&g.owns(k[y],this.id_)};h.prototype.delete=function(k){return t(k)&&g.owns(k,y)&&g.owns(k[y],this.id_)?delete k[y][this.id_]:!1};return h},"es6","es3");g.MapEntry=function(){}; | ||
g.polyfill("Map",function(e){function h(){var n={};return n.previous=n.next=n.head=n}function l(n,k){var p=n.head_;return g.iteratorPrototype(function(){if(p){for(;p.head!=n.head_;)p=p.previous;for(;p.next!=p.head;)return p=p.next,{done:!1,value:k(p)};p=null}return{done:!0,value:void 0}})}function m(n,k){var p=k&&typeof k;"object"==p||"function"==p?x.has(k)?p=x.get(k):(p=""+ ++y,x.set(k,p)):p="p_"+k;var u=n.data_[p];if(u&&g.owns(n.data_,p))for(n=0;n<u.length;n++){var C=u[n];if(k!==k&&C.key!==C.key|| | ||
k===C.key)return{id:p,list:u,index:n,entry:C}}return{id:p,list:u,index:-1,entry:void 0}}function t(n){this.data_={};this.head_=h();this.size=0;if(n){n=g.makeIterator(n);for(var k;!(k=n.next()).done;)k=k.value,this.set(k[0],k[1])}}function w(){if(g.ASSUME_NO_NATIVE_MAP||!e||"function"!=typeof e||!e.prototype.entries||"function"!=typeof Object.seal)return!1;try{var n=Object.seal({x:4}),k=new e(g.makeIterator([[n,"s"]]));if("s"!=k.get(n)||1!=k.size||k.get({x:4})||k.set({x:4},"t")!=k||2!=k.size)return!1; | ||
var p=k.entries(),u=p.next();if(u.done||u.value[0]!=n||"s"!=u.value[1])return!1;u=p.next();return u.done||4!=u.value[0].x||"t"!=u.value[1]||!p.next().done?!1:!0}catch(C){return!1}}if(g.USE_PROXY_FOR_ES6_CONFORMANCE_CHECKS){if(e&&g.ES6_CONFORMANCE)return e}else if(w())return e;var x=new WeakMap;t.prototype.set=function(n,k){n=0===n?0:n;var p=m(this,n);p.list||(p.list=this.data_[p.id]=[]);p.entry?p.entry.value=k:(p.entry={next:this.head_,previous:this.head_.previous,head:this.head_,key:n,value:k},p.list.push(p.entry), | ||
this.head_.previous.next=p.entry,this.head_.previous=p.entry,this.size++);return this};t.prototype.delete=function(n){n=m(this,n);return n.entry&&n.list?(n.list.splice(n.index,1),n.list.length||delete this.data_[n.id],n.entry.previous.next=n.entry.next,n.entry.next.previous=n.entry.previous,n.entry.head=null,this.size--,!0):!1};t.prototype.clear=function(){this.data_={};this.head_=this.head_.previous=h();this.size=0};t.prototype.has=function(n){return!!m(this,n).entry};t.prototype.get=function(n){return(n= | ||
m(this,n).entry)&&n.value};t.prototype.entries=function(){return l(this,function(n){return[n.key,n.value]})};t.prototype.keys=function(){return l(this,function(n){return n.key})};t.prototype.values=function(){return l(this,function(n){return n.value})};t.prototype.forEach=function(n,k){for(var p=this.entries(),u;!(u=p.next()).done;)u=u.value,n.call(k,u[1],u[0],this)};t.prototype[Symbol.iterator]=t.prototype.entries;var y=0;return t},"es6","es3"); | ||
g.checkStringArgs=function(e,h,l){if(null==e)throw new TypeError("The 'this' value for String.prototype."+l+" must not be null or undefined");if(h instanceof RegExp)throw new TypeError("First argument to String.prototype."+l+" must not be a regular expression");return e+""}; | ||
g.polyfill("String.prototype.endsWith",function(e){return e?e:function(h,l){var m=g.checkStringArgs(this,h,"endsWith");h+="";void 0===l&&(l=m.length);l=Math.max(0,Math.min(l|0,m.length));for(var t=h.length;0<t&&0<l;)if(m[--l]!=h[--t])return!1;return 0>=t}},"es6","es3");g.polyfill("Number.isNaN",function(e){return e?e:function(h){return"number"===typeof h&&isNaN(h)}},"es6","es3"); | ||
g.polyfill("String.prototype.startsWith",function(e){return e?e:function(h,l){var m=g.checkStringArgs(this,h,"startsWith");h+="";var t=m.length,w=h.length;l=Math.max(0,Math.min(l|0,m.length));for(var x=0;x<w&&l<t;)if(m[l++]!=h[x++])return!1;return x>=w}},"es6","es3");g.polyfill("Object.entries",function(e){return e?e:function(h){var l=[],m;for(m in h)g.owns(h,m)&&l.push([m,h[m]]);return l}},"es8","es3");var E=this; | ||
function F(){function e(a){this.opts={};this.defaults={};this.messages=Object.assign({},q);this.rules={any:Q,array:R,boolean:S,class:T,custom:U,currency:V,date:W,email:X,enum:Y,equal:Z,forbidden:aa,function:C,multi:u,number:p,object:k,objectID:n,string:y,tuple:x,url:w,uuid:t,mac:m,luhn:l};this.aliases={};this.cache=new Map;if(a){B(this.opts,a);a.defaults&&B(this.defaults,a.defaults);if(a.messages)for(var b in a.messages)this.addMessage(b,a.messages[b]);if(a.aliases)for(var c in a.aliases)this.alias(c, | ||
a.aliases[c]);if(a.customRules)for(var d in a.customRules)this.add(d,a.customRules[d]);if(a.plugins){a=a.plugins;if(!Array.isArray(a))throw Error("Plugins type must be array");a.forEach(this.plugin.bind(this))}this.opts.debug&&(a=function(f){return f},"undefined"===typeof window&&(a=h),this._formatter=a)}}function h(a){G||(G=K(),L={parser:"babel",useTabs:!1,printWidth:120,trailingComma:"none",tabWidth:4,singleQuote:!1,semi:!0,bracketSpacing:!0},H=K(),M={language:"js",theme:H.fromJson({keyword:["white", | ||
"bold"],built_in:"magenta",literal:"cyan",number:"magenta",regexp:"red",string:["yellow","bold"],symbol:"plain",class:"blue",attr:"plain",function:["white","bold"],title:"plain",params:"green",comment:"grey"})});a=G.format(a,L);return H.highlight(a,M)}function l(a){a.schema;a=a.messages;return{source:'\n\t\t\tif (typeof value !== "string") {\n\t\t\t\t'+this.makeError({type:"string",actual:"value",messages:a})+'\n\t\t\t\treturn value;\n\t\t\t}\n\n\t\t\tif (typeof value !== "string")\n\t\t\t\tvalue = String(value);\n\n\t\t\tval = value.replace(/\\D+/g, "");\n\n\t\t\tvar array = [0, 2, 4, 6, 8, 1, 3, 5, 7, 9];\n\t\t\tvar len = val ? val.length : 0,\n\t\t\t\tbit = 1,\n\t\t\t\tsum = 0;\n\t\t\twhile (len--) {\n\t\t\t\tsum += !(bit ^= 1) ? parseInt(val[len], 10) : array[val[len]];\n\t\t\t}\n\n\t\t\tif (!(sum % 10 === 0 && sum > 0)) {\n\t\t\t\t'+ | ||
this.makeError({type:"luhn",actual:"value",messages:a})+"\n\t\t\t}\n\n\t\t\treturn value;\n\t\t"}}function m(a){a.schema;a=a.messages;return{source:'\n\t\t\tif (typeof value !== "string") {\n\t\t\t\t'+this.makeError({type:"string",actual:"value",messages:a})+"\n\t\t\t\treturn value;\n\t\t\t}\n\n\t\t\tvar v = value.toLowerCase();\n\t\t\tif (!"+ba.toString()+".test(v)) {\n\t\t\t\t"+this.makeError({type:"mac",actual:"value",messages:a})+"\n\t\t\t}\n\t\t\t\n\t\t\treturn value;\n\t\t"}}function t(a){var b= | ||
a.schema;a=a.messages;var c=[];c.push('\n\t\tif (typeof value !== "string") {\n\t\t\t'+this.makeError({type:"string",actual:"value",messages:a})+"\n\t\t\treturn value;\n\t\t}\n\n\t\tvar val = value.toLowerCase();\n\t\tif (!"+ca.toString()+".test(val)) {\n\t\t\t"+this.makeError({type:"uuid",actual:"value",messages:a})+"\n\t\t\treturn value;\n\t\t}\n\n\t\tconst version = val.charAt(14) | 0;\n\t");7>parseInt(b.version)&&c.push("\n\t\t\tif ("+b.version+" !== version) {\n\t\t\t\t"+this.makeError({type:"uuidVersion", | ||
expected:b.version,actual:"version",messages:a})+"\n\t\t\t\treturn value;\n\t\t\t}\n\t\t");c.push('\n\t\tswitch (version) {\n\t\tcase 0:\n\t\tcase 1:\n\t\tcase 2:\n\t\tcase 6:\n\t\t\tbreak;\n\t\tcase 3:\n\t\tcase 4:\n\t\tcase 5:\n\t\t\tif (["8", "9", "a", "b"].indexOf(val.charAt(19)) === -1) {\n\t\t\t\t'+this.makeError({type:"uuid",actual:"value",messages:a})+"\n\t\t\t}\n\t\t}\n\n\t\treturn value;\n\t");return{source:c.join("\n")}}function w(a){var b=a.schema;a=a.messages;var c=[];c.push('\n\t\tif (typeof value !== "string") {\n\t\t\t'+ | ||
this.makeError({type:"string",actual:"value",messages:a})+"\n\t\t\treturn value;\n\t\t}\n\t");b.empty?c.push("\n\t\t\tif (value.length === 0) return value;\n\t\t"):c.push("\n\t\t\tif (value.length === 0) {\n\t\t\t\t"+this.makeError({type:"urlEmpty",actual:"value",messages:a})+"\n\t\t\t\treturn value;\n\t\t\t}\n\t\t");c.push("\n\t\tif (!"+da.toString()+".test(value)) {\n\t\t\t"+this.makeError({type:"url",actual:"value",messages:a})+"\n\t\t}\n\n\t\treturn value;\n\t");return{source:c.join("\n")}}function x(a, | ||
b,c){var d=a.schema,f=a.messages;a=[];if(null!=d.items){if(!Array.isArray(d.items))throw Error("Invalid '"+d.type+"' schema. The 'items' field must be an array.");if(0===d.items.length)throw Error("Invalid '"+d.type+"' schema. The 'items' field must not be an empty array.");}a.push("\n\t\tif (!Array.isArray(value)) {\n\t\t\t"+this.makeError({type:"tuple",actual:"value",messages:f})+"\n\t\t\treturn value;\n\t\t}\n\n\t\tvar len = value.length;\n\t");!1===d.empty&&a.push("\n\t\t\tif (len === 0) {\n\t\t\t\t"+ | ||
this.makeError({type:"tupleEmpty",actual:"value",messages:f})+"\n\t\t\t\treturn value;\n\t\t\t}\n\t\t");if(null!=d.items){a.push("\n\t\t\tif ("+d.empty+" !== false && len === 0) {\n\t\t\t\treturn value;\n\t\t\t}\n\n\t\t\tif (len !== "+d.items.length+") {\n\t\t\t\t"+this.makeError({type:"tupleLength",expected:d.items.length,actual:"len",messages:f})+"\n\t\t\t\treturn value;\n\t\t\t}\n\t\t");a.push("\n\t\t\tvar arr = value;\n\t\t\tvar parentField = field;\n\t\t");for(f=0;f<d.items.length;f++){a.push("\n\t\t\tvalue = arr["+ | ||
f+"];\n\t\t");var r=b+"["+f+"]",v=this.getRuleFromSchema(d.items[f]);a.push(this.compileRule(v,c,r,"\n\t\t\tarr["+f+"] = "+(c.async?"await ":"")+"context.fn[%%INDEX%%](arr["+f+'], (parentField ? parentField : "") + "[" + '+f+' + "]", parent, errors, context);\n\t\t',"arr["+f+"]"))}a.push("\n\t\treturn arr;\n\t")}else a.push("\n\t\treturn value;\n\t");return{source:a.join("\n")}}function y(a){var b=a.schema;a=a.messages;var c=[],d=!1;!0===b.convert&&(d=!0,c.push('\n\t\t\tif (typeof value !== "string") {\n\t\t\t\tvalue = String(value);\n\t\t\t}\n\t\t')); | ||
c.push('\n\t\tif (typeof value !== "string") {\n\t\t\t'+this.makeError({type:"string",actual:"value",messages:a})+"\n\t\t\treturn value;\n\t\t}\n\n\t\tvar origValue = value;\n\t");b.trim&&(d=!0,c.push("\n\t\t\tvalue = value.trim();\n\t\t"));b.trimLeft&&(d=!0,c.push("\n\t\t\tvalue = value.trimLeft();\n\t\t"));b.trimRight&&(d=!0,c.push("\n\t\t\tvalue = value.trimRight();\n\t\t"));b.padStart&&(d=!0,c.push("\n\t\t\tvalue = value.padStart("+b.padStart+", "+JSON.stringify(null!=b.padChar?b.padChar:" ")+ | ||
");\n\t\t"));b.padEnd&&(d=!0,c.push("\n\t\t\tvalue = value.padEnd("+b.padEnd+", "+JSON.stringify(null!=b.padChar?b.padChar:" ")+");\n\t\t"));b.lowercase&&(d=!0,c.push("\n\t\t\tvalue = value.toLowerCase();\n\t\t"));b.uppercase&&(d=!0,c.push("\n\t\t\tvalue = value.toUpperCase();\n\t\t"));b.localeLowercase&&(d=!0,c.push("\n\t\t\tvalue = value.toLocaleLowerCase();\n\t\t"));b.localeUppercase&&(d=!0,c.push("\n\t\t\tvalue = value.toLocaleUpperCase();\n\t\t"));c.push("\n\t\t\tvar len = value.length;\n\t"); | ||
!1===b.empty&&c.push("\n\t\t\tif (len === 0) {\n\t\t\t\t"+this.makeError({type:"stringEmpty",actual:"value",messages:a})+"\n\t\t\t}\n\t\t");null!=b.min&&c.push("\n\t\t\tif (len < "+b.min+") {\n\t\t\t\t"+this.makeError({type:"stringMin",expected:b.min,actual:"len",messages:a})+"\n\t\t\t}\n\t\t");null!=b.max&&c.push("\n\t\t\tif (len > "+b.max+") {\n\t\t\t\t"+this.makeError({type:"stringMax",expected:b.max,actual:"len",messages:a})+"\n\t\t\t}\n\t\t");null!=b.length&&c.push("\n\t\t\tif (len !== "+b.length+ | ||
") {\n\t\t\t\t"+this.makeError({type:"stringLength",expected:b.length,actual:"len",messages:a})+"\n\t\t\t}\n\t\t");if(null!=b.pattern){var f=b.pattern;"string"==typeof b.pattern&&(f=new RegExp(b.pattern,b.patternFlags));f="\n\t\t\tif (!"+f.toString()+".test(value))\n\t\t\t\t"+this.makeError({type:"stringPattern",expected:'"'+f.toString().replace(/"/g,"\\$&")+'"',actual:"origValue",messages:a})+"\n\t\t";c.push("\n\t\t\tif ("+b.empty+" === true && len === 0) {\n\t\t\t\t// Do nothing\n\t\t\t} else {\n\t\t\t\t"+ | ||
f+"\n\t\t\t}\n\t\t")}null!=b.contains&&c.push('\n\t\t\tif (value.indexOf("'+b.contains+'") === -1) {\n\t\t\t\t'+this.makeError({type:"stringContains",expected:'"'+b.contains+'"',actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");null!=b.enum&&(f=JSON.stringify(b.enum),c.push("\n\t\t\tif ("+f+".indexOf(value) === -1) {\n\t\t\t\t"+this.makeError({type:"stringEnum",expected:'"'+b.enum.join(", ")+'"',actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t"));!0===b.numeric&&c.push("\n\t\t\tif (!"+ea.toString()+ | ||
".test(value) ) {\n\t\t\t\t"+this.makeError({type:"stringNumeric",actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");!0===b.alpha&&c.push("\n\t\t\tif(!"+fa.toString()+".test(value)) {\n\t\t\t\t"+this.makeError({type:"stringAlpha",actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");!0===b.alphanum&&c.push("\n\t\t\tif(!"+ha.toString()+".test(value)) {\n\t\t\t\t"+this.makeError({type:"stringAlphanum",actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");!0===b.alphadash&&c.push("\n\t\t\tif(!"+ia.toString()+ | ||
".test(value)) {\n\t\t\t\t"+this.makeError({type:"stringAlphadash",actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");!0===b.hex&&c.push("\n\t\t\tif(value.length % 2 !== 0 || !"+ja.toString()+".test(value)) {\n\t\t\t\t"+this.makeError({type:"stringHex",actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");!0===b.singleLine&&c.push('\n\t\t\tif(value.includes("\\n")) {\n\t\t\t\t'+this.makeError({type:"stringSingleLine",messages:a})+"\n\t\t\t}\n\t\t");!0===b.base64&&c.push("\n\t\t\tif(!"+ka.toString()+ | ||
".test(value)) {\n\t\t\t\t"+this.makeError({type:"stringBase64",actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");c.push("\n\t\treturn value;\n\t");return{sanitized:d,source:c.join("\n")}}function n(a,b,c){b=a.schema;var d=a.messages;a=a.index;var f=[];c.customs[a]?c.customs[a].schema=b:c.customs[a]={schema:b};f.push("\n\t\tconst ObjectID = context.customs["+a+"].schema.ObjectID;\n\t\tif (!ObjectID.isValid(value)) {\n\t\t\t"+this.makeError({type:"objectID",actual:"value",messages:d})+"\n\t\t\treturn;\n\t\t}\n\t"); | ||
!0===b.convert?f.push("return new ObjectID(value)"):"hexString"===b.convert?f.push("return value.toString()"):f.push("return value");return{source:f.join("\n")}}function k(a,b,c){var d=a.schema;a=a.messages;var f=[];f.push('\n\t\tif (typeof value !== "object" || value === null || Array.isArray(value)) {\n\t\t\t'+this.makeError({type:"object",actual:"value",messages:a})+"\n\t\t\treturn value;\n\t\t}\n\t");var r=d.properties||d.props;if(r){f.push("var parentObj = value;");f.push("var parentField = field;"); | ||
for(var v=Object.keys(r),z=0;z<v.length;z++){var A=v[z],D=N(A),O=la.test(D)?"."+D:"['"+D+"']",I="parentObj"+O,P=(b?b+".":"")+A;f.push("\n// Field: "+N(P));f.push('field = parentField ? parentField + "'+O+'" : "'+D+'";');f.push("value = "+I+";");A=this.getRuleFromSchema(r[A]);f.push(this.compileRule(A,c,P,"\n\t\t\t\t"+I+" = "+(c.async?"await ":"")+"context.fn[%%INDEX%%](value, field, parentObj, errors, context);\n\t\t\t",I))}d.strict&&(f.push("\n\t\t\t\tif (errors.length === 0) {\n\t\t\t"),b=Object.keys(r), | ||
f.push("\n\t\t\t\t\tfield = parentField;\n\t\t\t\t\tvar invalidProps = [];\n\t\t\t\t\tvar props = Object.keys(parentObj);\n\n\t\t\t\t\tfor (let i = 0; i < props.length; i++) {\n\t\t\t\t\t\tif ("+JSON.stringify(b)+".indexOf(props[i]) === -1) {\n\t\t\t\t\t\t\tinvalidProps.push(props[i]);\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t\tif (invalidProps.length) {\n\t\t\t"),"remove"==d.strict?f.push("\n\t\t\t\t\t\tinvalidProps.forEach(function(field) {\n\t\t\t\t\t\t\tdelete parentObj[field];\n\t\t\t\t\t\t});\n\t\t\t\t"): | ||
f.push("\n\t\t\t\t\t"+this.makeError({type:"objectStrict",expected:'"'+b.join(", ")+'"',actual:"invalidProps.join(', ')",messages:a})+"\n\t\t\t\t"),f.push("\n\t\t\t\t\t}\n\t\t\t"),f.push("\n\t\t\t\t}\n\t\t\t"))}if(null!=d.minProps||null!=d.maxProps)d.strict?f.push("\n\t\t\t\tprops = Object.keys("+(r?"parentObj":"value")+");\n\t\t\t"):f.push("\n\t\t\t\tvar props = Object.keys("+(r?"parentObj":"value")+");\n\t\t\t\t"+(r?"field = parentField;":"")+"\n\t\t\t");null!=d.minProps&&f.push("\n\t\t\tif (props.length < "+ | ||
d.minProps+") {\n\t\t\t\t"+this.makeError({type:"objectMinProps",expected:d.minProps,actual:"props.length",messages:a})+"\n\t\t\t}\n\t\t");null!=d.maxProps&&f.push("\n\t\t\tif (props.length > "+d.maxProps+") {\n\t\t\t\t"+this.makeError({type:"objectMaxProps",expected:d.maxProps,actual:"props.length",messages:a})+"\n\t\t\t}\n\t\t");r?f.push("\n\t\t\treturn parentObj;\n\t\t"):f.push("\n\t\t\treturn value;\n\t\t");return{source:f.join("\n")}}function p(a){var b=a.schema;a=a.messages;var c=[];c.push("\n\t\tvar origValue = value;\n\t"); | ||
var d=!1;!0===b.convert&&(d=!0,c.push('\n\t\t\tif (typeof value !== "number") {\n\t\t\t\tvalue = Number(value);\n\t\t\t}\n\t\t'));c.push('\n\t\tif (typeof value !== "number" || isNaN(value) || !isFinite(value)) {\n\t\t\t'+this.makeError({type:"number",actual:"origValue",messages:a})+"\n\t\t\treturn value;\n\t\t}\n\t");null!=b.min&&c.push("\n\t\t\tif (value < "+b.min+") {\n\t\t\t\t"+this.makeError({type:"numberMin",expected:b.min,actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");null!=b.max&&c.push("\n\t\t\tif (value > "+ | ||
b.max+") {\n\t\t\t\t"+this.makeError({type:"numberMax",expected:b.max,actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");null!=b.equal&&c.push("\n\t\t\tif (value !== "+b.equal+") {\n\t\t\t\t"+this.makeError({type:"numberEqual",expected:b.equal,actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");null!=b.notEqual&&c.push("\n\t\t\tif (value === "+b.notEqual+") {\n\t\t\t\t"+this.makeError({type:"numberNotEqual",expected:b.notEqual,actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");!0===b.integer&&c.push("\n\t\t\tif (value % 1 !== 0) {\n\t\t\t\t"+ | ||
this.makeError({type:"numberInteger",actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");!0===b.positive&&c.push("\n\t\t\tif (value <= 0) {\n\t\t\t\t"+this.makeError({type:"numberPositive",actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");!0===b.negative&&c.push("\n\t\t\tif (value >= 0) {\n\t\t\t\t"+this.makeError({type:"numberNegative",actual:"origValue",messages:a})+"\n\t\t\t}\n\t\t");c.push("\n\t\treturn value;\n\t");return{sanitized:d,source:c.join("\n")}}function u(a,b,c){var d=a.schema;a.messages; | ||
a=[];a.push("\n\t\tvar hasValid = false;\n\t\tvar newVal = value;\n\t\tvar checkErrors = [];\n\t");for(var f=0;f<d.rules.length;f++){a.push("\n\t\t\tif (!hasValid) {\n\t\t\t\tvar _errors = [];\n\t\t");var r=this.getRuleFromSchema(d.rules[f]);a.push(this.compileRule(r,c,b,"var tmpVal = "+(c.async?"await ":"")+"context.fn[%%INDEX%%](value, field, parent, _errors, context);","tmpVal"));a.push("\n\t\t\t\tif (_errors.length == 0) {\n\t\t\t\t\thasValid = true;\n\t\t\t\t\tnewVal = tmpVal;\n\t\t\t\t} else {\n\t\t\t\t\tArray.prototype.push.apply(checkErrors, _errors);\n\t\t\t\t}\n\t\t\t}\n\t\t")}a.push("\n\t\tif (!hasValid) {\n\t\t\tArray.prototype.push.apply(errors, checkErrors);\n\t\t}\n\n\t\treturn newVal;\n\t"); | ||
return{source:a.join("\n")}}function C(a){a.schema;return{source:'\n\t\t\tif (typeof value !== "function")\n\t\t\t\t'+this.makeError({type:"function",actual:"value",messages:a.messages})+"\n\n\t\t\treturn value;\n\t\t"}}function aa(a){var b=a.schema;a=a.messages;var c=[];c.push("\n\t\tif (value !== null && value !== undefined) {\n\t");b.remove?c.push("\n\t\t\treturn undefined;\n\t\t"):c.push("\n\t\t\t"+this.makeError({type:"forbidden",actual:"value",messages:a})+"\n\t\t");c.push("\n\t\t}\n\n\t\treturn value;\n\t"); | ||
return{source:c.join("\n")}}function Z(a){var b=a.schema;a=a.messages;var c=[];b.field?(b.strict?c.push('\n\t\t\t\tif (value !== parent["'+b.field+'"])\n\t\t\t'):c.push('\n\t\t\t\tif (value != parent["'+b.field+'"])\n\t\t\t'),c.push("\n\t\t\t\t"+this.makeError({type:"equalField",actual:"value",expected:JSON.stringify(b.field),messages:a})+"\n\t\t")):(b.strict?c.push("\n\t\t\t\tif (value !== "+JSON.stringify(b.value)+")\n\t\t\t"):c.push("\n\t\t\t\tif (value != "+JSON.stringify(b.value)+")\n\t\t\t"), | ||
c.push("\n\t\t\t\t"+this.makeError({type:"equalValue",actual:"value",expected:JSON.stringify(b.value),messages:a})+"\n\t\t"));c.push("\n\t\treturn value;\n\t");return{source:c.join("\n")}}function Y(a){var b=a.schema;a=a.messages;return{source:"\n\t\t\tif ("+JSON.stringify(b.values||[])+".indexOf(value) === -1)\n\t\t\t\t"+this.makeError({type:"enumValue",expected:'"'+b.values.join(", ")+'"',actual:"value",messages:a})+"\n\t\t\t\n\t\t\treturn value;\n\t\t"}}function X(a){var b=a.schema;a=a.messages; | ||
var c=[],d="precise"==b.mode?ma:na,f=!1;c.push('\n\t\tif (typeof value !== "string") {\n\t\t\t'+this.makeError({type:"string",actual:"value",messages:a})+"\n\t\t\treturn value;\n\t\t}\n\t");b.empty?c.push("\n\t\t\tif (value.length === 0) return value;\n\t\t"):c.push("\n\t\t\tif (value.length === 0) {\n\t\t\t\t"+this.makeError({type:"emailEmpty",actual:"value",messages:a})+"\n\t\t\t\treturn value;\n\t\t\t}\n\t\t");b.normalize&&(f=!0,c.push("\n\t\t\tvalue = value.trim().toLowerCase();\n\t\t"));null!= | ||
b.min&&c.push("\n\t\t\tif (value.length < "+b.min+") {\n\t\t\t\t"+this.makeError({type:"emailMin",expected:b.min,actual:"value.length",messages:a})+"\n\t\t\t}\n\t\t");null!=b.max&&c.push("\n\t\t\tif (value.length > "+b.max+") {\n\t\t\t\t"+this.makeError({type:"emailMax",expected:b.max,actual:"value.length",messages:a})+"\n\t\t\t}\n\t\t");c.push("\n\t\tif (!"+d.toString()+".test(value)) {\n\t\t\t"+this.makeError({type:"email",actual:"value",messages:a})+"\n\t\t}\n\n\t\treturn value;\n\t");return{sanitized:f, | ||
source:c.join("\n")}}function W(a){var b=a.schema;a=a.messages;var c=[],d=!1;c.push("\n\t\tvar origValue = value;\n\t");!0===b.convert&&(d=!0,c.push("\n\t\t\tif (!(value instanceof Date)) {\n\t\t\t\tvalue = new Date(value);\n\t\t\t}\n\t\t"));c.push("\n\t\tif (!(value instanceof Date) || isNaN(value.getTime()))\n\t\t\t"+this.makeError({type:"date",actual:"origValue",messages:a})+"\n\n\t\treturn value;\n\t");return{sanitized:d,source:c.join("\n")}}function V(a){var b=a.schema;a=a.messages;var c=b.currencySymbol|| | ||
null,d=b.thousandSeparator||",",f=b.decimalSeparator||".",r=b.customRegex;b=!b.symbolOptional;b="(?=.*\\d)^(-?~1|~1-?)(([0-9]\\d{0,2}(~2\\d{3})*)|0)?(\\~3\\d{1,2})?$".replace(/~1/g,c?"\\"+c+(b?"":"?"):"").replace("~2",d).replace("~3",f);c=[];c.push("\n\t\tif (!value.match("+(r||new RegExp(b))+")) {\n\t\t\t"+this.makeError({type:"currency",actual:"value",messages:a})+"\n\t\t\treturn value;\n\t\t}\n\n\t\treturn value;\n\t");return{source:c.join("\n")}}function U(a,b,c){var d=[];d.push("\n\t\t"+this.makeCustomValidator({fnName:"check", | ||
path:b,schema:a.schema,messages:a.messages,context:c,ruleIndex:a.index})+"\n\t\treturn value;\n\t");return{source:d.join("\n")}}function T(a,b,c){b=a.schema;var d=a.messages;a=a.index;var f=[],r=b.instanceOf.name?b.instanceOf.name:"<UnknowClass>";c.customs[a]?c.customs[a].schema=b:c.customs[a]={schema:b};f.push("\n\t\tif (!(value instanceof context.customs["+a+"].schema.instanceOf))\n\t\t\t"+this.makeError({type:"classInstanceOf",actual:"value",expected:"'"+r+"'",messages:d})+"\n\t");f.push("\n\t\treturn value;\n\t"); | ||
return{source:f.join("\n")}}function S(a){var b=a.schema;a=a.messages;var c=[],d=!1;c.push("\n\t\tvar origValue = value;\n\t");!0===b.convert&&(d=!0,c.push('\n\t\t\tif (typeof value !== "boolean") {\n\t\t\t\tif (\n\t\t\t\tvalue === 1\n\t\t\t\t|| value === "true"\n\t\t\t\t|| value === "1"\n\t\t\t\t|| value === "on"\n\t\t\t\t) {\n\t\t\t\t\tvalue = true;\n\t\t\t\t} else if (\n\t\t\t\tvalue === 0\n\t\t\t\t|| value === "false"\n\t\t\t\t|| value === "0"\n\t\t\t\t|| value === "off"\n\t\t\t\t) {\n\t\t\t\t\tvalue = false;\n\t\t\t\t}\n\t\t\t}\n\t\t')); | ||
c.push('\n\t\tif (typeof value !== "boolean") {\n\t\t\t'+this.makeError({type:"boolean",actual:"origValue",messages:a})+"\n\t\t}\n\t\t\n\t\treturn value;\n\t");return{sanitized:d,source:c.join("\n")}}function R(a,b,c){var d=a.schema,f=a.messages;a=[];a.push("\n\t\tif (!Array.isArray(value)) {\n\t\t\t"+this.makeError({type:"array",actual:"value",messages:f})+"\n\t\t\treturn value;\n\t\t}\n\n\t\tvar len = value.length;\n\t");!1===d.empty&&a.push("\n\t\t\tif (len === 0) {\n\t\t\t\t"+this.makeError({type:"arrayEmpty", | ||
actual:"value",messages:f})+"\n\t\t\t}\n\t\t");null!=d.min&&a.push("\n\t\t\tif (len < "+d.min+") {\n\t\t\t\t"+this.makeError({type:"arrayMin",expected:d.min,actual:"len",messages:f})+"\n\t\t\t}\n\t\t");null!=d.max&&a.push("\n\t\t\tif (len > "+d.max+") {\n\t\t\t\t"+this.makeError({type:"arrayMax",expected:d.max,actual:"len",messages:f})+"\n\t\t\t}\n\t\t");null!=d.length&&a.push("\n\t\t\tif (len !== "+d.length+") {\n\t\t\t\t"+this.makeError({type:"arrayLength",expected:d.length,actual:"len",messages:f})+ | ||
"\n\t\t\t}\n\t\t");null!=d.contains&&a.push("\n\t\t\tif (value.indexOf("+JSON.stringify(d.contains)+") === -1) {\n\t\t\t\t"+this.makeError({type:"arrayContains",expected:JSON.stringify(d.contains),actual:"value",messages:f})+"\n\t\t\t}\n\t\t");!0===d.unique&&a.push("\n\t\t\tif(len > (new Set(value)).size) {\n\t\t\t\t"+this.makeError({type:"arrayUnique",expected:"Array.from(new Set(value.filter((item, index) => value.indexOf(item) !== index)))",actual:"value",messages:f})+"\n\t\t\t}\n\t\t");if(null!= | ||
d.enum){var r=JSON.stringify(d.enum);a.push("\n\t\t\tfor (var i = 0; i < value.length; i++) {\n\t\t\t\tif ("+r+".indexOf(value[i]) === -1) {\n\t\t\t\t\t"+this.makeError({type:"arrayEnum",expected:'"'+d.enum.join(", ")+'"',actual:"value[i]",messages:f})+"\n\t\t\t\t}\n\t\t\t}\n\t\t")}null!=d.items?(a.push("\n\t\t\tvar arr = value;\n\t\t\tvar parentField = field;\n\t\t\tfor (var i = 0; i < arr.length; i++) {\n\t\t\t\tvalue = arr[i];\n\t\t"),b+="[]",d=this.getRuleFromSchema(d.items),a.push(this.compileRule(d, | ||
c,b,"arr[i] = "+(c.async?"await ":"")+'context.fn[%%INDEX%%](arr[i], (parentField ? parentField : "") + "[" + i + "]", parent, errors, context)',"arr[i]")),a.push("\n\t\t\t}\n\t\t"),a.push("\n\t\treturn arr;\n\t")):a.push("\n\t\treturn value;\n\t");return{source:a.join("\n")}}function Q(){var a=[];a.push("\n\t\treturn value;\n\t");return{source:a.join("\n")}}function oa(a,b,c){return a.replace(b,void 0===c||null===c?"":"function"===typeof c.toString?c:typeof c)}function B(a,b,c){void 0===c&&(c={}); | ||
for(var d in b){var f=b[d];f="object"!==typeof f||Array.isArray(f)||null==f?!1:0<Object.keys(f).length;if(f)a[d]=a[d]||{},B(a[d],b[d],c);else if(!0!==c.skipIfExist||void 0===a[d])a[d]=b[d]}return a}function N(a){return a.replace(pa,function(b){switch(b){case '"':case "'":case "\\":return"\\"+b;case "\n":return"\\n";case "\r":return"\\r";case "\u2028":return"\\u2028";case "\u2029":return"\\u2029"}})}function K(){throw Error("Dynamic requires are not currently supported by rollup-plugin-commonjs"); | ||
}var q={required:"The '{field}' field is required.",string:"The '{field}' field must be a string.",stringEmpty:"The '{field}' field must not be empty.",stringMin:"The '{field}' field length must be greater than or equal to {expected} characters long.",stringMax:"The '{field}' field length must be less than or equal to {expected} characters long.",stringLength:"The '{field}' field length must be {expected} characters long.",stringPattern:"The '{field}' field fails to match the required pattern.",stringContains:"The '{field}' field must contain the '{expected}' text.", | ||
stringEnum:"The '{field}' field does not match any of the allowed values.",stringNumeric:"The '{field}' field must be a numeric string.",stringAlpha:"The '{field}' field must be an alphabetic string.",stringAlphanum:"The '{field}' field must be an alphanumeric string.",stringAlphadash:"The '{field}' field must be an alphadash string.",stringHex:"The '{field}' field must be a hex string.",stringSingleLine:"The '{field}' field must be a single line string.",stringBase64:"The '{field}' field must be a base64 string.", | ||
number:"The '{field}' field must be a number.",numberMin:"The '{field}' field must be greater than or equal to {expected}.",numberMax:"The '{field}' field must be less than or equal to {expected}.",numberEqual:"The '{field}' field must be equal to {expected}.",numberNotEqual:"The '{field}' field can't be equal to {expected}.",numberInteger:"The '{field}' field must be an integer.",numberPositive:"The '{field}' field must be a positive number.",numberNegative:"The '{field}' field must be a negative number.", | ||
array:"The '{field}' field must be an array.",arrayEmpty:"The '{field}' field must not be an empty array.",arrayMin:"The '{field}' field must contain at least {expected} items.",arrayMax:"The '{field}' field must contain less than or equal to {expected} items.",arrayLength:"The '{field}' field must contain {expected} items.",arrayContains:"The '{field}' field must contain the '{expected}' item.",arrayUnique:"The '{actual}' value in '{field}' field does not unique the '{expected}' values.",arrayEnum:"The '{actual}' value in '{field}' field does not match any of the '{expected}' values.", | ||
tuple:"The '{field}' field must be an array.",tupleEmpty:"The '{field}' field must not be an empty array.",tupleLength:"The '{field}' field must contain {expected} items.",boolean:"The '{field}' field must be a boolean.",currency:"The '{field}' must be a valid currency format",date:"The '{field}' field must be a Date.",dateMin:"The '{field}' field must be greater than or equal to {expected}.",dateMax:"The '{field}' field must be less than or equal to {expected}.",enumValue:"The '{field}' field value '{expected}' does not match any of the allowed values.", | ||
equalValue:"The '{field}' field value must be equal to '{expected}'.",equalField:"The '{field}' field value must be equal to '{expected}' field value.",forbidden:"The '{field}' field is forbidden.",function:"The '{field}' field must be a function.",email:"The '{field}' field must be a valid e-mail.",emailEmpty:"The '{field}' field must not be empty.",emailMin:"The '{field}' field length must be greater than or equal to {expected} characters long.",emailMax:"The '{field}' field length must be less than or equal to {expected} characters long.", | ||
luhn:"The '{field}' field must be a valid checksum luhn.",mac:"The '{field}' field must be a valid MAC address.",object:"The '{field}' must be an Object.",objectStrict:"The object '{field}' contains forbidden keys: '{actual}'.",objectMinProps:"The object '{field}' must contain at least {expected} properties.",objectMaxProps:"The object '{field}' must contain {expected} properties at most.",url:"The '{field}' field must be a valid URL.",urlEmpty:"The '{field}' field must not be empty.",uuid:"The '{field}' field must be a valid UUID.", | ||
uuidVersion:"The '{field}' field must be a valid UUID version provided.",classInstanceOf:"The '{field}' field must be an instance of the '{expected}' class.",objectID:"The '{field}' field must be an valid ObjectID"};q.required;q.string;q.stringEmpty;q.stringMin;q.stringMax;q.stringLength;q.stringPattern;q.stringContains;q.stringEnum;q.stringNumeric;q.stringAlpha;q.stringAlphanum;q.stringAlphadash;q.stringHex;q.stringSingleLine;q.stringBase64;q.number;q.numberMin;q.numberMax;q.numberEqual;q.numberNotEqual; | ||
q.numberInteger;q.numberPositive;q.numberNegative;q.array;q.arrayEmpty;q.arrayMin;q.arrayMax;q.arrayLength;q.arrayContains;q.arrayUnique;q.arrayEnum;q.tuple;q.tupleEmpty;q.tupleLength;q.currency;q.date;q.dateMin;q.dateMax;q.enumValue;q.equalValue;q.equalField;q.forbidden;q.email;q.emailEmpty;q.emailMin;q.emailMax;q.luhn;q.mac;q.object;q.objectStrict;q.objectMinProps;q.objectMaxProps;q.url;q.urlEmpty;q.uuid;q.uuidVersion;q.classInstanceOf;q.objectID;var ma=/^(([^<>()[\]\\.,;:\s@"]+(\.[^<>()[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/, | ||
na=/^\S+@\S+\.\S+$/,la=/^[_$a-zA-Z][_$a-zA-Z0-9]*$/,pa=/["'\\\n\r\u2028\u2029]/g,ea=/^-?[0-9]\d*(\.\d+)?$/,fa=/^[a-zA-Z]+$/,ha=/^[a-zA-Z0-9]+$/,ia=/^[a-zA-Z0-9_-]+$/,ja=/^[0-9a-fA-F]+$/,ka=/^(?:[A-Za-z0-9+\\/]{4})*(?:[A-Za-z0-9+\\/]{2}==|[A-Za-z0-9+/]{3}=)?$/,da=/^https?:\/\/\S+/,ca=/^([0-9a-f]{8}-[0-9a-f]{4}-[1-6][0-9a-f]{3}-[0-9a-f]{4}-[0-9a-f]{12}|[0]{8}-[0]{4}-[0]{4}-[0]{4}-[0]{12})$/i,ba=/^((([a-f0-9][a-f0-9]+[-]){5}|([a-f0-9][a-f0-9]+[:]){5})([a-f0-9][a-f0-9])$)|(^([a-f0-9][a-f0-9][a-f0-9][a-f0-9]+[.]){2}([a-f0-9][a-f0-9][a-f0-9][a-f0-9]))$/i, | ||
G,L,H,M;try{var J=(new Function("return Object.getPrototypeOf(async function(){}).constructor"))()}catch(a){}e.prototype.validate=function(a,b){return this.compile(b)(a)};e.prototype.wrapRequiredCheckSourceCode=function(a,b,c,d){var f=[],r=!0===a.schema.optional||"forbidden"===a.schema.type,v=!0===a.schema.optional||!0===a.schema.nullable||"forbidden"===a.schema.type;null!=a.schema.default?(r=!1,!0!==a.schema.nullable&&(v=!1),"function"===typeof a.schema.default?(c.customs[a.index]||(c.customs[a.index]= | ||
{}),c.customs[a.index].defaultFn=a.schema.default,a="context.customs["+a.index+"].defaultFn.call(this, context.rules["+a.index+"].schema, field, parent, context)"):a=JSON.stringify(a.schema.default),d="\n\t\t\t\tvalue = "+a+";\n\t\t\t\t"+d+" = value;\n\t\t\t"):d=this.makeError({type:"required",actual:"value",messages:a.messages});f.push("\n\t\t\tif (value === undefined) { "+((r?"\n// allow undefined\n":d)+" }\n\t\t\telse if (value === null) { ")+((v?"\n// allow null\n":d)+" }\n\t\t\t")+(b?"else { "+ | ||
b+" }":"")+"\n\t\t");return f.join("\n")};e.prototype.compile=function(a){function b(v,z){d.data=v;z&&z.meta&&(d.meta=z.meta);return r.call(c,v,d)}if(null===a||"object"!==typeof a)throw Error("Invalid schema.");var c=this,d={index:0,async:!0===a.$$async,rules:[],fn:[],customs:{},utils:{replace:oa}};this.cache.clear();delete a.$$async;if(d.async&&!J)throw Error("Asynchronous mode is not supported.");if(!0!==a.$$root)if(Array.isArray(a))a=this.getRuleFromSchema(a).schema;else{var f=Object.assign({}, | ||
a);a={type:"object",strict:f.$$strict,properties:f};delete f.$$strict}f=["var errors = [];","var field;","var parent = null;"];a=this.getRuleFromSchema(a);f.push(this.compileRule(a,d,null,(d.async?"await ":"")+"context.fn[%%INDEX%%](value, field, null, errors, context);","value"));f.push("if (errors.length) {");f.push("\n\t\t\treturn errors.map(err => {\n\t\t\t\tif (err.message) {\n\t\t\t\t\terr.message = context.utils.replace(err.message, /\\{field\\}/g, err.field);\n\t\t\t\t\terr.message = context.utils.replace(err.message, /\\{expected\\}/g, err.expected);\n\t\t\t\t\terr.message = context.utils.replace(err.message, /\\{actual\\}/g, err.actual);\n\t\t\t\t}\n\n\t\t\t\treturn err;\n\t\t\t});\n\t\t"); | ||
f.push("}");f.push("return true;");a=f.join("\n");var r=new (d.async?J:Function)("value","context",a);this.opts.debug&&console.log(this._formatter("// Main check function\n"+r.toString()));this.cache.clear();b.async=d.async;return b};e.prototype.compileRule=function(a,b,c,d,f){var r=[],v=this.cache.get(a.schema);v?(a=v,a.cycle=!0,a.cycleStack=[],r.push(this.wrapRequiredCheckSourceCode(a,"\n\t\t\t\tvar rule = context.rules["+a.index+"];\n\t\t\t\tif (rule.cycleStack.indexOf(value) === -1) {\n\t\t\t\t\trule.cycleStack.push(value);\n\t\t\t\t\t"+ | ||
d.replace(/%%INDEX%%/g,a.index)+"\n\t\t\t\t\trule.cycleStack.pop(value);\n\t\t\t\t}\n\t\t\t",b,f))):(this.cache.set(a.schema,a),a.index=b.index,b.rules[b.index]=a,v=null!=c?c:"$$root",b.index++,c=a.ruleFunction.call(this,a,c,b),c.source=c.source.replace(/%%INDEX%%/g,a.index),c=new (b.async?J:Function)("value","field","parent","errors","context",c.source),b.fn[a.index]=c.bind(this),r.push(this.wrapRequiredCheckSourceCode(a,d.replace(/%%INDEX%%/g,a.index),b,f)),r.push(this.makeCustomValidator({vName:f, | ||
path:v,schema:a.schema,context:b,messages:a.messages,ruleIndex:a.index})),this.opts.debug&&console.log(this._formatter("// Context.fn["+a.index+"]\n"+c.toString())));return r.join("\n")};e.prototype.getRuleFromSchema=function(a){a=this.resolveType(a);var b=this.aliases[a.type];b&&(delete a.type,a=B(a,b,{skipIfExist:!0}));b=this.rules[a.type];if(!b)throw Error("Invalid '"+a.type+"' type in validator schema.");return{messages:Object.assign({},this.messages,a.messages),schema:B(a,this.defaults[a.type], | ||
{skipIfExist:!0}),ruleFunction:b}};e.prototype.parseShortHand=function(a){a=a.split("|").map(function(d){return d.trim()});var b=a[0];var c=b.endsWith("[]")?this.getRuleFromSchema({type:"array",items:b.slice(0,-2)}).schema:{type:a[0]};a.slice(1).map(function(d){var f=d.indexOf(":");if(-1!==f){var r=d.substr(0,f).trim();d=d.substr(f+1).trim();"true"===d||"false"===d?d="true"===d:Number.isNaN(Number(d))||(d=Number(d));c[r]=d}else d.startsWith("no-")?c[d.slice(3)]=!1:c[d]=!0});return c};e.prototype.makeError= | ||
function(a){var b=a.type,c=a.field,d=a.expected,f=a.actual,r={type:'"'+b+'"',message:'"'+a.messages[b]+'"'};r.field=c?'"'+c+'"':"field";null!=d&&(r.expected=d);null!=f&&(r.actual=f);return"errors.push({ "+Object.keys(r).map(function(v){return v+": "+r[v]}).join(", ")+" });"};e.prototype.makeCustomValidator=function(a){var b=a.vName;void 0===b&&(b="value");var c=a.fnName;void 0===c&&(c="custom");var d=a.ruleIndex,f=a.path,r=a.schema,v=a.context,z=a.messages;a="rule"+d;var A="fnCustomErrors"+d;if("function"== | ||
typeof r[c]){v.customs[d]?(v.customs[d].messages=z,v.customs[d].schema=r):v.customs[d]={messages:z,schema:r};if(this.opts.useNewCustomCheckerFunction)return"\n \t\tconst "+a+" = context.customs["+d+"];\n\t\t\t\t\tconst "+A+" = [];\n\t\t\t\t\t"+b+" = "+(v.async?"await ":"")+a+".schema."+c+".call(this, "+b+", "+A+" , "+a+'.schema, "'+f+'", parent, context);\n\t\t\t\t\tif (Array.isArray('+A+" )) {\n \t\t"+A+" .forEach(err => errors.push(Object.assign({ message: "+a+".messages[err.type], field }, err)));\n\t\t\t\t\t}\n\t\t\t\t"; | ||
r="res_"+a;return"\n\t\t\t\tconst "+a+" = context.customs["+d+"];\n\t\t\t\tconst "+r+" = "+(v.async?"await ":"")+a+".schema."+c+".call(this, "+b+", "+a+'.schema, "'+f+'", parent, context);\n\t\t\t\tif (Array.isArray('+r+")) {\n\t\t\t\t\t"+r+".forEach(err => errors.push(Object.assign({ message: "+a+".messages[err.type], field }, err)));\n\t\t\t\t}\n\t\t"}return""};e.prototype.add=function(a,b){this.rules[a]=b};e.prototype.addMessage=function(a,b){this.messages[a]=b};e.prototype.alias=function(a,b){if(this.rules[a])throw Error("Alias name must not be a rule name"); | ||
this.aliases[a]=b};e.prototype.plugin=function(a){if("function"!==typeof a)throw Error("Plugin fn type must be function");return a(this)};e.prototype.resolveType=function(a){var b=this;if("string"===typeof a)a=this.parseShortHand(a);else if(Array.isArray(a)){if(0===a.length)throw Error("Invalid schema.");a={type:"multi",rules:a};a.rules.map(function(r){return b.getRuleFromSchema(r)}).every(function(r){return!0===r.schema.optional})&&(a.optional=!0)}if(a.$$type){var c=this.getRuleFromSchema(a.$$type).schema; | ||
delete a.$$type;var d=Object.assign({},a),f;for(f in a)delete a[f];B(a,c,{skipIfExist:!0});a.props=d}return a};e.prototype.normalize=function(a){var b=this,c=this.resolveType(a);this.aliases[c.type]&&(c=B(c,this.normalize(this.aliases[c.type]),{skipIfExists:!0}));c=B(c,this.defaults[c.type],{skipIfExist:!0});if("multi"===c.type)return c.rules=c.rules.map(function(d){return b.normalize(d)}),c.optional=c.rules.every(function(d){return!0===d.optional}),c;if("array"===c.type)return c.items=this.normalize(c.items), | ||
c;"object"===c.type&&c.props&&Object.entries(c.props).forEach(function(d){return c.props[d[0]]=b.normalize(d[1])});"object"===typeof a&&(a.type?(a=this.normalize(a.type),B(c,a,{skipIfExists:!0})):Object.entries(a).forEach(function(d){return c[d[0]]=b.normalize(d[1])}));return c};return e}"object"===typeof exports&&"undefined"!==typeof module?module.exports=F():"function"===typeof define&&define.amd?define(F):(E="undefined"!==typeof globalThis?globalThis:E||self,E.FastestValidator=F()) |
@@ -7,2 +7,3 @@ | ||
| "class" | ||
| "currency" | ||
| "custom" | ||
@@ -108,2 +109,37 @@ | "date" | ||
/** | ||
* Validation schema definition for "currency" built-in validator | ||
* @see https://github.com/icebob/fastest-validator#currency | ||
*/ | ||
export interface RuleCurrency extends RuleCustom { | ||
/** | ||
* Name of built-in validator | ||
*/ | ||
type: "currency"; | ||
/** | ||
* The currency symbol expected in string (as prefix) | ||
* @default null | ||
*/ | ||
currencySymbol?: string; | ||
/** | ||
* Toggle to make the currency symbol optional in string | ||
* @default false | ||
*/ | ||
symbolOptional?: boolean; | ||
/** | ||
* Thousand place separator character | ||
* @default ',' | ||
*/ | ||
thousandSeparator?: string; | ||
/** | ||
* Decimal place character | ||
* @default '.' | ||
*/ | ||
decimalSeparator?: string; | ||
/** | ||
* Custom regular expression to validate currency strings | ||
*/ | ||
customRegex?: RegExp | string; | ||
} | ||
/** | ||
* Validation schema definition for "date" built-in validator | ||
@@ -386,3 +422,3 @@ * @see https://github.com/icebob/fastest-validator#date | ||
*/ | ||
contains?: string[]; | ||
contains?: string; | ||
/** | ||
@@ -393,7 +429,7 @@ * The value must be an element of the enum array | ||
/** | ||
* The value must be an alphabetic string | ||
* The value must be a numeric string | ||
*/ | ||
numeric?: boolean; | ||
/** | ||
* The value must be a numeric string | ||
* The value must be an alphabetic string | ||
*/ | ||
@@ -761,2 +797,3 @@ alpha?: boolean; | ||
| RuleClass | ||
| RuleCurrency | ||
| RuleDate | ||
@@ -1055,2 +1092,15 @@ | RuleEmail | ||
}; | ||
/** | ||
* Normalize a schema, type or short hand definition by expanding it to a full form. The 'normalized' | ||
* form is the equivalent schema with any short hands undone. This ensure that each rule; always includes | ||
* a 'type' key, arrays always have an 'items' key, 'multi' always have a 'rules' key and objects always | ||
* have their properties defined in a 'props' key | ||
* | ||
* @param { ValidationSchema | string | any } value The value to normalize | ||
* @return {ValidationRule | ValidationSchema } The normalized form of the given rule or schema | ||
*/ | ||
normalize( | ||
value: ValidationSchema | string | any | ||
): ValidationRule | ValidationSchema | ||
} |
@@ -9,6 +9,5 @@ "use strict"; | ||
src.push(` | ||
var prevErrLen = errors.length; | ||
var errBefore; | ||
var hasValid = false; | ||
var newVal = value; | ||
var checkErrors = []; | ||
`); | ||
@@ -19,11 +18,13 @@ | ||
if (!hasValid) { | ||
errBefore = errors.length; | ||
var _errors = []; | ||
`); | ||
const rule = this.getRuleFromSchema(schema.rules[i]); | ||
src.push(this.compileRule(rule, context, path, `var tmpVal = ${context.async ? "await " : ""}context.fn[%%INDEX%%](value, field, parent, errors, context);`, "tmpVal")); | ||
src.push(this.compileRule(rule, context, path, `var tmpVal = ${context.async ? "await " : ""}context.fn[%%INDEX%%](value, field, parent, _errors, context);`, "tmpVal")); | ||
src.push(` | ||
if (errors.length == errBefore) { | ||
if (_errors.length == 0) { | ||
hasValid = true; | ||
newVal = tmpVal; | ||
} else { | ||
Array.prototype.push.apply(checkErrors, _errors); | ||
} | ||
@@ -35,4 +36,4 @@ } | ||
src.push(` | ||
if (hasValid) { | ||
errors.length = prevErrLen; | ||
if (!hasValid) { | ||
Array.prototype.push.apply(errors, checkErrors); | ||
} | ||
@@ -39,0 +40,0 @@ |
@@ -72,21 +72,24 @@ "use strict"; | ||
if (schema.strict) { | ||
sourceCode.push(` | ||
if (errors.length === 0) { | ||
`); | ||
const allowedProps = Object.keys(subSchema); | ||
sourceCode.push(` | ||
field = parentField; | ||
var invalidProps = []; | ||
var props = Object.keys(parentObj); | ||
field = parentField; | ||
var invalidProps = []; | ||
var props = Object.keys(parentObj); | ||
for (let i = 0; i < props.length; i++) { | ||
if (${JSON.stringify(allowedProps)}.indexOf(props[i]) === -1) { | ||
invalidProps.push(props[i]); | ||
for (let i = 0; i < props.length; i++) { | ||
if (${JSON.stringify(allowedProps)}.indexOf(props[i]) === -1) { | ||
invalidProps.push(props[i]); | ||
} | ||
} | ||
} | ||
if (invalidProps.length) { | ||
if (invalidProps.length) { | ||
`); | ||
if (schema.strict == "remove") { | ||
sourceCode.push(` | ||
invalidProps.forEach(function(field) { | ||
delete parentObj[field]; | ||
}); | ||
invalidProps.forEach(function(field) { | ||
delete parentObj[field]; | ||
}); | ||
`); | ||
@@ -99,2 +102,5 @@ } else { | ||
sourceCode.push(` | ||
} | ||
`); | ||
sourceCode.push(` | ||
} | ||
@@ -101,0 +107,0 @@ `); |
@@ -129,3 +129,3 @@ "use strict"; | ||
context.customs[rule.index].defaultFn = rule.schema.default; | ||
defaultValue = `context.customs[${rule.index}].defaultFn()`; | ||
defaultValue = `context.customs[${rule.index}].defaultFn.call(this, context.rules[${rule.index}].schema, field, parent, context)`; | ||
} else { | ||
@@ -305,35 +305,4 @@ defaultValue = JSON.stringify(rule.schema.default); | ||
getRuleFromSchema(schema) { | ||
if (typeof schema === "string") { | ||
schema = this.parseShortHand(schema); | ||
} else if (Array.isArray(schema)) { | ||
if (schema.length == 0) | ||
throw new Error("Invalid schema."); | ||
schema = this.resolveType(schema); | ||
schema = { | ||
type: "multi", | ||
rules: schema | ||
}; | ||
// Check 'optional' flag | ||
const isOptional = schema.rules | ||
.map(s => this.getRuleFromSchema(s)) | ||
.every(rule => rule.schema.optional == true); | ||
if (isOptional) | ||
schema.optional = true; | ||
} | ||
if (schema.$$type) { | ||
const type = schema.$$type; | ||
const otherShorthandProps = this.getRuleFromSchema(type).schema; | ||
delete schema.$$type; | ||
const props = Object.assign({}, schema); | ||
for (const key in schema) { // clear object without changing reference | ||
delete schema[key]; | ||
} | ||
deepExtend(schema, otherShorthandProps, { skipIfExist: true }); | ||
schema.props = props; | ||
} | ||
const alias = this.aliases[schema.type]; | ||
@@ -507,4 +476,93 @@ if (alias) { | ||
} | ||
/** | ||
* Resolve the schema 'type' by: | ||
* - parsing short hands into full type definitions | ||
* - expanding arrays into 'multi' types with a rules property | ||
* - objects which have a root $$type property into a schema which | ||
* explicitly has a 'type' property and a 'props' property. | ||
* | ||
* @param schema The schema to resolve the type of | ||
*/ | ||
resolveType(schema) { | ||
if (typeof schema === "string") { | ||
schema = this.parseShortHand(schema); | ||
} else if (Array.isArray(schema)) { | ||
if (schema.length === 0) | ||
throw new Error("Invalid schema."); | ||
schema = { | ||
type: "multi", | ||
rules: schema | ||
}; | ||
// Check 'optional' flag | ||
const isOptional = schema.rules | ||
.map(s => this.getRuleFromSchema(s)) | ||
.every(rule => rule.schema.optional === true); | ||
if (isOptional) | ||
schema.optional = true; | ||
} | ||
if (schema.$$type) { | ||
const type = schema.$$type; | ||
const otherShorthandProps = this.getRuleFromSchema(type).schema; | ||
delete schema.$$type; | ||
const props = Object.assign({}, schema); | ||
for (const key in schema) { // clear object without changing reference | ||
delete schema[key]; | ||
} | ||
deepExtend(schema, otherShorthandProps, { skipIfExist: true }); | ||
schema.props = props; | ||
} | ||
return schema; | ||
} | ||
/** | ||
* Normalize a schema, type or short hand definition by expanding it to a full form. The 'normalized' | ||
* form is the equivalent schema with any short hands undone. This ensure that each rule; always includes | ||
* a 'type' key, arrays always have an 'items' key, 'multi' always have a 'rules' key and objects always | ||
* have their properties defined in a 'props' key | ||
* | ||
* @param {Object|String} value The value to normalize | ||
* @returns {Object} The normalized form of the given rule or schema | ||
*/ | ||
normalize(value) { | ||
let result = this.resolveType(value); | ||
if(this.aliases[result.type]) | ||
result = deepExtend(result, this.normalize(this.aliases[result.type]), { skipIfExists: true}); | ||
result = deepExtend(result, this.defaults[result.type], { skipIfExist: true }); | ||
if(result.type === "multi") { | ||
result.rules = result.rules.map(r => this.normalize(r)); | ||
result.optional = result.rules.every(r => r.optional === true); | ||
return result; | ||
} | ||
if(result.type === "array") { | ||
result.items = this.normalize(result.items); | ||
return result; | ||
} | ||
if(result.type === "object") { | ||
if(result.props) { | ||
Object.entries(result.props).forEach(([k,v]) => result.props[k] = this.normalize(v)); | ||
} | ||
} | ||
if(typeof value === "object") { | ||
if(value.type) { | ||
const config = this.normalize(value.type); | ||
deepExtend(result, config, { skipIfExists: true }); | ||
} | ||
else{ | ||
Object.entries(value).forEach(([k,v]) => result[k] = this.normalize(v)); | ||
} | ||
} | ||
return result; | ||
} | ||
} | ||
module.exports = Validator; |
{ | ||
"name": "fastest-validator", | ||
"version": "1.11.1", | ||
"version": "1.12.0", | ||
"description": "The fastest JS validator library for NodeJS", | ||
@@ -46,24 +46,24 @@ "main": "index.js", | ||
"devDependencies": { | ||
"@ampproject/rollup-plugin-closure-compiler": "^0.26.0", | ||
"@types/jest": "^26.0.10", | ||
"@types/mongodb": "^3.5.26", | ||
"benchmarkify": "^2.1.2", | ||
"cli-highlight": "^2.1.4", | ||
"coveralls": "^3.0.9", | ||
"eslint": "^7.7.0", | ||
"jest": "^26.4.2", | ||
"jest-cli": "^26.4.2", | ||
"mongodb": "^3.6.0", | ||
"nodemon": "^2.0.4", | ||
"@ampproject/rollup-plugin-closure-compiler": "^0.27.0", | ||
"@types/jest": "^27.0.2", | ||
"@types/mongodb": "^4.0.7", | ||
"benchmarkify": "^3.0.0", | ||
"cli-highlight": "^2.1.11", | ||
"coveralls": "^3.1.1", | ||
"eslint": "^8.0.1", | ||
"jest": "^27.2.5", | ||
"jest-cli": "^27.2.5", | ||
"mongodb": "^4.1.3", | ||
"nodemon": "^2.0.13", | ||
"npm-check": "^5.9.0", | ||
"prettier": "^2.1.1", | ||
"rollup": "^1.32.1", | ||
"prettier": "^2.4.1", | ||
"rollup": "^2.58.0", | ||
"rollup-plugin-buble": "^0.19.8", | ||
"rollup-plugin-commonjs": "^10.1.0", | ||
"rollup-plugin-copy": "^3.3.0", | ||
"rollup-plugin-copy": "^3.4.0", | ||
"rollup-plugin-uglify-es": "^0.0.1", | ||
"ts-jest": "^26.3.0", | ||
"ts-node": "^9.0.0", | ||
"tsd": "^0.13.1", | ||
"typescript": "^4.0.2" | ||
"ts-jest": "^27.0.7", | ||
"ts-node": "^10.3.0", | ||
"tsd": "^0.18.0", | ||
"typescript": "^4.4.4" | ||
}, | ||
@@ -82,3 +82,3 @@ "jest": { | ||
"ts-jest": { | ||
"tsConfig": "test/typescript/tsconfig.json", | ||
"tsconfig": "test/typescript/tsconfig.json", | ||
"diagnostics": true | ||
@@ -85,0 +85,0 @@ } |
@@ -25,3 +25,3 @@ 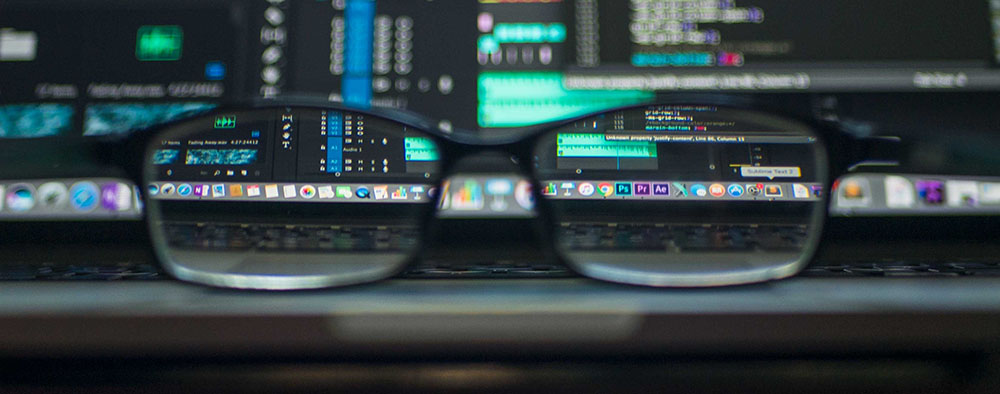 | ||
# How fast? | ||
## How fast? | ||
Very fast! 8 million validations/sec (on Intel i7-4770K, Node.JS: 12.14.1) | ||
@@ -46,5 +46,8 @@ ``` | ||
## Installation | ||
## Approach | ||
In order to achieve lowest cost/highest performance redaction fastest-validator creates and compiles functions using the `Function` constructor. It's important to distinguish this from the dangers of a runtime eval, no user input is involved in creating the validation schema that compiles into the function. This is as safe as writing code normally and having it compiled by V8 in the usual way. | ||
### NPM | ||
# Installation | ||
## NPM | ||
You can install it via [NPM](http://npmjs.org/). | ||
@@ -59,5 +62,5 @@ ``` | ||
## Usage | ||
# Usage | ||
### Validate | ||
## Validate | ||
The first step is to compile the schema to a compiled "checker" function. After that, to validate your object, just call this "checker" function. | ||
@@ -95,3 +98,3 @@ > This method is the fastest. | ||
### Browser usage | ||
## Browser usage | ||
```html | ||
@@ -116,3 +119,3 @@ <script src="https://unpkg.com/fastest-validator"></script> | ||
### Deno usage | ||
## Deno usage | ||
With `esm.sh`, now Typescript is supported | ||
@@ -132,3 +135,3 @@ | ||
### Supported frameworks | ||
## Supported frameworks | ||
- *Moleculer*: Natively supported | ||
@@ -279,3 +282,3 @@ - *Fastify*: By using [fastify-fv](https://github.com/erfanium/fastify-fv) | ||
type: "date", | ||
default: () => new Date() | ||
default: (schema, field, parent, context) => new Date() | ||
} | ||
@@ -1084,3 +1087,3 @@ }; | ||
-------- | -------- | ----------- | ||
`version` | `4` | UUID version in range 0-6. | ||
`version` | `null` | UUID version in range 0-6. The `null` disables version checking. | ||
@@ -1087,0 +1090,0 @@ ## `objectID` |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
375387
5196
1538