fastfall

call your callbacks in a waterfall, without overhead
Benchmark for doing 3 calls setImmediate
100 thousands times:
These benchmarks where taken via bench.js
on node 4.2.2, on a MacBook
Pro Retina 2014 (i7, 16GB of RAM).
If you need zero-overhead series function call, check out
fastseries, for parallel calls check out
fastparallel, and for a fast work queue
use fastq.
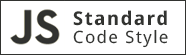
Install
npm install fastfall --save
Usage
var fall = require('fastfall')()
fall([
function a (cb) {
console.log('called a')
cb(null, 'a')
},
function b (a, cb) {
console.log('called b with:', a)
cb(null, 'a', 'b')
},
function c (a, b, cb) {
console.log('called c with:', a, b)
cb(null, 'a', 'b', 'c')
}], function result (err, a, b, c) {
console.log('result arguments', arguments)
})
You can also set this
when you create a fall:
var that = { hello: 'world' }
var fall = require('fastfall')(that)
fall([a, b, c], result)
function a (cb) {
console.log(this)
console.log('called a')
cb(null, 'a')
}
function b (a, cb) {
console.log('called b with:', a)
cb(null, 'a', 'b')
}
function c (a, b, cb) {
console.log('called c with:', a, b)
cb(null, 'a', 'b', 'c')
}
function result (err, a, b, c) {
console.log('result arguments', arguments)
}
You can also set this
when you run a task:
var that = { hello: 'world' }
var fall = require('fastfall')()
fall(new State('world'), [
a, b, c,
], console.log)
function State (value) {
this.value = value
}
function a (cb) {
console.log(this.value)
console.log('called a')
cb(null, 'a')
}
function b (a, cb) {
console.log('called b with:', a)
cb(null, 'a', 'b')
}
function c (a, b, cb) {
console.log('called c with:', a, b)
cb(null, 'a', 'b', 'c')
}
Compile a waterfall
var fall = require('fastfall')([
function a (arg, cb) {
console.log('called a')
cb(null, arg)
},
function b (a, cb) {
console.log('called b with:', a)
cb(null, 'a', 'b')
},
function c (a, b, cb) {
console.log('called c with:', a, b)
cb(null, 'a', 'b', 'c')
}])
fall(42, function result (err, a, b, c) {
console.log('result arguments', arguments)
})
You can set this
by doing:
var that = { hello: 'world' }
var fall = require('fastfall')(that, [
function a (arg, cb) {
console.log('this is', this)
console.log('called a')
cb(null, arg)
},
function b (a, cb) {
console.log('called b with:', a)
cb(null, 'a', 'b')
},
function c (a, b, cb) {
console.log('called c with:', a, b)
cb(null, 'a', 'b', 'c')
}])
fall(42, function result (err, a, b, c) {
console.log('result arguments', arguments)
})
or you can simply attach it to an object:
var that = { hello: 'world' }
that.doSomething = require('fastfall')([
function a (arg, cb) {
console.log('this is', this)
console.log('called a')
cb(null, arg)
},
function b (a, cb) {
console.log('called b with:', a)
cb(null, 'a', 'b')
},
function c (a, b, cb) {
console.log('called c with:', a, b)
cb(null, 'a', 'b', 'c')
}])
that.doSomething(42, function result (err, a, b, c) {
console.log('this is', this)
console.log('result arguments', arguments)
})
API
fastfall([this], [functions])
Creates a fall
, it can either be pre-filled with a this
value
and an array of functions.
If there is no list of functions, a not-compiled fall
is returned, if there is a list of function a compiled fall
is returned.
fall([this], functions, [done])
Calls the functions in a waterfall, forwarding the arguments from one to
another. Calls done
when it has finished.
fall(args..., [done])
Calls the compiled functions in a waterfall, forwarding the arguments from one to
another. Additionally, a user can specify some arguments for the first
function, too. Calls done
when it has finished.
License
MIT