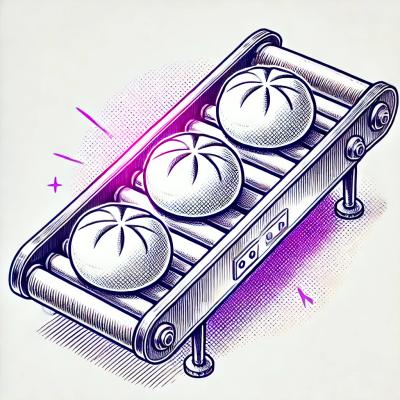
Security News
Bun 1.2 Released with 90% Node.js Compatibility and Built-in S3 Object Support
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
fetch-decorators
Advanced tools
A set of composable ES7 decorators around the fetch
api
Automate things request and response body parsing so you don't have to.
No dependency (oh, except a fetch polyfill maybe)
npm i -S fetch-decorators
class Messages {
@extractJson
@bodify
@fetchify({method: 'POST'})
post(userId) {
return `/users/${userId}/messages`;
}
}
const messages = new Messages();
messages.post('ArnaudRinquin')({
content: 'Hello World',
public: true,
draft: false,
}).then(({response, data}) => {
// response === the original fetch response
// data === the JSON object returned by the server
});
@fetchify
: decorates a function returning a url to a fetch
call with your options.@bodify
: prepare passed data (and extra options) into fetch-ready body options.@extractJson
, @extractText,
@extractBlob
: decorates a function returning a Response
to extract its result as json, text or blob.@extractAuto
: decorates a function returning a Response
to extract its result automatically based on response Content-Type
header.These decorators have been designed to be composable, give it a try!
This helper wraps the original function into a fetch call so it may just return a string, and then be called with optional data, headers, options.
(originalArgs) => url:string
becomes:
(originalArgs) => (options:?object) => fetchResponse:promise
import { fetchify } from 'fetch-decorators';
class Users {
constructor(baseUrl) {
this.baseUrl = baseUrl;
}
@fetchify()
get(userId) {
return `${this.baseUrl}/users/${userId}`;
}
@fetchify({
method: 'POST',
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json',
}
})
create() {
return `${this.baseUrl}/users`;
}
}
const userApi = new UserApi('/api');
userApi.createUser()({
body: JSON.stringify({
firstName: 'Walter',
lastName: 'White',
}
})).then(function(response){
// Regular `fetch` response
);
userApi.getUser('fakeUserId123')().then(function(response){
// Regular `fetch` response
});
Takes body data and options and calls the decorated function with a proper fetch options
object where options.body
is passed data as a string.
(options) => orignalResult
becomes:
(originalArgs) => (data:object, extraOptions:?object) => orignalResult(options)
import { bodify } from 'fetch-decorators';
class Messages {
@bodify
create(userId) {
return function(options) {
return fetch(`/api/users/${userId}/messages`, options);
};
}
}
const messages = new Messages();
const messages = {
content: 'Hello',
draft: false,
};
const options = { method: 'POST' };
users.create('fakeUserId')(message, options).then(function(response){
// response === the original fetch response
});
These decorators wrap functions returning a fetch promise with the matching Response extraction function.
(originalArgs) => fetchResponse:promise
becomes:
(originalArgs) => (options:?object) => extractionResult:promise
where the extractionResult
promise resolves with : {response:Response, data:any}
import { extractJson } from 'fetch-decorators';
class Users {
@extractJson
get(userId) {
return (options) => fetch(`/api/users/${userId}`, options);
}
}
const users = new Users();
users.get('userId123')().then(function({response, data}){
// response === the original fetch response
// data === the extracted data, here a `user` JSON object
});
This extractor has the same signature and behaviour as other extractors but will use the Reponse Content-Type
header to determine the right Response method to use for data extraction.
Content types are matched this way:
'application/json': 'json'
'text/plain': 'text'
'default': 'blob'
All these decorators where designed so it's easy to use them together, just stack them! Note: obviously, the order matters.
import {
fetchify,
bodify,
extractJson,
} from 'fetch-decorators';
class Messages {
@extractJson
@bodify
@fetchify({method: 'POST'})
post(userId) {
return `/users/${userId}/messages`;
}
// Approximate equivalent without decorators
// Thanks to ES6, the volume of code is roughly the same
// But the complexity is higher and you'll probably
// have a lot of code duplication
mehPost(userId, data, extraOptions) {
return fetch(`/users/${userId}/messages`, {
method: 'POST',
...extraOptions,
body: JSON.stringify(data),
}).then((response) => response.json());
}
}
const messagesApi = new Messages();
// Request body, as an object
const message = {
content: 'Hello World',
public: true,
draft: false,
};
// Some extra options
const authHeaders = {
headers: {
'X-AUTH-MUCH-SECURE': '123FOO456BAR',
},
};
messagesApi.post('ArnaudRinquin')(message, authHeaders).then(({response, data}) => {
// response === the original fetch response
// data === the JSON object returned by the server
});
Is it just syntactic sugar?
Yes.
I have a more complex case where my [ headers are dynamic | I have to change fetch options | whatever ]. How do I do?
Then you should manually write your call to fetch
instead of shoehorning these decorators in your code.
FAQs
A set of ES7 composable decorators around the fetch api
The npm package fetch-decorators receives a total of 9 weekly downloads. As such, fetch-decorators popularity was classified as not popular.
We found that fetch-decorators demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.