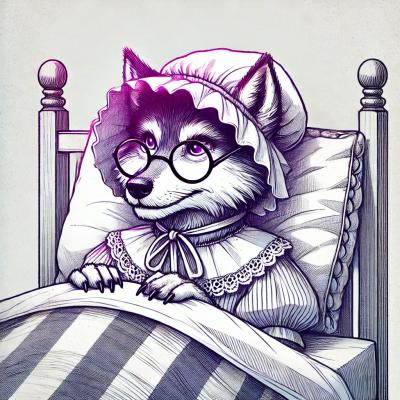
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
This is a simple Cooley-Tukey FFT implementation ported from https://gist.github.com/mbitsnbites/a065127577ff89ff885dd0a932ec2477.
This is a simple Cooley-Tukey FFT implementation ported from https://gist.github.com/mbitsnbites/a065127577ff89ff885dd0a932ec2477.
This works in native and in web using a shim of Bigarray
that uses Float64Array
under the hood.
The API has mutable and immutable APIs, for performance or simplicity respectively.
let shouldApplyHammingWindow = false;
let samplingRate = 8192;
let data = Fft.generateSine(
~frequency=200.,
~samplingRate,
~size=8192,
(),
);
/* Make sure to call getMaxAmplitude before applying any window to the data,
as the windowing function mutate the array, which would alter the min/max
values. */
let maxAmplitude = Fft.getMaxAmplitude(data);
if (shouldApplyHammingWindow) {
Fft.hammingWindow(~data);
};
let spectrum = Fft.fft(~data, ~maxAmplitude, ~samplingRate);
assert(abs_float(Fft.BA.get(spectrum, 200) -. 1.0) < 0.000001);
Performs an FFT on the given data and returns an array of floats representing the spectrum of the signal. Each index is a frequency in Hz, the value at each index is the amplitude of that frequency. The amplitudes in the spectrum are scaled such that the peak in the time domain has the same amplitude as the peak in the frequency domain.
let fft = (
~data : MyBigarray.t(Complex.t),
~maxAmplitude: float,
~samplingRate: float,
) => MyBigarray.t(float);
Helper to generate a sine or sum of sine waves (for a square wave).
let generateSine = (
~frequency : int,
~samplingRate=256 : int,
~size=256 : int,
~offset=0. : float,
~numberOfSines=1 : int,
() : unit
) => MyBigarray.t(Complex.t)
Returns the max real component in the given array of data. This is used to scale the FFT so that the peak in the frequency domain matches the peak in the time domain.
let getMaxAmplitude = (~data : MyBigarray.t(Complex.t)) => Complex.t
Mutates the data passed, applying a Hamming window to it.
amount
is used to tweak how much to apply the windowing function onto the signal, and is a number between 0 and 1.
let hammingWindow = (
~data : MyBigarray.t(Complex.t),
~amount=1. : float,
() : unit
) => unit
Mutates the data passed, applying a Hann window to it.
amount
is used to tweak how much to apply the windowing function onto the signal, and is a number between 0 and 1.
let hannWindow = (
~data : MyBigarray.t(Complex.t)
~amount=1. : float,
() : unit
) => unit
In place version of the main FFT algorithm. Does not do any scaling of the amplitudes (see fft
for explanation of the scaling done).
let fftInplace = (data : MyBigarray.t(Complex.t)) => unit
The following benchmarks were done in native.
For 2 arrays of floats
Using complex module:
Using complex module:
Using complex module:
Using complex module (with global and mutable bigarray bucket):
Using complex module:
FAQs
This is a simple Cooley-Tukey FFT implementation ported from https://gist.github.com/mbitsnbites/a065127577ff89ff885dd0a932ec2477.
The npm package fft.re receives a total of 0 weekly downloads. As such, fft.re popularity was classified as not popular.
We found that fft.re demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.