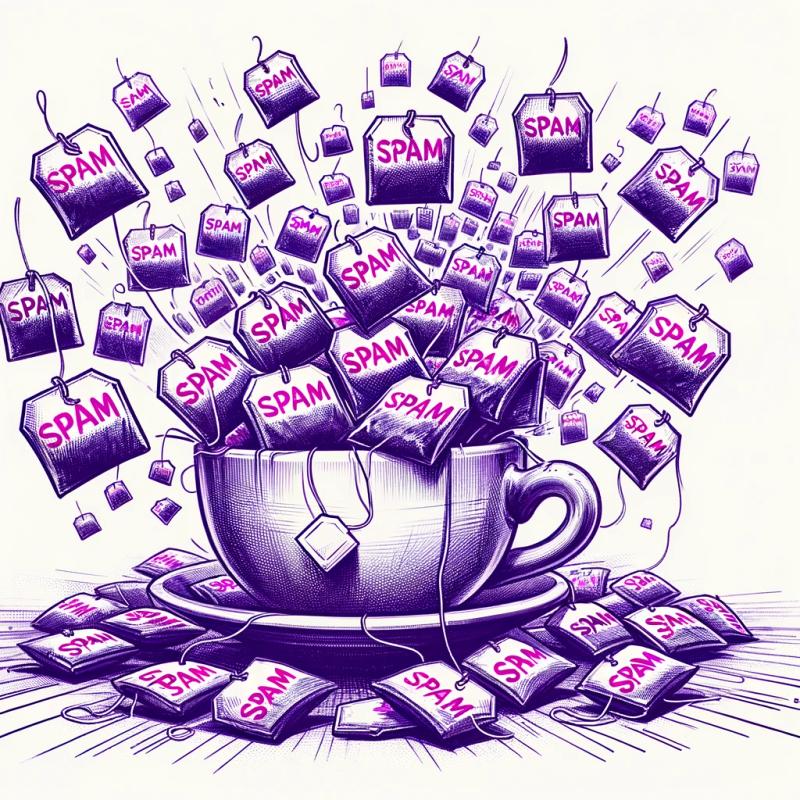
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
find-index
Advanced tools
Changelog
[1.1.1] - 2019-02-12
Readme
An implementation of the ES6 method Array.prototype.findIndex
as a standalone module and a
ponyfill.
Finds an item in an array matching a predicate function, and returns its index.
Fast both when thisArg
is used and also when it isn't.
npm install find-index
var findIndex = require('find-index/findIndex')
var findIndex = require('find-index/ponyfill') // will use native Array#findIndex if available.
var findLastIndex = require('find-index/findLastIndex') // search backwards from end
findIndex(array, callback[, thisArg])
findLastIndex(array, callback[, thisArg])
Parameters:
array
The array to operate on.
callback
Function to execute on each value in the array, taking three arguments:
element
The current element being processed in the array.
index
The index of the current element being processed in the array.
array
The array findIndex was called upon.
thisArg
Object to use as this when executing callback.
Based on array-findindex
$ iojs --harmony_arrays perf/benchmark.js
native Array.prototype.findIndex: 6347ms
findIndex: 1633ms
findIndex ponyfill: 6384ms
findLastIndex: 1508ms
npm lodash.findindex: 2900ms
npm array-findindex: 3512ms
FAQs
finds an item in an array matching a predicate function, and returns its index
The npm package find-index receives a total of 661,262 weekly downloads. As such, find-index popularity was classified as popular.
We found that find-index demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.