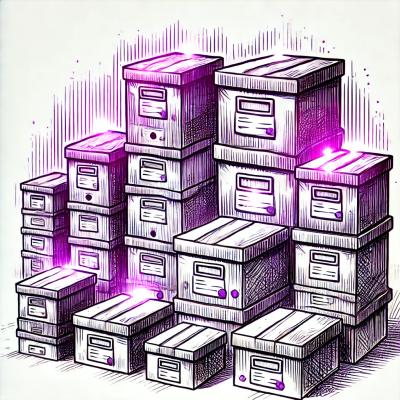
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
find-node-modules
Advanced tools
The find-node-modules npm package is a utility that helps you locate the node_modules directories in your project. This can be particularly useful in monorepos or projects with complex directory structures where node_modules might not be in the root directory.
Find node_modules in the current directory
This feature allows you to find all node_modules directories starting from the current directory. The code sample demonstrates how to use the package to get an array of paths to node_modules directories.
const findNodeModules = require('find-node-modules');
const paths = findNodeModules();
console.log(paths);
Find node_modules in a specific directory
This feature allows you to specify a starting directory to search for node_modules directories. The code sample shows how to find node_modules directories starting from a given path.
const findNodeModules = require('find-node-modules');
const paths = findNodeModules('/path/to/start');
console.log(paths);
Find node_modules with custom options
This feature allows you to customize the search with options such as the starting directory (cwd) and whether to return relative paths. The code sample demonstrates how to use these options.
const findNodeModules = require('find-node-modules');
const paths = findNodeModules({ cwd: '/path/to/start', relative: false });
console.log(paths);
The find-up package is used to find a file or directory by walking up parent directories. It is more general-purpose compared to find-node-modules, as it can locate any file or directory, not just node_modules.
The pkg-dir package finds the root directory of a Node.js project by looking for a package.json file. While it doesn't specifically find node_modules directories, it helps in locating the project root, which can be useful in conjunction with other tools.
The resolve package is used to resolve module paths as Node.js does. It can be used to find the location of a module, including its node_modules directory. It offers more functionality related to module resolution compared to find-node-modules.
This is a little node module to find the path of every parent node_modules directory. It's useful for things like Sass, where you can't specify the exact path to individual modules (in which case findup-sync would be sufficient), and you can't just give an array of parent node_modules which might exist, because it will error if they don't.
In most cases you're trying to find node_modules directories, findup-sync should be sufficient. This library is specifically for if you want an array containing all the parent node_modules paths. If you loop through the output of this library, you should be using findup-sync instead.
$ npm install --save find-node-modules
var findNodeModules = require('find-node-modules');
findNodeModules();
//=> ['node_modules', '../../node_modules']
findNodeModules({ cwd: './someDir' });
//=> ['../node_modules', '../../../node_modules']
findNodeModules('./someDir');
//=> ['../node_modules', '../../../node_modules']
findNodeModules({ cwd: './someDir', relative: false });
//=> ['/path/to/something/node_modules', '/path/node_modules']
This is released under the MIT license.
FAQs
Return an array of all parent node_modules directories
The npm package find-node-modules receives a total of 687,179 weekly downloads. As such, find-node-modules popularity was classified as popular.
We found that find-node-modules demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.