flat-embed
Advanced tools
Comparing version 1.1.0 to 1.2.0
# Changelog | ||
## v1.2.0 | ||
* Add methods: `getParts`, `getDisplayedParts` and `setDisplayedParts` | ||
## v1.1.0 | ||
@@ -4,0 +8,0 @@ |
@@ -1,2 +0,2 @@ | ||
/*! flat-embed v1.1.0 | (c) 2019 Tutteo Ltd. (Flat) | Apache-2.0 License | https://github.com/FlatIO/embed-client */ | ||
/*! flat-embed v1.2.0 | (c) 2020 Tutteo Ltd. (Flat) | Apache-2.0 License | https://github.com/FlatIO/embed-client */ | ||
(function (global, factory) { | ||
@@ -730,2 +730,38 @@ typeof exports === 'object' && typeof module !== 'undefined' ? module.exports = factory() : | ||
} | ||
/** | ||
* Get all the parts information | ||
* | ||
* @return {Promise} | ||
* @fullfill {array} List of the parts | ||
*/ | ||
}, { | ||
key: "getParts", | ||
value: function getParts() { | ||
return this.call('getParts'); | ||
} | ||
/** | ||
* Get the displayed parts | ||
* | ||
* @return {Promise} | ||
* @fullfill {array} List of the displayed parts | ||
*/ | ||
}, { | ||
key: "getDisplayedParts", | ||
value: function getDisplayedParts() { | ||
return this.call('getDisplayedParts'); | ||
} | ||
/** | ||
* Choose the parts to display | ||
* | ||
* @param {array} parts List of the parts to display (UUIDs, indexes/idx, names or abbv) | ||
* @return {Promise} | ||
*/ | ||
}, { | ||
key: "setDisplayedParts", | ||
value: function setDisplayedParts(parts) { | ||
return this.call('setDisplayedParts', parts); | ||
} | ||
}]); | ||
@@ -732,0 +768,0 @@ |
@@ -1,2 +0,2 @@ | ||
/*! flat-embed v1.1.0 | (c) 2019 Tutteo Ltd. (Flat) | Apache-2.0 License | https://github.com/FlatIO/embed-client */ | ||
!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?module.exports=t():"function"==typeof define&&define.amd?define(t):((e=e||self).Flat=e.Flat||{},e.Flat.Embed=t())}(this,function(){"use strict";function o(e){return(o="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}function n(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function r(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}function e(e,t,n){return t&&r(e.prototype,t),n&&r(e,n),e}if(void 0===window.postMessage)throw new Error("The Flat Embed JS API is not supported in this browser");function i(e,t){var n=function(e){var t=e.baseUrl||"https://flat-embed.com";t+="/"+(e.score||"blank");var n=Object.assign({jsapi:!0},e.embedParams);return t+"?"+Object.keys(n).map(function(e){return"".concat(encodeURIComponent(e),"=").concat(encodeURIComponent(n[e]))}).join("&")}(t),r=document.createElement("iframe");return r.setAttribute("src",n),r.setAttribute("width",t.width||"100%"),r.setAttribute("height",t.height||"100%"),r.setAttribute("allowfullscreen",!0),r.setAttribute("allow","midi"),r.setAttribute("frameborder","0"),e.appendChild(r),r}function s(e,t,n){if(!e.element.contentWindow||!e.element.contentWindow.postMessage)throw new Error("No `contentWindow` or `contentWindow.postMessage` avaialble on the element");var r={method:t,parameters:n};e.element.contentWindow.postMessage(r,e.origin)}var a=function(){function t(e){return n(this,t),this.embed=e,this.promises={},this.eventCallbacks={},this}return e(t,[{key:"pushCall",value:function(e,t,n){this.promises[e]=this.promises[e]||[],this.promises[e].push({resolve:t,reject:n})}},{key:"subscribeEvent",value:function(e,t){return this.eventCallbacks[e]=this.eventCallbacks[e]||[],this.eventCallbacks[e].push(t),1===this.eventCallbacks[e].length}},{key:"unsubscribeEvent",value:function(e,t){if(!this.eventCallbacks[e])return!1;if(t){var n=this.eventCallbacks[e].indexOf(t);0<=n&&this.eventCallbacks[e].splice(n,1)}else this.eventCallbacks[e]=[];return!t||0===this.eventCallbacks[e].length}},{key:"process",value:function(e){e.method?this.processMethodResponse(e):e.event&&this.processEvent(e)}},{key:"processMethodResponse",value:function(e){if(this.promises[e.method]){var t=this.promises[e.method].shift();t&&(e.error?t.reject(e.error):t.resolve(e.response))}}},{key:"processEvent",value:function(t){var n=this;this.eventCallbacks[t.event]&&0!==this.eventCallbacks[t.event].length&&this.eventCallbacks[t.event].forEach(function(e){e.call(n.embed,t.parameters)})}}]),t}(),u=new WeakMap,l=new WeakMap;return function(){function Embed(r){var o=this,e=1<arguments.length&&void 0!==arguments[1]?arguments[1]:{};if(n(this,Embed),r=function(e){if(window.jQuery&&e instanceof window.jQuery&&(e=e[0]),"string"==typeof e&&(e=document.getElementById(e)),!(e instanceof window.HTMLElement))throw new TypeError("The first parameter must be an existing DOM element or an identifier.");if("IFRAME"!==e.nodeName){var t=e.querySelector("iframe");t&&(e=t)}return e}(r),u.has(r))return u.get(r);"IFRAME"!==r.nodeName&&(r=i(r,e)),this.origin="*",this.element=r,this.embedCallback=new a;var t=new Promise(function(n){window.addEventListener("message",function(e){if(r.contentWindow===e.source){"*"===o.origin&&(o.origin=e.origin);var t=function(e){return"string"==typeof e&&(e=JSON.parse(e)),e}(e.data);"ready"!==t.event&&"ping"!==t.method?o.embedCallback.process(t):n()}},!1),s(o,"ping")});return u.set(this.element,this),l.set(this,t),this}return e(Embed,[{key:"ready",value:function(){return Promise.resolve(l.get(this))}},{key:"call",value:function(n){var r=this,o=1<arguments.length&&void 0!==arguments[1]?arguments[1]:{};return new Promise(function(e,t){return r.ready().then(function(){r.embedCallback.pushCall(n,e,t),s(r,n,o)})})}},{key:"on",value:function(e,t){if("string"!=typeof e)throw new TypeError("An event name (string) is required");if("function"!=typeof t)throw new TypeError("An callback (function) is required");this.embedCallback.subscribeEvent(e,t)&&this.call("addEventListener",e).catch(function(){})}},{key:"off",value:function(e,t){if("string"!=typeof e)throw new TypeError("An event name (string) is required");this.embedCallback.unsubscribeEvent(e,t)&&this.call("removeEventListener",e).catch(function(){})}},{key:"loadFlatScore",value:function(e,t){return this.call("loadFlatScore",{score:e,revision:t})}},{key:"loadMusicXML",value:function(e){return this.call("loadMusicXML",e)}},{key:"loadJSON",value:function(e){return this.call("loadJSON",e)}},{key:"getJSON",value:function(){return this.call("getJSON")}},{key:"getMusicXML",value:function(n){var r=this;return new Promise(function(t,e){if("object"!==o(n=n||{}))return e(new TypeError("Options must be an object"));r.call("getMusicXML",n).then(function(e){return t("string"==typeof e?e:new Uint8Array(e))}).catch(e)})}},{key:"getPNG",value:function(n){var r=this;return new Promise(function(t,e){if("object"!==o(n=n||{}))return e(new TypeError("Options must be an object"));r.call("getPNG",n).then(function(e){return t("string"==typeof e?e:new Uint8Array(e))}).catch(e)})}},{key:"getMIDI",value:function(){return this.call("getMIDI").then(function(e){return new Uint8Array(e)})}},{key:"getFlatScoreMetadata",value:function(){return this.call("getFlatScoreMetadata")}},{key:"getEmbedConfig",value:function(){return this.call("getEmbedConfig")}},{key:"setEditorConfig",value:function(e){return this.call("setEditorConfig",e)}},{key:"fullscreen",value:function(e){return this.call("fullscreen",e)}},{key:"play",value:function(){return this.call("play")}},{key:"pause",value:function(){return this.call("pause")}},{key:"stop",value:function(){return this.call("stop")}},{key:"mute",value:function(){return this.call("mute")}},{key:"print",value:function(){return this.call("print")}},{key:"getZoom",value:function(){return this.call("getZoom")}},{key:"setZoom",value:function(e){return this.call("setZoom",e)}},{key:"getAutoZoom",value:function(){return this.call("getAutoZoom")}},{key:"setAutoZoom",value:function(e){return this.call("setAutoZoom",e)}},{key:"focusScore",value:function(){return this.call("focusScore")}},{key:"getCursorPosition",value:function(){return this.call("getCursorPosition")}},{key:"setCursorPosition",value:function(e){return this.call("setCursorPosition",e)}}]),Embed}()}); | ||
/*! flat-embed v1.2.0 | (c) 2020 Tutteo Ltd. (Flat) | Apache-2.0 License | https://github.com/FlatIO/embed-client */ | ||
!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?module.exports=t():"function"==typeof define&&define.amd?define(t):((e=e||self).Flat=e.Flat||{},e.Flat.Embed=t())}(this,function(){"use strict";function i(e){return(i="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e})(e)}function n(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function r(e,t){for(var n=0;n<t.length;n++){var r=t[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(e,r.key,r)}}function e(e,t,n){return t&&r(e.prototype,t),n&&r(e,n),e}if(void 0===window.postMessage)throw new Error("The Flat Embed JS API is not supported in this browser");function o(e,t){var n=function(e){var t=e.baseUrl||"https://flat-embed.com";t+="/"+(e.score||"blank");var n=Object.assign({jsapi:!0},e.embedParams);return t+"?"+Object.keys(n).map(function(e){return"".concat(encodeURIComponent(e),"=").concat(encodeURIComponent(n[e]))}).join("&")}(t),r=document.createElement("iframe");return r.setAttribute("src",n),r.setAttribute("width",t.width||"100%"),r.setAttribute("height",t.height||"100%"),r.setAttribute("allowfullscreen",!0),r.setAttribute("allow","midi"),r.setAttribute("frameborder","0"),e.appendChild(r),r}function s(e,t,n){if(!e.element.contentWindow||!e.element.contentWindow.postMessage)throw new Error("No `contentWindow` or `contentWindow.postMessage` avaialble on the element");var r={method:t,parameters:n};e.element.contentWindow.postMessage(r,e.origin)}var a=function(){function t(e){return n(this,t),this.embed=e,this.promises={},this.eventCallbacks={},this}return e(t,[{key:"pushCall",value:function(e,t,n){this.promises[e]=this.promises[e]||[],this.promises[e].push({resolve:t,reject:n})}},{key:"subscribeEvent",value:function(e,t){return this.eventCallbacks[e]=this.eventCallbacks[e]||[],this.eventCallbacks[e].push(t),1===this.eventCallbacks[e].length}},{key:"unsubscribeEvent",value:function(e,t){if(!this.eventCallbacks[e])return!1;if(t){var n=this.eventCallbacks[e].indexOf(t);0<=n&&this.eventCallbacks[e].splice(n,1)}else this.eventCallbacks[e]=[];return!t||0===this.eventCallbacks[e].length}},{key:"process",value:function(e){e.method?this.processMethodResponse(e):e.event&&this.processEvent(e)}},{key:"processMethodResponse",value:function(e){if(this.promises[e.method]){var t=this.promises[e.method].shift();t&&(e.error?t.reject(e.error):t.resolve(e.response))}}},{key:"processEvent",value:function(t){var n=this;this.eventCallbacks[t.event]&&0!==this.eventCallbacks[t.event].length&&this.eventCallbacks[t.event].forEach(function(e){e.call(n.embed,t.parameters)})}}]),t}(),u=new WeakMap,l=new WeakMap;return function(){function Embed(r){var i=this,e=1<arguments.length&&void 0!==arguments[1]?arguments[1]:{};if(n(this,Embed),r=function(e){if(window.jQuery&&e instanceof window.jQuery&&(e=e[0]),"string"==typeof e&&(e=document.getElementById(e)),!(e instanceof window.HTMLElement))throw new TypeError("The first parameter must be an existing DOM element or an identifier.");if("IFRAME"!==e.nodeName){var t=e.querySelector("iframe");t&&(e=t)}return e}(r),u.has(r))return u.get(r);"IFRAME"!==r.nodeName&&(r=o(r,e)),this.origin="*",this.element=r,this.embedCallback=new a;var t=new Promise(function(n){window.addEventListener("message",function(e){if(r.contentWindow===e.source){"*"===i.origin&&(i.origin=e.origin);var t=function(e){return"string"==typeof e&&(e=JSON.parse(e)),e}(e.data);"ready"!==t.event&&"ping"!==t.method?i.embedCallback.process(t):n()}},!1),s(i,"ping")});return u.set(this.element,this),l.set(this,t),this}return e(Embed,[{key:"ready",value:function(){return Promise.resolve(l.get(this))}},{key:"call",value:function(n){var r=this,i=1<arguments.length&&void 0!==arguments[1]?arguments[1]:{};return new Promise(function(e,t){return r.ready().then(function(){r.embedCallback.pushCall(n,e,t),s(r,n,i)})})}},{key:"on",value:function(e,t){if("string"!=typeof e)throw new TypeError("An event name (string) is required");if("function"!=typeof t)throw new TypeError("An callback (function) is required");this.embedCallback.subscribeEvent(e,t)&&this.call("addEventListener",e).catch(function(){})}},{key:"off",value:function(e,t){if("string"!=typeof e)throw new TypeError("An event name (string) is required");this.embedCallback.unsubscribeEvent(e,t)&&this.call("removeEventListener",e).catch(function(){})}},{key:"loadFlatScore",value:function(e,t){return this.call("loadFlatScore",{score:e,revision:t})}},{key:"loadMusicXML",value:function(e){return this.call("loadMusicXML",e)}},{key:"loadJSON",value:function(e){return this.call("loadJSON",e)}},{key:"getJSON",value:function(){return this.call("getJSON")}},{key:"getMusicXML",value:function(n){var r=this;return new Promise(function(t,e){if("object"!==i(n=n||{}))return e(new TypeError("Options must be an object"));r.call("getMusicXML",n).then(function(e){return t("string"==typeof e?e:new Uint8Array(e))}).catch(e)})}},{key:"getPNG",value:function(n){var r=this;return new Promise(function(t,e){if("object"!==i(n=n||{}))return e(new TypeError("Options must be an object"));r.call("getPNG",n).then(function(e){return t("string"==typeof e?e:new Uint8Array(e))}).catch(e)})}},{key:"getMIDI",value:function(){return this.call("getMIDI").then(function(e){return new Uint8Array(e)})}},{key:"getFlatScoreMetadata",value:function(){return this.call("getFlatScoreMetadata")}},{key:"getEmbedConfig",value:function(){return this.call("getEmbedConfig")}},{key:"setEditorConfig",value:function(e){return this.call("setEditorConfig",e)}},{key:"fullscreen",value:function(e){return this.call("fullscreen",e)}},{key:"play",value:function(){return this.call("play")}},{key:"pause",value:function(){return this.call("pause")}},{key:"stop",value:function(){return this.call("stop")}},{key:"mute",value:function(){return this.call("mute")}},{key:"print",value:function(){return this.call("print")}},{key:"getZoom",value:function(){return this.call("getZoom")}},{key:"setZoom",value:function(e){return this.call("setZoom",e)}},{key:"getAutoZoom",value:function(){return this.call("getAutoZoom")}},{key:"setAutoZoom",value:function(e){return this.call("setAutoZoom",e)}},{key:"focusScore",value:function(){return this.call("focusScore")}},{key:"getCursorPosition",value:function(){return this.call("getCursorPosition")}},{key:"setCursorPosition",value:function(e){return this.call("setCursorPosition",e)}},{key:"getParts",value:function(){return this.call("getParts")}},{key:"getDisplayedParts",value:function(){return this.call("getDisplayedParts")}},{key:"setDisplayedParts",value:function(e){return this.call("setDisplayedParts",e)}}]),Embed}()}); |
{ | ||
"name": "flat-embed", | ||
"version": "1.1.0", | ||
"version": "1.2.0", | ||
"description": "Interact and get events from Flat's Sheet Music Embed", | ||
"license": "Apache-2.0", | ||
"author": "Flat Team <team@flat.io>", | ||
"author": "Flat Team <developers@flat.io>", | ||
"main": "dist/embed.js", | ||
@@ -44,6 +44,6 @@ "jsnext:main": "src/embed.js", | ||
"chokidar": "^3.0.0", | ||
"eslint": "^5.16.0", | ||
"eslint": "^6.2.1", | ||
"jquery": "^3.4.1", | ||
"karma": "^4.1.0", | ||
"karma-chrome-launcher": "^2.2.0", | ||
"karma-chrome-launcher": "^3.0.0", | ||
"karma-mocha": "^1.3.0", | ||
@@ -54,6 +54,6 @@ "karma-mocha-reporter": "^2.2.5", | ||
"rollup-plugin-babel": "^4.3.2", | ||
"rollup-plugin-commonjs": "^9.3.4", | ||
"rollup-plugin-node-resolve": "^4.2.4", | ||
"rollup-plugin-commonjs": "^10.0.0", | ||
"rollup-plugin-node-resolve": "^5.0.0", | ||
"uglify-js": "^3.5.12" | ||
} | ||
} |
# Flat Sheet Music Embed Client | ||
[](https://travis-ci.org/FlatIO/embed-client) | ||
[](https://greenkeeper.io/) | ||
[](https://www.npmjs.org/package/flat-embed) | ||
[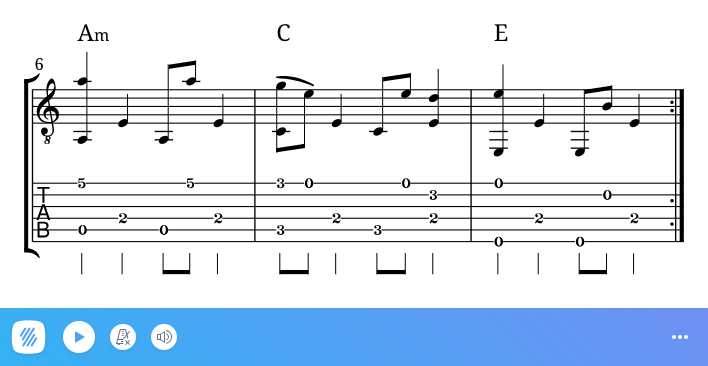](https://flat.io/developers/embed) | ||
[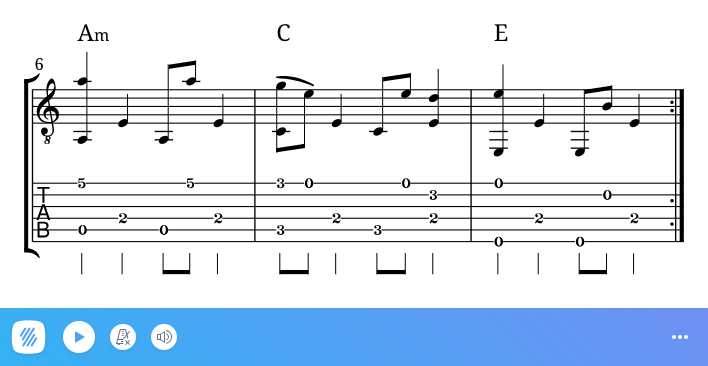](https://flat.io/embed) | ||
Use this JavaScript Client to interact and receive events from our [Sheet Music Embed](https://flat.io/developers/embed). | ||
Use this JavaScript Client to interact and receive events from our [Sheet Music Embed](https://flat.io/embed). | ||
@@ -25,3 +24,3 @@ If you have any feedback or questions regarding this product, [please feel free to contact our developers support](mailto:developers@flat.io). | ||
```html | ||
<script src="https://prod.flat-cdn.com/embed-js/v1.1.0/embed.min.js"></script> | ||
<script src="https://prod.flat-cdn.com/embed-js/v1.2.0/embed.min.js"></script> | ||
``` | ||
@@ -35,3 +34,3 @@ | ||
<div id="embed-container"></div> | ||
<script src="https://prod.flat-cdn.com/embed-js/v1.1.0/embed.min.js"></script> | ||
<script src="https://prod.flat-cdn.com/embed-js/v1.2.0/embed.min.js"></script> | ||
<script> | ||
@@ -48,2 +47,18 @@ var container = document.getElementById('embed-container'); | ||
``` | ||
Otherwise, if you are using our embed in an ES6 project: | ||
```js | ||
import Embed from 'flat-embed'; | ||
const container = document.getElementById('embed-container'); | ||
const embed = new Embed(container, { | ||
score: '<score-id-you-want-to-load>', | ||
embedParams: { | ||
appId: '<your-app-id>', | ||
controlsPosition: 'bottom', | ||
} | ||
}); | ||
``` | ||
*[>> Open this demo in JSFiddle](https://jsfiddle.net/gierschv/jr91116y/)* | ||
@@ -83,3 +98,3 @@ | ||
* [Viewer Methods](#viewer-methods) | ||
* [Viewer API](#viewer-api) | ||
* [`ready`](#ready-promisevoid-error): Wait until the JavaScript is ready | ||
@@ -110,2 +125,6 @@ * [`on`](#onevent-string-callback-function-void): Subscribe to events | ||
* [`setCursorPosition`](#setcursorpositionposition-object-promiseobject-error): Set a new position for the cursor | ||
* [`getParts`](#getparts-promisearray-error): Get the list of all the parts | ||
* [`getDisplayedParts`](#getdisplayedparts-promisearray-error): Get the displayed parts | ||
* [`setDisplayedParts`](#setdisplayedpartsparts-promisevoid-error): Choose the parts to display | ||
* [Editor API](#editor-api) | ||
* [Events API](#events-api) | ||
@@ -121,3 +140,3 @@ * [`scoreLoaded`](#event-scoreLoaded): A new score has been loaded | ||
## Viewer Methods | ||
## Viewer API | ||
@@ -484,4 +503,57 @@ You can call the methods using any `Flat.Embed` object. By default, the methods (except `on` and `off`) return a [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) that will be resolved once the method is called, the value is set or get: | ||
## Editor Methods | ||
### `getParts(): Promise(<Array, Error>)` | ||
Get the list of all the parts of the current score. | ||
```js | ||
embed.getParts().then(function (parts) { | ||
// parts: [ | ||
// { | ||
// idx: 0 | ||
// uuid: "ff78f481-2658-a94e-b3b2-c81f6d83bff0" | ||
// name: "Grand Piano" | ||
// abbreviation: "Pno." | ||
// isTransposing: false | ||
// }, | ||
// { | ||
// idx: 1 | ||
// uuid: "ab0ec60f-13ca-765d-34c6-0f181e58a672" | ||
// name: "Drum Set" | ||
// abbreviation: "Drs." | ||
// isTransposing: false | ||
// } | ||
//] | ||
}); | ||
``` | ||
### `getDisplayedParts(): Promise(<Array, Error>)` | ||
Get the list of the displayed parts. You can update the displayed parts with [`setDisplayedParts()`](#setdisplayedpartsparts-promisevoid-error). | ||
```js | ||
embed.getDisplayedParts().then(function (parts) { | ||
// parts: [ | ||
// { | ||
// idx: 0 | ||
// uuid: "ff78f481-2658-a94e-b3b2-c81f6d83bff0" | ||
// name: "Grand Piano" | ||
// abbreviation: "Pno." | ||
// isTransposing: false | ||
// } | ||
//] | ||
}); | ||
``` | ||
### `setDisplayedParts(parts): Promise(<void, Error>)` | ||
Set the list of the parts to display. This list (array) can either contain the UUIDs of the parts to display, their indexes (idx) starting from 0, the names of the parts or their abbreviations. | ||
```js | ||
embed.setDisplayedParts(['Violin', 'Viola']).then(function () { | ||
// display update queued | ||
}); | ||
``` | ||
## Editor API | ||
You can enable the editor mode by setting the `mode` to `edit` when creating the embed: | ||
@@ -498,2 +570,4 @@ | ||
[Check out an implementation example of the editor](https://flat.io/developers/docs/embed/javascript-editor.html). | ||
## Events API | ||
@@ -500,0 +574,0 @@ |
@@ -377,4 +377,34 @@ import './lib/compatibility'; | ||
} | ||
/** | ||
* Get all the parts information | ||
* | ||
* @return {Promise} | ||
* @fullfill {array} List of the parts | ||
*/ | ||
getParts() { | ||
return this.call('getParts'); | ||
} | ||
/** | ||
* Get the displayed parts | ||
* | ||
* @return {Promise} | ||
* @fullfill {array} List of the displayed parts | ||
*/ | ||
getDisplayedParts() { | ||
return this.call('getDisplayedParts'); | ||
} | ||
/** | ||
* Choose the parts to display | ||
* | ||
* @param {array} parts List of the parts to display (UUIDs, indexes/idx, names or abbv) | ||
* @return {Promise} | ||
*/ | ||
setDisplayedParts(parts) { | ||
return this.call('setDisplayedParts', parts); | ||
} | ||
} | ||
export default Embed; |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
109584
1228
639