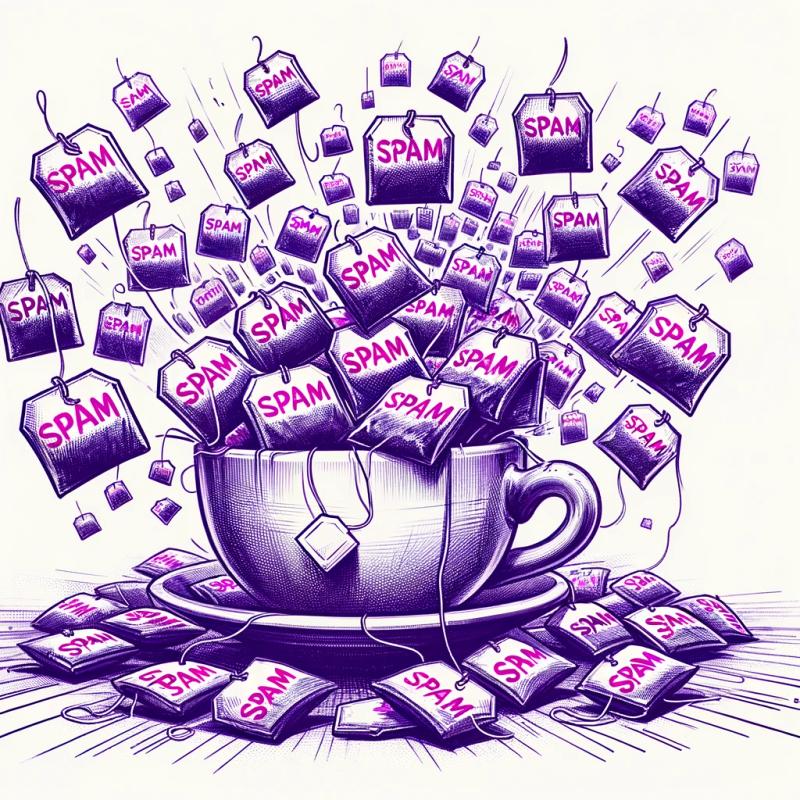
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
foreach-prop
Advanced tools
Changelog
Readme
Array-like methods for objects
:warning: Some javascript implementations don't follow the object key order. Keep that in mind when you use
keyOf
,lastKeyOf
,findKey
andfind
methods.
npm i foreach-prop
<script src="https://cdn.jsdelivr.net/npm/foreach-prop@latest/dist/each-prop.umd.js"></script>
for production
<script src="https://cdn.jsdelivr.net/npm/foreach-prop@latest/dist/each-prop.umd.min.js"></script>
<script src="https://unpkg.com/foreach-prop@latest/dist/each-prop.umd.js"></script>
for production
<script src="https://unpkg.com/foreach-prop@latest/dist/each-prop.umd.min.js"></script>
example
import { map } from "foreach-prop";
const object = {
key1: "str",
key2: 1,
};
const result = map(object, (value, key, extra1) => {
return key + extra1;
}, " $$");
console.log(result);
{
key1: "key1 $$",
key2: "key2 $$",
}
See the API section for more details.
const { forEach } = require("foreach-prop");
forEach(object, callback);
After adding the script
tag, eachProp
object will be available globally, containing all methods detailed in the API section.
eachProp.forEach(object, callback);
Similar to Array.prototype.forEach
. It calls the provided callback function for every key-value-pair
in the object. Once initiated there is no way to stop the execution of this function, if you intend to stop the iteration at some point have a look at findKey
method.
forEach(object, function callback(value, key, ...extra) => void, ...extra): void;
Any extra
argument will be passed down to the callback function.
The callback function inherits the this
value from the function call, so if you want a specific this
value in your callback function, you can call it using the call
method of the Function.prototype
.
forEach.call(thisArg, object, callback, ...extra);
Similar to Array.prototype.map
. It calls the provided callback function for every key-value-pair
in the object and returns a new object.
map(object, function callback(value, key, ...extra) => any, ...extra): object;
Any extra
argument will be passed down to the callback function.
The callback function inherits the this
value from the function call, so if you want a specific this
value in your callback function, you can call it using the call
method of the Function.prototype
.
map.call(thisArg, object, callback, ...extra);
Similar to Array.prototype.indexOf
. It returns the key of the first value that equals the provided one, or null
if not found.
keyOf(object, value): string | null;
Similar to Array.prototype.lastIndexOf
. It returns the key of the last value that equals the provided one, or null
if not found.
lastKeyOf(object, value): string | null;
added in: v0.2.0
Similar to Array.prototype.includes
. It returns whether or not a value is present in an object.
includes(object, value): boolean;
Similar to Array.prototype.findIndex
. It calls the provided callback function for every key-value-pair
in the object and returns the key once the provided callback function return a truthy value. It returns null
if nothing found.
findKey(object, function callback(value, key, ...extra) => any, ...extra): string | null;
Any extra
argument will be passed down to the callback function.
The callback function inherits the this
value from the function call, so if you want a specific this
value in your callback function, you can call it using the call
method of the Function.prototype
.
findKey.call(thisArg, object, callback, ...extra);
added in: v0.1.0
Similar to Array.prototype.find
. It calls the provided callback function for every key-value-pair
in the object and returns the value once the provided callback function return a truthy value. It returns undefined
if nothing found.
find(object, function callback(value, key, ...extra) => any, ...extra): any;
Note that the returned value may be
undefined
even if the condition is met and the value isundefined
.
example
const undef;
// undef is undefined
const object = { key1: undef };
// object.key1 is also undefined
const value = find(object, (val, key) => {
return key === "key1"
});
console.log(value);
// it logs undefined
// because undef is undefined
undefined
Any extra
argument will be passed down to the callback function.
The callback function inherits the this
value from the function call, so if you want a specific this
value in your callback function, you can call it using the call
method of the Function.prototype
.
find.call(thisArg, object, callback, ...extra);
Similar to Array.prototype.filter
. It calls the provided callback function for every key-value-pair
in the object and returns a new object containing the key-value-pairs corresponding to those where the provided callback function returned a truthy value.
filter(object, function callback(value, key, ...extra) => any, ...extra): object;
Any extra
argument will be passed down to the callback function.
The callback function inherits the this
value from the function call, so if you want a specific this
value in your callback function, you can call it using the call
method of the Function.prototype
.
filter.call(thisArg, object, callback, ...extra);
Similar to Array.prototype.reduce
but with a major difference: if no initial value provided it defaults to undefined
.
reduce(object, function callback(current, value, key, ...extra) => any, initial?, ...extra): any;
Any extra
argument will be passed down to the callback function.
The callback function inherits the this
value from the function call, so if you want a specific this
value in your callback function, you can call it using the call
method of the Function.prototype
.
reduce.call(thisArg, object, callback, initial?, ...extra);
added in: v0.2.0
Similar to Array.prototype.some
. It returns whether at least one of the key-value-pairs satisfy the provided callback function.
some(object, function callback(value, key, ...extra) => any, ...extra): boolean;
Any extra
argument will be passed down to the callback function.
The callback function inherits the this
value from the function call, so if you want a specific this
value in your callback function, you can call it using the call
method of the Function.prototype
.
some.call(thisArg, object, callback, ...extra): boolean;
added in: v0.2.0
Similar to Array.prototype.every
. It returns whether all key-value-pairs satisfy the provided callback function.
every(object, function callback(value, key, ...extra) => any, ...extra): boolean;
Any extra
argument will be passed down to the callback function.
The callback function inherits the this
value from the function call, so if you want a specific this
value in your callback function, you can call it using the call
method of the Function.prototype
.
every.call(thisArg, object, callback, ...extra): boolean;
added in: v2.1.0
Similar to new Array()
. It creates a new object with the given keys. If a value is provided, every property will be populated with the given value or undefined
otherwise.
create(keys: Array<string | number>, value?: any): object;
example
const object = create(['a', 'b'], true);
console.log(object);
{ a: true, b: true }
added in: v2.1.0
Similar to Array.prototype.fill
with a difference, it returns a new object instead of modifying the given one. Every property in the new object will be set to the provided value.
fill(object, value): object;
MIT © 2019 Manuel Fernández
FAQs
Array-like methods for objects
The npm package foreach-prop receives a total of 89 weekly downloads. As such, foreach-prop popularity was classified as not popular.
We found that foreach-prop demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.