Comparing version 0.5.0 to 0.5.1
{ | ||
"name": "formik", | ||
"description": "An elegant way to handle forms in React", | ||
"version": "0.5.0", | ||
"version": "0.5.1", | ||
"license": "MIT", | ||
@@ -6,0 +6,0 @@ "author": "Jared Palmer <jared@palmer.net>", |
159
README.md
@@ -1,2 +0,2 @@ | ||
# Formik | ||
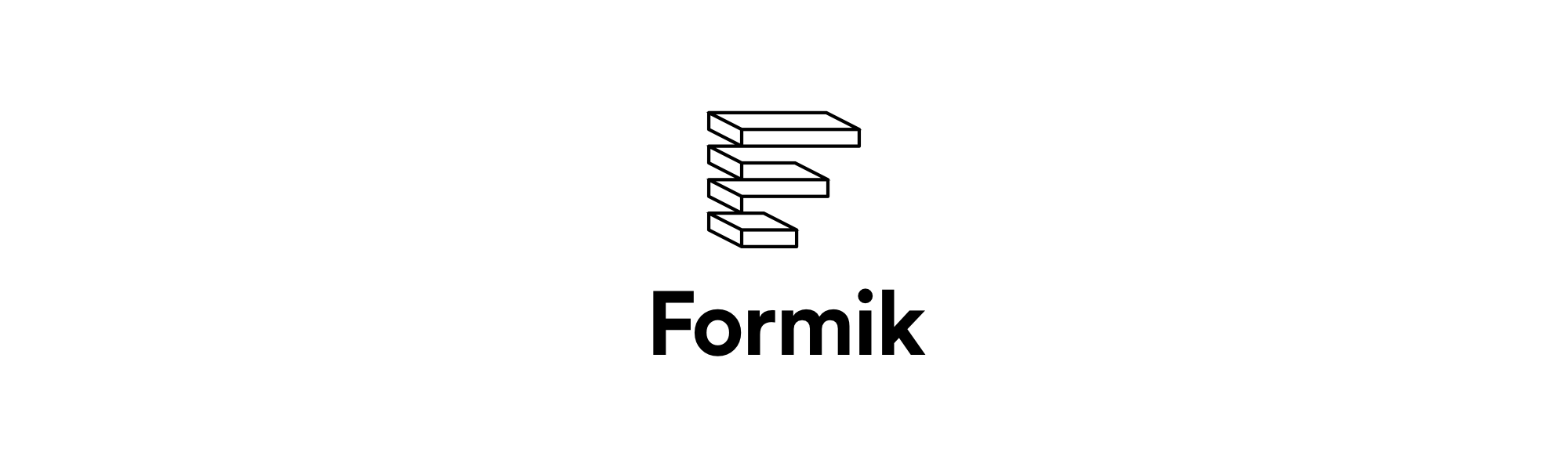 | ||
@@ -27,2 +27,27 @@ #### Forms in React, *without tears.* | ||
- [Simple Example](#simple-example) | ||
- [API](#api) | ||
- [`Formik(options)`](#formikoptions) | ||
- [`options`](#options) | ||
- [`displayName: string`](#displayname-string) | ||
- [`handleSubmit: (payload, FormikBag) => void`)](#handlesubmit-payload-formikbag--void) | ||
- [`mapPropsToValues?: (props) => props`](#mappropstovalues-props--props) | ||
- [`mapValuesToPayload?: (values) => payload`](#mapvaluestopayload-values--payload) | ||
- [`validationSchema: Schema`](#validationschema-schema) | ||
- [Injected props and methods](#injected-props-and-methods) | ||
- [`error?: any`](#error-any) | ||
- [`errors: { [field]: string }`](#errors--field-string-) | ||
- [`handleBlur: (e: any) => void`](#handleblur-e-any--void) | ||
- [`handleChange: (e: React.ChangeEvent<any>) => void`](#handlechange-e-reactchangeeventany--void) | ||
- [`handleChangeValue: (name: string, value: any) => void`](#handlechangevalue-name-string-value-any--void) | ||
- [`handleReset: () => void`](#handlereset---void) | ||
- [`handleSubmit: (e: React.FormEvent<HTMLFormEvent>) => void`](#handlesubmit-e-reactformeventhtmlformevent--void) | ||
- [`isSubmitting: boolean`](#issubmitting-boolean) | ||
- [`resetForm: (nextProps?: Props) => void`](#resetform-nextprops-props--void) | ||
- [`setError(err: any) => void`](#seterrorerr-any--void) | ||
- [`setErrors(fields: { [field]: string }) => void`](#seterrorsfields--field-string---void) | ||
- [`setSubmitting(boolean) => void`](#setsubmittingboolean--void) | ||
- [`setTouched(fields: { [field]: string }) => void`](#settouchedfields--field-string---void) | ||
- [`setValues(fields: { [field]: any }) => void`](#setvaluesfields--field-any---void) | ||
- [`touched: { [field]: string }`](#touched--field-string-) | ||
- [`values: { [field]: any }`](#values--field-any-) | ||
- [Recipes](#recipes) | ||
@@ -32,7 +57,2 @@ - [Ways to call `Formik`](#ways-to-call-formik) | ||
- [Example: Resetting a form when props change](#example-resetting-a-form-when-props-change) | ||
- [API](#api) | ||
- [`Formik(options)`](#formikoptions) | ||
- [Arguments](#arguments) | ||
- [Returns](#returns) | ||
- [Injected props and methods (a.k.a. the "Formik Bag")](#injected-props-and-methods-aka-the-formik-bag) | ||
- [Authors](#authors) | ||
@@ -157,3 +177,4 @@ | ||
// do stuff with your payload | ||
// e.preventDefault(), setSubmitting, setError(undefined) are called before handle submit is. so you don | ||
// e.preventDefault(), setSubmitting, setError(undefined) are // called before handleSubmit is. So you don't have to. | ||
// HandleSubmit will only be executed if form values pass Yup validation. | ||
CallMyApi(props.user.id, payload) | ||
@@ -177,2 +198,77 @@ .then( | ||
## API | ||
### `Formik(options)` | ||
Create A higher-order React component class that passes props and form handlers (the "`FormikBag`") into your component derived from supplied options. | ||
#### `options` | ||
##### `displayName: string` | ||
Set the display name of your component. | ||
##### `handleSubmit: (payload, FormikBag) => void`) | ||
Your form submission handler. It is passed the result of `mapValuesToPayload` (if specified), or the result of `mapPropsToValues`, or all props that are not functions (in that order of precedence) and the "`FormikBag`". | ||
##### `mapPropsToValues?: (props) => props` | ||
If this option is specified, then Formik will transfer its results into updatable form state and make these values available to the new component as `props.values`. If `mapPropsToValues` is not specified, then Formik will map all props that are not functions to the new component's `props.values`. That is, if you omit it, Formik will only pass `props` where `typeof props[k] !== 'function'`, where `k` is some key. | ||
##### `mapValuesToPayload?: (values) => payload` | ||
If this option is specified, then Formik will run this function just before calling `handleSubmit`. Use it to transform the your form's `values` back into a shape that's consumable for other parts of your application or API. If `mapValuesToPayload` **is not** specified, then Formik will map all `values` directly to `payload` (which will be passed to `handleSubmit`). While this transformation can be moved into `handleSubmit`, consistently defining it in `mapValuesToPayload` separates concerns and helps you stay organized. | ||
##### `validationSchema: Schema` | ||
[A Yup schema](https://github.com/jquense/yup). This is used for validation on each onChange event. Errors are mapped by key to the `WrappedComponent`'s `props.errors`. Its keys should almost always match those of `WrappedComponent's` `props.values`. | ||
#### Injected props and methods | ||
The following props and methods will be injected into the `WrappedComponent` (i.e. your form): | ||
##### `error?: any` | ||
A top-level error object, can be whatever you need. | ||
##### `errors: { [field]: string }` | ||
Form validation errors. Keys match the shape of the `validationSchema` defined in Formik options. This should therefore also map to your `values` object as well. Internally, Formik transforms raw [Yup validation errors](https://github.com/jquense/yup#validationerrorerrors-string--arraystring-value-any-path-string) on your behalf. | ||
##### `handleBlur: (e: any) => void` | ||
`onBlur` event handler. Useful for when you need to track whether an input has been `touched` or not. This should be passed to `<input onBlur={handleBlur} ... />` | ||
##### `handleChange: (e: React.ChangeEvent<any>) => void` | ||
General input change event handler. This will update the form value according to an input's `name` attribute. If `name` is not present, `handleChange` will look for an input's `id` attribute. Note: "input" here means all HTML inputs. | ||
##### `handleChangeValue: (name: string, value: any) => void` | ||
Custom input change handler. Use this when you have custom inputs. `name` should match the key of form value you wish to update. | ||
##### `handleReset: () => void` | ||
Reset handler. This should be passed to `<button onClick={handleReset}>...</button>` | ||
##### `handleSubmit: (e: React.FormEvent<HTMLFormEvent>) => void` | ||
Submit handler. This should be passed to `<form onSubmit={handleSubmit}>...</form>` | ||
##### `isSubmitting: boolean` | ||
Submitting state. Either `true` or `false`. Formik will set this to `true` on your behalf before calling `handleSubmit` to reduce boilerplate. | ||
##### `resetForm: (nextProps?: Props) => void` | ||
Imperatively reset the form. This will clear `errors` and `touched`, set `isSubmitting` to `false` and rerun `mapPropsToValues` with the current `WrappedComponent`'s `props` or what's passed as an argument. That latter is useful for calling `resetForm` within `componentWillReceiveProps`. | ||
##### `setError(err: any) => void` | ||
Set a top-level `error` object. This can only be done manually. It is an escape hatch. | ||
##### `setErrors(fields: { [field]: string }) => void` | ||
Set `errors` manually. | ||
##### `setSubmitting(boolean) => void` | ||
Set a `isSubmitting` manually. | ||
##### `setTouched(fields: { [field]: string }) => void` | ||
Set `touched` manually. | ||
##### `setValues(fields: { [field]: any }) => void` | ||
Set `values` manually. | ||
##### `touched: { [field]: string }` | ||
Touched fields. Use this to keep track of which fields have been visited. Use `handleBlur` to toggle on a given input. Keys work like `errors` and `values`. | ||
##### `values: { [field]: any }` | ||
Your form's values, the result of `mapPropsToValues` (if specified) or all props that are not functions passed to your `WrappedComponent`. | ||
## Recipes | ||
@@ -182,3 +278,3 @@ | ||
Formik is a Higher Order Component factory or "Monad" in functional programming lingo. In practice, you use it exactly like React Redux's `connect` or Apollo's `graphql`. Thus are basically three ways to call Formik on your component: | ||
Formik is a Higher Order Component factory or "Monad" in functional programming lingo. In practice, you use it exactly like React Redux's `connect` or Apollo's `graphql`. There are basically three ways to call Formik on your component: | ||
@@ -247,3 +343,3 @@ You can assign the HoC returned by Formik to a variable (i.e. `withFormik`) for later use. | ||
There isn't a hard rule whether one is better than the other. The decision comes down to either whether you want to colocate this logic with your form or not. (Note: if you need `refs` you'll need to convert your stateless functional form component into a React class anyway). | ||
There isn't a hard rule whether one is better than the other. The decision comes down to whether you want to colocate this logic with your form or not. (Note: if you need `refs` you'll need to convert your stateless functional form component into a React class anyway). | ||
@@ -272,3 +368,2 @@ #### Example: Resetting a form when props change | ||
type="text" | ||
ref={i => this.myInput = i} | ||
name="thing" | ||
@@ -289,45 +384,3 @@ value={this.props.values.thing} | ||
## API | ||
### `Formik(options)` | ||
Create a Formik Higher-order React component. | ||
#### Arguments | ||
- **`options`** (*Object*): Formik configuration object: | ||
- **`displayName: string`** (*string*): Set the display name of your component. | ||
- **`validationSchema: Schema`**: (*Yup Schema*): [A Yup schema](https://github.com/jquense/yup). This is used on each onChange event for validation. Errors are mapped by key to the `WrappedComponent`'s `props.errors`. Its keys should almost always match those of `WrappedComponent's` `props.values`. | ||
- **`mapPropsToValues?: (props) => props`** (*Function*): If this option is specified, then Formik will transfer its results into updatable form state and make these values available to the new component as `props.values`. If `mapPropsToValues` is **not** specified, then Formik will map all props that are not functions to the new component's `props.values`. That is, if you omit it, Formik will only pass `props` where `typeof props[k] !== 'function'`, where `k` is some key. | ||
- **`mapValuesToPayload?: (values) => payload`** (*Function*): If this option is specified, then Formik will run this function just before calling `handleSubmit`. Use it to transform the your form's `values` back into a shape that's consumable for other parts of your application or API. If `mapValuesToPayload` is **not** specified, then Formik will map all `values` directly to `payload` (which will be passed to `handleSubmit`). While this transformation can be moved into `handleSubmit`, consistently defining it in `mapValuesToPayload` separates concerns and helps you stay organized. | ||
- **`handleSubmit: (payload, FormikBag) => void`** (*Function*): Your form submission handler. It is passed the result of `mapValuesToPayload` (if specified), or the result of `mapPropsToValues`, or all props that are not functions (in that order of precedence) and the "`FormikBag`". | ||
#### Returns | ||
A higher-order React component class that passes props and form handlers ("the FormikBag") into your component derived from supplied options. | ||
##### Injected props and methods (a.k.a. the "Formik Bag") | ||
The following props and methods will be injected into the `WrappedComponent` (i.e. your form): | ||
- **`values: { [field]: any }`** Your form's values, the result of `mapPropsToValues` (if specified) or all props that are not functions passed to your `WrappedComponent`. | ||
- **`isSubmitting: boolean`**Submitting state. Either true or false. Formik will set this to true on your behalf before calling `handleSubmit` to reduce boilerplate. | ||
- **`errors: { [field]: string }`** Form validation errors. Keys match the shape of the `validationSchema` defined in Formik options. This should therefore also map to your `values` object as well. Internally, Formik transforms raw [Yup validation errors](https://github.com/jquense/yup#validationerrorerrors-string--arraystring-value-any-path-string) on your behalf. | ||
- **`error?: any`** - A top-level error object, can be whatever you need. | ||
- **`touched: { [field]: string }`** Touched fields. Use this to keep track of which fields have been visited. Use `handleBlur` to toggle on a given input. Keys work like `errors` and `values`. | ||
- **`handleBlur: (e: any) => void`** `onBlur` event handler. Useful for when you need to track whether an input has been `touched` or not. This should be passed to `<input onBlur={handleBlur} ... />` | ||
- **`handleSubmit: (e: React.FormEvent<HTMLFormEvent>) => void`** Submit handler. This should be passed to `<form onSubmit={handleSubmit}>...</form>` | ||
- **`handleReset: () => void`** Reset handler. This should be passed to `<button onClick={handleReset}>...</button>` | ||
- **`handleChange: (e: React.ChangeEvent<any>) => void`** General input change event handler. This will update the form value according to an input's `name` attribute. If `name` is not present, `handleChange` will look for an input's `id` attribute. Note: "input" here means all HTML inputs. | ||
- **`handleChangeValue: (name: string, value: any) => void`** Custom input change handler. Use this when you have custom inputs. `name` should match the key of form value you wish to update. | ||
- **`resetForm: (nextProps?: Props) => void`** Imperatively reset the form. This will clear `errors` and `touched`, set `isSubmitting` to `false` and rerun `mapPropsToValues` with the current `WrappedComponent`'s `props` or what's passed as an argument. That latter is useful for calling `resetForm` within `componentWillReceiveProps`. | ||
- **`setErrors(fields: { [field]: string }) => void`** Set `errors` manually. | ||
- **`setTouched(fields: { [field]: string }) => void`** Set `touched` manually. | ||
- **`setValues(fields: { [field]: any }) => void`** Set `values` manually. | ||
- **`setError(err: any) => void`** Set a top-level `error` object. This can only be done manually. It is an escape hatch. | ||
- **`setSubmitting(boolean) => void`** Set a `isSubmitting` manually. | ||
## Authors | ||
@@ -338,2 +391,2 @@ | ||
MIT License. | ||
MIT License. |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
162638
384