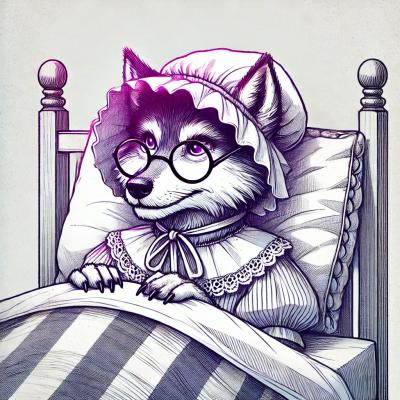
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
npm install formtools --save
Higher order components (HoC) for React to manage forms and fields.
These components are usefull for handling simple or medium-complex forms, and when you don't want to use tools like redux to manage the state of your fields.
The 'Field' HoC adds common behaviour to a form field, mainly normalization, validation, and keeping the field 'controlled'. The goal here is to keep your field completely stateless.
The 'Form' HoC acts as a state container with some extra functionalities. These functionalities are passed down as props.
These components are very unopinionated on how to inplement them in your app. They also don't depend on each other, which make them suitable for test.
// Field example A: just a controlled input
import React from 'react';
import { Field } from 'formtools';
function Input({ onChange, value }) {
return (
<input type="text" value={value} onChange={onChange} />
);
}
export default Field({
valueMap: event => event.target.value
})(Input);
// Field example B: more complex
import React from 'react';
import {
Field,
expect,
targetValue,
defaultFieldProps
} from 'formtools';
function Input({ error, success, label, setRef, ...props }) {
// determine the className for the input element
let cx;
if (error) cx = 'error';
if (success) cx = 'success';
return (
<div className="field">
<label htmlFor={props.name}>{label}</label>
<input
ref={setRef} // set ref, i.e. to call focus() in Form component
className={cx}
{...defaultFieldProps(props)} // helper which adds value, events, etc.
type="text"
/>
{error && <div className="errorMessage">{error}</div>}
</div>
);
}
export default Field({
valueMap: targetValue, // helper: event => event.target.value
normalize: val => val.toUpperCase(),
validate: [
// access child field properties within validation rules
expect((val, _, field) => field('name').value.length > 0, 'Please fill in your name first'),
expect(val => val.length >= 3, 'This value is to short'),
expect(val => val.length < 9, 'This value is to long')
]
})(Input);
// Form example:
import React, { Component } from 'react';
import { Form } from 'formtools';
import Zipcode from './Zipcode';
import checkZipcode from './api';
@Form()
class RegistrationForm extends Component {
handleSubmit = () => {
// function from HoC
const { validate, getValues, asyncError } = this.props;
// validate every field and display error / successs if possible
// return true of every field passes validation
if (!validate()) return;
const values = getValues();
// example: check with api if zipcode is valid
checkZipcode(values.zipcode)
.then(data => {
// on error manually overwrite error message of Zipcode field
if (!data) asyncError('zipcode', 'Invalid zipcode!');
// do something with all the field values :)
else someAction(values)
});
}
render() {
return (
<div>
<Zipcode name="zipcode">
... other fields
<button onClick={this.handleSubmit}>Submit</button>
</div>
)
}
}
Typical decorator pattern. Usage:
// es6
const Enhanced = Field({})(Component);
// es7
@Field({})
All of the config settings are totally optional.
valueMap
A function which describes where to find the value in a onChange-handler. Typical usecase is 'event => event.target.value'.
normalize
A function which takes a value and transforms it into another value. I.e.:
val => val.toUpperCase()
validation
Can be a function or an array of functions. Must be used together with to expect function. I.e:
expect((value, ownProps, otherField) => value.length > 0, 'Error message on failure')
expect calls your validator function with 3 arguments:
In case of multiple validators, the validation will stop on the first error, so it is wise to sort your validators by priority.
These props will be passed to the wrapped component:
if no validators are set, success and error both will stay false
Validation will occur:
Typical decorator pattern. Usage:
// es6
const Enhanced = Form()(Component);
// es7
@Form()
Exposed methods via props:
Returns an object with fieldnames as key and their corresponding values.
Validates all fields by default, unless a fieldname or an array of fieldnames is given, and returns true if all fields passed validation, false otherwise. Soft mode is disabled by default. When set to true, validation happens silently in the background without rendering error/success
Resets all fields by default, unless a fieldname or an array of fieldnames is given. All values of the field will be '' (empty), and the props success, error, touched and dirty will be set to false.
Same as clear(), except that reset will set the value back to initValue if possible
Manually overwrite an error message of a field after a async call.
Clears to above error and sets the validation before the async-error was set.
Returns a dom-node of a field. See setRef prop in Field.
Allows you to subscribe to common events. Usage:
this.props.events(
'onChange',
(value, name) => console.log(`The value of ${name} is '${value}'`)
);
Return an object with data:
FAQs
Higher order components for React to manage forms and fields
We found that formtools demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.