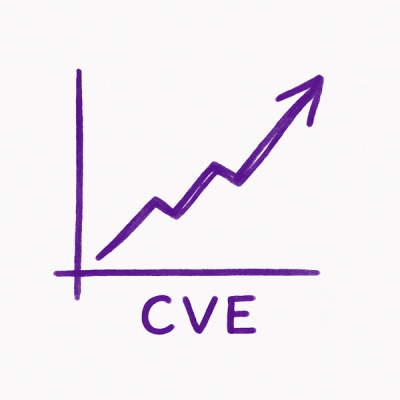
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Collection of file system utility functions for Gnome JavaScript (GJS).
fs-gjs
provides two sets of functions, synchronous and asynchronous. First can be accessed vias SyncFs
class and the latter via Fs
class. Both have almost exactly the same API, with the only difference that asynchronous functions need to be awaited.
import { Fs } from "./node_modules/fs-gjs/index.js";
// Read file as bytes
const bytes = await Fs.readFile("/path/to/file");
// Read file as text
const text = await Fs.readTextFile("/path/to/file");
import { Fs } from "./node_modules/fs-gjs/index.js";
// Write bytes to file
const bytes = new Uint8Array([1, 2, 3]);
await Fs.writeFile("/path/to/file", bytes);
// Write text to file
const text = "Hello, world!";
await Fs.writeTextFile("/path/to/file", text);
import { Fs } from "./node_modules/fs-gjs/index.js";
// Append bytes to file
const bytes = new Uint8Array([1, 2, 3]);
await Fs.appendFile("/path/to/file", bytes);
// Append text to file
const text = "Hello, world!";
await Fs.appendTextFile("/path/to/file", text);
import { Fs } from "./node_modules/fs-gjs/index.js";
await Fs.copyFile("/path/to/source", "/path/to/destination");
import { Fs } from "./node_modules/fs-gjs/index.js";
await Fs.moveFile("/path/to/source", "/path/to/destination");
import { Fs } from "./node_modules/fs-gjs/index.js";
// Permanently delete file
await Fs.deleteFile("/path/to/file");
// Move file to trash
await Fs.deleteFile("/path/to/file", { trash: true });
import { Fs } from "./node_modules/fs-gjs/index.js";
await Fs.makeDir("/path/to/directory");
import { Fs } from "./node_modules/fs-gjs/index.js";
await Fs.makeLink("/path/to/link", "/path/to/target");
import { Fs } from "./node_modules/fs-gjs/index.js";
await Fs.chmod("/path/to/file", 0o755);
// or
await Fs.chmod("/path/to/file", "rwxr-xr-x");
// or
await Fs.chmod("/path/to/file", {
owner: { read: true, write: true, execute: true },
group: { read: true, write: false, execute: true },
others: { read: true, write: false, execute: true },
});
import { Fs } from "./node_modules/fs-gjs/index.js";
await Fs.chown("/path/to/file", /* uid */ 1000, /* gid */ 1000);
import { Fs } from "./node_modules/fs-gjs/index.js";
// get array of FileInfo objects
await Fs.listDir("/path/to/directory");
// get array of file names
await Fs.listFilenames("/path/to/directory");
import { Fs } from "./node_modules/fs-gjs/index.js";
await Fs.fileExists("/path/to/file");
import { Fs } from "./node_modules/fs-gjs/index.js";
await Fs.fileInfo("/path/to/file");
IOStreams can be opened in one of three modes:
CREATE
- a new file will be created, will fail if file already existsREPLACE
- a new file will be created, will replace existing file if it existsOPEN
- an existing file will be opened, will fail if file does not existimport { Fs } from "./node_modules/fs-gjs/index.js";
const stream = await Fs.openIOStream("/path/to/file", "CREATE");
// Read the first 1024 bytes from stream
const bytes = await stream.read(1024);
// Read all the remaining bytes from stream
const bytes = await stream.readAll();
// Write bytes to stream
const bytes = new Uint8Array([1, 2, 3]);
await stream.write(bytes);
// Skip 1024 bytes from the stream
await stream.skip(1024);
// Move cursor position by 1024 bytes forward
await stream.seek(1024);
// Move cursor to 1024 from the stream start
await stream.seekFromStart(1024);
// Move cursor to 1024 from the stream end
await stream.seekFromEnd(1024);
// Truncate the stream to the length of 1024 bytes
await stream.truncate(1024);
// Get current cursor position
const position = await stream.currentPosition();
// Close the stream
await stream.close();
1.0.1 (July 27, 2023)
GLib.Bytes.unref_to_array
(#5)It was discovered that calls of the GLib.Bytes.unref_to_array
were leaving the underlying Bytes
object in a state that was causing GC to fail when trying to free up the memory taken by it. All the instances where this function was used have been updated to use the GLib.Bytes.toArray
function instead, which should fix this issue.
FAQs
Collection of file system utility functions for Gnome JavaScript (GJS).
The npm package fs-gjs receives a total of 7 weekly downloads. As such, fs-gjs popularity was classified as not popular.
We found that fs-gjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.