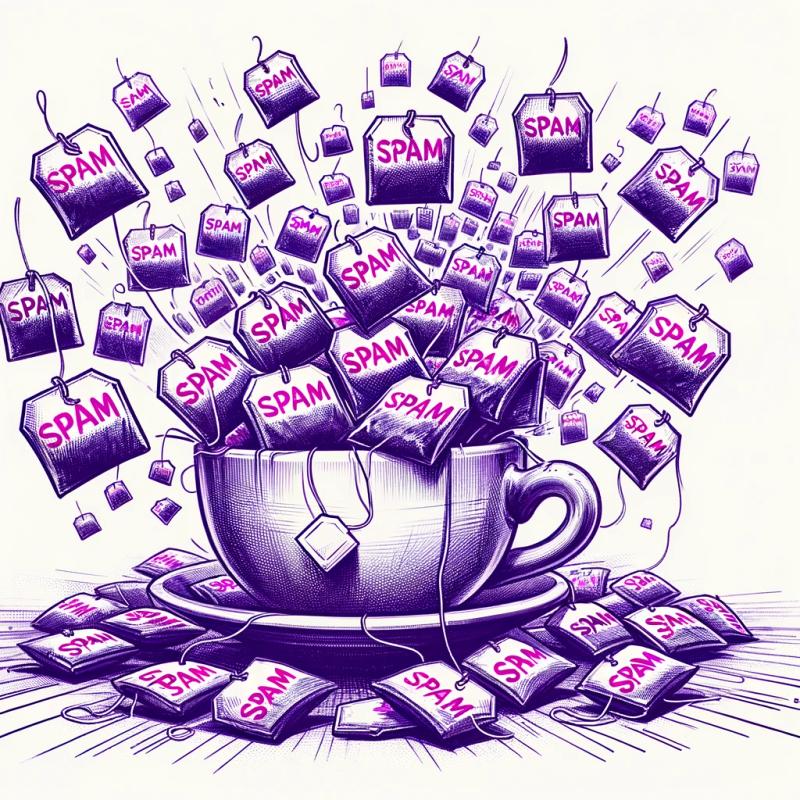
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
futoin-asyncevent
Advanced tools
Readme
Stability: 3 - Stable
Implementation of a well-known event emitter pattern, but with fundamental requirement: execute all events asynchronously - there must be no emitter functionality function frames on the stack.
All other event emitters implementations are synchronous - they call handlers when event is emitted.
As there is a known security-related timing problem of setTimeout()
calls in browser, enhanced
event loop is used from futoin-asyncsteps module.
Second important feature - strict check of allowed event types.
Documentation --> FutoIn Guide
Reference implementation of:
FTN15: Native Event API
Version: 1.1
Spec: FTN15: Native Event API v1.x
Author: Andrey Galkin
EventEmitter
instance is hidden in target[EventEmitter.SYM_EVENT_EMITTER]
property.
on()
, off()
, once()
and emit()
are defined as properties which proxy callemit()
uses ES6 spread oprerator (e.g. ...args
):
on()
/once()
calls are cheapoff()
call uses array#filter()
off()
calloff()
removes handler both from "on" and "once" arraysonce( 'event', () => o.once( 'event', ... ) )
approach IS NOT SAFE as it leads to missed events!Command line:
$ npm install futoin-asyncevent --save
or
$ yarn add futoin-asyncevent
Hint: checkout FutoIn CID for all tools setup.
Pre-built ES5 CJS modules are available under es5/
. These modules
can be used with webpack
without transpiler - default "browser" entry point
points to ES5 version.
Webpack dists are also available under dist/
folder, but their usage should be limited
to sites without build process.
Warning: older browsers should use dist/polyfill-asyncevent.js
for Symbol
.
The following globals are available:
const $asyncevent = require('futoin-asyncevent');
class FirstClass {
constructor() {
// dynamically extend & pre-configure allowed events
$asyncevent(this, ['event_one', 'event_two', 'event_three']);
}
someFunc() {
this.emit( 'event_one', 'some_arg', 2, true );
}
}
const o = new FirstClass();
const h = (a, b, c) => console.log(a, b, c);
// Basic event operation
// ---------------------
o.on('event_one', h);
o.off('event_one', h);
o.once('event_two', () => console.log('Second'));
o.someFunc();
// Advanced
// --------
// instanceof hook (ES6)
(o instanceof $asyncevent.EventEmitter) === true
// update max listeners warning threshold
$asyncevent.EventEmitter.setMaxListeners( o, 16 );
// Additional events in derived class
// ----------------------------------
class DerivedClass extends FirstClass {
constructor() {
super();
// Transparently checks, if EventEmitter has been already
// registered with other events
$asyncevent(this, ['another_event']);
}
}
// Fail on duplicate event names
// ----------------------------------
class FailClass extends FirstClass {
constructor() {
super();
// It's not allowed to override already registered event for
// safety reasons.
$asyncevent(this, ['event_one']);
}
}
The concept is described in FutoIn specification: FTN15: Native Event API v1.x
Asynchronous Event Emitter.
window.$asyncevent - browser-only reference to futoin-asyncsteps module
window.FutoIn.$asyncevent - browser-only reference to futoin-asyncsteps module
window.futoin.EventEmitter - browser-only reference to futoin-asyncsteps.EventEmitter
Attach event emitter properties to object. Call it in c-tor.
Asynchronous Event Emitter.
Kind: global class
Note: Please avoid inheriting it, use EventEmitter.attach() instead!
Attach event handler.
Kind: instance method of EventEmitter
Param | Type | Description |
---|---|---|
evt | string | preconfigured event name |
handler | callable | async event handler |
Attach once-only event handler.
Kind: instance method of EventEmitter
Param | Type | Description |
---|---|---|
evt | string | preconfigured event name |
handler | callable | async event handler |
Remove event handler.
Kind: instance method of EventEmitter
Param | Type | Description |
---|---|---|
evt | string | preconfigured event name |
handler | callable | async event handler |
Emit async event.
Kind: instance method of EventEmitter
Param | Type | Description |
---|---|---|
evt | string | event name |
Attach event emitter to any instance
Kind: static method of EventEmitter
Param | Type | Default | Description |
---|---|---|---|
instance | object | target object | |
allowed_events | array | list of allowed event names | |
max_listeners | integer | 8 | maximum allowed handlers per event name |
Dynamically update max listener count
Kind: static method of EventEmitter
Param | Type | Description |
---|---|---|
instance | object | target object |
max_listeners | integer | maximum allowed handlers per event name |
window.$asyncevent - browser-only reference to futoin-asyncsteps module
Reference to EventEmitter class
Kind: static property of $asyncevent
window.FutoIn.$asyncevent - browser-only reference to futoin-asyncsteps module
Reference to EventEmitter class
Kind: static property of $asyncevent
window.futoin.EventEmitter - browser-only reference to futoin-asyncsteps.EventEmitter
Kind: global variable
Attach event handler.
Kind: instance method of EventEmitter
Param | Type | Description |
---|---|---|
evt | string | preconfigured event name |
handler | callable | async event handler |
Attach once-only event handler.
Kind: instance method of EventEmitter
Param | Type | Description |
---|---|---|
evt | string | preconfigured event name |
handler | callable | async event handler |
Remove event handler.
Kind: instance method of EventEmitter
Param | Type | Description |
---|---|---|
evt | string | preconfigured event name |
handler | callable | async event handler |
Emit async event.
Kind: instance method of EventEmitter
Param | Type | Description |
---|---|---|
evt | string | event name |
Attach event emitter to any instance
Kind: static method of EventEmitter
Param | Type | Default | Description |
---|---|---|---|
instance | object | target object | |
allowed_events | array | list of allowed event names | |
max_listeners | integer | 8 | maximum allowed handlers per event name |
Dynamically update max listener count
Kind: static method of EventEmitter
Param | Type | Description |
---|---|---|
instance | object | target object |
max_listeners | integer | maximum allowed handlers per event name |
Attach event emitter properties to object. Call it in c-tor.
Kind: global constant
See: EventEmitter.attach()
Reference to EventEmitter class
Kind: static property of $asyncevent
documented by jsdoc-to-markdown.
FAQs
FutoIn AsyncEvent - FTN15 compliant event emitter
The npm package futoin-asyncevent receives a total of 2 weekly downloads. As such, futoin-asyncevent popularity was classified as not popular.
We found that futoin-asyncevent demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.