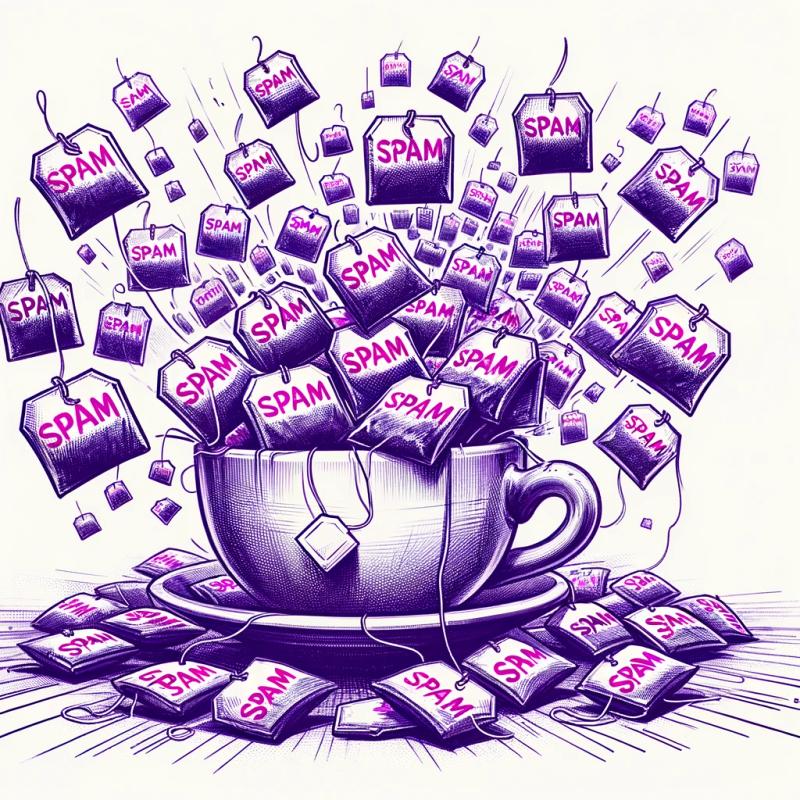
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
futoin-invoker
Advanced tools
Readme
Documentation --> FutoIn Guide.
FutoIn Invoker is request initiating part in FutoIn microservice concept. It invokes a FutoIn interface method as described in FTN3: FutoIn Interface Definition. Invoker is not necessary a client - e.g. server may initiate request for callback to client.
Unlike HTTP REST API, FutoIn perfectly fits for "all-in-one" process model with efficient internal calls. Invoker is heavily optimized to reliably process input and output data checks.
Strict FutoIn interface (iface) definition and transport protocol is defined in FTN3 spec mentioned above. As it is based on JSON, both client and server can be implemented in a few minutes almost in any technology. However, Invoker and Executor concept provide significant benefits for efficiency, reliability and error control.
The core of invoker is CCM - Connection and Credentials Manager. It has the following advantages:
The primary communication channel is WebSockets. Large raw data upload and download is also supported through automatic fallback to HTTP(S).
SimpleCCM
- a light version without heavy processing of iface definition (ideal for browser).AdvancedCCM
- full featured CCM (extends SimpleCCM).Communication methods:
Message coding formats:
BinaryData
constraintNote: Invoker and Executor are platform/technology-neutral concepts. The implementation is already available in JS and PHP.
Reference implementation of:
FTN7: FutoIn Invoker Concept
Version: 1.7
FTN3: FutoIn Interface Definition
Version: 1.9
FTN3.1: FutoIn Interface - Common Types
Version: 1.0
FTN5: FutoIn HTTP integration
Version: 1.3
FTN9: FutoIn Interface - AuditLog
Version: 1.0 (client)
FTN14: FutoIn Cache
Version: 1.0 (client)
FTN4: FutoIn Interface - Ping-Pong
Version: 1.0 (client)
Author: Andrey Galkin
Documentation --> FutoIn Guide
Command line:
$ npm install futoin-invoker --save
or
$ yarn add futoin-invoker
Hint: checkout FutoIn CID for all tools setup.
All public classes can be accessed through module:
const AdvancedCCM = require('futoin-invoker').AdvancedCCM;
or included modular way, e.g.:
const AdvancedCCM = require('futoin-invoker/AdvancedCCM');
Pre-built ES5 CJS modules are available under es5/
. These modules
can be used with webpack
without transpiler - default "browser" entry point
points to ES5 version.
Webpack dists are also available under dist/
folder, but their usage should be limited
to sites without build process.
Warning: check AsyncSteps and AsyncEvent polyfill for older browsers.
The following globals are available:
NOTE: more complex examples should be found in futoin-executor
var async_steps = require( 'futoin-asyncsteps' );
var SimpleCCM = require( 'futoin-invoker/SimpleCCM' );
// Initalize CCM, no configuration is required
var ccm = new SimpleCCM();
async_steps()
.add(
function( as ){
// Register interfaces without loading their specs
ccm.register( as, 'localone', 'some.iface:1.0',
'https://localhost/some/path' );
ccm.register( as, 'localtwo', 'other.iface:1.0',
'https://localhost/some/path',
'user:pass' ); // optional credentials
as.add( function( as ){
// Get NativeIface representation of remote interface
// after registration is complete
var localone = ccm.iface( 'localone' );
var localtwo = ccm.iface( 'localtwo' );
// SimpleCCM is not aware of available functions.
// It is the only way to perform a call.
localone.call( as, 'somefunc', {
arg1 : 1,
arg2 : 'abc',
arg3 : true,
} );
as.add( function( as, res ){
// As function prototype is not know
// all invalid HTTP 200 responses may
// get returned as "raw data" in res
// parameter.
console.log( res.result1, res.result2 );
} );
} );
},
function( as, err )
{
console.log( err + ': ' + as.state.error_info );
console.log( as.state.last_exception.stack );
}
)
.execute();
var async_steps = require( 'futoin-asyncsteps' );
var invoker = require( 'futoin-invoker' );
// Define interface, which should normally be put into
// file named "some.iface-1.0-iface.json" and put into
// a folder added to the "specDirs" option.
var some_iface_v1_0 = {
"iface" : "some.iface",
"version" : "1.0",
"ftn3rev" : "1.1",
"funcs" : {
"somefunc" : {
"params" : {
"arg1" : {
"type" : "integer"
},
"arg2" : {
"type" : "string"
},
"arg3" : {
"type" : "boolean"
}
},
"result" : {
"result1" : {
"type" : "number"
},
"result2" : {
"type" : "any"
}
},
"throws" : [
"MyError"
]
}
},
"requires" : [
"SecureChannel",
"AllowAnonymous"
]
};
var other_iface_v1_0 = {
"iface" : "other.iface",
"version" : "1.0",
"ftn3rev" : "1.1"
}
// Initialize CCM. We provide interface definitions directly
var ccm = new invoker.AdvancedCCM({
specDirs : [ __dirname + '/specs', some_iface_v1_0, other_iface_v1_0 ]
});
// AsyncSteps processing is required
async_steps()
.add(
function( as ){
// Register interfaces - it is done only once
ccm.register( as, 'localone',
'some.iface:1.0', 'https://localhost/some/path' );
ccm.register( as, 'localtwo',
'other.iface:1.0', 'https://localhost/some/path',
'user:pass' ); // optional credentials
as.add( function( as ){
// Get NativeIface representation of remote interface
// after registration is complete
var localone = ccm.iface( 'localone' );
var localtwo = ccm.iface( 'localtwo' );
localone.somefunc( as, 1, 'abc', true );
as.add( function( as, res ){
console.log( res.result1, res.result2 );
} );
} );
},
function( as, err )
{
console.log( err + ': ' + as.state.error_info );
console.log( as.state.last_exception.stack );
}
)
.execute();
The concept is described in FutoIn specification: FTN7: Interface Invoker Concept v1.x
SimpleCCM
Advanced CCM - Reference Implementation
NativeIface
Cache Native interface
Register with CacheFace.register()
NOTE: it is not directly available in Invoker module interface, include separately
FutoIn interface info
NativeIface
AuditLog Native interface
Register with LogFace.register().
NOTE: it is not directly available Invoker module interface, include separately
Native Interface for FutoIn ifaces
Base for FTN4 ping-based interfaces
Simple CCM - Reference Implementation
Base Connection and Credentials Manager with limited error control
SimpleCCMOptions
window.SimpleCCM - Browser-only reference to futoin-asyncsteps.SimpleCCM
window.SimpleCCM - Browser-only reference to futoin-asyncsteps.SimpleCCM
futoin.SimpleCCM - Browser-only reference to futoin-asyncsteps.SimpleCCM
window.AdvancedCCM - Browser-only reference to futoin-asyncsteps.AdvancedCCM
futoin.AdvancedCCM - Browser-only reference to futoin-asyncsteps.AdvancedCCM
futoin.Invoker - Browser-only reference to futoin-invoker module
window.FutoInInvoker - Browser-only reference to futoin-invoker module
SimpleCCM
Advanced CCM - Reference Implementation
Kind: global class
Extends: SimpleCCM
See
SimpleCCM
NativeInterface
object
object
object
Param | Type | Description |
---|---|---|
options | object | see AdvancedCCMOptions |
Register standard MasterService end-point (adds steps to as)
Kind: instance method of AdvancedCCM
Overrides: register
Emits: register
Param | Type | Description |
---|---|---|
as | AsyncSteps | AsyncSteps instance as registration may be waiting for external resources |
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
endpoint | string | URI OR any other resource identifier of function( ccmimpl, info ) returning iface implementing peer, accepted by CCM implementation OR instance of Executor |
[credentials] | string | optional, authentication credentials: 'master' - enable MasterService authentication logic (Advanced CCM only) '{user}:{clear-text-password}' - send as is in the 'sec' section NOTE: some more reserved words and/or patterns can appear in the future |
[options] | object | fine tune global CCM options per endpoint |
NativeInterface
Get native interface wrapper for invocation of iface methods
Kind: instance method of AdvancedCCM
Overrides: iface
Returns: NativeInterface
- - native interface
Param | Type | Description |
---|---|---|
name | string | see register() |
Unregister previously registered interface (should not be used, unless really needed)
Kind: instance method of AdvancedCCM
Overrides: unRegister
Emits: unregister
Param | Type | Description |
---|---|---|
name | string | see register() |
object
Shortcut to iface( "#defense" )
Kind: instance method of AdvancedCCM
Overrides: defense
Returns: object
- native defense interface
object
Returns extended API interface as defined in FTN9 IF AuditLogService
Kind: instance method of AdvancedCCM
Overrides: log
Returns: object
- FTN9 native face
object
Returns extended API interface as defined in [FTN14 Cache][]
Kind: instance method of AdvancedCCM
Overrides: cache
Returns: object
- FTN14 native face
Param | Type | Default | Description |
---|---|---|---|
[bucket] | string | "default" | cache bucket name |
Assert that interface registered by name matches major version and minor is not less than required. This function must generate fatal error and forbid any further execution
Kind: instance method of AdvancedCCM
Overrides: assertIface
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
Alias interface name with another name
Kind: instance method of AdvancedCCM
Overrides: alias
Emits: register
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
alias | string | alternative name for registered interface |
Shutdown CCM (close all active comms)
Kind: instance method of AdvancedCCM
Overrides: close
Emits: close
Configure named AsyncSteps Limiter instance
Kind: instance method of AdvancedCCM
Overrides: limitZone
Param | Type | Description |
---|---|---|
name | string | zone name |
options | object | options to pass to Limiter c-tor |
CCM regiser event. Fired on new interface registration. ( name, ifacever, info )
Kind: event emitted by AdvancedCCM
Overrides: register
CCM regiser event. Fired on interface unregistration. ( name, info )
Kind: event emitted by AdvancedCCM
Overrides: unregister
CCM close event. Fired on CCM shutdown.
Kind: event emitted by AdvancedCCM
Overrides: close
NativeIface
Cache Native interface
Register with CacheFace.register()
NOTE: it is not directly available in Invoker module interface, include separately
Kind: global class
Extends: NativeIface
Param | Type | Description |
---|---|---|
_ccm | SimpleCCM | CCM instance |
info | object | internal info |
Get or Set cached value
NOTE: the actual cache key is formed with concatenation of key_prefix and join of params values
Kind: instance method of CacheFace
Param | Type | Description |
---|---|---|
as | AsyncSteps | step interface |
key_prefix | string | unique key prefix |
callable | function | func( as, params.. ) - a callable which is called to generated value on cache miss |
params | Array | parameters to be passed to callable |
ttl_ms | integer | time to live in ms to use, if value is set on cache miss |
InterfaceInfo
Get interface info
Kind: instance method of CacheFace
Overrides: ifaceInfo
Returns: InterfaceInfo
- - interface info
Results with DerivedKeyAccessor through as.success()
Kind: instance method of CacheFace
Overrides: bindDerivedKey
Param | Type | Description |
---|---|---|
as | AsyncSteps | step interface |
Fired when interface establishes connection.
Kind: event emitted by CacheFace
Overrides: connect
Fired when interface connection is closed.
Kind: event emitted by CacheFace
Overrides: disconnect
Interface close event. Fired on interface unregistration.
Kind: event emitted by CacheFace
Overrides: close
Interface communication error. Fired during call processing. ( error_info, rawreq )
Kind: event emitted by CacheFace
Overrides: commError
Cache Native interface registration helper
Kind: static method of CacheFace
Param | Type | Default | Description |
---|---|---|---|
as | AsyncSteps | step interface | |
ccm | AdvancedCCM | CCM instance | |
name | string | registration name for CCM | |
endpoint | string | endpoint URL | |
[credentials] | * |
| see CCM register() |
[options] | object | {} | registration options |
[options.version] | string | "1.0" | iface version |
[options.ttl_ms] | integer | 1000 | default TTL |
FutoIn interface info
Kind: global class
string
string
string
object
array
Param | Type | Description |
---|---|---|
raw_info | object | futoin spec as is |
string
Get FutoIn interface name
Kind: instance method of InterfaceInfo
Returns: string
- name
string
Get FutoIn interface version
Kind: instance method of InterfaceInfo
Returns: string
- version
string
Get list of inherited interfaces starting from the most derived, may be null
Kind: instance method of InterfaceInfo
Returns: string
- inherited interface name-ver
object
Get list of available functions, may be null
Kind: instance method of InterfaceInfo
Returns: object
- list of functions
array
Get list of interface constraints, may be null
Kind: instance method of InterfaceInfo
Returns: array
- list of constraints
NativeIface
AuditLog Native interface
Register with LogFace.register().
NOTE: it is not directly available Invoker module interface, include separately
Kind: global class
Extends: NativeIface
NativeIface
Log message
Kind: instance method of LogFace
Param | Type | Description |
---|---|---|
lvl | string | debug |
txt | string | message to log |
Log message
Kind: instance method of LogFace
Param | Type | Description |
---|---|---|
lvl | string | debug |
txt | string | message to log |
data | string | raw data |
Log message in debug level
Kind: instance method of LogFace
Param | Type | Description |
---|---|---|
txt | string | message to log |
Log message in info level
Kind: instance method of LogFace
Param | Type | Description |
---|---|---|
txt | string | message to log |
Log message in warn level
Kind: instance method of LogFace
Param | Type | Description |
---|---|---|
txt | string | message to log |
Log message in error level
Kind: instance method of LogFace
Param | Type | Description |
---|---|---|
txt | string | message to log |
Log message in security level
Kind: instance method of LogFace
Param | Type | Description |
---|---|---|
txt | string | message to log |
InterfaceInfo
Get interface info
Kind: instance method of LogFace
Overrides: ifaceInfo
Returns: InterfaceInfo
- - interface info
Results with DerivedKeyAccessor through as.success()
Kind: instance method of LogFace
Overrides: bindDerivedKey
Param | Type | Description |
---|---|---|
as | AsyncSteps | step interface |
Fired when interface establishes connection.
Kind: event emitted by LogFace
Overrides: connect
Fired when interface connection is closed.
Kind: event emitted by LogFace
Overrides: disconnect
Interface close event. Fired on interface unregistration.
Kind: event emitted by LogFace
Overrides: close
Interface communication error. Fired during call processing. ( error_info, rawreq )
Kind: event emitted by LogFace
Overrides: commError
Debug log level
Kind: static constant of LogFace
Info log level
Kind: static constant of LogFace
Warn log level
Kind: static constant of LogFace
Error log level
Kind: static constant of LogFace
Security log level
Kind: static constant of LogFace
AuditLog Native interface registration helper
Kind: static method of LogFace
Param | Type | Default | Description |
---|---|---|---|
as | AsyncSteps | step interface | |
ccm | AdvancedCCM | CCM instance | |
endpoint | string | endpoint URL | |
[credentials] | * |
| see CCM register() |
[options] | object | {} | registration options |
[options.version] | string | "1.0" | iface version |
Native Interface for FutoIn ifaces
Kind: global class
Param | Type | Description |
---|---|---|
ccmimpl | AdvancedCCMImpl | CCM instance |
info | InterfaceInfo | interface info |
InterfaceInfo
Get interface info
Kind: instance method of NativeIface
Returns: InterfaceInfo
- - interface info
Results with DerivedKeyAccessor through as.success()
Kind: instance method of NativeIface
Param | Type | Description |
---|---|---|
as | AsyncSteps | step interface |
Fired when interface establishes connection.
Kind: event emitted by NativeIface
Fired when interface connection is closed.
Kind: event emitted by NativeIface
Interface close event. Fired on interface unregistration.
Kind: event emitted by NativeIface
Interface communication error. Fired during call processing. ( error_info, rawreq )
Kind: event emitted by NativeIface
Must be object with version => spec pairs in child class, if set.
Kind: static property of NativeIface
Must be module name prefix, example: 'MyModule/specs/name_'.
If version 1.0 is requested then spec is loaded from 'MyModule/specs/name_1_0'
Kind: static property of NativeIface
object
Get hardcoded iface definition, if available.
Kind: static method of NativeIface
Returns: object
- interface spec of required version
Note: this helper is designed for derived native interfaces
which define _specs or _specs_module_prefix static members.
Param | Type | Description |
---|---|---|
version | string | iface version |
Base for FTN4 ping-based interfaces
Register ping interface
Kind: static method of PingFace
Note: Iface spec is embedded
Param | Type | Default | Description |
---|---|---|---|
as | AsyncSteps | step interface | |
ccm | AdvancedCCM | CCM instance | |
name | string | registration name for CCM | |
endpoint | string | endpoint URL | |
[credentials] | * |
| see CCM register() |
[options] | object | {} | registration options |
[options.version] | string | "1.0" | iface version |
Simple CCM - Reference Implementation
Base Connection and Credentials Manager with limited error control
Kind: global class
See
Param | Type | Description |
---|---|---|
[options] | object | map of options |
Register standard MasterService end-point (adds steps to as)
Kind: instance method of SimpleCCM
Emits: register
Param | Type | Description |
---|---|---|
as | AsyncSteps | AsyncSteps instance as registration may be waiting for external resources |
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
endpoint | string | URI OR any other resource identifier of function( ccmimpl, info ) returning iface implementing peer, accepted by CCM implementation OR instance of Executor |
[credentials] | string | optional, authentication credentials: 'master' - enable MasterService authentication logic (Advanced CCM only) '{user}:{clear-text-password}' - send as is in the 'sec' section NOTE: some more reserved words and/or patterns can appear in the future |
[options] | object | fine tune global CCM options per endpoint |
NativeInterface
Get native interface wrapper for invocation of iface methods
Kind: instance method of SimpleCCM
Returns: NativeInterface
- - native interface
Param | Type | Description |
---|---|---|
name | string | see register() |
Unregister previously registered interface (should not be used, unless really needed)
Kind: instance method of SimpleCCM
Emits: unregister
Param | Type | Description |
---|---|---|
name | string | see register() |
object
Shortcut to iface( "#defense" )
Kind: instance method of SimpleCCM
Returns: object
- native defense interface
object
Returns extended API interface as defined in FTN9 IF AuditLogService
Kind: instance method of SimpleCCM
Returns: object
- FTN9 native face
object
Returns extended API interface as defined in [FTN14 Cache][]
Kind: instance method of SimpleCCM
Returns: object
- FTN14 native face
Param | Type | Default | Description |
---|---|---|---|
[bucket] | string | "default" | cache bucket name |
Assert that interface registered by name matches major version and minor is not less than required. This function must generate fatal error and forbid any further execution
Kind: instance method of SimpleCCM
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
Alias interface name with another name
Kind: instance method of SimpleCCM
Emits: register
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
alias | string | alternative name for registered interface |
Shutdown CCM (close all active comms)
Kind: instance method of SimpleCCM
Emits: close
Configure named AsyncSteps Limiter instance
Kind: instance method of SimpleCCM
Param | Type | Description |
---|---|---|
name | string | zone name |
options | object | options to pass to Limiter c-tor |
CCM regiser event. Fired on new interface registration. ( name, ifacever, info )
Kind: event emitted by SimpleCCM
CCM regiser event. Fired on interface unregistration. ( name, info )
Kind: event emitted by SimpleCCM
CCM close event. Fired on CCM shutdown.
Kind: event emitted by SimpleCCM
Maximum FutoIn message payload size (not related to raw data)
Kind: static constant of SimpleCCM
Default: 65536
Runtime iface resolution v1.x
Kind: static constant of SimpleCCM
AuthService v1.x
Kind: static constant of SimpleCCM
Defense system v1.x
Kind: static constant of SimpleCCM
Access Control system v1.x
Kind: static constant of SimpleCCM
Audit Logging v1.x
Kind: static constant of SimpleCCM
cache v1.x iface name prefix
Kind: static constant of SimpleCCM
Kind: global class
Buffer
boolean
object
boolean
Boolean
Boolean
Boolean
boolean
Boolean
SpecTools
Control JSON Schema validation in development.
Kind: static property of SpecTools
Param | Type | Description |
---|---|---|
set | boolean | value to set |
Enumeration of standard errors
Kind: static constant of SpecTools
Deprecated
Enumeration of standard errors
Kind: static constant of SpecTools
Load FutoIn iface definition.
NOTE: Browser uses XHR to load specs, Node.js searches in local fs.
Kind: static method of SpecTools
Param | Type | Description |
---|---|---|
as | AsyncSteps | step interface |
info | Object | destination object with "iface" and "version" fields already set |
specdirs | Array | each element - search path/url (string) or raw iface (object) |
[load_cache] | Object | arbitrary object to use for caching |
Buffer
Generate HMAC
NOTE: for simplicity, 'sec' field must not be present
Kind: static method of SpecTools
Returns: Buffer
- Binary HMAC signature
Throws:
FutoInError
Param | Type | Description |
---|---|---|
as | AsyncSteps | step interface |
info | object | Interface raw info object |
ftnreq | object | Request Object |
boolean
Secure compare to cover time-based side-channels for attacks
Kind: static method of SpecTools
Returns: boolean
- true, if match
Note: Pure JS is used in browser and crypto-based in Node.js
Param | Type | Description |
---|---|---|
a | string | first string |
b | string | second String |
Call after loading all depedency modules.
Mitigates CVE-2018-3721 and similar.
Kind: static method of SpecTools
object
Get process-wide load cache.
Kind: static method of SpecTools
Returns: object
- Global load cache instance.
boolean
Secure compare to cover time-based side-channels for attacks
Kind: static method of SpecTools
Returns: boolean
- true, if match
Param | Type | Description |
---|---|---|
a | Buffer | first buffer |
b | Buffer | second buffer |
Boolean
Check if value matches required type
Kind: static method of SpecTools
Returns: Boolean
- true on success
Param | Type | Description |
---|---|---|
as | AsyncSteps | AsyncSteps interface |
info | Object | previously loaded iface |
type | string | standard or custom iface type |
val | * | value to check |
Boolean
Check if request message is valid
Kind: static method of SpecTools
Returns: Boolean
- true on success
Param | Type | Description |
---|---|---|
as | AsyncSteps | AsyncSteps interface |
info | Object | previously loaded iface |
name | string | interface function name |
req | object | request message |
Boolean
Check if response message is valid
Kind: static method of SpecTools
Returns: Boolean
- true on success
Param | Type | Description |
---|---|---|
as | AsyncSteps | AsyncSteps interface |
info | Object | previously loaded iface |
name | string | interface function name |
rsp | object | response message |
boolean
Deprecated
Check if parameter value matches required type
Kind: static method of SpecTools
Returns: boolean
- true on success
Param | Type | Description |
---|---|---|
info | Object | previously loaded iface |
funcname | string | function name |
varname | string | parameter name |
value | * | value to check |
Deprecated
Check if result value matches required type
Kind: static method of SpecTools
Param | Type | Description |
---|---|---|
as | AsyncSteps | step interface |
info | Object | previously loaded iface |
funcname | string | function name |
varname | string | result variable name |
value | * | value to check |
Parse raw futoin spec (preloaded)
Kind: static method of SpecTools
Param | Type | Description |
---|---|---|
as | AsyncSteps | step interface |
info | Object | destination object with "iface" and "version" fields already set |
specdirs | Array | each element - search path/url (string) or raw iface (object) |
raw_spec | Object | iface definition object |
[load_cache] | Object | cache of already loaded interfaces |
Boolean
Check if value matches required type
Kind: static method of SpecTools
Returns: Boolean
- true on success
Note: THIS ONE IS SLOW for debugging purposes
See: SpecTools#checkCompiledType
Param | Type | Description |
---|---|---|
info | Object | previously loaded iface |
type | string | standard or custom iface type |
val | * | value to check |
On error message for details in debugging.
Kind: event emitted by SpecTools
SimpleCCMOptions
Kind: global class
Extends: SimpleCCMOptions
This is a pseudo-class for documentation purposes
NOTE: Each option can be set on global level and overriden per interface.
Search dirs for spec definition or spec instance directly. It can be single value or array of values. Each value is either path/URL (string) or iface spec instance (object).
Kind: static property of AdvancedCCMOptions
Default: []
Base64 encoded key for MAC generation. See FTN8
Kind: static property of AdvancedCCMOptions
Hash algorithm for HMAC generation: HMD5, HS256 (default), HS384, HS512
Kind: static property of AdvancedCCMOptions
Deprecated
Base64 encoded key for legacy HMAC generation. See FTN6/FTN7
Kind: static property of AdvancedCCMOptions
Deprecated
Hash algorithm for legacy HMAC generation: MD5(default), SHA224, SHA256, SHA384, SHA512
Kind: static property of AdvancedCCMOptions
Send "obf" (On Behalf Of) user information as defined in FTN3 v1.3 when invoked from Executor's request processing task
Kind: static property of AdvancedCCMOptions
Default: true
Instance implementing MasterAuth interface
Kind: static property of AdvancedCCMOptions
Kind: global class
This is a pseudo-class for documentation purposes.
NOTE: Each option can be set on global level and overriden per interface.
Overall call timeout (int)
Kind: static property of SimpleCCMOptions
Default: 3000
Production mode - disables some checks without compomising security
Kind: static property of SimpleCCMOptions
Default: NODE_ENV === 'production'
Communication configuration callback( type, specific-args )
Kind: static property of SimpleCCMOptions
Default:
Client-side executor for bi-directional communication channels
Kind: static property of SimpleCCMOptions
browser-only. Origin of target for window.postMessage()
Kind: static property of SimpleCCMOptions
How many times to retry the call on CommError. NOTE: actual attempt count is retryCount + 1
Kind: static property of SimpleCCMOptions
Default: 1
Which message coder to use by default.
Kind: static property of SimpleCCMOptions
Default: JSON
Which message coder to use for BinaryData interfaces.
Kind: static property of SimpleCCMOptions
Default: MPCK
Enables marking as SecureChannel through options.
Kind: static property of SimpleCCMOptions
Default: false
Maximum number of concurrent requests per communication channel.
Kind: static property of SimpleCCMOptions
Default: 16
Message sniffer callback( iface_info, msg, is_incomming ). Useful for audit logging.
Kind: static method of SimpleCCMOptions
Default: dummy
Bi-directional channel disconnect sniffer callback( iface_info ). Useful for audit logging.
Kind: static method of SimpleCCMOptions
Default: dummy
window.SimpleCCM - Browser-only reference to futoin-asyncsteps.SimpleCCM
Kind: global variable
Param | Type | Description |
---|---|---|
[options] | object | map of options |
Register standard MasterService end-point (adds steps to as)
Kind: instance method of SimpleCCM
Emits: register
Param | Type | Description |
---|---|---|
as | AsyncSteps | AsyncSteps instance as registration may be waiting for external resources |
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
endpoint | string | URI OR any other resource identifier of function( ccmimpl, info ) returning iface implementing peer, accepted by CCM implementation OR instance of Executor |
[credentials] | string | optional, authentication credentials: 'master' - enable MasterService authentication logic (Advanced CCM only) '{user}:{clear-text-password}' - send as is in the 'sec' section NOTE: some more reserved words and/or patterns can appear in the future |
[options] | object | fine tune global CCM options per endpoint |
NativeInterface
Get native interface wrapper for invocation of iface methods
Kind: instance method of SimpleCCM
Returns: NativeInterface
- - native interface
Param | Type | Description |
---|---|---|
name | string | see register() |
Unregister previously registered interface (should not be used, unless really needed)
Kind: instance method of SimpleCCM
Emits: unregister
Param | Type | Description |
---|---|---|
name | string | see register() |
object
Shortcut to iface( "#defense" )
Kind: instance method of SimpleCCM
Returns: object
- native defense interface
object
Returns extended API interface as defined in FTN9 IF AuditLogService
Kind: instance method of SimpleCCM
Returns: object
- FTN9 native face
object
Returns extended API interface as defined in [FTN14 Cache][]
Kind: instance method of SimpleCCM
Returns: object
- FTN14 native face
Param | Type | Default | Description |
---|---|---|---|
[bucket] | string | "default" | cache bucket name |
Assert that interface registered by name matches major version and minor is not less than required. This function must generate fatal error and forbid any further execution
Kind: instance method of SimpleCCM
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
Alias interface name with another name
Kind: instance method of SimpleCCM
Emits: register
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
alias | string | alternative name for registered interface |
Shutdown CCM (close all active comms)
Kind: instance method of SimpleCCM
Emits: close
Configure named AsyncSteps Limiter instance
Kind: instance method of SimpleCCM
Param | Type | Description |
---|---|---|
name | string | zone name |
options | object | options to pass to Limiter c-tor |
CCM regiser event. Fired on new interface registration. ( name, ifacever, info )
Kind: event emitted by SimpleCCM
CCM regiser event. Fired on interface unregistration. ( name, info )
Kind: event emitted by SimpleCCM
CCM close event. Fired on CCM shutdown.
Kind: event emitted by SimpleCCM
Maximum FutoIn message payload size (not related to raw data)
Kind: static constant of SimpleCCM
Default: 65536
Runtime iface resolution v1.x
Kind: static constant of SimpleCCM
AuthService v1.x
Kind: static constant of SimpleCCM
Defense system v1.x
Kind: static constant of SimpleCCM
Access Control system v1.x
Kind: static constant of SimpleCCM
Audit Logging v1.x
Kind: static constant of SimpleCCM
cache v1.x iface name prefix
Kind: static constant of SimpleCCM
window.SimpleCCM - Browser-only reference to futoin-asyncsteps.SimpleCCM
Kind: global variable
Param | Type | Description |
---|---|---|
[options] | object | map of options |
Register standard MasterService end-point (adds steps to as)
Kind: instance method of SimpleCCM
Emits: register
Param | Type | Description |
---|---|---|
as | AsyncSteps | AsyncSteps instance as registration may be waiting for external resources |
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
endpoint | string | URI OR any other resource identifier of function( ccmimpl, info ) returning iface implementing peer, accepted by CCM implementation OR instance of Executor |
[credentials] | string | optional, authentication credentials: 'master' - enable MasterService authentication logic (Advanced CCM only) '{user}:{clear-text-password}' - send as is in the 'sec' section NOTE: some more reserved words and/or patterns can appear in the future |
[options] | object | fine tune global CCM options per endpoint |
NativeInterface
Get native interface wrapper for invocation of iface methods
Kind: instance method of SimpleCCM
Returns: NativeInterface
- - native interface
Param | Type | Description |
---|---|---|
name | string | see register() |
Unregister previously registered interface (should not be used, unless really needed)
Kind: instance method of SimpleCCM
Emits: unregister
Param | Type | Description |
---|---|---|
name | string | see register() |
object
Shortcut to iface( "#defense" )
Kind: instance method of SimpleCCM
Returns: object
- native defense interface
object
Returns extended API interface as defined in FTN9 IF AuditLogService
Kind: instance method of SimpleCCM
Returns: object
- FTN9 native face
object
Returns extended API interface as defined in [FTN14 Cache][]
Kind: instance method of SimpleCCM
Returns: object
- FTN14 native face
Param | Type | Default | Description |
---|---|---|---|
[bucket] | string | "default" | cache bucket name |
Assert that interface registered by name matches major version and minor is not less than required. This function must generate fatal error and forbid any further execution
Kind: instance method of SimpleCCM
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
Alias interface name with another name
Kind: instance method of SimpleCCM
Emits: register
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
alias | string | alternative name for registered interface |
Shutdown CCM (close all active comms)
Kind: instance method of SimpleCCM
Emits: close
Configure named AsyncSteps Limiter instance
Kind: instance method of SimpleCCM
Param | Type | Description |
---|---|---|
name | string | zone name |
options | object | options to pass to Limiter c-tor |
CCM regiser event. Fired on new interface registration. ( name, ifacever, info )
Kind: event emitted by SimpleCCM
CCM regiser event. Fired on interface unregistration. ( name, info )
Kind: event emitted by SimpleCCM
CCM close event. Fired on CCM shutdown.
Kind: event emitted by SimpleCCM
Maximum FutoIn message payload size (not related to raw data)
Kind: static constant of SimpleCCM
Default: 65536
Runtime iface resolution v1.x
Kind: static constant of SimpleCCM
AuthService v1.x
Kind: static constant of SimpleCCM
Defense system v1.x
Kind: static constant of SimpleCCM
Access Control system v1.x
Kind: static constant of SimpleCCM
Audit Logging v1.x
Kind: static constant of SimpleCCM
cache v1.x iface name prefix
Kind: static constant of SimpleCCM
futoin.SimpleCCM - Browser-only reference to futoin-asyncsteps.SimpleCCM
Kind: global variable
Param | Type | Description |
---|---|---|
[options] | object | map of options |
Register standard MasterService end-point (adds steps to as)
Kind: instance method of SimpleCCM
Emits: register
Param | Type | Description |
---|---|---|
as | AsyncSteps | AsyncSteps instance as registration may be waiting for external resources |
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
endpoint | string | URI OR any other resource identifier of function( ccmimpl, info ) returning iface implementing peer, accepted by CCM implementation OR instance of Executor |
[credentials] | string | optional, authentication credentials: 'master' - enable MasterService authentication logic (Advanced CCM only) '{user}:{clear-text-password}' - send as is in the 'sec' section NOTE: some more reserved words and/or patterns can appear in the future |
[options] | object | fine tune global CCM options per endpoint |
NativeInterface
Get native interface wrapper for invocation of iface methods
Kind: instance method of SimpleCCM
Returns: NativeInterface
- - native interface
Param | Type | Description |
---|---|---|
name | string | see register() |
Unregister previously registered interface (should not be used, unless really needed)
Kind: instance method of SimpleCCM
Emits: unregister
Param | Type | Description |
---|---|---|
name | string | see register() |
object
Shortcut to iface( "#defense" )
Kind: instance method of SimpleCCM
Returns: object
- native defense interface
object
Returns extended API interface as defined in FTN9 IF AuditLogService
Kind: instance method of SimpleCCM
Returns: object
- FTN9 native face
object
Returns extended API interface as defined in [FTN14 Cache][]
Kind: instance method of SimpleCCM
Returns: object
- FTN14 native face
Param | Type | Default | Description |
---|---|---|---|
[bucket] | string | "default" | cache bucket name |
Assert that interface registered by name matches major version and minor is not less than required. This function must generate fatal error and forbid any further execution
Kind: instance method of SimpleCCM
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
Alias interface name with another name
Kind: instance method of SimpleCCM
Emits: register
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
alias | string | alternative name for registered interface |
Shutdown CCM (close all active comms)
Kind: instance method of SimpleCCM
Emits: close
Configure named AsyncSteps Limiter instance
Kind: instance method of SimpleCCM
Param | Type | Description |
---|---|---|
name | string | zone name |
options | object | options to pass to Limiter c-tor |
CCM regiser event. Fired on new interface registration. ( name, ifacever, info )
Kind: event emitted by SimpleCCM
CCM regiser event. Fired on interface unregistration. ( name, info )
Kind: event emitted by SimpleCCM
CCM close event. Fired on CCM shutdown.
Kind: event emitted by SimpleCCM
Maximum FutoIn message payload size (not related to raw data)
Kind: static constant of SimpleCCM
Default: 65536
Runtime iface resolution v1.x
Kind: static constant of SimpleCCM
AuthService v1.x
Kind: static constant of SimpleCCM
Defense system v1.x
Kind: static constant of SimpleCCM
Access Control system v1.x
Kind: static constant of SimpleCCM
Audit Logging v1.x
Kind: static constant of SimpleCCM
cache v1.x iface name prefix
Kind: static constant of SimpleCCM
window.AdvancedCCM - Browser-only reference to futoin-asyncsteps.AdvancedCCM
Kind: global variable
NativeInterface
object
object
object
Param | Type | Description |
---|---|---|
options | object | see AdvancedCCMOptions |
Register standard MasterService end-point (adds steps to as)
Kind: instance method of AdvancedCCM
Overrides: register
Emits: register
Param | Type | Description |
---|---|---|
as | AsyncSteps | AsyncSteps instance as registration may be waiting for external resources |
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
endpoint | string | URI OR any other resource identifier of function( ccmimpl, info ) returning iface implementing peer, accepted by CCM implementation OR instance of Executor |
[credentials] | string | optional, authentication credentials: 'master' - enable MasterService authentication logic (Advanced CCM only) '{user}:{clear-text-password}' - send as is in the 'sec' section NOTE: some more reserved words and/or patterns can appear in the future |
[options] | object | fine tune global CCM options per endpoint |
NativeInterface
Get native interface wrapper for invocation of iface methods
Kind: instance method of AdvancedCCM
Overrides: iface
Returns: NativeInterface
- - native interface
Param | Type | Description |
---|---|---|
name | string | see register() |
Unregister previously registered interface (should not be used, unless really needed)
Kind: instance method of AdvancedCCM
Overrides: unRegister
Emits: unregister
Param | Type | Description |
---|---|---|
name | string | see register() |
object
Shortcut to iface( "#defense" )
Kind: instance method of AdvancedCCM
Overrides: defense
Returns: object
- native defense interface
object
Returns extended API interface as defined in FTN9 IF AuditLogService
Kind: instance method of AdvancedCCM
Overrides: log
Returns: object
- FTN9 native face
object
Returns extended API interface as defined in [FTN14 Cache][]
Kind: instance method of AdvancedCCM
Overrides: cache
Returns: object
- FTN14 native face
Param | Type | Default | Description |
---|---|---|---|
[bucket] | string | "default" | cache bucket name |
Assert that interface registered by name matches major version and minor is not less than required. This function must generate fatal error and forbid any further execution
Kind: instance method of AdvancedCCM
Overrides: assertIface
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
Alias interface name with another name
Kind: instance method of AdvancedCCM
Overrides: alias
Emits: register
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
alias | string | alternative name for registered interface |
Shutdown CCM (close all active comms)
Kind: instance method of AdvancedCCM
Overrides: close
Emits: close
Configure named AsyncSteps Limiter instance
Kind: instance method of AdvancedCCM
Overrides: limitZone
Param | Type | Description |
---|---|---|
name | string | zone name |
options | object | options to pass to Limiter c-tor |
CCM regiser event. Fired on new interface registration. ( name, ifacever, info )
Kind: event emitted by AdvancedCCM
Overrides: register
CCM regiser event. Fired on interface unregistration. ( name, info )
Kind: event emitted by AdvancedCCM
Overrides: unregister
CCM close event. Fired on CCM shutdown.
Kind: event emitted by AdvancedCCM
Overrides: close
futoin.AdvancedCCM - Browser-only reference to futoin-asyncsteps.AdvancedCCM
Kind: global variable
NativeInterface
object
object
object
Param | Type | Description |
---|---|---|
options | object | see AdvancedCCMOptions |
Register standard MasterService end-point (adds steps to as)
Kind: instance method of AdvancedCCM
Overrides: register
Emits: register
Param | Type | Description |
---|---|---|
as | AsyncSteps | AsyncSteps instance as registration may be waiting for external resources |
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
endpoint | string | URI OR any other resource identifier of function( ccmimpl, info ) returning iface implementing peer, accepted by CCM implementation OR instance of Executor |
[credentials] | string | optional, authentication credentials: 'master' - enable MasterService authentication logic (Advanced CCM only) '{user}:{clear-text-password}' - send as is in the 'sec' section NOTE: some more reserved words and/or patterns can appear in the future |
[options] | object | fine tune global CCM options per endpoint |
NativeInterface
Get native interface wrapper for invocation of iface methods
Kind: instance method of AdvancedCCM
Overrides: iface
Returns: NativeInterface
- - native interface
Param | Type | Description |
---|---|---|
name | string | see register() |
Unregister previously registered interface (should not be used, unless really needed)
Kind: instance method of AdvancedCCM
Overrides: unRegister
Emits: unregister
Param | Type | Description |
---|---|---|
name | string | see register() |
object
Shortcut to iface( "#defense" )
Kind: instance method of AdvancedCCM
Overrides: defense
Returns: object
- native defense interface
object
Returns extended API interface as defined in FTN9 IF AuditLogService
Kind: instance method of AdvancedCCM
Overrides: log
Returns: object
- FTN9 native face
object
Returns extended API interface as defined in [FTN14 Cache][]
Kind: instance method of AdvancedCCM
Overrides: cache
Returns: object
- FTN14 native face
Param | Type | Default | Description |
---|---|---|---|
[bucket] | string | "default" | cache bucket name |
Assert that interface registered by name matches major version and minor is not less than required. This function must generate fatal error and forbid any further execution
Kind: instance method of AdvancedCCM
Overrides: assertIface
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
ifacever | string | interface identifier and its version separated by colon |
Alias interface name with another name
Kind: instance method of AdvancedCCM
Overrides: alias
Emits: register
Param | Type | Description |
---|---|---|
name | string | unique identifier in scope of CCM instance |
alias | string | alternative name for registered interface |
Shutdown CCM (close all active comms)
Kind: instance method of AdvancedCCM
Overrides: close
Emits: close
Configure named AsyncSteps Limiter instance
Kind: instance method of AdvancedCCM
Overrides: limitZone
Param | Type | Description |
---|---|---|
name | string | zone name |
options | object | options to pass to Limiter c-tor |
CCM regiser event. Fired on new interface registration. ( name, ifacever, info )
Kind: event emitted by AdvancedCCM
Overrides: register
CCM regiser event. Fired on interface unregistration. ( name, info )
Kind: event emitted by AdvancedCCM
Overrides: unregister
CCM close event. Fired on CCM shutdown.
Kind: event emitted by AdvancedCCM
Overrides: close
futoin.Invoker - Browser-only reference to futoin-invoker module
window.FutoInInvoker - Browser-only reference to futoin-invoker module
Kind: global variable
documented by jsdoc-to-markdown.
FAQs
Transparently, efficiently and securely invoke remote or local service methods with strict API definition for Node and Browser
The npm package futoin-invoker receives a total of 17 weekly downloads. As such, futoin-invoker popularity was classified as not popular.
We found that futoin-invoker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.