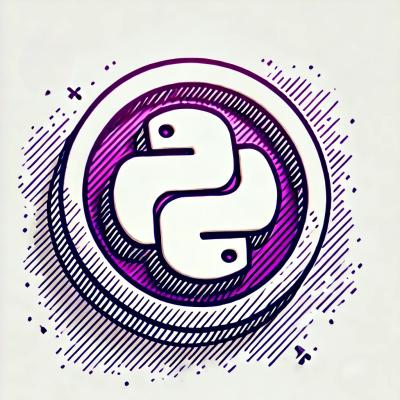
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
The 'got' npm package is a human-friendly and powerful HTTP request library for Node.js. It provides an easy-to-use API for making HTTP requests and supports many features like streams, pagination, JSON parsing, and more.
Simplified HTTP requests
This feature allows you to perform HTTP GET requests with a promise-based API. The example shows how to fetch a webpage and log the HTML content.
const got = require('got');
got('https://sindresorhus.com').then(response => {
console.log(response.body);
}).catch(error => {
console.log(error.response.body);
});
JSON support
This feature automatically parses JSON responses. The example demonstrates fetching JSON data from an API and logging the parsed object.
const got = require('got');
got('https://api.example.com/data', { responseType: 'json' }).then(response => {
console.log(response.body);
}).catch(error => {
console.log(error.response.body);
});
POST requests
This feature allows you to send POST requests with JSON bodies. The example shows how to send a POST request with a JSON payload and receive a JSON response.
const got = require('got');
got.post('https://api.example.com/submit', {
json: {
key: 'value'
},
responseType: 'json'
}).then(response => {
console.log(response.body);
}).catch(error => {
console.log(error.response.body);
});
Error handling
This feature provides comprehensive error handling for various types of request failures. The example demonstrates how to handle different error scenarios when a request fails.
const got = require('got');
got('https://api.example.com/wrong-endpoint').then(response => {
console.log(response.body);
}).catch(error => {
if (error.response) {
console.log('The server responded with a non-2xx status code.');
} else if (error.request) {
console.log('The request was made but no response was received');
} else {
console.log('An error occurred when trying to perform the request.');
}
});
Stream support
This feature allows you to use got as a stream. The example shows how to stream a webpage's content and write it to a file.
const got = require('got');
const fs = require('fs');
const stream = got.stream('https://sindresorhus.com');
stream.pipe(fs.createWriteStream('index.html'));
Axios is a promise-based HTTP client for the browser and Node.js. It provides an API similar to got but also works in the browser. Axios has interceptors that allow you to transform requests and responses before they are handled by then or catch.
Request is a simplified HTTP request client that was very popular but is now deprecated. It had a callback-based API but also supported promises. Got is considered a modern alternative to Request with promise support by default.
Node-fetch is a light-weight module that brings the Fetch API to Node.js. It is a minimalistic and straightforward API that resembles the Fetch API provided by modern browsers, making it familiar to front-end developers.
Superagent is a small progressive client-side HTTP request library. It has a fluent API that allows chaining methods to configure requests, and it can be used on both server and client side. Compared to got, it has a more object-oriented style.
Simplified HTTP/HTTPS requests
A nicer interface to the built-in http
module.
It supports following redirects, promises, streams, retries, automagically handling gzip/deflate and some convenience options.
Created because request
is bloated (several megabytes!).
$ npm install --save got
const got = require('got');
// Callback mode
got('todomvc.com', (error, body, response) => {
console.log(body);
//=> '<!doctype html> ...'
});
// Promise mode
got('todomvc.com')
.then(response => {
console.log(response.body);
//=> '<!doctype html> ...'
})
.catch(error => {
console.log(error.response.body);
//=> 'Internal server error ...'
});
// Stream mode
got.stream('todomvc.com').pipe(fs.createWriteStream('index.html'));
// For POST, PUT and PATCH methods got.stream returns a WritableStream
fs.createReadStream('index.html').pipe(got.stream.post('todomvc.com'));
It's a GET
request by default, but can be changed in options
.
Returns a Promise for a response
object with a body
property, a url
property with the request URL or the final URL after redirects, and a requestUrl
property with the original request URL.
Otherwise calls callback with response
object (same as in previous case).
Type: string
, object
The URL to request or a http.request
options object.
Properties from options
will override properties in the parsed url
.
Type: object
Any of the http.request
options.
Type: string
, buffer
, readableStream
, object
This is mutually exclusive with stream mode.
Body that will be sent with a POST
request.
If present in options
and options.method
is not set, options.method
will be set to POST
.
If content-length
or transfer-encoding
is not set in options.headers
and body
is a string or buffer, content-length
will be set to the body length.
If body
is a plain object, it will be stringified with querystring.stringify
and sent as application/x-www-form-urlencoded
.
Type: string
, null
Default: 'utf8'
Encoding to be used on setEncoding
of the response data. If null
, the body is returned as a Buffer.
Type: boolean
Default: false
This is mutually exclusive with stream mode.
Parse response body with JSON.parse
and set accept
header to application/json
.
Type: string
, object
Query string object that will be added to the request URL. This will override the query string in url
.
Type: number
Milliseconds after which the request will be aborted and an error event with ETIMEDOUT
code will be emitted.
Type: number
, function
Default: 5
Number of request retries when network errors happens. Delays between retries counts with function 1000 * Math.pow(2, retry) + Math.random() * 100
, where retry
is attempt number (starts from 0).
Option accepts function
with retry
and error
arguments. Function must return delay in milliseconds (0
return value cancels retry).
Note: if retries
is number
, ENOTFOUND
and ENETUNREACH
error will not be retried (see full list in is-retry-allowed
module).
Type: boolean
Default: true
Defines if redirect responses should be followed automatically.
Function to be called when error or data are received. If omitted, a promise will be returned.
Error
object with HTTP status code as statusCode
property.
The data you requested.
The response object.
When in stream mode, you can listen for events:
request
event to get the request object of the request.
Tip: You can use request
event to abort request:
got.stream('github.com')
.on('request', req => setTimeout(() => req.abort(), 50));
response
event to get the response object of the final request.
redirect
event to get the response object of a redirect. The second argument is options for the next request to the redirect location.
error
event emitted in case of protocol error (like ENOTFOUND
etc.) or status error (4xx or 5xx). The second argument is the body of the server response in case of status error. The third argument is response object.
Sets options.method
to the method name and makes a request.
Each error contains (if available) statusCode
, statusMessage
, host
, hostname
, method
and path
properties to make debugging easier.
In Promise mode, the response
is attached to the error.
When a request fails. Contains a code
property with error class code, like ECONNREFUSED
.
When reading from response stream fails.
When json
option is enabled and JSON.parse
fails.
When server response code is not 2xx. Contains statusCode
and statusMessage
.
When server redirects you more than 10 times.
You can use the tunnel
module with the agent
option to work with proxies:
const got = require('got');
const tunnel = require('tunnel');
got('todomvc.com', {
agent: tunnel.httpOverHttp({
proxy: {
host: 'localhost'
}
})
}, () => {});
You can use the cookie
module to include cookies in a request:
const got = require('got');
const cookie = require('cookie');
got('google.com', {
headers: {
cookie: cookie.serialize('foo', 'bar')
}
});
You can use the form-data
module to create POST request with form data:
const fs = require('fs');
const got = require('got');
const FormData = require('form-data');
const form = new FormData();
form.append('my_file', fs.createReadStream('/foo/bar.jpg'));
got.post('google.com', {
body: form
});
You can use the oauth-1.0a
module to create a signed OAuth request:
const got = require('got');
const OAuth = require('oauth-1.0a');
const oauth = OAuth({
consumer: {
public: process.env.CONSUMER_KEY,
secret: process.env.CONSUMER_SECRET
},
signature_method: 'HMAC-SHA1'
});
const token = {
public: process.env.ACCESS_TOKEN,
secret: process.env.ACCESS_TOKEN_SECRET
};
const url = 'https://api.twitter.com/1.1/statuses/home_timeline.json';
got(url, {
headers: oauth.toHeader(oauth.authorize({url, method: 'GET'}, token)),
json: true
});
Requests can also be sent via unix domain sockets. Use the following URL scheme: PROTOCOL://unix:SOCKET:PATH
.
PROTOCOL
- http
or https
(optional)SOCKET
- absolute path to a unix domain socket, e.g. /var/run/docker.sock
PATH
- request path, e.g. /v2/keys
got('http://unix:/var/run/docker.sock:/containers/json');
// or without protocol (http by default)
got('unix:/var/run/docker.sock:/containers/json');
It's a good idea to set the 'user-agent'
header so the provider can more easily see how their resource is used. By default, it's the URL to this repo.
var got = require('got');
var pkg = require('./package.json');
got('todomvc.com', {
headers: {
'user-agent': 'my-module/' + pkg.version + ' (https://github.com/username/my-module)'
}
}, function () {});
It is a known issue with old good Node 0.10.x http.Agent
and agent.maxSockets
, which is set to 5
. This can cause low performance and in rare cases deadlocks. To avoid this you can set it manually:
require('http').globalAgent.maxSockets = Infinity;
require('https').globalAgent.maxSockets = Infinity;
This should only ever be done if you have Node version 0.10.x and at the top-level app layer.
Sindre Sorhus | Vsevolod Strukchinsky |
MIT © Sindre Sorhus
FAQs
Human-friendly and powerful HTTP request library for Node.js
The npm package got receives a total of 8,395,162 weekly downloads. As such, got popularity was classified as popular.
We found that got demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.