Comparing version
@@ -6,2 +6,3 @@ import type { Context } from '../../context'; | ||
realm?: string; | ||
hashFunction?: Function; | ||
}, ...users: { | ||
@@ -8,0 +9,0 @@ username: string; |
@@ -40,4 +40,4 @@ "use strict"; | ||
for (const user of users) { | ||
const usernameEqual = await (0, buffer_1.timingSafeEqual)(user.username, requestUser.username); | ||
const passwordEqual = await (0, buffer_1.timingSafeEqual)(user.password, requestUser.password); | ||
const usernameEqual = await (0, buffer_1.timingSafeEqual)(user.username, requestUser.username, options.hashFunction); | ||
const passwordEqual = await (0, buffer_1.timingSafeEqual)(user.password, requestUser.password, options.hashFunction); | ||
if (usernameEqual && passwordEqual) { | ||
@@ -44,0 +44,0 @@ // Authorized OK |
export declare const equal: (a: ArrayBuffer, b: ArrayBuffer) => boolean; | ||
export declare const timingSafeEqual: (a: string | object | boolean, b: string | object | boolean) => Promise<boolean>; | ||
export declare const timingSafeEqual: (a: string | object | boolean, b: string | object | boolean, hashFunction?: Function) => Promise<boolean>; |
@@ -23,7 +23,10 @@ "use strict"; | ||
exports.equal = equal; | ||
const timingSafeEqual = async (a, b) => { | ||
const sa = await (0, crypto_1.sha256)(a); | ||
const sb = await (0, crypto_1.sha256)(b); | ||
const timingSafeEqual = async (a, b, hashFunction) => { | ||
if (!hashFunction) { | ||
hashFunction = crypto_1.sha256; | ||
} | ||
const sa = await hashFunction(a); | ||
const sb = await hashFunction(b); | ||
return sa === sb && a === b; | ||
}; | ||
exports.timingSafeEqual = timingSafeEqual; |
{ | ||
"name": "hono", | ||
"version": "0.5.1", | ||
"version": "0.5.2", | ||
"description": "[炎] Ultrafast web framework for Cloudflare Workers.", | ||
@@ -81,2 +81,3 @@ "main": "dist/index.js", | ||
"@cloudflare/workers-types": "^3.3.0", | ||
"@types/crypto-js": "^4.1.1", | ||
"@types/jest": "^27.4.0", | ||
@@ -87,2 +88,3 @@ "@types/mustache": "^4.1.2", | ||
"@typescript-eslint/parser": "^5.9.0", | ||
"crypto-js": "^4.1.1", | ||
"eslint": "^7.26.0", | ||
@@ -89,0 +91,0 @@ "eslint-config-prettier": "^8.3.0", |
@@ -26,3 +26,3 @@ # Hono | ||
- **Zero-dependencies** - using only Web standard API. | ||
- **Middleware** - builtin middleware and your own middleware. | ||
- **Middleware** - built-in middleware and ability to extend with your own middleware. | ||
- **Optimized** - for Cloudflare Workers. | ||
@@ -32,3 +32,3 @@ | ||
**Hono is fastest** compared to other routers for Cloudflare Workers. | ||
**Hono is fastest**, compared to other routers for Cloudflare Workers. | ||
@@ -46,3 +46,3 @@ ```plain | ||
A demonstration to create an application of Cloudflare Workers with Hono. | ||
A demonstration to create an application for Cloudflare Workers with Hono. | ||
@@ -153,3 +153,3 @@ 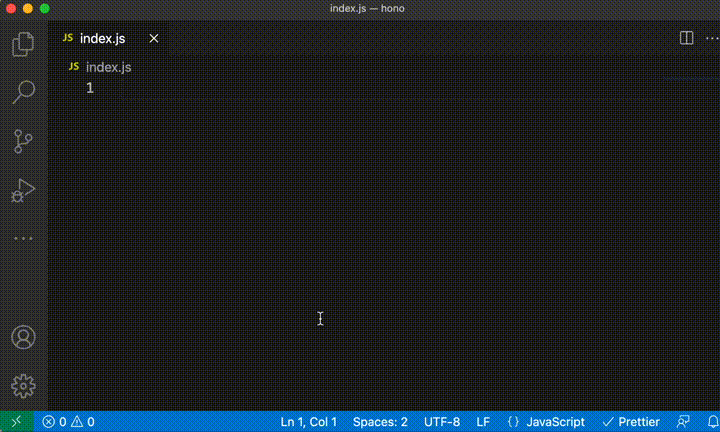 | ||
### Builtin Middleware | ||
### Built-in Middleware | ||
@@ -175,3 +175,3 @@ ```js | ||
Available builtin middleware are listed on [src/middleware](https://github.com/yusukebe/hono/tree/master/src/middleware). | ||
Available built-in middleware is listed on [src/middleware](https://github.com/yusukebe/hono/tree/master/src/middleware). | ||
@@ -281,3 +281,3 @@ ### Custom Middleware | ||
Render texts as `Content-Type:text/plain`. | ||
Render text as `Content-Type:text/plain`. | ||
@@ -390,16 +390,19 @@ ```js | ||
Using `wrangler` or `miniflare`, you can develop the application locally and publish it with few commands. | ||
Using [Wrangler](https://developers.cloudflare.com/workers/cli-wrangler/) or [Miniflare](https://miniflare.dev), you can develop the application locally and publish it with few commands. | ||
Let's write your first code for Cloudflare Workers with Hono. | ||
### 1. Install Wrangler | ||
--- | ||
Install Cloudflare Command Line "[Wrangler](https://github.com/cloudflare/wrangler)". | ||
### Caution | ||
```sh | ||
$ npm i @cloudflare/wrangler -g | ||
``` | ||
**Wrangler 1.x** does not support importing middleware. We recommend two ways: | ||
### 2. `npm init` | ||
1. Use [Wragler 2.0 Beta](https://github.com/cloudflare/wrangler2). | ||
2. Build without webpack 4.x. For example, you can use esbuild. See [the starter template](https://github.com/yusukebe/hono-minimal). | ||
--- | ||
### 1. `npm init` | ||
Make a npm skeleton directory. | ||
@@ -413,12 +416,20 @@ | ||
### 3. `wrangler init` | ||
### 2. `wrangler init` | ||
Init as a wrangler project. | ||
Initialize as a wrangler project. | ||
```sh | ||
$ wrangler init | ||
``` | ||
$ npx wrangler@beta init | ||
``` | ||
### 4. `npm install hono` | ||
Answer the questions. If you want, you can answer `y`. | ||
``` | ||
Would you like to install wrangler into your package.json? (y/n) <--- n | ||
Would you like to use TypeScript? (y/n) <--- n | ||
Would you like to create a Worker at src/index.js? (y/n) <--- n | ||
``` | ||
### 3. `npm install hono` | ||
Install `hono` from the npm registry. | ||
@@ -430,3 +441,3 @@ | ||
### 5. Write your app | ||
### 4. Write your app | ||
@@ -436,2 +447,3 @@ Only 4 lines!! | ||
```js | ||
// index.js | ||
import { Hono } from 'hono' | ||
@@ -445,3 +457,3 @@ const app = new Hono() | ||
### 6. Run | ||
### 5. Run | ||
@@ -451,6 +463,6 @@ Run the development server locally. Then, access `http://127.0.0.1:8787/` in your Web browser. | ||
```sh | ||
$ wrangler dev | ||
$ npx wrangler@beta dev index.js | ||
``` | ||
### 7. Publish | ||
### 6. Publish | ||
@@ -460,3 +472,3 @@ Deploy to Cloudflare. That's all! | ||
```sh | ||
$ wrangler publish | ||
$ npx wrangler@beta publish index.js | ||
``` | ||
@@ -466,3 +478,3 @@ | ||
You can start making your application of Cloudflare Workers with [the starter template](https://github.com/yusukebe/hono-minimal). It is a realy minimal using TypeScript, esbuild, and Miniflare. | ||
You can start making your Cloudflare Workers application with [the starter template](https://github.com/yusukebe/hono-minimal). It is really minimal using TypeScript, esbuild, and Miniflare. | ||
@@ -469,0 +481,0 @@ To generate a project skelton, run this command. |
72632
1.1%1651
0.24%508
2.42%24
9.09%