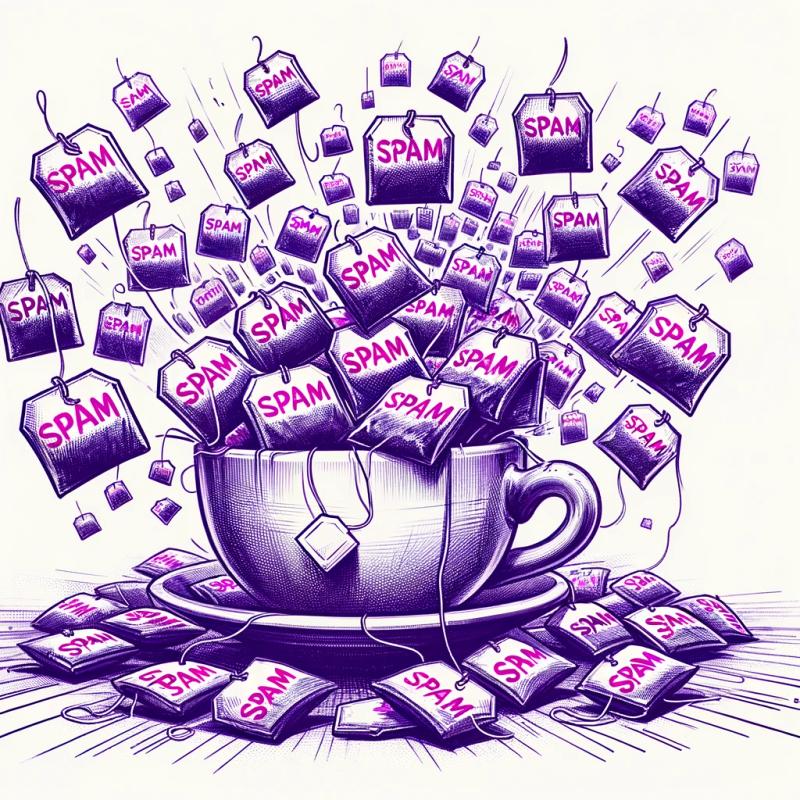
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
iconnectivity-js
Advanced tools
Readme
This library allows you to communicate with iConnectivity devices using JS and the Web MIDI API. It is still in very early development, but the basics already work.
The library's goal is to provide an abstraction layer that allows access to all methods described in the Common System Exclusive Commands document.
If you want to check out a demo project, head over to the demo package.
The easiest way to get started is using the DeviceManager
. Here's an example:
import {
DeviceManager,
getAllInfo,
getAutomaticFailoverState,
} from "iconnectivity-js";
const example = async () => {
// Request access to MIDI devices.
// It's important to request SysEx access as well.
const midiAccess = await navigator.requestMIDIAccess({ sysex: true });
// Initialize the device manager.
// This will automatically trigger a search for devices.
const manager = new DeviceManager(midiAccess);
// Wait for the first device(s) to be found.
const devices = await manager.waitForDevices();
// At this point, we can be sure that at least one device was found.
const device = devices[0];
// Log some information about the device and its state.
console.log({
input: device.input.name,
output: device.output.name,
info: device.info,
extendedInfo: await getAllInfo({ device }),
});
// If the device is a PlayAUDIO12,
// we can get the current failover state.
const failoverState = await getAutomaticFailoverState({ device });
console.log({ failoverState });
};
example();
You can use wrapper functions for supported commands, with more coming in the future. A list of supported commands can be found below. For example, to fetch the current failover state:
import { getAutomaticFailoverState } from "iconnectivity-js";
// The device passed to this function could be
// one of the devices found by the DeviceManager.
const state = await getAutomaticFailoverState({ device });
The device
property doesn't necessarily need to be a device that the
DeviceManager
outputs. You could also set it to a custom Connection
object:
import { getAutomaticFailoverState } from "iconnectivity-js";
const access = await navigator.requestMIDIAccess({ sysex: true });
// Get the first available MIDI input and output.
const input = access.inputs.values()[0];
const output = access.outputs.values()[0];
// We now send the request to the first MIDI output
// and listen to a response on the first MIDI input.
const state = await getAutomaticFailoverState({
device: new Connection(input, output),
});
Even if a given command isn't wrapped by this library yet, you can still call it
and parse the response yourself by using the sendCommand
function. This
function allows you to For example:
import { CommandOptions, mergeNumber, sendCommand } from "iconnectivity-js";
/** Returns the number of MIDI ports the given device offers. */
const getMidiPortCount = async (options: CommandOptions) => {
const result = await sendCommand({
...options,
command: MidiCommand.GetMIDIInfo,
});
// The number of ports is stored in the
// 20th and 21st byte of the response.
return mergeNumber(result.slice(19, 21));
};
console.log("Port count:", await getMidiPortCount({ device }));
This list contains all commands currently documented by iConnectivity. The ones that already have a wrapper function are checked. If you want to contribute to this list by writing wrapper functions for more commands, feel free to open a PR.
getAllInfo
getActiveScene
setActiveScene
getAutomaticFailoverState
setAutomaticFailoverState
FAQs
[View the Live Demo](https://icjs.leolabs.org) | [NPM](https://npmjs.com/package/iconnectivity-js)
The npm package iconnectivity-js receives a total of 0 weekly downloads. As such, iconnectivity-js popularity was classified as not popular.
We found that iconnectivity-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.