Comparing version 2.0.0-10 to 2.0.0-11
@@ -73,2 +73,6 @@ "use strict"; | ||
}, | ||
insertInContainerBefore: (parentNode, childNode, beforeNode) => { | ||
(0, _dom.insertBeforeNode)(parentNode, childNode, beforeNode); | ||
onRender(); | ||
}, | ||
removeChildFromContainer: (parentNode, childNode) => { | ||
@@ -75,0 +79,0 @@ (0, _dom.removeChildNode)(parentNode, childNode); |
@@ -16,4 +16,2 @@ "use strict"; | ||
var _dom = require("./dom"); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
@@ -34,4 +32,3 @@ | ||
config, | ||
terminalWidth, | ||
skipStaticElements | ||
terminalWidth | ||
} = options; | ||
@@ -48,7 +45,3 @@ | ||
if (node.childNodes.length > 0) { | ||
const childNodes = node.childNodes.filter(childNode => { | ||
return skipStaticElements ? !childNode.static : true; | ||
}); | ||
for (const [index, childNode] of Object.entries(childNodes)) { | ||
for (const [index, childNode] of Object.entries(node.childNodes)) { | ||
const childYogaNode = buildLayout(childNode, options).yogaNode; | ||
@@ -96,7 +89,3 @@ yogaNode.insertChild(childYogaNode, index); | ||
if (node.childNodes.length > 0) { | ||
const childNodes = node.childNodes.filter(childNode => { | ||
return skipStaticElements ? !childNode.static : true; | ||
}); | ||
for (const [index, childNode] of Object.entries(childNodes)) { | ||
for (const [index, childNode] of Object.entries(node.childNodes)) { | ||
const { | ||
@@ -114,9 +103,4 @@ yogaNode: childYogaNode | ||
const renderNodeToOutput = (node, output, offsetX = 0, offsetY = 0, { | ||
transformers, | ||
skipStaticElements | ||
transformers | ||
}) => { | ||
if (node.static && skipStaticElements) { | ||
return; | ||
} | ||
const { | ||
@@ -149,23 +133,5 @@ yogaNode | ||
renderNodeToOutput(childNode, output, x, y, { | ||
transformers: newTransformers, | ||
skipStaticElements | ||
transformers: newTransformers | ||
}); | ||
} | ||
}; // Since <Static> components can be placed anywhere in the tree, this helper finds and returns them | ||
const getStaticNodes = element => { | ||
const staticNodes = []; | ||
for (const childNode of element.childNodes) { | ||
if (childNode.static) { | ||
staticNodes.push(childNode); | ||
} | ||
if (Array.isArray(childNode.childNodes) && childNode.childNodes.length > 0) { | ||
staticNodes.push(...getStaticNodes(childNode)); | ||
} | ||
} | ||
return staticNodes; | ||
}; // Build layout, apply styles, build text output of all nodes and return it | ||
@@ -181,3 +147,2 @@ | ||
let lastYogaNode; | ||
let lastStaticYogaNode; | ||
return node => { | ||
@@ -188,40 +153,2 @@ if (lastYogaNode) { | ||
if (lastStaticYogaNode) { | ||
lastStaticYogaNode.freeRecursive(); | ||
} | ||
const staticElements = getStaticNodes(node); | ||
if (staticElements.length > 1) { | ||
if (process.env.NODE_ENV !== 'production') { | ||
console.error('Warning: There can only be one <Static> component'); | ||
} | ||
} // <Static> component must be built and rendered separately, so that the layout of the other output is unaffected | ||
let staticOutput; | ||
if (staticElements.length === 1) { | ||
const rootNode = (0, _dom.createNode)('root'); | ||
(0, _dom.appendChildNode)(rootNode, staticElements[0]); | ||
const { | ||
yogaNode: staticYogaNode | ||
} = buildLayout(rootNode, { | ||
config, | ||
terminalWidth, | ||
skipStaticElements: false | ||
}); | ||
staticYogaNode.calculateLayout(_yogaLayoutPrebuilt.default.UNDEFINED, _yogaLayoutPrebuilt.default.UNDEFINED, _yogaLayoutPrebuilt.default.DIRECTION_LTR); // Save current Yoga node tree to free up memory later | ||
lastStaticYogaNode = staticYogaNode; | ||
staticOutput = new _output.default({ | ||
width: staticYogaNode.getComputedWidth(), | ||
height: staticYogaNode.getComputedHeight() | ||
}); | ||
renderNodeToOutput(rootNode, staticOutput, 0, 0, { | ||
transformers: [], | ||
skipStaticElements: false | ||
}); | ||
} | ||
const { | ||
@@ -231,4 +158,3 @@ yogaNode | ||
config, | ||
terminalWidth, | ||
skipStaticElements: true | ||
terminalWidth | ||
}); | ||
@@ -243,9 +169,5 @@ yogaNode.calculateLayout(_yogaLayoutPrebuilt.default.UNDEFINED, _yogaLayoutPrebuilt.default.UNDEFINED, _yogaLayoutPrebuilt.default.DIRECTION_LTR); // Save current node tree to free up memory later | ||
renderNodeToOutput(node, output, 0, 0, { | ||
transformers: [], | ||
skipStaticElements: true | ||
transformers: [] | ||
}); | ||
return { | ||
output: output.get(), | ||
staticOutput: staticOutput ? `${staticOutput.get()}\n` : undefined | ||
}; | ||
return output.get(); | ||
}; | ||
@@ -252,0 +174,0 @@ }; |
@@ -48,8 +48,2 @@ "use strict"; | ||
}); | ||
Object.defineProperty(exports, "Static", { | ||
enumerable: true, | ||
get: function () { | ||
return _Static.default; | ||
} | ||
}); | ||
@@ -70,4 +64,2 @@ var _render = _interopRequireDefault(require("./render")); | ||
var _Static = _interopRequireDefault(require("./components/Static")); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } |
@@ -10,6 +10,6 @@ "use strict"; | ||
var _logUpdate = _interopRequireDefault(require("log-update")); | ||
var _lodash = _interopRequireDefault(require("lodash.throttle")); | ||
var _logUpdate = _interopRequireDefault(require("./vendor/log-update")); | ||
var _createReconciler = _interopRequireDefault(require("./create-reconciler")); | ||
@@ -19,4 +19,2 @@ | ||
var _diffString = _interopRequireDefault(require("./diff-string")); | ||
var _dom = require("./dom"); | ||
@@ -57,3 +55,2 @@ | ||
let lastOutput = ''; | ||
let lastStaticOutput = ''; | ||
@@ -65,20 +62,7 @@ const onRender = () => { | ||
const { | ||
output, | ||
staticOutput | ||
} = render(rootNode); | ||
const output = render(rootNode); | ||
if (options.debug) { | ||
options.stdout.write((staticOutput || '') + output); | ||
options.stdout.write(output); | ||
return; | ||
} // If <Static> part of the output has changed, calculate the difference | ||
// between the last <Static> output and log it to stdout. | ||
// To ensure static output is cleanly rendered before main output, clear main output first | ||
if (staticOutput && staticOutput !== lastStaticOutput) { | ||
log.clear(); | ||
options.stdout.write((0, _diffString.default)(lastStaticOutput, staticOutput)); | ||
log(output); | ||
lastStaticOutput = staticOutput; | ||
} | ||
@@ -85,0 +69,0 @@ |
{ | ||
"name": "ink", | ||
"version": "2.0.0-10", | ||
"version": "2.0.0-11", | ||
"description": "React for CLI", | ||
@@ -41,2 +41,3 @@ "license": "MIT", | ||
"dependencies": { | ||
"ansi-escapes": "^3.1.0", | ||
"arrify": "^1.0.1", | ||
@@ -46,3 +47,2 @@ "chalk": "^2.4.1", | ||
"lodash.throttle": "^4.1.1", | ||
"log-update": "^2.3.0", | ||
"prop-types": "^15.6.2", | ||
@@ -63,4 +63,8 @@ "react-reconciler": "^0.17.0", | ||
"babel-eslint": "^10.0.1", | ||
"delay": "^4.1.0", | ||
"eslint-config-xo-react": "^0.17.0", | ||
"eslint-plugin-react": "^7.11.1", | ||
"import-jsx": "^1.3.0", | ||
"ms": "^2.1.1", | ||
"p-queue": "^3.0.0", | ||
"react": "^16.6.1", | ||
@@ -67,0 +71,0 @@ "strip-ansi": "^4.0.0", |
@@ -540,29 +540,3 @@ <h1 align="center"> | ||
#### <Static> | ||
`<Static>` component allows permanently rendering output to stdout and preserving it across renders. | ||
Components passed to `<Static>` as children will be written to stdout only once and will never be rerendered. | ||
`<Static>` output comes first, before any other output from your components, no matter where it is in the tree. | ||
In order for this mechanism to work properly, at most one `<Static>` component must be present in your node tree and components that were rendered must never update their output. Ink will detect new children appended to `<Static>` and render them to stdout. | ||
Example use case for this component is Jest's output: | ||
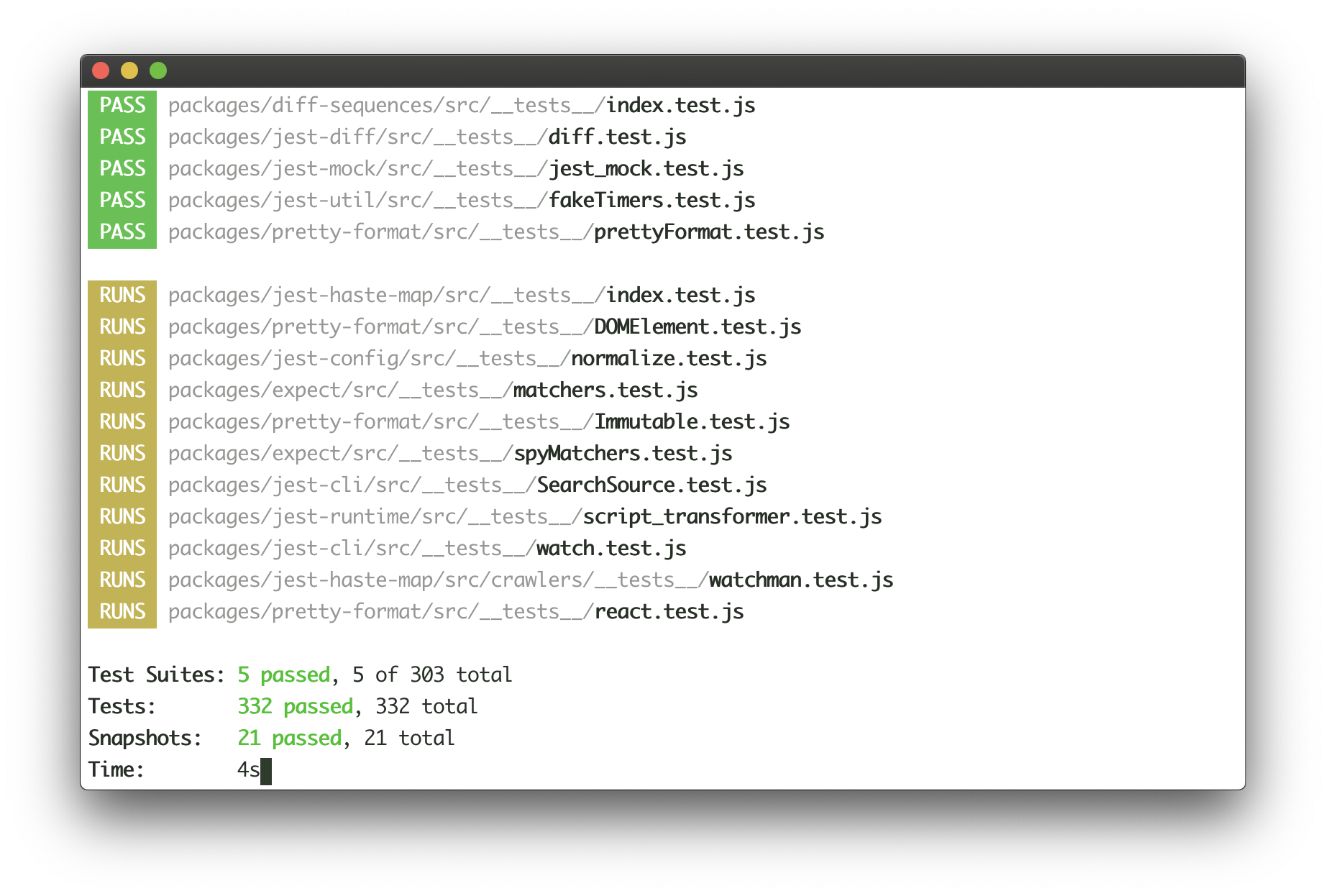 | ||
Jest continuosuly writes the list of completed tests to the output, while updating test results at the bottom of the output in real-time. Here's how this user interface could be implemented with Ink: | ||
```jsx | ||
<Fragment> | ||
<Static> | ||
{tests.map(test => ( | ||
<Test key={test.id} title={test.title}/> | ||
))} | ||
</Static> | ||
<Box marginTop={1}> | ||
<TestResults passed={results.passed} failed={results.failed}/> | ||
</Box> | ||
</Fragment> | ||
``` | ||
#### <StdinContext> | ||
@@ -569,0 +543,0 @@ |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Environment variable access
Supply chain riskPackage accesses environment variables, which may be a sign of credential stuffing or data theft.
Found 1 instance in 1 package
23
1
54973
17
976
614
+ Addedansi-escapes@^3.1.0
- Removedlog-update@^2.3.0
- Removedlog-update@2.3.0(transitive)
- Removedwrap-ansi@3.0.1(transitive)