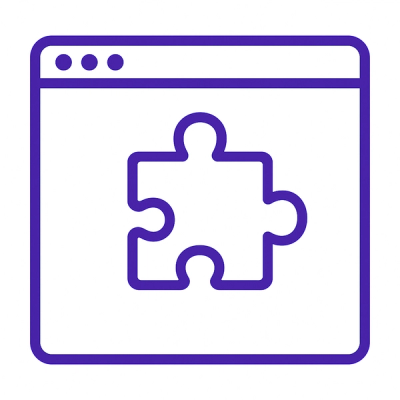
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
instagram-web-api
Advanced tools
Simple, easy and very complete implementation of the Instagram private web API.
npm install instagram-web-api
Intance Instagram
and call login
method; this stores the credentials in memory.
const Instagram = require('instagram-web-api')
const { username, password } = process.env
const client = new Instagram({ username, password })
client
.login()
.then(() => {
client
.getProfile()
.then(console.log)
})
Using async
/await
in Node >= 8
const Instagram = require('instagram-web-api')
const { username, password } = process.env
const client = new Instagram({ username, password })
;(async () => {
await client.login()
const profile = await client.getProfile()
console.log(profile)
})()
Save cookies to disk by using a though-cookie
store.
// Packages
const Instagram = require('instagram-web-api')
const FileCookieStore = require('tough-cookie-filestore2')
const { username, password } = process.env // Only required when no cookies are stored yet
const cookieStore = new FileCookieStore('./cookies.json')
const client = new Instagram({ username, password, cookieStore })
;(async () => {
// URL or path of photo
const photo =
'https://scontent-scl1-1.cdninstagram.com/t51.2885-15/e35/22430378_307692683052790_5667315385519570944_n.jpg'
await client.login()
// Upload Photo to feed or story, just configure 'post' to 'feed' or 'story'
const { media } = await client.uploadPhoto({ photo: photo, caption: 'testing', post: 'feed' })
console.log(`https://www.instagram.com/p/${media.code}/`)
})()
const client = new Instagram({ username: '', password: '' }, { language: 'es-CL' })
Initializes the client.
credentials
username
: The username of accountpassword
: The password of accountcookieStore
: An optional though-cookie
cookie storage, which allows for persistent cookies. Default is undefined
opts
language
: The language of response from API. Default is en-US
proxy
: String
of a proxy to tunnel all requests. Default is undefined
const { username, password, cookies } = await client.login({ username: '', password: '' })
const { authenticated, user } = await client.login({ username: '', password: '' })
Login in the account, this method returns
user
(true
when username is valid) andauthenticated
(true
when login was successful)
credentials
username
: The username of accountpassword
: The password of accountawait client.logout()
Logout in the account.
const feed = await client.getHome('KGEAxpEdUwUrxxoJvxRoQeXFGooSlADHZ8UaDdSWbnOIxxoUUhyciJ7EGlxNlZjaYcUaXTgUM00qyBrgBhUsLezIGqVTlxqausga5W-fVax9xRryaBdN1EnIGvdQFgzxoMgaFoLO7v7xWQA=')
Get home feed timeline, media shared by the people you follow.
params
end_cursor
(String
) for paginationconst instagram = await client.getUserByUsername({ username: 'instagram' })
const me = await client.getUserByUsername({ username: client.credentials.username })
Get user by username, this method not require authentication for public profiles.
params
username
: The username of the profileconst followers = await client.getFollowers({ userId: '1284161654' })
Get followers for given userId. Be aware that the response gets slightly altered for easier usage.
params
userId
: The user idfirst
: Amount of followers to request. Default is 20
after
: Optional end_cursor
(String
) for pagination.const followings = await client.getFollowings({ userId: '1284161654' })
Get followings for given userId. Be aware that the response gets slightly altered for easier usage.
params
userId
: The user idfirst
: Amount of followings to request. Default is 20
after
: Optional end_cursor
(String
) for pagination.const activity = await client.getActivity()
Get activity of account, news following, liked, etc.
const profile = await client.getProfile()
Get profile the account
first_name
,last_name
,username
,phone_number
,gender
,birthday
,biography
,external_url
andchaining_enabled
.
await client.updateProfile({ biography: '❤️', website: 'https://jlobos.com/', gender: 1 })
Update profile the account.
params
name
: The full name. Default is
email
: The email of account. Default is
username
: The username of account. Default is client.credentials.username
phoneNumber
: The Phone Number. Default is
gender
: Number
1
male, 2
female and 3
not specifiedbiography
: The Bio. Default is
website
: The Website. Default is
similarAccountSuggestions
: Boolean
Include your account when recommending similar accounts people might want to follow. Default is true
const fs = require('fs')
const photo = fs.join(__dirname, 'photo.jpg')
await client.changeProfilePhoto({ photo })
Change the profile photo.
params
photo
: A String
of path file or URLawait client.deleteMedia({ mediaId: '1442533050805297981' })
Delete a media, photo, video, etc. by the id.
params
mediaId
: The media idconst photo = 'https://scontent-scl1-1.cdninstagram.com/t51.2885-15/e35/16465198_658888867648924_4042368904838774784_n.jpg'
await client.uploadPhoto({ photo, caption: '❤️', post: 'feed' })
Upload a photo to Instagram. Only jpeg images allowed.
params
photo
: A String
of path file or URLcaption
: The caption of photo. Default is
post
: The local post, feed
or story
const location = await client.getMediaFeedByLocation({ locationId: '26914683' })
Get latitude, longitude, top posts, last media, country, city, and more related to the location.
params
locationId
: The location idconst tag = client.getMediaFeedByHashtag({ hashtag: 'unicorn' })
Explore last media and top posts feed related to a hashtag.
params
hashtag
: A hashtag, not including the "#"const venues = client.locationSearch({ query: 'chile', latitude: -33.45, longitude: -70.6667 })
Search venues by latitude and longitude.
params
latitude
: Latitudelongitude
: Longitudequery
: A optional location name. Default is
const media = await client.getMediaByShortcode({ shortcode: 'BQE6Cq2AqM9' })
Get data of a media by the Instagram shortcode
params
shortcode
: A shortcodeawait client.addComment({ mediaId: 1442533050805297981, text: 'awesome' })
Add comment to a media item.
params
mediaId
: The media idtext
: Comment textreplyToCommentId
: Optional comment id to which to replyawait client.deleteComment({ mediaId: '1442533050805297981', commentId: '17848908229146688' })
Delete a comment.
params
mediaId
: The media idcommentId
: The comment idawait client.getChallenge({ challengeUrl: '/challenge/1284161654/a1B2c3d4E6/' })
Get information about a challenge.
params
challengeUrl
: A String
with a challenge pathconst challengeUrl = '/challenge/1284161654/a1B2c3d4E6/'
await client.updateChallenge({ challengeUrl, choice: 0 })
await client.updateChallenge({ challengeUrl, securityCode: 123456 })
Request or submit a verification code for the given challenge.
params
challengeUrl
: A String
with a challenge pathchoice
: Number
0
for phone and 1
for email. Default is ``securityCode
: Number
the received verification code for the challenge. Default is ``await client.resetChallenge({ challengeUrl: '/challenge/1284161654/a1B2c3d4E6/' })
Reset a challenge to start over again.
params
challengeUrl
: A String
with a challenge pathawait client.replayChallenge({ challengeUrl: '/challenge/1284161654/a1B2c3d4E6/' })
Request a new verification message.
params
challengeUrl
: A String
with a challenge pathawait client.approve({ userId: '1284161654' })
Approve a friendship request.
params
userId
: The user idawait client.ignore({ userId: '1284161654' })
Reject a friendship request.
params
userId
: The user idawait client.follow({ userId: '1284161654' })
Follow a user.
params
userId
: The user idawait client.unfollow({ userId: '1284161654' })
Unfollow a user.
params
userId
: The user idawait client.block({ userId: '1284161654' })
Block a user.
params
userId
: The user idawait client.unblock({ userId: '1284161654' })
Unblock a user.
params
userId
: The user idawait client.like({ mediaId: '1442533050805297981' })
Like a media item.
params
mediaId
: The media idawait client.unlike({ mediaId: '1442533050805297981' })
Unlike a media item.
params
mediaId
: The media idawait client.save({ mediaId: '1442533050805297981' })
Save a media item.
params
mediaId
: The media idawait client.unsave({ mediaId: '1442533050805297981' })
Unsave a media item.
params
mediaId
: The media idawait client.search({ query: 'unicorn' })
Search users, places, or hashtags.
params
query
: Query
context
: The context of search, hashtag
, place
, user
or blended
. Default is blended
await client.getPhotosByHashtag({ hashtag: 'unicorn' })
Get photos for hashtag.
params
hashtag
: A String
with a hashtagfirst
: A number
of records to returnafter
: The query cursor String
for paginationawait client.getPhotosByUsername({ username: 'unicorn' })
Gets user photos.
params
username
: A String
with a hashtagfirst
: A number
of records to returnafter
: The query cursor String
for paginationawait client.getPrivateProfilesFollowRequests(cursor)
await client.getChainsData({ userId })
This will return the similar accounts, that you see, when you click on the ARROW in a profile.
params
userId
: The user idawait client.getMediaLikes({ shortcode: 'B-0000000', first: '49', after: '' })
This will return the media likes.
params
shortcode
: The shortcode media like this: https://www.instagram.com/p/B-00000000/, only put shortcode like this : B-000000000first
: A number
of records to return max is 49
after
: The query cursor String
for paginationawait client.getMediaComments({ shortcode: 'B-0000000', first: '12', after: '' }).catch((error) => {
console.log(error);
})
.then((response) => {
console.log(response);
});
//The query cursor 'after' maybe return an array, if array you need to convert like this:
let pointer = response.page_info.end_cursor;
// this will try to convert array to json stringify
try{
pointer = JSON.parse(pointer);
pointer = JSON.stringify(pointer);
}catch(e){
console.log('Pointer is not array!, don't need to be converted!');
}
This will return the media comments.
params
shortcode
: The shortcode media like this: https://www.instagram.com/p/B-00000000/, only put shortcode like this : B-000000000first
: A number
of records to return max is 49
after
: The query cursor String
for paginationMIT © Jesús Lobos
FAQs
Instagram Private Web API client written in JS
The npm package instagram-web-api receives a total of 734 weekly downloads. As such, instagram-web-api popularity was classified as not popular.
We found that instagram-web-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.