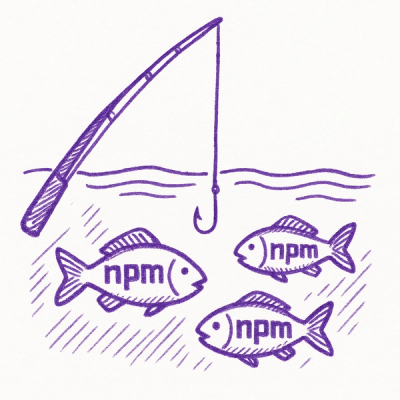
Security News
/Research
npm Phishing Email Targets Developers with Typosquatted Domain
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.
intento-nodejs
Advanced tools
An adapter to query Intento API.
Intento provides a single API to Cognitive AI services from many vendors.
To get more information check out the site.
In case you don't have a key to use Intento API, please register here inten.to
npm install intento-nodejs
or
yarn add intento-nodejs
See CLI example as one of the ways to use this SDK.
Requires serve
to be installed globally in your system
yarn global add serve
yarn build # it will prepare minified files, see ./dist folder
yarn test-in-browser
# Visit http://localhost:5000/samples/browser-app
Go to an example browser app at http://localhost:5000/samples/browser-app
Initialise the client
const IntentoConnector = require('intento-nodejs')
const client = new IntentoConnector({ apikey: YOUR_INTENTO_KEY })
This is an intent to translate text from one language to another.
Related documentation
More examples in the sample app
Simple translate text text
to language to
:
client.ai.text.translate
.fulfill({ text: "How's it going?", to: 'es' })
.then(data => {
console.log('Translation results:\n', data, '\n\n')
})
This is an intent to analyze the sentiment of the provided text.
Related documentation
More examples in the sample app
client.ai.text.sentiment
.fulfill({
text: 'We love this place',
lang: 'en',
provider: 'ai.text.sentiment.ibm.natural_language_understanding',
})
.then(data => {
console.log('Sentiment analysis results:\n', data, '\n\n')
})
This is an intent to get meanings of text in selected language.
Related documentation
More examples in the sample app
client.ai.text.dictionary
.fulfill({
text: 'meaning',
from: 'en',
to: 'ru',
})
.then(data => {
console.log('Dictionary results:\n', JSON.stringify(data, null, 4), '\n')
})
In all cases a response object is a list of objects. Each object in that list describes one provider. The structure of the description is following:
{
id: 'provider.id',
name: 'Provider Name',
score: 0,
price: 0,
symmetric: [...],
pairs: [...],
}
symmetric
- is a list of language codes for which translation in both directions is available.
pairs
- is a list of plain objects with structure { from: 'lang-code-1', to: 'lang-code-2' }
. It means that for current provider translation from lang-code-1
to lang-code-2
is available.
client.ai.text.translate
.providers()
.then(data => data.forEach(p => console.info(p.name)))
client.ai.text.sentiment
.providers()
.then(data => data.forEach(p => console.info(p.name)))
client.ai.text.dictionary
.providers()
.then(data => data.forEach(p => console.info(p.name)))
More information in ai.text.translate.md
More information in ai.text.sentiment.md
More information in ai.text.dictionary.md
Intento provides the smart routing feature, so that the translation request is automatically routed to the best provider. The best provider is determined based on the following information:
To use the smart routing, just omit the provider
parameter:
client.ai.text.translate
.fulfill({
text: "How's it going?",
to: 'es'
})
.then(console.log)
Response:
{
"id": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"done": true,
"response": [
{
"results": [
"¿Qué tal va todo?"
],
"meta": {
"detected_source_language": [
"en"
]
},
"service": {
"provider": {
"id": "ai.text.translate.deepl.api",
"name": "DeepL API",
"vendor": "DeepL",
"description": "API",
"logo": "https://inten.to/static/img/api/deepl_translate.png"
}
}
}
],
"meta": {
// information about initial request
},
"error": null
}
By default, when the provider is missing, requests are routed to a provider with the best expected price/performance ratio. This behavior may be controlled by specifying the desired routing strategy in the routing
parameter. To set up routing for your account contact us at hello@inten.to.
client.ai.text.translate
.fulfill({
text: 'A sample text',
to: 'es',
routing: 'best-quality',
})
.then(console.log)
Both for smart routing mode and basic mode, a failover is supported. By default, the failover is off, thus when the selected provider fails, an error is returned. To enable the failover mode, set the failover
to true
. By default, failover is governed by the default routing strategy (best
). To control this behavior, another routing strategy may be specified via routing
parameter. Alternatively, you may specify a list of providers to consider for the failover (failover_list
). This option overrides the routing strategy for the failover procedure.
In the following example we set the provider, but specify the list of alternatives to control the failover:
client.ai.text.translate
.fulfill({
text: 'A sample text',
to: 'es',
provider: 'ai.text.translate.microsoft.translator_text_api.2-0',
failover: true,
failover_list: [
'ai.text.translate.google.translate_api.2-0',
'ai.text.translate.yandex.translate_api.1-5'
]
})
.then(console.log)
Intento supports two modes of using 3rd party services:
In the tech proxy mode, the custom credentials are passed in the auth
service field. auth
field is a dictionary, it's keys are provider IDs. For each ID specify your own key(s) you want to use and values set to a list of keys for the specified provider. There could be more than one key for the provider in the case you want to work with a pool of keys (more on this advanced feature later).
client.ai.text.translate
.fulfill({
text: "A sample text",
to: 'es',
provider: 'some-provider-id',
auth: {
'some-provider-id': [
{ ... custom auth structure with 'YOUR_KEY_TO_SOME_PROVIDER_1' ... },
{ ... custom auth structure with 'YOUR_KEY_TO_SOME_PROVIDER_2' ... },
],
'another-provider-id': [
{ ... another custom auth structure with 'YOUR_KEY_TO_ANOTHER_PROVIDER_1' ... },
{ ... another custom auth structure with 'YOUR_KEY_TO_ANOTHER_PROVIDER_2' ... },
]
})
.then(console.log)
Auth object structure is different for different providers and may be obtained together with other provider details by requesting info for this provider:
client.ai.text.translate
.provider('ai.text.translate.google.translate_api.2-0')
.then(console.log)
For example for google translate custom auth structure is { key: YOUR_GOOGLE_KEY }
.
client.ai.text.translate
.fulfill({
text: "A sample text",
to: 'es',
provider: 'ai.text.translate.google.translate_api.2-0',
auth: {
'ai.text.translate.google.translate_api.2-0': [
{
key: process.env.YOUR_GOOGLE_KEY
}
]
}
})
.then(console.log)
Response:
{
"id": "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
"done": true,
"response": [
{
"results": [
"Un texto de muestra"
],
"meta": {
"detected_source_language": [
"en"
]
},
"service": {
"provider": {
"id": "ai.text.translate.google.translate_api.2-0",
"name": "Google Cloud Basic Translation API",
"vendor": "Google Cloud",
"description": "Basic Translation API",
"logo": "https://inten.to/static/img/api/ggl_translate.png"
}
}
}
],
"meta": {
// information about initial request
},
"error": null
}
Describe data
dynamicly through content
For example we'd like to translate the same text for different languages. One can make similar requests for each language like this:
const IntentoConnector = require('intento-nodejs')
const client = new IntentoConnector({ apikey: process.env.INTENTO_API_KEY })
const options = {
path: '/ai/text/translate',
method: 'POST',
// content: ...
}
['es', 'ru', 'da'].forEach(lang => {
client
.makeRequest({
...options, // path, method
content: {
context: {
text: 'A sample text',
to: lang,
},
},
})
.then(console.info)
})
So you can pass a plain javascript object as a content
parameter.
data
argument from a curl request directlyOne can pass request parameters as raw json specified as a data
parameter.
Make sure your json is valid. For example one can validate json online
For a curl
instruction from the docs
curl -XPOST -H 'apikey: YOUR_API_KEY' 'https://api.inten.to/ai/text/translate' -d '{
"context": {
"text": "Validation is the king",
"to": "es"
},
"service": {
"provider": "ai.text.translate.microsoft.translator_text_api.2-0"
}
}'
run following:
const IntentoConnector = require('intento-nodejs')
const client = new IntentoConnector({ apikey: process.env.INTENTO_API_KEY })
client
.makeRequest({
path: '/ai/text/translate',
method: 'POST',
data: `{
"context": {
"text": "Validation is the king",
"to": "es"
},
"service": {
"provider": "ai.text.translate.microsoft.translator_text_api.2-0"
}
}`,
})
.then(console.info)
To get more examples:
git clone git@github.com:intento/intento-nodejs.git
cd intento-nodejs
INTENTO_API_KEY=YOUR_SECRET_KEY node example.js
Though for the latter you need to have INTENTO_API_KEY
in your environment.
Hardcode your keys in the script your are experimenting with :)
For Unix-like machines run:
INTENTO_API_KEY=YOUR_SECRET_KEY GOOGLE_API_KEY=YOUR_GOOGLE_API_KEY node example.js
For Windows machines run:
SET INTENTO_API_KEY=YOUR_SECRET_KEY GOOGLE_API_KEY=YOUR_GOOGLE_API_KEY node example.js
Assuming your are in intento-nodejs
folder run:
cp .env.example .env
Then edit .env
, put your api keys there.
Make that environmental variables available in the current context
export $(cat .env) # once per terminal
Run example.js
script
node example.js
Use your favorite "env" package like dotenv
or node-env-file
.
FAQs
Intento API Client Library for Node.js
The npm package intento-nodejs receives a total of 37 weekly downloads. As such, intento-nodejs popularity was classified as not popular.
We found that intento-nodejs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.
Security News
Knip hits 500 releases with v5.62.0, refining TypeScript config detection and updating plugins as monthly npm downloads approach 12M.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.