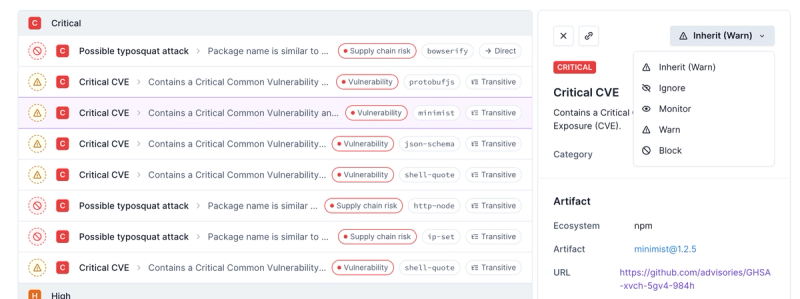
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
itty-router
Advanced tools
Readme
It's an itty bitty router. Like... super tiny, with zero dependencies. For reals.
Did we mention it supports route/query params like in Express.js?
yarn add itty-router
or if you've been transported back to 2017...
npm install itty-router
/api/:foo/:id?
)?page=3
){ params: { foo: 'bar' }, query: { page: '3' }}
import { Router } from 'itty-router'
// create a Router
const router = Router()
// basic GET route (with optional ? flag)... delivers route params and query params as objects to handler
router.get('/todos/:id?', ({ params, query }) =>
console.log('will match both /todos and /todos/13', `id=${params.id}`, `limit=${query.limit}`)
)
// works with POST, DELETE, PATCH, etc
router.post('/todos', () => console.log('posted a todo'))
// ...or any other method we haven't yet thought of (thanks to @mvasigh implementation of Proxy <3)
router.future('/todos', () => console.log(`this caught using the FUTURE method!`))
// then handle a request!
router.handle({ method: 'GET', url: 'https://foo.com/todos/13?foo=bar' })
// ...and viola! the following payload/context is passed to the matching route handler:
// {
// params: { id: '13' },
// query: { foo: 'bar' },
// ...whatever else was in the original request object/class (e.g. method, url, etc)
// }
import { Router } from 'itty-router'
const router = Router() // no "new", as this is not a real ES6 class/constructor!
.{methodName}(route:string, handler1:function, handler2:function, ...)
The "instantiated" router translates any attribute (e.g. .get
, .post
, .patch
, .whatever
) as a function that binds a "route" (string) to route handlers (functions) on that method type (e.g. router.get --> GET
, router.post --> POST
). When the url fed to .handle({ url })
matches the route and method, the handlers are fired sequentially. Each is given the original request/context, with any parsed route/query params injected as well. The first handler that returns (anything) will end the chain, allowing early exists from errors, inauthenticated requests, etc. This mechanism allows ANY method to be handled, including completely custom methods (we're very curious how creative individuals will abuse this flexibility!). The only "method" currently off-limits is handle
, as that's used for route handling (see below).
// register a route on the "GET" method
router.get('/todos/:user/:item?', (req) => {
let { params, query, url } = req
let { user, item } = params
console.log('GET TODOS from', url, { user, item })
})
.handle(request = { method:string = 'GET', url:string })
The only requirement for the .handle(request)
method is an object with a valid full url (e.g. https://example.com/foo
). The method
property is optional and defaults to GET
(which maps to routes registered with router.get()
). This method will return the first route handler that actually returns something. For async/middleware examples, please see below.
router.handle({
method: 'GET', // optional, default = 'GET'
url: 'https://example.com/todos/jane/13', // required
})
// matched handler from step #2 (above) will execute, with the following output:
// GET TODOS from https://example.com/todos/jane/13 { user: 'jane', item: '13' }
import { Router } from 'itty-router'
// create a router
const router = Router() // note the intentional lack of "new"
// register some routes
router.get('/foo', () => new Response('Foo Index!'))
router.get('/foo/:id', ({ params }) => new Response(`Details for item ${params.id}.`))
router.get('*', () => new Response('Not Found.', { status: 404 })
// attach the router handle to the event handler
addEventListener('fetch', event => event.respondWith(router.handle(event.request)))
// withUser modifies original request, then continues without returning
const withUser = (req) => {
req.user = { name: 'Mittens', age: 3 }
}
// requireUser optionally returns (early) if user not found on request
const requireUser = (req) => {
if (!req.user) return new Response('Not Authenticated', { status: 401 })
}
// showUser returns a response with the user, as it is assumed to exist at this point
const showUser = (req) => new Response(JSON.stringify(req.user))
router.get('/pass/user', withUser, requireUser, showUser) // withUser injects user, allowing requireUser to not return/continue
router.get('/fail/user', requireUser, showUser) // requireUser returns early because req.user doesn't exist
router.handle({ url: 'https://example.com/pass/user' }) // --> STATUS 200: { name: 'Mittens', age: 3 }
router.handle({ url: 'https://example.com/fail/user' }) // --> STATUS 401: Not Authenticated
// withUser modifies original request, then continues without returning
const withUser = (req) => {
req.user = { name: 'Mittens', age: 3 }
}
router.get('*', withUser) // embeds user before all other matching routes
router.get('/user', (req) => new Response(JSON.stringify(req.user))) // user embedded already!
router.handle({ url: 'https://example.com/user' }) // --> STATUS 200: { name: 'Mittens', age: 3 }
const parentRouter = Router()
const todosRouter = Router()
todosRouter.get('/todos/:id?', ({ params }) => console.log({ id: params.id }))
parentRouter.get('/todos/*', todosRouter.handle) // all /todos/* routes will route through the todosRouter
const router = Router({ base: '/api/v0' })
router.get('/todos', indexHandler)
router.get('/todos/:id', itemHandler)
router.handle({ url: 'https://example.com/api/v0/todos' }) // --> fires indexHandler
yarn dev
yarn verify
const Router = (o = {}) =>
new Proxy({}, {
get: (t, k) => k === 'handle'
? async (q) => {
for ([p, hs] of t[(q.method || 'GET').toLowerCase()] || []) {
if (m = (u = new URL(q.url)).pathname.match(p)) {
q.params = m.groups
q.query = Object.fromEntries(u.searchParams.entries())
for (h of hs) {
if ((s = await h(q)) !== undefined) return s
}
}
}
}
: (p, ...hs) =>
(t[k] = t[k] || []).push([
`^${(o.base || '')+p
.replace(/(\/?)\*/g, '($1.*)?')
.replace(/(\/:([^\/\?]+)(\?)?)/gi, '/$3(?<$2>[^/]+)$3')
}$`,
hs
]) && t
})
This repo goes out to my past and present colleagues at Arundo - who have brought me such inspiration, fun,
and drive over the last couple years. In particular, the absurd brevity of this code is thanks to a
clever [abuse] of Proxy
, courtesy of the brilliant @mvasigh.
This trick allows methods (e.g. "get", "post") to by defined dynamically by the router as they are requested,
drastically reducing boilerplate.
Until this library makes it to a production release of v1.x, minor versions may contain breaking changes to the API. After v1.x, semantic versioning will be honored, and breaking changes will only occur under the umbrella of a major version bump.
{ base: '/some/path' }
to Router
for route prefixing, fix: trailing wildcard issue (e.g. /foo/*
should match /foo
)FAQs
A tiny, zero-dependency router, designed to make beautiful APIs in any environment.
The npm package itty-router receives a total of 31,386 weekly downloads. As such, itty-router popularity was classified as popular.
We found that itty-router demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.