Comparing version 1.8.0 to 1.9.0
@@ -7,3 +7,3 @@ { | ||
"authors": [ | ||
"_nK <https://nkdev.info>" | ||
"nK <https://nkdev.info>" | ||
], | ||
@@ -10,0 +10,0 @@ "keywords": [ |
/*! | ||
* Name : Video Worker (wrapper for Youtube, Vimeo and Local videos) | ||
* Version : 1.2.1 | ||
* Author : _nK https://nkdev.info | ||
* Version : 1.8.0 | ||
* Author : nK <https://nkdev.info> | ||
* GitHub : https://github.com/nk-o/jarallax | ||
*/ | ||
!function(e){"use strict";function t(e){e=e||{};for(var t=1;t<arguments.length;t++)if(arguments[t])for(var i in arguments[t])arguments[t].hasOwnProperty(i)&&(e[i]=arguments[t][i]);return e}function i(){this._done=[],this._fail=[]}function o(e,t,i){e.addEventListener?e.addEventListener(t,i):e.attachEvent("on"+t,function(){i.call(e)})}i.prototype={execute:function(e,t){var i=e.length;for(t=Array.prototype.slice.call(t);i--;)e[i].apply(null,t)},resolve:function(){this.execute(this._done,arguments)},reject:function(){this.execute(this._fail,arguments)},done:function(e){this._done.push(e)},fail:function(e){this._fail.push(e)}};var a=function(){function e(e,o){var a=this;a.url=e,a.options_default={autoplay:1,loop:1,mute:1,controls:0,startTime:0,endTime:0},a.options=t({},a.options_default,o),a.videoID=a.parseURL(e),a.videoID&&(a.ID=i++,a.loadAPI(),a.init())}var i=0;return e}();a.prototype.parseURL=function(e){function t(e){var t=/.*(?:youtu.be\/|v\/|u\/\w\/|embed\/|watch\?v=)([^#\&\?]*).*/,i=e.match(t);return!(!i||11!==i[1].length)&&i[1]}function i(e){var t=/https?:\/\/(?:www\.|player\.)?vimeo.com\/(?:channels\/(?:\w+\/)?|groups\/([^\/]*)\/videos\/|album\/(\d+)\/video\/|video\/|)(\d+)(?:$|\/|\?)/,i=e.match(t);return!(!i||!i[3])&&i[3]}function o(e){for(var t=e.split(/,(?=mp4\:|webm\:|ogv\:|ogg\:)/),i={},o=0,a=0;a<t.length;a++){var n=t[a].match(/^(mp4|webm|ogv|ogg)\:(.*)/);n&&n[1]&&n[2]&&(i["ogv"===n[1]?"ogg":n[1]]=n[2],o=1)}return!!o&&i}var a=t(e),n=i(e),r=o(e);return a?(this.type="youtube",a):n?(this.type="vimeo",n):!!r&&(this.type="local",r)},a.prototype.isValid=function(){return!!this.videoID},a.prototype.on=function(e,t){this.userEventsList=this.userEventsList||[],(this.userEventsList[e]||(this.userEventsList[e]=[])).push(t)},a.prototype.off=function(e,t){if(this.userEventsList&&this.userEventsList[e])if(t)for(var i=0;i<this.userEventsList[e].length;i++)this.userEventsList[e][i]===t&&(this.userEventsList[e][i]=!1);else delete this.userEventsList[e]},a.prototype.fire=function(e){var t=[].slice.call(arguments,1);if(this.userEventsList&&"undefined"!=typeof this.userEventsList[e])for(var i in this.userEventsList[e])this.userEventsList[e][i]&&this.userEventsList[e][i].apply(this,t)},a.prototype.play=function(e){var t=this;t.player&&("youtube"===t.type&&t.player.playVideo&&("undefined"!=typeof e&&t.player.seekTo(e||0),t.player.playVideo()),"vimeo"===t.type&&("undefined"!=typeof e&&t.player.setCurrentTime(e),t.player.getPaused().then(function(e){e&&t.player.play()})),"local"===t.type&&("undefined"!=typeof e&&(t.player.currentTime=e),t.player.play()))},a.prototype.pause=function(){this.player&&("youtube"===this.type&&this.player.pauseVideo&&this.player.pauseVideo(),"vimeo"===this.type&&this.player.pause(),"local"===this.type&&this.player.pause())},a.prototype.getImageURL=function(e){var t=this;if(t.videoImage)return void e(t.videoImage);if("youtube"===t.type){var i=["maxresdefault","sddefault","hqdefault","0"],o=0,a=new Image;a.onload=function(){120!==(this.naturalWidth||this.width)||o===i.length-1?(t.videoImage="https://img.youtube.com/vi/"+t.videoID+"/"+i[o]+".jpg",e(t.videoImage)):(o++,this.src="https://img.youtube.com/vi/"+t.videoID+"/"+i[o]+".jpg")},a.src="https://img.youtube.com/vi/"+t.videoID+"/"+i[o]+".jpg"}if("vimeo"===t.type){var n=new XMLHttpRequest;n.open("GET","https://vimeo.com/api/v2/video/"+t.videoID+".json",!0),n.onreadystatechange=function(){if(4===this.readyState&&this.status>=200&&this.status<400){var i=JSON.parse(this.responseText);t.videoImage=i[0].thumbnail_large,e(t.videoImage)}},n.send(),n=null}},a.prototype.getIframe=function(t){var i=this;return i.$iframe?void t(i.$iframe):void i.onAPIready(function(){function a(e,t,i){var o=document.createElement("source");o.src=t,o.type=i,e.appendChild(o)}var n;if(i.$iframe||(n=document.createElement("div"),n.style.display="none"),"youtube"===i.type){i.playerOptions={},i.playerOptions.videoId=i.videoID,i.playerOptions.playerVars={autohide:1,rel:0,autoplay:0},i.options.controls||(i.playerOptions.playerVars.iv_load_policy=3,i.playerOptions.playerVars.modestbranding=1,i.playerOptions.playerVars.controls=0,i.playerOptions.playerVars.showinfo=0,i.playerOptions.playerVars.disablekb=1);var r,p;i.playerOptions.events={onReady:function(e){i.options.mute&&e.target.mute(),i.options.autoplay&&i.play(i.options.startTime),i.fire("ready",e)},onStateChange:function(e){i.options.loop&&e.data===YT.PlayerState.ENDED&&i.play(i.options.startTime),r||e.data!==YT.PlayerState.PLAYING||(r=1,i.fire("started",e)),e.data===YT.PlayerState.PLAYING&&i.fire("play",e),e.data===YT.PlayerState.PAUSED&&i.fire("pause",e),e.data===YT.PlayerState.ENDED&&i.fire("end",e),i.options.endTime&&(e.data===YT.PlayerState.PLAYING?p=setInterval(function(){i.options.endTime&&i.player.getCurrentTime()>=i.options.endTime&&(i.options.loop?i.play(i.options.startTime):i.pause())},150):clearInterval(p))}};var s=!i.$iframe;if(s){var l=document.createElement("div");l.setAttribute("id",i.playerID),n.appendChild(l),document.body.appendChild(n)}i.player=i.player||new e.YT.Player(i.playerID,i.playerOptions),s&&(i.$iframe=document.getElementById(i.playerID),i.videoWidth=parseInt(i.$iframe.getAttribute("width"),10)||1280,i.videoHeight=parseInt(i.$iframe.getAttribute("height"),10)||720)}if("vimeo"===i.type){i.playerOptions="",i.playerOptions+="player_id="+i.playerID,i.playerOptions+="&autopause=0",i.options.controls||(i.playerOptions+="&badge=0&byline=0&portrait=0&title=0"),i.playerOptions+="&autoplay="+(i.options.autoplay?"1":"0"),i.playerOptions+="&loop="+(i.options.loop?1:0),i.$iframe||(i.$iframe=document.createElement("iframe"),i.$iframe.setAttribute("id",i.playerID),i.$iframe.setAttribute("src","https://player.vimeo.com/video/"+i.videoID+"?"+i.playerOptions),i.$iframe.setAttribute("frameborder","0"),n.appendChild(i.$iframe),document.body.appendChild(n)),i.player=i.player||new Vimeo.Player(i.$iframe),i.player.getVideoWidth().then(function(e){i.videoWidth=e||1280}),i.player.getVideoHeight().then(function(e){i.videoHeight=e||720}),i.player.setVolume(i.options.mute?0:100);var d;i.player.on("timeupdate",function(e){d||i.fire("started",e),d=1,i.options.endTime&&i.options.endTime&&e.seconds>=i.options.endTime&&(i.options.loop?i.play(i.options.startTime):i.pause())}),i.player.on("play",function(e){i.fire("play",e),i.options.startTime&&0===e.seconds&&i.play(i.options.startTime)}),i.player.on("pause",function(e){i.fire("pause",e)}),i.player.on("ended",function(e){i.fire("end",e)}),i.player.on("loaded",function(e){i.fire("ready",e)})}if("local"===i.type){if(!i.$iframe){i.$iframe=document.createElement("video"),i.options.mute&&(i.$iframe.muted=!0),i.options.loop&&(i.$iframe.loop=!0),i.$iframe.setAttribute("id",i.playerID),n.appendChild(i.$iframe),document.body.appendChild(n);for(var u in i.videoID)a(i.$iframe,i.videoID[u],"video/"+u)}i.player=i.player||i.$iframe;var y;o(i.player,"playing",function(e){y||i.fire("started",e),y=1}),o(i.player,"timeupdate",function(){i.options.endTime&&i.options.endTime&&this.currentTime>=i.options.endTime&&(i.options.loop?i.play(i.options.startTime):i.pause())}),o(i.player,"play",function(e){i.fire("play",e)}),o(i.player,"pause",function(e){i.fire("pause",e)}),o(i.player,"ended",function(e){i.fire("end",e)}),o(i.player,"loadedmetadata",function(){i.videoWidth=this.videoWidth||1280,i.videoHeight=this.videoHeight||720,i.fire("ready"),i.options.autoplay&&i.play(i.options.startTime)})}t(i.$iframe)})},a.prototype.init=function(){var e=this;e.playerID="VideoWorker-"+e.ID};var n=0,r=0;a.prototype.loadAPI=function(){var t=this;if(!n||!r){var i="";if("youtube"!==t.type||n||(n=1,i="//www.youtube.com/iframe_api"),"vimeo"!==t.type||r||(r=1,i="//player.vimeo.com/api/player.js"),i){"file://"===e.location.origin&&(i="http:"+i);var o=document.createElement("script"),a=document.getElementsByTagName("head")[0];o.src=i,a.appendChild(o),a=null,o=null}}};var p=0,s=0,l=new i,d=new i;a.prototype.onAPIready=function(t){var i=this;if("youtube"===i.type&&("undefined"!=typeof YT&&0!==YT.loaded||p?"object"==typeof YT&&1===YT.loaded?t():l.done(function(){t()}):(p=1,e.onYouTubeIframeAPIReady=function(){e.onYouTubeIframeAPIReady=null,l.resolve("done"),t()})),"vimeo"===i.type)if("undefined"!=typeof Vimeo||s)"undefined"!=typeof Vimeo?t():d.done(function(){t()});else{s=1;var o=setInterval(function(){"undefined"!=typeof Vimeo&&(clearInterval(o),d.resolve("done"),t())},20)}"local"===i.type&&t()},e.VideoWorker=a}(window),/*! | ||
!function(){"use strict";function e(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function t(){this._done=[],this._fail=[]}function i(e,t,i){e.addEventListener?e.addEventListener(t,i):e.attachEvent("on"+t,function(){i.call(e)})}var o="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e},a=function(){function e(e,t){for(var i=0;i<t.length;i++){var o=t[i];o.enumerable=o.enumerable||!1,o.configurable=!0,"value"in o&&(o.writable=!0),Object.defineProperty(e,o.key,o)}}return function(t,i,o){return i&&e(t.prototype,i),o&&e(t,o),t}}();t.prototype={execute:function(e,t){var i=e.length;for(t=Array.prototype.slice.call(t);i--;)e[i].apply(null,t)},resolve:function(){this.execute(this._done,arguments)},reject:function(){this.execute(this._fail,arguments)},done:function(e){this._done.push(e)},fail:function(e){this._fail.push(e)}};var n=0,r=0,p=0,l=0,s=0,u=new t,d=new t,y=function(){function t(i,o){e(this,t);var a=this;a.url=i,a.options_default={autoplay:1,loop:1,mute:1,volume:0,controls:0,startTime:0,endTime:0},a.options=a.extend({},a.options_default,o),a.videoID=a.parseURL(i),a.videoID&&(a.ID=n++,a.loadAPI(),a.init())}return a(t,[{key:"extend",value:function(e){var t=arguments;return e=e||{},Object.keys(arguments).forEach(function(i){t[i]&&Object.keys(t[i]).forEach(function(o){e[o]=t[i][o]})}),e}},{key:"parseURL",value:function(e){function t(e){var t=/.*(?:youtu.be\/|v\/|u\/\w\/|embed\/|watch\?v=)([^#\&\?]*).*/,i=e.match(t);return!(!i||11!==i[1].length)&&i[1]}function i(e){var t=/https?:\/\/(?:www\.|player\.)?vimeo.com\/(?:channels\/(?:\w+\/)?|groups\/([^\/]*)\/videos\/|album\/(\d+)\/video\/|video\/|)(\d+)(?:$|\/|\?)/,i=e.match(t);return!(!i||!i[3])&&i[3]}function o(e){var t=e.split(/,(?=mp4\:|webm\:|ogv\:|ogg\:)/),i={},o=0;return t.forEach(function(e){var t=e.match(/^(mp4|webm|ogv|ogg)\:(.*)/);t&&t[1]&&t[2]&&(i["ogv"===t[1]?"ogg":t[1]]=t[2],o=1)}),!!o&&i}var a=t(e),n=i(e),r=o(e);return a?(this.type="youtube",a):n?(this.type="vimeo",n):!!r&&(this.type="local",r)}},{key:"isValid",value:function(){return!!this.videoID}},{key:"on",value:function(e,t){this.userEventsList=this.userEventsList||[],(this.userEventsList[e]||(this.userEventsList[e]=[])).push(t)}},{key:"off",value:function(e,t){var i=this;this.userEventsList&&this.userEventsList[e]&&(t?this.userEventsList[e].forEach(function(o,a){o===t&&(i.userEventsList[e][a]=!1)}):delete this.userEventsList[e])}},{key:"fire",value:function(e){var t=this,i=[].slice.call(arguments,1);this.userEventsList&&"undefined"!=typeof this.userEventsList[e]&&this.userEventsList[e].forEach(function(e){e&&e.apply(t,i)})}},{key:"play",value:function(e){var t=this;t.player&&("youtube"===t.type&&t.player.playVideo&&("undefined"!=typeof e&&t.player.seekTo(e||0),YT.PlayerState.PLAYING!==t.player.getPlayerState()&&t.player.playVideo()),"vimeo"===t.type&&("undefined"!=typeof e&&t.player.setCurrentTime(e),t.player.getPaused().then(function(e){e&&t.player.play()})),"local"===t.type&&("undefined"!=typeof e&&(t.player.currentTime=e),t.player.paused&&t.player.play()))}},{key:"pause",value:function(){var e=this;e.player&&("youtube"===e.type&&e.player.pauseVideo&&YT.PlayerState.PLAYING===e.player.getPlayerState()&&e.player.pauseVideo(),"vimeo"===e.type&&e.player.getPaused().then(function(t){t||e.player.pause()}),"local"===e.type&&(e.player.paused||e.player.pause()))}},{key:"getImageURL",value:function(e){var t=this;if(t.videoImage)return void e(t.videoImage);if("youtube"===t.type){var i=["maxresdefault","sddefault","hqdefault","0"],o=0,a=new Image;a.onload=function(){120!==(this.naturalWidth||this.width)||o===i.length-1?(t.videoImage="https://img.youtube.com/vi/"+t.videoID+"/"+i[o]+".jpg",e(t.videoImage)):(o++,this.src="https://img.youtube.com/vi/"+t.videoID+"/"+i[o]+".jpg")},a.src="https://img.youtube.com/vi/"+t.videoID+"/"+i[o]+".jpg"}if("vimeo"===t.type){var n=new XMLHttpRequest;n.open("GET","https://vimeo.com/api/v2/video/"+t.videoID+".json",!0),n.onreadystatechange=function(){if(4===this.readyState&&this.status>=200&&this.status<400){var i=JSON.parse(this.responseText);t.videoImage=i[0].thumbnail_large,e(t.videoImage)}},n.send(),n=null}}},{key:"getIframe",value:function(e){var t=this;return t.$iframe?void e(t.$iframe):void t.onAPIready(function(){function o(e,t,i){var o=document.createElement("source");o.src=t,o.type=i,e.appendChild(o)}var a=void 0;if(t.$iframe||(a=document.createElement("div"),a.style.display="none"),"youtube"===t.type){t.playerOptions={},t.playerOptions.videoId=t.videoID,t.playerOptions.playerVars={autohide:1,rel:0,autoplay:0},t.options.controls||(t.playerOptions.playerVars.iv_load_policy=3,t.playerOptions.playerVars.modestbranding=1,t.playerOptions.playerVars.controls=0,t.playerOptions.playerVars.showinfo=0,t.playerOptions.playerVars.disablekb=1);var n=void 0,r=void 0;t.playerOptions.events={onReady:function(e){t.options.mute?e.target.mute():t.options.volume&&e.target.setVolume(t.options.volume),t.options.autoplay&&t.play(t.options.startTime),t.fire("ready",e)},onStateChange:function(e){t.options.loop&&e.data===YT.PlayerState.ENDED&&t.play(t.options.startTime),n||e.data!==YT.PlayerState.PLAYING||(n=1,t.fire("started",e)),e.data===YT.PlayerState.PLAYING&&t.fire("play",e),e.data===YT.PlayerState.PAUSED&&t.fire("pause",e),e.data===YT.PlayerState.ENDED&&t.fire("end",e),t.options.endTime&&(e.data===YT.PlayerState.PLAYING?r=setInterval(function(){t.options.endTime&&t.player.getCurrentTime()>=t.options.endTime&&(t.options.loop?t.play(t.options.startTime):t.pause())},150):clearInterval(r))}};var p=!t.$iframe;if(p){var l=document.createElement("div");l.setAttribute("id",t.playerID),a.appendChild(l),document.body.appendChild(a)}t.player=t.player||new window.YT.Player(t.playerID,t.playerOptions),p&&(t.$iframe=document.getElementById(t.playerID),t.videoWidth=parseInt(t.$iframe.getAttribute("width"),10)||1280,t.videoHeight=parseInt(t.$iframe.getAttribute("height"),10)||720)}if("vimeo"===t.type){t.playerOptions="",t.playerOptions+="player_id="+t.playerID,t.playerOptions+="&autopause=0",t.options.controls||(t.playerOptions+="&badge=0&byline=0&portrait=0&title=0"),t.playerOptions+="&autoplay="+(t.options.autoplay?"1":"0"),t.playerOptions+="&loop="+(t.options.loop?1:0),t.$iframe||(t.$iframe=document.createElement("iframe"),t.$iframe.setAttribute("id",t.playerID),t.$iframe.setAttribute("src","https://player.vimeo.com/video/"+t.videoID+"?"+t.playerOptions),t.$iframe.setAttribute("frameborder","0"),a.appendChild(t.$iframe),document.body.appendChild(a)),t.player=t.player||new Vimeo.Player(t.$iframe),t.player.getVideoWidth().then(function(e){t.videoWidth=e||1280}),t.player.getVideoHeight().then(function(e){t.videoHeight=e||720}),t.options.startTime&&t.options.autoplay&&t.player.setCurrentTime(t.options.startTime),t.options.mute?t.player.setVolume(0):t.options.volume&&t.player.setVolume(t.options.volume);var s=void 0;t.player.on("timeupdate",function(e){s||t.fire("started",e),s=1,t.options.endTime&&t.options.endTime&&e.seconds>=t.options.endTime&&(t.options.loop?t.play(t.options.startTime):t.pause())}),t.player.on("play",function(e){t.fire("play",e),t.options.startTime&&0===e.seconds&&t.play(t.options.startTime)}),t.player.on("pause",function(e){t.fire("pause",e)}),t.player.on("ended",function(e){t.fire("end",e)}),t.player.on("loaded",function(e){t.fire("ready",e)})}if("local"===t.type){t.$iframe||(t.$iframe=document.createElement("video"),t.options.mute?t.$iframe.muted=!0:t.$iframe.volume&&(t.$iframe.volume=t.options.volume/100),t.options.loop&&(t.$iframe.loop=!0),t.$iframe.setAttribute("id",t.playerID),a.appendChild(t.$iframe),document.body.appendChild(a),Object.keys(t.videoID).forEach(function(e){o(t.$iframe,t.videoID[e],"video/"+e)})),t.player=t.player||t.$iframe;var u=void 0;i(t.player,"playing",function(e){u||t.fire("started",e),u=1}),i(t.player,"timeupdate",function(){t.options.endTime&&t.options.endTime&&this.currentTime>=t.options.endTime&&(t.options.loop?t.play(t.options.startTime):t.pause())}),i(t.player,"play",function(e){t.fire("play",e)}),i(t.player,"pause",function(e){t.fire("pause",e)}),i(t.player,"ended",function(e){t.fire("end",e)}),i(t.player,"loadedmetadata",function(){t.videoWidth=this.videoWidth||1280,t.videoHeight=this.videoHeight||720,t.fire("ready"),t.options.autoplay&&t.play(t.options.startTime)})}e(t.$iframe)})}},{key:"init",value:function(){var e=this;e.playerID="VideoWorker-"+e.ID}},{key:"loadAPI",value:function(){var e=this;if(!r||!p){var t="";if("youtube"!==e.type||r||(r=1,t="https://www.youtube.com/iframe_api"),"vimeo"!==e.type||p||(p=1,t="https://player.vimeo.com/api/player.js"),t){var i=document.createElement("script"),o=document.getElementsByTagName("head")[0];i.src=t,o.appendChild(i),o=null,i=null}}}},{key:"onAPIready",value:function(e){var t=this;if("youtube"===t.type&&("undefined"!=typeof YT&&0!==YT.loaded||l?"object"===("undefined"==typeof YT?"undefined":o(YT))&&1===YT.loaded?e():u.done(function(){e()}):(l=1,window.onYouTubeIframeAPIReady=function(){window.onYouTubeIframeAPIReady=null,u.resolve("done"),e()})),"vimeo"===t.type)if("undefined"!=typeof Vimeo||s)"undefined"!=typeof Vimeo?e():d.done(function(){e()});else{s=1;var i=setInterval(function(){"undefined"!=typeof Vimeo&&(clearInterval(i),d.resolve("done"),e())},20)}"local"===t.type&&e()}}]),t}();window.VideoWorker=y,/*! | ||
* Name : Video Background Extension for Jarallax | ||
* Version : 1.0.0 | ||
* Author : _nK http://nkdev.info | ||
* Author : nK http://nkdev.info | ||
* GitHub : https://github.com/nk-o/jarallax | ||
*/ | ||
function(){"use strict";if("undefined"!=typeof jarallax){var e=jarallax.constructor,t=e.prototype.init;e.prototype.init=function(){var e=this;t.apply(e),e.video&&e.video.getIframe(function(t){var i=t.parentNode;e.css(t,{position:e.image.position,top:"0px",left:"0px",right:"0px",bottom:"0px",width:"100%",height:"100%",maxWidth:"none",maxHeight:"none",visibility:"hidden",margin:0,zIndex:-1}),e.$video=t,e.image.$container.appendChild(t),i.parentNode.removeChild(i)})};var i=e.prototype.coverImage;e.prototype.coverImage=function(){var e=this;i.apply(e),e.video&&"IFRAME"===e.image.$item.nodeName&&e.css(e.image.$item,{height:e.image.$item.getBoundingClientRect().height+400+"px",marginTop:-200+parseFloat(e.css(e.image.$item,"margin-top"))+"px"})};var o=e.prototype.initImg;e.prototype.initImg=function(){var e=this,t=o.apply(e);if(e.options.videoSrc||(e.options.videoSrc=e.$item.getAttribute("data-jarallax-video")||!1),e.options.videoSrc){var i=new VideoWorker(e.options.videoSrc,{startTime:e.options.videoStartTime||0,endTime:e.options.videoEndTime||0});if(i.isValid()&&(e.image.useImgTag=!0,i.on("ready",function(){var t=e.onScroll;e.onScroll=function(){t.apply(e),e.isVisible()?i.play():i.pause()}}),i.on("started",function(){e.image.$default_item=e.image.$item,e.image.$item=e.$video,e.image.width=e.options.imgWidth=e.video.videoWidth||1280,e.image.height=e.options.imgHeight=e.video.videoHeight||720,e.coverImage(),e.clipContainer(),e.onScroll(),e.image.$default_item&&(e.image.$default_item.style.display="none")}),e.video=i,"local"!==i.type&&i.getImageURL(function(t){e.image.src=t,e.init()})),"local"!==i.type)return!1;if(!t)return e.image.src="data:image/gif;base64,R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7",!0}return t};var a=e.prototype.destroy;e.prototype.destroy=function(){var e=this;a.apply(e)}}}(); | ||
function(){if("undefined"!=typeof jarallax){var e=jarallax.constructor,t=e.prototype.init;e.prototype.init=function(){var e=this;t.apply(e),e.video&&e.video.getIframe(function(t){var i=t.parentNode;e.css(t,{position:e.image.position,top:"0px",left:"0px",right:"0px",bottom:"0px",width:"100%",height:"100%",maxWidth:"none",maxHeight:"none",margin:0,zIndex:-1}),e.$video=t,e.image.$container.appendChild(t),i.parentNode.removeChild(i)})};var o=e.prototype.coverImage;e.prototype.coverImage=function(){var e=this,t=o.apply(e),i=e.image.$item.nodeName;if(t&&e.video&&("IFRAME"===i||"VIDEO"===i)){var a=t.image.height,n=a*e.image.width/e.image.height,r=(t.container.width-n)/2,p=t.image.marginTop;t.container.width>n&&(n=t.container.width,a=n*e.image.height/e.image.width,r=0,p+=(t.image.height-a)/2),"IFRAME"===i&&(a+=400,p-=200),e.css(e.$video,{width:n+"px",marginLeft:r+"px",height:a+"px",marginTop:p+"px"})}return t};var a=e.prototype.initImg;e.prototype.initImg=function(){var e=this,t=a.apply(e);return e.options.videoSrc||(e.options.videoSrc=e.$item.getAttribute("data-jarallax-video")||null),e.options.videoSrc?(e.defaultInitImgResult=t,!0):t};var n=e.prototype.canInitParallax;e.prototype.canInitParallax=function(){var e=this,t=n.apply(e);if(!e.options.videoSrc)return t;var i=new y(e.options.videoSrc,{startTime:e.options.videoStartTime||0,endTime:e.options.videoEndTime||0,mute:e.options.videoVolume?0:1,volume:e.options.videoVolume||0});if(i.isValid())if(t){if(e.image.useImgTag=!0,i.on("ready",function(){if(e.options.videoPlayOnlyVisible){var t=e.onScroll;e.onScroll=function(){t.apply(e),e.isVisible()?i.play():i.pause()}}else i.play()}),i.on("started",function(){e.image.$default_item=e.image.$item,e.image.$item=e.$video,e.image.width=e.video.videoWidth||1280,e.image.height=e.video.videoHeight||720,e.options.imgWidth=e.image.width,e.options.imgHeight=e.image.height,e.coverImage(),e.clipContainer(),e.onScroll(),e.image.$default_item&&(e.image.$default_item.style.display="none")}),e.video=i,!e.defaultInitImgResult)return"local"!==i.type?(i.getImageURL(function(t){e.image.src=t,e.init()}),!1):(e.image.src="data:image/gif;base64,R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7",!0)}else e.defaultInitImgResult||i.getImageURL(function(t){var i=e.$item.getAttribute("style");i&&e.$item.setAttribute("data-jarallax-original-styles",i),e.css(e.$item,{"background-image":'url("'+t+'")',"background-position":"center","background-size":"cover"})});return t};var r=e.prototype.destroy;e.prototype.destroy=function(){var e=this;e.image.$default_item&&(e.image.$item=e.image.$default_item,delete e.image.$default_item),r.apply(e)},i(window,"DOMContentLoaded",function(){jarallax(document.querySelectorAll("[data-jarallax-video]"))})}}()}(); |
/*! | ||
* Name : Just Another Parallax [Jarallax] | ||
* Version : 1.8.0 | ||
* Author : _nK https://nkdev.info | ||
* Author : nK <https://nkdev.info> | ||
* GitHub : https://github.com/nk-o/jarallax | ||
*/ | ||
!function(e){"use strict";function t(e){var t=["O","Moz","ms","Ms","Webkit"],n=t.length;if(void 0!==l.style[e])return!0;for(e=e.charAt(0).toUpperCase()+e.substr(1);--n>-1&&void 0===l.style[t[n]+e];);return n>=0}function n(){a=e.innerWidth||document.documentElement.clientWidth,r=e.innerHeight||document.documentElement.clientHeight}function i(e,t,n){e.addEventListener?e.addEventListener(t,n):e.attachEvent("on"+t,function(){n.call(e)})}function o(t){e.requestAnimationFrame(function(){"scroll"!==t.type&&n();for(var e=0,i=h.length;e<i;e++)"scroll"!==t.type&&(h[e].coverImage(),h[e].clipContainer()),h[e].onScroll()})}Date.now||(Date.now=function(){return(new Date).getTime()}),e.requestAnimationFrame||!function(){for(var t=["webkit","moz"],n=0;n<t.length&&!e.requestAnimationFrame;++n){var i=t[n];e.requestAnimationFrame=e[i+"RequestAnimationFrame"],e.cancelAnimationFrame=e[i+"CancelAnimationFrame"]||e[i+"CancelRequestAnimationFrame"]}if(/iP(ad|hone|od).*OS 6/.test(e.navigator.userAgent)||!e.requestAnimationFrame||!e.cancelAnimationFrame){var o=0;e.requestAnimationFrame=function(e){var t=Date.now(),n=Math.max(o+16,t);return setTimeout(function(){e(o=n)},n-t)},e.cancelAnimationFrame=clearTimeout}}();var a,r,l=document.createElement("div"),s=t("transform"),c=t("perspective"),m=navigator.userAgent,p=m.toLowerCase().indexOf("android")>-1,u=/iPad|iPhone|iPod/.test(m)&&!e.MSStream,d=m.toLowerCase().indexOf("firefox")>-1,g=m.indexOf("MSIE ")>-1||m.indexOf("Trident/")>-1||m.indexOf("Edge/")>-1,f=document.all&&!e.atob;n();var h=[],y=function(){function e(e,n){var i,o=this;if(o.$item=e,o.defaults={type:"scroll",speed:.5,imgSrc:null,imgWidth:null,imgHeight:null,elementInViewport:null,zIndex:-100,noAndroid:!1,noIos:!0,onScroll:null,onInit:null,onDestroy:null,onCoverImage:null},i=JSON.parse(o.$item.getAttribute("data-jarallax")||"{}"),o.options=o.extend({},o.defaults,i,n),!(!s||p&&o.options.noAndroid||u&&o.options.noIos)){o.options.speed=Math.min(2,Math.max(-1,parseFloat(o.options.speed)));var a=o.options.elementInViewport;a&&"object"==typeof a&&"undefined"!=typeof a.length&&(a=a[0]),!a instanceof Element&&(a=null),o.options.elementInViewport=a,o.instanceID=t++,o.image={src:o.options.imgSrc||null,$container:null,$item:null,width:o.options.imgWidth||null,height:o.options.imgHeight||null,useImgTag:u||p||g,position:!c||d?"absolute":"fixed"},o.initImg()&&o.init()}}var t=0;return e}();y.prototype.css=function(t,n){if("string"==typeof n)return e.getComputedStyle?e.getComputedStyle(t).getPropertyValue(n):t.style[n];n.transform&&(c&&(n.transform+=" translateZ(0)"),n.WebkitTransform=n.MozTransform=n.msTransform=n.OTransform=n.transform);for(var i in n)t.style[i]=n[i];return t},y.prototype.extend=function(e){e=e||{};for(var t=1;t<arguments.length;t++)if(arguments[t])for(var n in arguments[t])arguments[t].hasOwnProperty(n)&&(e[n]=arguments[t][n]);return e},y.prototype.initImg=function(){var e=this;return null===e.image.src&&(e.image.src=e.css(e.$item,"background-image").replace(/^url\(['"]?/g,"").replace(/['"]?\)$/g,"")),!(!e.image.src||"none"===e.image.src)},y.prototype.init=function(){function e(){t.coverImage(),t.clipContainer(),t.onScroll(!0),t.options.onInit&&t.options.onInit.call(t),setTimeout(function(){t.$item&&t.css(t.$item,{"background-image":"none","background-attachment":"scroll","background-size":"auto"})},0)}var t=this,n={position:"absolute",top:0,left:0,width:"100%",height:"100%",overflow:"hidden",pointerEvents:"none"},i={};t.$item.setAttribute("data-jarallax-original-styles",t.$item.getAttribute("style")),"static"===t.css(t.$item,"position")&&t.css(t.$item,{position:"relative"}),"auto"===t.css(t.$item,"z-index")&&t.css(t.$item,{zIndex:0}),t.image.$container=document.createElement("div"),t.css(t.image.$container,n),t.css(t.image.$container,{visibility:"hidden","z-index":t.options.zIndex}),t.image.$container.setAttribute("id","jarallax-container-"+t.instanceID),t.$item.appendChild(t.image.$container),t.image.useImgTag?(t.image.$item=document.createElement("img"),t.image.$item.setAttribute("src",t.image.src),i=t.extend({"max-width":"none"},n,i)):(t.image.$item=document.createElement("div"),i=t.extend({"background-position":"50% 50%","background-size":"100% auto","background-repeat":"no-repeat no-repeat","background-image":'url("'+t.image.src+'")'},n,i));for(var o=0,a=t.$item;null!==a&&a!==document&&0===o;){var r=t.css(a,"-webkit-transform")||t.css(a,"-moz-transform")||t.css(a,"transform");r&&"none"!==r&&(o=1,t.css(t.image.$container,{transform:"translateX(0) translateY(0)"})),a=a.parentNode}(o||"opacity"===t.options.type||"scale"===t.options.type||"scale-opacity"===t.options.type)&&(t.image.position="absolute"),i.position=t.image.position,t.css(t.image.$item,i),t.image.$container.appendChild(t.image.$item),t.image.width&&t.image.height?e():t.getImageSize(t.image.src,function(n,i){t.image.width=n,t.image.height=i,e()}),h.push(t)},y.prototype.destroy=function(){for(var e=this,t=0,n=h.length;t<n;t++)if(h[t].instanceID===e.instanceID){h.splice(t,1);break}var i=e.$item.getAttribute("data-jarallax-original-styles");e.$item.removeAttribute("data-jarallax-original-styles"),"null"===i?e.$item.removeAttribute("style"):e.$item.setAttribute("style",i),e.$clipStyles&&e.$clipStyles.parentNode.removeChild(e.$clipStyles),e.image.$container.parentNode.removeChild(e.image.$container),e.options.onDestroy&&e.options.onDestroy.call(e),delete e.$item.jarallax;for(var o in e)delete e[o]},y.prototype.getImageSize=function(e,t){if(e&&t){var n=new Image;n.onload=function(){t(n.width,n.height)},n.src=e}},y.prototype.clipContainer=function(){if(!f){var e=this,t=e.image.$container.getBoundingClientRect(),n=t.width,i=t.height;if(!e.$clipStyles){e.$clipStyles=document.createElement("style"),e.$clipStyles.setAttribute("type","text/css"),e.$clipStyles.setAttribute("id","#jarallax-clip-"+e.instanceID);var o=document.head||document.getElementsByTagName("head")[0];o.appendChild(e.$clipStyles)}var a=["#jarallax-container-"+e.instanceID+" {"," clip: rect(0 "+n+"px "+i+"px 0);"," clip: rect(0, "+n+"px, "+i+"px, 0);","}"].join("\n");e.$clipStyles.styleSheet?e.$clipStyles.styleSheet.cssText=a:e.$clipStyles.innerHTML=a}},y.prototype.coverImage=function(){var e=this;if(e.image.width&&e.image.height){var t=e.image.$container.getBoundingClientRect(),n=t.width,i=t.height,o=t.left,a=e.image.width,l=e.image.height,s=e.options.speed,c="scroll"===e.options.type||"scroll-opacity"===e.options.type,m=0,p=0,u=i,d=0,g=0;c&&(m=s<0?s*Math.max(i,r):s*(i+r),s>1?u=Math.abs(m-r):s<0?u=m/s+Math.abs(m):u+=Math.abs(r-i)*(1-s),m/=2),p=u*a/l,p<n&&(p=n,u=p*l/a),c?(d=o+(n-p)/2,g=(r-u)/2):(d=(n-p)/2,g=(i-u)/2),"absolute"===e.image.position&&(d-=o),e.parallaxScrollDistance=m,e.css(e.image.$item,{width:p+"px",height:u+"px",marginLeft:d+"px",marginTop:g+"px"}),e.options.onCoverImage&&e.options.onCoverImage.call(e)}},y.prototype.isVisible=function(){return this.isElementInViewport||!1},y.prototype.onScroll=function(e){var t=this;if(t.image.width&&t.image.height){var n=t.$item.getBoundingClientRect(),i=n.top,o=n.height,l={visibility:"visible",backgroundPosition:"50% 50%"},s=n;if(t.options.elementInViewport&&(s=t.options.elementInViewport.getBoundingClientRect()),t.isElementInViewport=s.bottom>=0&&s.right>=0&&s.top<=r&&s.left<=a,e||t.isElementInViewport){var c=Math.max(0,i),m=Math.max(0,o+i),p=Math.max(0,-i),u=Math.max(0,i+o-r),d=Math.max(0,o-(i+o-r)),g=Math.max(0,-i+r-o),f=1-2*(r-i)/(r+o),h=1;if(o<r?h=1-(p||u)/o:m<=r?h=m/r:d<=r&&(h=d/r),"opacity"!==t.options.type&&"scale-opacity"!==t.options.type&&"scroll-opacity"!==t.options.type||(l.transform="",l.opacity=h),"scale"===t.options.type||"scale-opacity"===t.options.type){var y=1;t.options.speed<0?y-=t.options.speed*h:y+=t.options.speed*(1-h),l.transform="scale("+y+")"}if("scroll"===t.options.type||"scroll-opacity"===t.options.type){var v=t.parallaxScrollDistance*f;"absolute"===t.image.position&&(v-=i),l.transform="translateY("+v+"px)"}t.css(t.image.$item,l),t.options.onScroll&&t.options.onScroll.call(t,{section:n,beforeTop:c,beforeTopEnd:m,afterTop:p,beforeBottom:u,beforeBottomEnd:d,afterBottom:g,visiblePercent:h,fromViewportCenter:f})}}},i(e,"scroll",o),i(e,"resize",o),i(e,"orientationchange",o),i(e,"load",o);var v=function(e){("object"==typeof HTMLElement?e instanceof HTMLElement:e&&"object"==typeof e&&null!==e&&1===e.nodeType&&"string"==typeof e.nodeName)&&(e=[e]);var t,n=arguments[1],i=Array.prototype.slice.call(arguments,2),o=e.length,a=0;for(a;a<o;a++)if("object"==typeof n||"undefined"==typeof n?e[a].jarallax||(e[a].jarallax=new y(e[a],n)):e[a].jarallax&&(t=e[a].jarallax[n].apply(e[a].jarallax,i)),"undefined"!=typeof t)return t;return e};v.constructor=y;var x=e.jarallax;if(e.jarallax=v,e.jarallax.noConflict=function(){return e.jarallax=x,this},"undefined"!=typeof jQuery){var $=function(){var t=arguments||[];Array.prototype.unshift.call(t,this);var n=v.apply(e,t);return"object"!=typeof n?n:this};$.constructor=y;var b=jQuery.fn.jarallax;jQuery.fn.jarallax=$,jQuery.fn.jarallax.noConflict=function(){return jQuery.fn.jarallax=b,this}}i(e,"DOMContentLoaded",function(){v(document.querySelectorAll("[data-jarallax], [data-jarallax-video]"))})}(window); | ||
!function(){"use strict";function e(e,t){if(!(e instanceof t))throw new TypeError("Cannot call a class as a function")}function t(e){var t=["O","Moz","ms","Ms","Webkit"],i=t.length;if(void 0!==s.style[e])return!0;for(e=e.charAt(0).toUpperCase()+e.substr(1);--i>-1&&void 0===s.style[t[i]+e];);return i>=0}function i(e,t,i){e.addEventListener?e.addEventListener(t,i):e.attachEvent("on"+t,function(){i.call(e)})}function n(){h=window.innerWidth||document.documentElement.clientWidth,v=window.innerHeight||document.documentElement.clientHeight}function o(){if(x.length){b=void 0!==window.pageYOffset?window.pageYOffset:(document.documentElement||document.body.parentNode||document.body).scrollTop;var e=!w||w.width!==h||w.height!==v,t=e||!w||w.y!==b;(e||t)&&x.forEach(function(i){e&&i.onResize(),t&&i.onScroll()}),w={width:h,height:v,y:b},y(o)}}var a="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(e){return typeof e}:function(e){return e&&"function"==typeof Symbol&&e.constructor===Symbol&&e!==Symbol.prototype?"symbol":typeof e},r=function(){function e(e,t){for(var i=0;i<t.length;i++){var n=t[i];n.enumerable=n.enumerable||!1,n.configurable=!0,"value"in n&&(n.writable=!0),Object.defineProperty(e,n.key,n)}}return function(t,i,n){return i&&e(t.prototype,i),n&&e(t,n),t}}(),s=document.createElement("div"),l=t("transform"),m=t("perspective"),c=navigator.userAgent,p=c.toLowerCase().indexOf("android")>-1,u=/iPad|iPhone|iPod/.test(c)&&!window.MSStream,d=c.toLowerCase().indexOf("firefox")>-1,f=c.indexOf("MSIE ")>-1||c.indexOf("Trident/")>-1||c.indexOf("Edge/")>-1,g=document.all&&!window.atob,y=window.requestAnimationFrame||window.webkitRequestAnimationFrame||window.mozRequestAnimationFrame||function(e){setTimeout(e,1e3/60)},h=void 0,v=void 0,b=void 0;n(),i(window,"resize",n),i(window,"orientationchange",n),i(window,"load",n);var x=[],w=!1,$=0,j=function(){function t(i,n){e(this,t);var o=this;o.instanceID=$++,o.$item=i,o.defaults={type:"scroll",speed:.5,imgSrc:null,imgElement:".jarallax-img",imgSize:"cover",imgPosition:"50% 50%",imgRepeat:"no-repeat",keepImg:!1,elementInViewport:null,zIndex:-100,noAndroid:!1,noIos:!1,videoSrc:null,videoStartTime:0,videoEndTime:0,videoVolume:0,videoPlayOnlyVisible:!0,onScroll:null,onInit:null,onDestroy:null,onCoverImage:null};var r=o.$item.getAttribute("data-jarallax"),s=JSON.parse(r||"{}");r&&console.warn("Detected usage of deprecated data-jarallax JSON options, you should use pure data-attribute options. See info here - https://github.com/nk-o/jarallax/issues/53");var l=o.$item.dataset,c={};Object.keys(l).forEach(function(e){var t=e.substr(0,1).toLowerCase()+e.substr(1);t&&"undefined"!=typeof o.defaults[t]&&(c[t]=l[e])}),o.options=o.extend({},o.defaults,s,c,n),o.pureOptions=o.extend({},o.options),Object.keys(o.options).forEach(function(e){"true"===o.options[e]?o.options[e]=!0:"false"===o.options[e]&&(o.options[e]=!1)}),o.options.speed=Math.min(2,Math.max(-1,parseFloat(o.options.speed)));var g=o.options.elementInViewport;g&&"object"===("undefined"==typeof g?"undefined":a(g))&&"undefined"!=typeof g.length&&(g=g[0]),g instanceof Element||(g=null),o.options.elementInViewport=g,o.image={src:o.options.imgSrc||null,$container:null,useImgTag:u||p||f,position:!m||d?"absolute":"fixed"},o.initImg()&&o.canInitParallax()&&o.init()}return r(t,[{key:"css",value:function(e,t){return"string"==typeof t?window.getComputedStyle?window.getComputedStyle(e).getPropertyValue(t):e.style[t]:(t.transform&&(m&&(t.transform+=" translateZ(0)"),t.WebkitTransform=t.transform,t.MozTransform=t.transform,t.msTransform=t.transform,t.OTransform=t.transform),Object.keys(t).forEach(function(i){e.style[i]=t[i]}),e)}},{key:"extend",value:function(e){var t=arguments;return e=e||{},Object.keys(arguments).forEach(function(i){t[i]&&Object.keys(t[i]).forEach(function(n){e[n]=t[i][n]})}),e}},{key:"getWindowData",value:function(){return{width:h,height:v,y:b}}},{key:"initImg",value:function(){var e=this,t=e.options.imgElement;return t&&"string"==typeof t&&(t=e.$item.querySelector(t)),t instanceof Element||(t=null),t&&(e.options.keepImg?e.image.$item=t.cloneNode(!0):(e.image.$item=t,e.image.$itemParent=t.parentNode),e.image.useImgTag=!0,e.image.useCustomImgTag=!0),!!e.image.$item||(null===e.image.src&&(e.image.src=e.css(e.$item,"background-image").replace(/^url\(['"]?/g,"").replace(/['"]?\)$/g,"")),!(!e.image.src||"none"===e.image.src))}},{key:"canInitParallax",value:function(){return l&&!(p&&this.options.noAndroid)&&!(u&&this.options.noIos)}},{key:"init",value:function(){var e=this,t={position:"absolute",top:0,left:0,width:"100%",height:"100%",overflow:"hidden",pointerEvents:"none"},i={};if(!e.options.keepImg){var n=e.$item.getAttribute("style");if(n&&e.$item.setAttribute("data-jarallax-original-styles",n),e.image.$item&&e.image.useCustomImgTag){var o=e.image.$item.getAttribute("style");o&&e.image.$item.setAttribute("data-jarallax-original-styles",o)}}"static"===e.css(e.$item,"position")&&e.css(e.$item,{position:"relative"}),"auto"===e.css(e.$item,"z-index")&&e.css(e.$item,{zIndex:0}),e.image.$container=document.createElement("div"),e.css(e.image.$container,t),e.css(e.image.$container,{"z-index":e.options.zIndex}),e.image.$container.setAttribute("id","jarallax-container-"+e.instanceID),e.$item.appendChild(e.image.$container),e.image.useImgTag?(e.image.$item||(e.image.$item=document.createElement("img"),e.image.$item.setAttribute("src",e.image.src)),i=e.extend({"object-fit":e.options.imgSize,"font-family":"object-fit: "+e.options.imgSize+"; object-position: "+e.options.imgPosition+";","max-width":"none"},t,i)):(e.image.$item=document.createElement("div"),i=e.extend({"background-position":e.options.imgPosition,"background-size":e.options.imgSize,"background-repeat":e.options.imgRepeat,"background-image":'url("'+e.image.src+'")'},t,i));for(var a=0,r=e.$item;null!==r&&r!==document&&0===a;){var s=e.css(r,"-webkit-transform")||e.css(r,"-moz-transform")||e.css(r,"transform");s&&"none"!==s&&(a=1,e.css(e.image.$container,{transform:"translateX(0) translateY(0)"})),r=r.parentNode}(a||"opacity"===e.options.type||"scale"===e.options.type||"scale-opacity"===e.options.type||1===e.options.speed)&&(e.image.position="absolute"),i.position=e.image.position,e.css(e.image.$item,i),e.image.$container.appendChild(e.image.$item),e.coverImage(),e.clipContainer(),e.onScroll(!0),e.options.onInit&&e.options.onInit.call(e),"none"!==e.css(e.$item,"background-image")&&e.css(e.$item,{"background-image":"none"}),e.addToParallaxList()}},{key:"addToParallaxList",value:function(){x.push(this),1===x.length&&o()}},{key:"removeFromParallaxList",value:function(){var e=this;x.forEach(function(t,i){t.instanceID===e.instanceID&&x.splice(i,1)})}},{key:"destroy",value:function(){var e=this;e.removeFromParallaxList();var t=e.$item.getAttribute("data-jarallax-original-styles");if(e.$item.removeAttribute("data-jarallax-original-styles"),t?e.$item.setAttribute("style",t):e.$item.removeAttribute("style"),e.image.$item&&e.image.useCustomImgTag){var i=e.image.$item.getAttribute("data-jarallax-original-styles");e.image.$item.removeAttribute("data-jarallax-original-styles"),i?e.image.$item.setAttribute("style",t):e.image.$item.removeAttribute("style"),e.image.$itemParent&&e.image.$itemParent.appendChild(e.image.$item)}e.$clipStyles&&e.$clipStyles.parentNode.removeChild(e.$clipStyles),e.image.$container&&e.image.$container.parentNode.removeChild(e.image.$container),e.options.onDestroy&&e.options.onDestroy.call(e),delete e.$item.jarallax}},{key:"clipContainer",value:function(){if(!g&&"fixed"===this.image.position){var e=this,t=e.image.$container.getBoundingClientRect(),i=t.width,n=t.height;if(!e.$clipStyles){e.$clipStyles=document.createElement("style"),e.$clipStyles.setAttribute("type","text/css"),e.$clipStyles.setAttribute("id","jarallax-clip-"+e.instanceID);var o=document.head||document.getElementsByTagName("head")[0];o.appendChild(e.$clipStyles)}var a=["#jarallax-container-"+e.instanceID+" {"," clip: rect(0 "+i+"px "+n+"px 0);"," clip: rect(0, "+i+"px, "+n+"px, 0);","}"].join("\n");e.$clipStyles.styleSheet?e.$clipStyles.styleSheet.cssText=a:e.$clipStyles.innerHTML=a}}},{key:"coverImage",value:function(){var e=this,t=e.image.$container.getBoundingClientRect(),i=t.height,n=e.options.speed,o="scroll"===e.options.type||"scroll-opacity"===e.options.type,a=0,r=i,s=0;return o&&(a=n<0?n*Math.max(i,v):n*(i+v),n>1?r=Math.abs(a-v):n<0?r=a/n+Math.abs(a):r+=Math.abs(v-i)*(1-n),a/=2),e.parallaxScrollDistance=a,s=o?(v-r)/2:(i-r)/2,e.css(e.image.$item,{height:r+"px",marginTop:s+"px",left:"fixed"===e.image.position?t.left+"px":"0",width:t.width+"px"}),e.options.onCoverImage&&e.options.onCoverImage.call(e),{image:{height:r,marginTop:s},container:t}}},{key:"isVisible",value:function(){return this.isElementInViewport||!1}},{key:"onScroll",value:function(e){var t=this,i=t.$item.getBoundingClientRect(),n=i.top,o=i.height,a={},r=i;if(t.options.elementInViewport&&(r=t.options.elementInViewport.getBoundingClientRect()),t.isElementInViewport=r.bottom>=0&&r.right>=0&&r.top<=v&&r.left<=h,e||t.isElementInViewport){var s=Math.max(0,n),l=Math.max(0,o+n),m=Math.max(0,-n),c=Math.max(0,n+o-v),p=Math.max(0,o-(n+o-v)),u=Math.max(0,-n+v-o),d=1-2*(v-n)/(v+o),f=1;if(o<v?f=1-(m||c)/o:l<=v?f=l/v:p<=v&&(f=p/v),"opacity"!==t.options.type&&"scale-opacity"!==t.options.type&&"scroll-opacity"!==t.options.type||(a.transform="",a.opacity=f),"scale"===t.options.type||"scale-opacity"===t.options.type){var g=1;t.options.speed<0?g-=t.options.speed*f:g+=t.options.speed*(1-f),a.transform="scale("+g+")"}if("scroll"===t.options.type||"scroll-opacity"===t.options.type){var y=t.parallaxScrollDistance*d;"absolute"===t.image.position&&(y-=n),a.transform="translateY("+y+"px)"}t.css(t.image.$item,a),t.options.onScroll&&t.options.onScroll.call(t,{section:i,beforeTop:s,beforeTopEnd:l,afterTop:m,beforeBottom:c,beforeBottomEnd:p,afterBottom:u,visiblePercent:f,fromViewportCenter:d})}}},{key:"onResize",value:function(){this.coverImage(),this.clipContainer()}}]),t}(),S=function(e){("object"===("undefined"==typeof HTMLElement?"undefined":a(HTMLElement))?e instanceof HTMLElement:e&&"object"===("undefined"==typeof e?"undefined":a(e))&&null!==e&&1===e.nodeType&&"string"==typeof e.nodeName)&&(e=[e]);var t=arguments[1],i=Array.prototype.slice.call(arguments,2),n=e.length,o=0,r=void 0;for(o;o<n;o++)if("object"===("undefined"==typeof t?"undefined":a(t))||"undefined"==typeof t?e[o].jarallax||(e[o].jarallax=new j(e[o],t)):e[o].jarallax&&(r=e[o].jarallax[t].apply(e[o].jarallax,i)),"undefined"!=typeof r)return r;return e};S.constructor=j;var I=window.jarallax;if(window.jarallax=S,window.jarallax.noConflict=function(){return window.jarallax=I,this},"undefined"!=typeof jQuery){var E=function(){var e=arguments||[];Array.prototype.unshift.call(e,this);var t=S.apply(window,e);return"object"!==("undefined"==typeof t?"undefined":a(t))?t:this};E.constructor=j;var k=jQuery.fn.jarallax;jQuery.fn.jarallax=E,jQuery.fn.jarallax.noConflict=function(){return jQuery.fn.jarallax=k,this}}i(window,"DOMContentLoaded",function(){S(document.querySelectorAll("[data-jarallax]"))})}(); |
{ | ||
"name": "jarallax", | ||
"version": "1.8.0", | ||
"version": "1.9.0", | ||
"description": "Parallax plugin for background images and videos", | ||
"license": "WTFPL", | ||
"homepage": "https://github.com/nk-o/jarallax", | ||
"author": "_nK <https://nkdev.info>", | ||
"author": "nK <https://nkdev.info>", | ||
"main": "dist/jarallax.min.js", | ||
"scripts": { | ||
"build": "gulp", | ||
"watch": "gulp watch" | ||
"watch": "gulp watch", | ||
"test": "gulp test" | ||
}, | ||
@@ -29,7 +30,24 @@ "repository": { | ||
"devDependencies": { | ||
"babel-preset-env": "^1.6.0", | ||
"babel": "^6.23.0", | ||
"del": "^2.2.2", | ||
"eslint": "^4.6.0", | ||
"eslint-config-airbnb": "^15.1.0", | ||
"eslint-plugin-import": "^2.7.0", | ||
"eslint-plugin-jsx-a11y": "^5.1.1", | ||
"eslint-plugin-react": "^7.4.0", | ||
"gulp": "^3.9.1", | ||
"gulp-header": "^1.8.9", | ||
"gulp-if": "^2.0.2", | ||
"gulp-iife": "^0.3.0", | ||
"gulp-load-plugins": "^1.5.0", | ||
"gulp-babel": "^6.1.2", | ||
"gulp-rename": "^1.2.2", | ||
"gulp-uglify": "^2.0.0" | ||
"gulp-uglify": "^2.0.0", | ||
"node-qunit-phantomjs": "^1.5.0", | ||
"qunitjs": "^2.4.0", | ||
"json-file": "^0.1.0", | ||
"jquery": "^3.2.1", | ||
"object-fit-images": "^3.2.3" | ||
} | ||
} |
188
README.md
# Just Another Parallax | ||
Smooth parallax scrolling effect for background images using ***CSS transforms*** with graceful degradation for old browsers. Parallax plugin with ***NO dependencies***. jQuery supported. ***Youtube***, ***Vimeo*** and ***Local Videos*** parallax supported. | ||
Smooth parallax scrolling effect for background images, videos and inline elements. Code in pure JavaScript with ***NO dependencies*** + jQuery supported. ***Youtube***, ***Vimeo*** and ***Local Videos*** parallax supported. | ||
@@ -16,3 +16,3 @@ ## [Demo](https://free.nkdev.info/jarallax/) | ||
[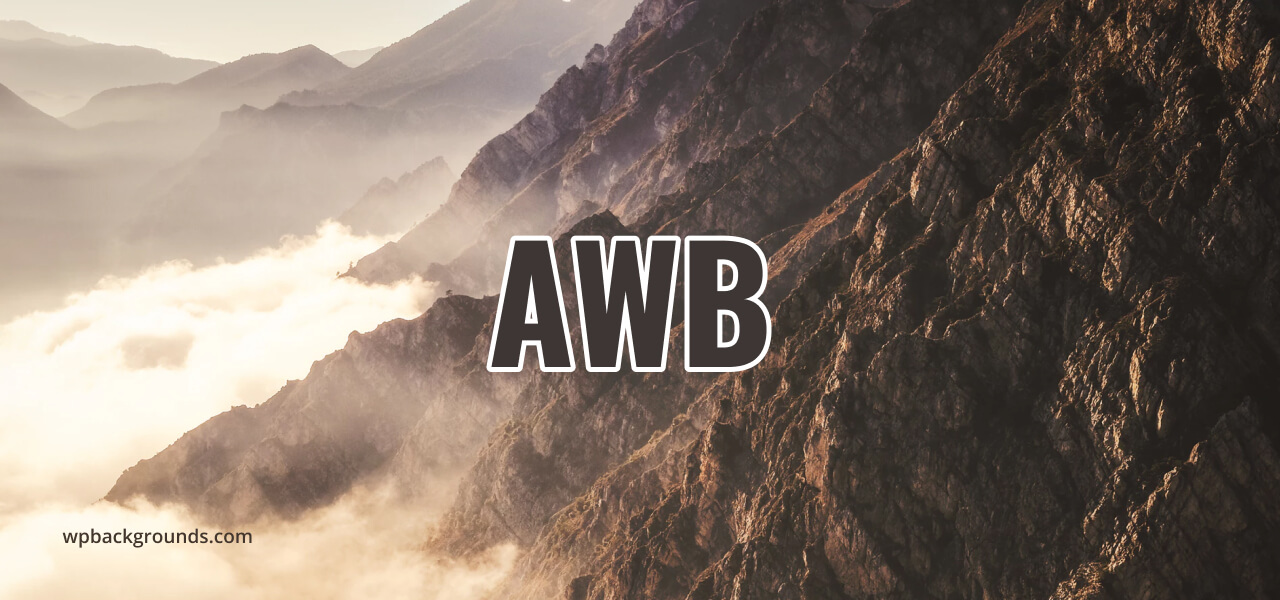](https://nkdev.info/downloads/advanced-wordpress-backgrounds/) | ||
[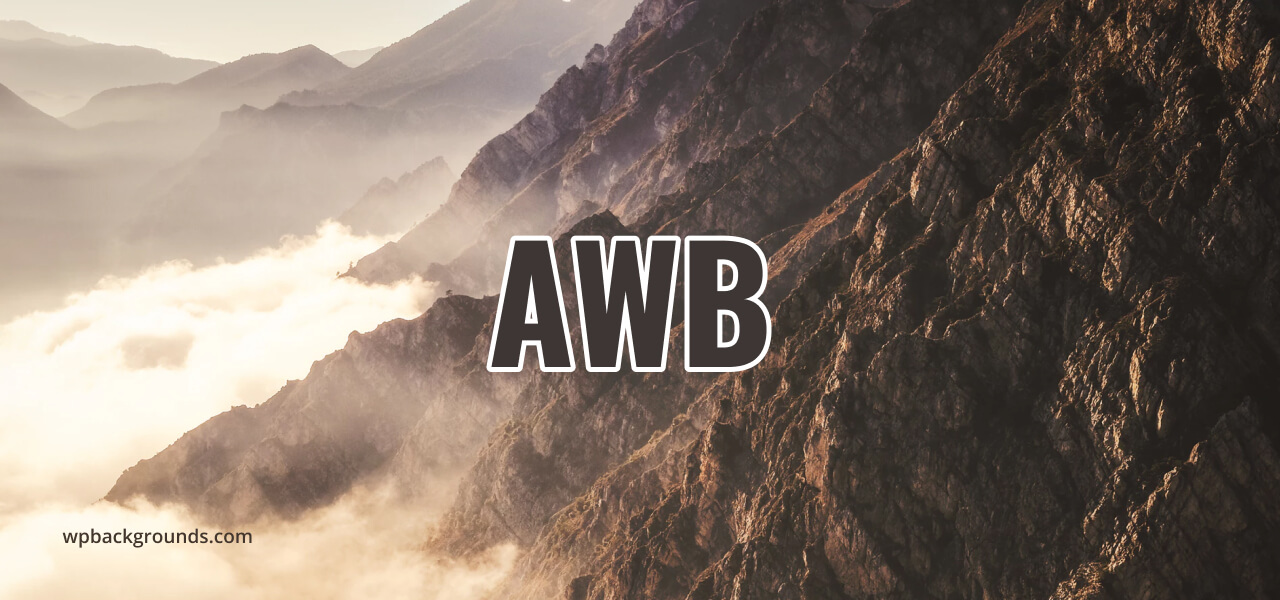](https://wordpress.org/plugins/advanced-backgrounds/) | ||
@@ -32,6 +32,9 @@ We made WordPress plugin to easily make backgrounds for content in your blog with all Jarallax features. | ||
<!-- Jarallax --> | ||
<script src='jarallax/jarallax.js'></script> | ||
<script src="jarallax/jarallax.js"></script> | ||
<!-- Include it if you want to use Video parallax --> | ||
<script src='jarallax/jarallax-video.js'></script> | ||
<script src="jarallax/jarallax-video.js"></script> | ||
<!-- Include it if you want to parallax any element --> | ||
<script src="jarallax/jarallax-element.js"></script> | ||
``` | ||
@@ -43,6 +46,9 @@ | ||
<!-- Jarallax --> | ||
<script src='https://cdnjs.cloudflare.com/ajax/libs/jarallax/1.7.3/jarallax.min.js'></script> | ||
<script src="https://cdnjs.cloudflare.com/ajax/libs/jarallax/1.9.0/jarallax.min.js"></script> | ||
<!-- Include it if you want to use Video parallax --> | ||
<script src='https://cdnjs.cloudflare.com/ajax/libs/jarallax/1.7.3/jarallax-video.min.js'></script> | ||
<script src="https://cdnjs.cloudflare.com/ajax/libs/jarallax/1.9.0/jarallax-video.min.js"></script> | ||
<!-- Include it if you want to parallax any element --> | ||
<script src="https://cdnjs.cloudflare.com/ajax/libs/jarallax/1.9.0/jarallax-element.min.js"></script> | ||
``` | ||
@@ -55,20 +61,37 @@ | ||
## Supported plugins | ||
You can add these plugins before jarallax initialize. | ||
- [object-fit-images](https://github.com/bfred-it/object-fit-images) polyfill for `object-fit` styles; | ||
- [lazysizes](https://github.com/aFarkas/lazysizes) lazy-load images with srcset support; | ||
## Set up your HTML | ||
```html | ||
<!-- Image Parallax --> | ||
<div class='jarallax' style='background-image: url(<background_image_url_here>)'> | ||
<!-- Background Image Parallax --> | ||
<div class="jarallax"> | ||
<img class="jarallax-img" src="<background_image_url_here>" alt=""> | ||
Your content here... | ||
</div> | ||
<!-- Alternate: Background Image Parallax --> | ||
<div class="jarallax" style="background-image: url('<background_image_url_here>');"> | ||
Your content here... | ||
</div> | ||
``` | ||
### Additional styles | ||
Mandatory requirement for plugin works properly - the selected item should be NOT position: static (for ex. relative). | ||
This styles need to add relative position and correct background image position before Jarallax initialize end. | ||
These styles need to correct background image position before Jarallax initialized: | ||
```css | ||
.jarallax { | ||
background-size: cover; | ||
background-repeat: no-repeat; | ||
background-position: 50% 50%; | ||
position: relative; | ||
z-index: 0; | ||
} | ||
.jarallax > .jarallax-img { | ||
position: absolute; | ||
object-fit: cover; | ||
top: 0; | ||
left: 0; | ||
width: 100%; | ||
height: 100%; | ||
z-index: -1; | ||
} | ||
``` | ||
@@ -80,3 +103,4 @@ | ||
```html | ||
<div data-jarallax='{"speed": 0.2}' class='jarallax' style='background-image: url(<background_image_url_here>)'> | ||
<div data-jarallax data-speed="0.2" class="jarallax"> | ||
<img class="jarallax-img" src="<background_image_url_here>" alt=""> | ||
Your content here... | ||
@@ -100,16 +124,16 @@ </div> | ||
## Video Usage Examples | ||
## Background Video Usage Examples | ||
```html | ||
<!-- YouTube Parallax --> | ||
<div class='jarallax' data-jarallax-video='https://www.youtube.com/watch?v=ab0TSkLe-E0'> | ||
<!-- Background YouTube Parallax --> | ||
<div class="jarallax" data-jarallax-video="https://www.youtube.com/watch?v=ab0TSkLe-E0"> | ||
Your content here... | ||
</div> | ||
<!-- Vimeo Parallax --> | ||
<div class='jarallax' data-jarallax-video='https://vimeo.com/110138539'> | ||
<!-- Background Vimeo Parallax --> | ||
<div class="jarallax" data-jarallax-video="https://vimeo.com/110138539"> | ||
Your content here... | ||
</div> | ||
<!-- Local Video Parallax --> | ||
<div class='jarallax' data-jarallax-video='mp4:./video/local-video.mp4,webm:./video/local-video.webm,ogv:./video/local-video.ogv'> | ||
<!-- Background Local Video Parallax --> | ||
<div class="jarallax" data-jarallax-video="mp4:./video/local-video.mp4,webm:./video/local-video.webm,ogv:./video/local-video.ogv"> | ||
Your content here... | ||
@@ -120,2 +144,16 @@ </div> | ||
## Any Element Prallax Usage Examples | ||
```html | ||
<!-- Element will be parallaxed on inverted 140 pixels from the screen center --> | ||
<div data-jarallax-element="-140"> | ||
Your content here... | ||
</div> | ||
<!-- Element will be parallaxed on 250 pixels from the screen center --> | ||
<div data-jarallax-element="250"> | ||
Your content here... | ||
</div> | ||
``` | ||
Note: this is more like experimental feature, so the behavior could be changed in the future releases. | ||
# Options | ||
@@ -138,3 +176,3 @@ Options can be passed in data attributes or in object when you initialize jarallax from script. | ||
<td>scroll</td> | ||
<td>scroll, scale, opacity, scroll-opacity, scale-opacity</td> | ||
<td>scroll, scale, opacity, scroll-opacity, scale-opacity.</td> | ||
</tr> | ||
@@ -145,3 +183,3 @@ <tr> | ||
<td>0.5</td> | ||
<td>Parallax effect speed. Provide numbers from -1.0 to 2.0</td> | ||
<td>Parallax effect speed. Provide numbers from -1.0 to 2.0.</td> | ||
</tr> | ||
@@ -152,20 +190,39 @@ <tr> | ||
<td>null</td> | ||
<td>Image path. By default used image from background.</td> | ||
<td>Image url. By default used image from background.</td> | ||
</tr> | ||
<tr> | ||
<td>imgWidth</td> | ||
<td>number</td> | ||
<td>null</td> | ||
<td rowspan='2'>You can provide the natural width and natural height of an image to speed up loading.</td> | ||
<td>imgElement</td> | ||
<td>dom / selector</td> | ||
<td>.jarallax-img</td> | ||
<td>Image tag that will be used as background.</td> | ||
</tr> | ||
<tr> | ||
<td>imgHeight</td> | ||
<td>number</td> | ||
<td>null</td> | ||
<td>imgSize</td> | ||
<td>string</td> | ||
<td>cover</td> | ||
<td>Image size. If you use <code><img></code> tag for background, you should add <code>object-fit</code> values, else use <code>background-size</code> values.</td> | ||
</tr> | ||
<tr> | ||
<td>imgPosition</td> | ||
<td>string</td> | ||
<td>50% 50%</td> | ||
<td>Image position. If you use <code><img></code> tag for background, you should add <code>object-position</code> values, else use <code>background-position</code> values.</td> | ||
</tr> | ||
<tr> | ||
<td>imgRepeat</td> | ||
<td>string</td> | ||
<td>no-repeat</td> | ||
<td>Image repeat. Supported only <code>background-position</code> values.</td> | ||
</tr> | ||
<tr> | ||
<td>keepImg</td> | ||
<td>boolean</td> | ||
<td>false</td> | ||
<td>Kepp <code><img></code> tag in it's default place after Jarallax inited.</td> | ||
</tr> | ||
<tr> | ||
<td>elementInViewport</td> | ||
<td>dom</td> | ||
<td>null</td> | ||
<td>Use custom DOM / jQuery element to check if parallax block in viewport. More info here - <a href="https://github.com/nk-o/jarallax/issues/13">Issue 13</a></td> | ||
<td>Use custom DOM / jQuery element to check if parallax block in viewport. More info here - <a href="https://github.com/nk-o/jarallax/issues/13">Issue 13</a>.</td> | ||
</tr> | ||
@@ -187,4 +244,4 @@ <tr> | ||
<td>boolean</td> | ||
<td>true</td> | ||
<td>Disable parallax on iOs devices. Jarallax disabled by default on iOs because of strong lags ;(</td> | ||
<td>false</td> | ||
<td>Disable parallax on iOs devices.</td> | ||
</tr> | ||
@@ -194,3 +251,3 @@ </tbody> | ||
### Options For Video | ||
### Options For Video (+ supported all default options) | ||
Required `jarallax/jarallax-video.js` file. | ||
@@ -212,3 +269,3 @@ | ||
<td>null</td> | ||
<td>You can use Youtube, Vimeo or local videos. Also you can use data attribute <code>data-jarallax-video</code></td> | ||
<td>You can use Youtube, Vimeo or local videos. Also you can use data attribute <code>data-jarallax-video</code>.</td> | ||
</tr> | ||
@@ -219,3 +276,3 @@ <tr> | ||
<td>0</td> | ||
<td>Start time in seconds when video will be started (this value will be applied also after loop)</td> | ||
<td>Start time in seconds when video will be started (this value will be applied also after loop).</td> | ||
</tr> | ||
@@ -226,7 +283,47 @@ <tr> | ||
<td>0</td> | ||
<td>End time in seconds when video will be ended</td> | ||
<td>End time in seconds when video will be ended.</td> | ||
</tr> | ||
<tr> | ||
<td>videoVolume</td> | ||
<td>float</td> | ||
<td>0</td> | ||
<td>Video volume from 0 to 100.</td> | ||
</tr> | ||
<tr> | ||
<td>videoPlayOnlyVisible</td> | ||
<td>boolean</td> | ||
<td>true</td> | ||
<td>Play video only when it is visible on the screen.</td> | ||
</tr> | ||
</tbody> | ||
</table> | ||
### Options For Element Parallax | ||
Required `jarallax/jarallax-element.js` file. | ||
<table class='table table-bordered table-striped'> | ||
<thead> | ||
<tr> | ||
<th>name</th> | ||
<th>type</th> | ||
<th>default</th> | ||
<th style='width: 60%;'>description</th> | ||
</tr> | ||
</thead> | ||
<tbody> | ||
<tr> | ||
<td>type</td> | ||
<td>string</td> | ||
<td>element</td> | ||
<td>Will only work with <code>element</code> value.</td> | ||
</tr> | ||
<tr> | ||
<td>speed</td> | ||
<td>float</td> | ||
<td>0</td> | ||
<td>Parallax distance in pixels. Supported any value. Also you can use data attribute <code>data-jarallax-element</code>.</td> | ||
</tr> | ||
</tbody> | ||
</table> | ||
# Events | ||
@@ -319,12 +416,7 @@ Evenets used the same way as Options. | ||
<tr> | ||
<td>clipContainer</td> | ||
<td>onResize</td> | ||
<td>-</td> | ||
<td>Clip parallax container. Called on window resize and load.</td> | ||
<td>Fit image and clip parallax container. Called on window resize and load.</td> | ||
</tr> | ||
<tr> | ||
<td>coverImage</td> | ||
<td>-</td> | ||
<td>Fit image in parallax container. Called on window resize and load.</td> | ||
</tr> | ||
<tr> | ||
<td>onScroll</td> | ||
@@ -368,6 +460,6 @@ <td>-</td> | ||
# Credits | ||
Images https://www.pexels.com/ | ||
Local Video https://www.videezy.com/ | ||
Images https://unsplash.com/ | ||
Local Video https://videos.pexels.com/ | ||
# License | ||
Copyright (c) 2017 nK Licensed under the WTFPL license. |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
54192050
41
3943
449
21
4