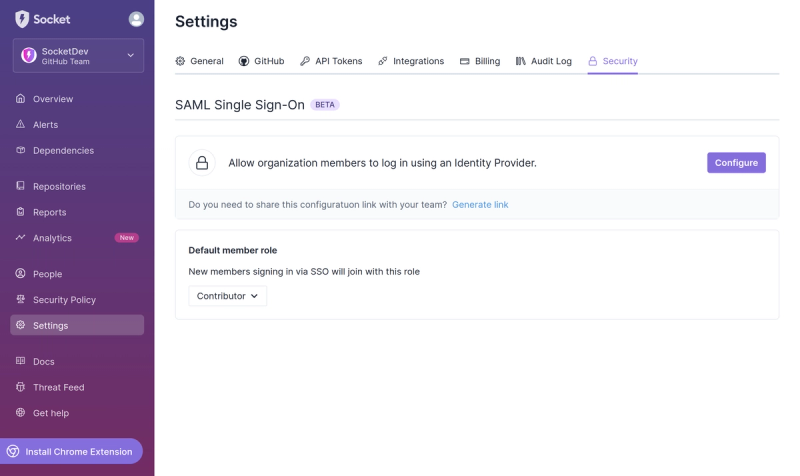
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
javascript-algorithms
Advanced tools
Readme
_______
__ __ \______ ________________
_ / / /_ __ __ \ _ \_ ___/
/ /_/ /_ / / / / / __/ /
\____/ /_/ /_/ /_/\___//_/
________ _____
___ __ \___________ ______(_)__________________ _______
__ / / / _ \_ __ __ \_ /__ ___/ ___/ __ /_ __ \
_ /_/ // __/ / / / / / / _ / / /__ / /_/ /_ / / /
/_____/ \___//_/ /_/ /_//_/ /_/ \___/ \__,_/ /_/ /_/
#Install $npm install javascript-algorithms
##Binary Search Tree
var bst = new js_algorithms.BinarySearchTree();
bst.insert({x: 4}, function (a, b) {
return a.x > b.x;//if you want add less value to left brench, use '>' operator,
});
bst.getMax();
bst.getMin();
bst.find(23, function (a) {
return a.x;
});
bst.remove(function(a){
return a.x == 23;
});
##Doubly Linked List
var list = new js_algorithms.DoublyLinkedList();
list.insert({name:"omer",surname:"demircan"});
list.find(function(a){
return a.name == "omer";
});
list.remove(function(a){
return a.surname == "çevik";
});
##Hash
var hash = new js_algorithms.Hash({size: 137});
hash.put({name: "omer"});
hash.get({name: "omer"})
##Linked List
var llist = new js_algorithms.LinkedList();
llist.insert({name: "omer"});
llist.find(function(a){
return a.name == "omer";
});
llist.remove(function(a){
return a.name == "pamir";
});
##Graphs
var g = new js_algorithms.Graph(GRAPH_SIZE);
g.addEdge("a", "b", 3);
g.addEdge("a", "c", 5);
g.addEdge("b", "e", 1);
var p = g.dijkstra("a","e"); //Output : [a,b,e]
g.clearVisitedList();
var g.depthFirstSearch(START_NODE);
g.clearVisitedList();//clear before visited list (set to false all of them)
var g.breadthFirstSearch(START_NODE);
##Sort Algorithms
var s = new js_algorithms.Sorting();
//select any arr variable
var arr = s.CreateRandomArray(11).data; //[,4,6,7,3,66,3]
var arr = [{x:4,y:6},{x:2,y:7},{x:66,y:3},{x:14,y:0},{x:6,y:5},{x:2,y:5}];
s.selectionSorting(arr, function(a, b){
return a.x < b.x;
});
s.insertionSort(arr, function(a, b){
return a.x < b.x;
});
s.shellSort(arr, function(a, b){
return a.x < b.x;
});
//only mergeSort callback is different from other
s.mergeSort(arr, function(a){
return a.x;
});
s.quickSort(arr, function(a, b){
return a.x < b.x
});
##Huffman
var huffman = new js_algorithms.Huffman("omer demircan");
huffman.encode();//returned : 1011101100...
FAQs
algorithms for javascript
The npm package javascript-algorithms receives a total of 4 weekly downloads. As such, javascript-algorithms popularity was classified as not popular.
We found that javascript-algorithms demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.