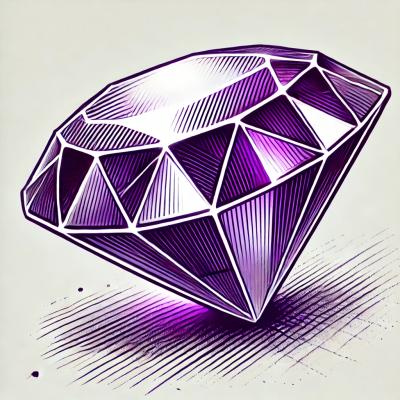
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
javascript-state-machine
Advanced tools
The `javascript-state-machine` npm package is a lightweight, flexible, and powerful state machine library for JavaScript. It allows you to define states and transitions in a clear and concise manner, making it easier to manage complex state logic in your applications.
Basic State Machine
This code demonstrates how to create a basic state machine with initial state 'solid' and transitions between 'solid', 'liquid', and 'gas' states.
const StateMachine = require('javascript-state-machine');
const fsm = new StateMachine({
init: 'solid',
transitions: [
{ name: 'melt', from: 'solid', to: 'liquid' },
{ name: 'freeze', from: 'liquid', to: 'solid' },
{ name: 'vaporize', from: 'liquid', to: 'gas' },
{ name: 'condense', from: 'gas', to: 'liquid' }
]
});
console.log(fsm.state); // 'solid'
fsm.melt();
console.log(fsm.state); // 'liquid'
Callbacks
This code demonstrates how to add callbacks to state transitions. The `onMelt` and `onFreeze` methods are called when the respective transitions occur.
const StateMachine = require('javascript-state-machine');
const fsm = new StateMachine({
init: 'solid',
transitions: [
{ name: 'melt', from: 'solid', to: 'liquid' },
{ name: 'freeze', from: 'liquid', to: 'solid' }
],
methods: {
onMelt: function() { console.log('I melted'); },
onFreeze: function() { console.log('I froze'); }
}
});
fsm.melt(); // 'I melted'
fsm.freeze(); // 'I froze'
State Machine with Data
This code demonstrates how to create a state machine with additional data. The `food` property is accessible within the state machine methods.
const StateMachine = require('javascript-state-machine');
const fsm = new StateMachine({
init: 'hungry',
transitions: [
{ name: 'eat', from: 'hungry', to: 'full' }
],
data: { food: 'pizza' },
methods: {
onEat: function() { console.log(`Eating ${this.food}`); }
}
});
fsm.eat(); // 'Eating pizza'
XState is a more feature-rich and robust state machine library that supports hierarchical state machines, parallel states, and statecharts. It is more suitable for complex state management needs and offers extensive tooling and visualization options.
Machina is another state machine library that provides a more comprehensive set of features, including hierarchical states, event emitters, and a more declarative API. It is designed for more complex state management scenarios.
FSM-as-Promised is a state machine library that integrates with JavaScript promises, making it easier to handle asynchronous state transitions. It is useful for applications that require asynchronous operations within state transitions.
A library for finite state machines.
VERSION 3.0 Is a significant rewrite from earlier versions. Existing 2.x users should be sure to read the Upgrade Guide.
In a browser:
<script src='state-machine.js'></script>
after downloading the source or the minified version
Using npm:
npm install --save-dev javascript-state-machine
In Node.js:
var StateMachine = require('javascript-state-machine');
A state machine can be constructed using:
var fsm = new StateMachine({
init: 'solid',
transitions: [
{ name: 'melt', from: 'solid', to: 'liquid' },
{ name: 'freeze', from: 'liquid', to: 'solid' },
{ name: 'vaporize', from: 'liquid', to: 'gas' },
{ name: 'condense', from: 'gas', to: 'liquid' }
],
methods: {
onMelt: function() { console.log('I melted') },
onFreeze: function() { console.log('I froze') },
onVaporize: function() { console.log('I vaporized') },
onCondense: function() { console.log('I condensed') }
}
});
... which creates an object with a current state property:
fsm.state
... methods to transition to a different state:
fsm.melt()
fsm.freeze()
fsm.vaporize()
fsm.condense()
... observer methods called automatically during the lifecycle of a transition:
onMelt()
onFreeze()
onVaporize()
onCondense()
... along with the following helper methods:
fsm.is(s)
- return true if state s
is the current statefsm.can(t)
- return true if transition t
can occur from the current statefsm.cannot(t)
- return true if transition t
cannot occur from the current statefsm.transitions()
- return list of transitions that are allowed from the current statefsm.allTransitions()
- return list of all possible transitionsfsm.allStates()
- return list of all possible statesA state machine consists of a set of States
A state machine changes state by using Transitions
A state machine can perform actions during a transition by observing Lifecycle Events
A state machine can also have arbitrary Data and Methods.
Multiple instances of a state machine can be created using a State Machine Factory.
Read more about
You can Contribute to this project with issues or pull requests.
See RELEASE NOTES file.
See MIT LICENSE file.
If you have any ideas, feedback, requests or bug reports, you can reach me at jake@codeincomplete.com, or via my website: Code inComplete
FAQs
A finite state machine library
We found that javascript-state-machine demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.