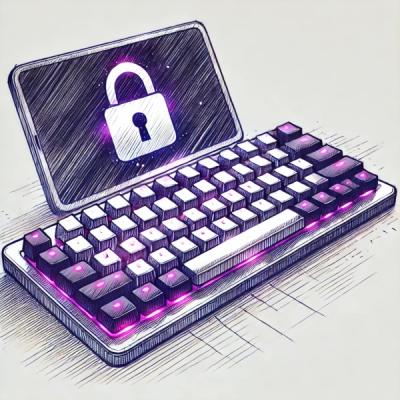
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
jest-each
Advanced tools
Package description
The jest-each npm package is an extension for Jest, a popular JavaScript testing framework. It allows you to run a single test multiple times with different sets of data, making it easier to test functions with various inputs and expected outputs.
Parameterized Tests
This feature allows you to run the same test with different sets of data. In this example, the test checks if the sum of two numbers equals the expected result for multiple sets of inputs.
const each = require('jest-each').default;
each([ [1, 1, 2], [1, 2, 3], [2, 1, 3] ]).test('adds %d and %d to equal %d', (a, b, expected) => {
expect(a + b).toBe(expected);
});
Table-Driven Tests
This feature allows you to define test cases in a tabular format, making it easier to read and manage multiple test cases. The example demonstrates how to use a table to test the addition of two numbers.
const each = require('jest-each').default;
each`
a | b | expected
${1} | ${1} | ${2}
${1} | ${2} | ${3}
${2} | ${1} | ${3}
`.test('adds $a and $b to equal $expected', ({ a, b, expected }) => {
expect(a + b).toBe(expected);
});
Mocha is a feature-rich JavaScript test framework running on Node.js and in the browser. It allows for flexible and accurate reporting, while mapping uncaught exceptions to the correct test cases. Mocha can be used with various assertion libraries, and while it doesn't have built-in support for parameterized tests like jest-each, it can achieve similar functionality with the help of additional libraries like 'mocha-each'.
AVA is a test runner for Node.js with a concise API, detailed error output, and process isolation. It supports running tests concurrently, which can lead to faster test execution. AVA supports parameterized tests through its 'test.each' method, similar to jest-each, making it a good alternative for those looking for a minimalistic and fast testing framework.
Tape is a minimalist JavaScript test runner that works in both Node.js and the browser. It does not have built-in support for parameterized tests, but you can achieve similar functionality by writing loops within your test cases. Tape is known for its simplicity and ease of use, making it a good choice for smaller projects or those who prefer a lightweight testing solution.
Changelog
0.5.0
--noStackTrace
option to disable stack traces.Readme
A parameterised testing library for Jest inspired by mocha-each.
jest-each allows you to provide multiple arguments to your test
/describe
which results in the test/suite being run once per row of parameters.
.test
to runs multiple tests with parameterised data
.it
.test.only
to only run the parameterised tests
.it.only
or .fit
.test.skip
to skip the parameterised tests
.it.skip
or .xit
or .xtest
.describe
to runs test suites with parameterised data.describe.only
to only run the parameterised suite of tests
.fdescribe
.describe.skip
to skip the parameterised suite of tests
.xdescribe
done
.test
.test
with Tagged Template Literals
.describe
npm i --save-dev jest-each
yarn add -D jest-each
jest-each is a default export so it can be imported with whatever name you like.
// es6
import each from 'jest-each';
// es5
const each = require('jest-each');
each([parameters]).test(name, testFn)
each
:Array
of Arrays with the arguments that are passed into the testFn
for each row.test
:String
the title of the test
, use %s
in the name string to positionally inject parameter values into the test titleFunction
the test logic, this is the function that will receive the parameters of each row as function argumentseach([parameters]).describe(name, suiteFn)
each
:Array
of Arrays with the arguments that are passed into the suiteFn
for each row.describe
:String
the title of the describe
, use %s
in the name string to positionally inject parameter values into the suite titleFunction
the suite of test
/it
s to be ran, this is the function that will receive the parameters in each row as function arguments.test(name, fn)
Alias: .it(name, fn)
each([
[1, 1, 2],
[1, 2, 3],
[2, 1, 3],
]).test('returns the result of adding %s to %s', (a, b, expected) => {
expect(a + b).toBe(expected);
});
.test.only(name, fn)
Aliases: .it.only(name, fn)
or .fit(name, fn)
each([
[1, 1, 2],
[1, 2, 3],
[2, 1, 3],
]).test.only('returns the result of adding %s to %s', (a, b, expected) => {
expect(a + b).toBe(expected);
});
.test.skip(name, fn)
Aliases: .it.skip(name, fn)
or .xit(name, fn)
or .xtest(name, fn)
each([
[1, 1, 2]
[1, 2, 3],
[2, 1, 3],
]).test.skip('returns the result of adding %s to %s', (a, b, expected) => {
expect(a + b).toBe(expected);
});
.test(name, fn(done))
Alias: .it(name, fn(done))
each([
['hello'],
['mr'],
['spy'],
]).test('gives 007 secret message ', (str, done) => {
const asynchronousSpy = (message) => {
expect(message).toBe(str);
done();
};
callSomeAsynchronousFunction(asynchronousSpy)(str);
});
.describe(name, fn)
each([
[1, 1, 2],
[1, 2, 3],
[2, 1, 3],
]).describe('.add(%s, %s)', (a, b, expected) => {
test(`returns ${expected}`, () => {
expect(a + b).toBe(expected);
});
test('does not mutate first arg', () => {
a + b;
expect(a).toBe(a);
});
test('does not mutate second arg', () => {
a + b;
expect(b).toBe(b);
});
});
.describe.only(name, fn)
Aliases: .fdescribe(name, fn)
each([
[1, 1, 2],
[1, 2, 3],
[2, 1, 3],
]).describe.only('.add(%s, %s)', (a, b, expected) => {
test(`returns ${expected}`, () => {
expect(a + b).toBe(expected);
});
});
.describe.skip(name, fn)
Aliases: .xdescribe(name, fn)
each([
[1, 1, 2],
[1, 2, 3],
[2, 1, 3],
]).describe.skip('.add(%s, %s)', (a, b, expected) => {
test(`returns ${expected}`, () => {
expect(a + b).toBe(expected);
});
});
each[tagged template].test(name, suiteFn)
each`
a | b | expected
${1} | ${1} | ${2}
${1} | ${2} | ${3}
${2} | ${1} | ${3}
`.test('returns $expected when adding $a to $b', ({ a, b, expected }) => {
expect(a + b).toBe(expected);
});
each
takes a tagged template string with:|
${value}
syntax..test
:String
the title of the test
, use $variable
in the name string to inject test values into the test title from the tagged template expressionsFunction
the test logic, this is the function that will receive the parameters of each row as function argumentseach[tagged template].describe(name, suiteFn)
each`
a | b | expected
${1} | ${1} | ${2}
${1} | ${2} | ${3}
${2} | ${1} | ${3}
`.describe('$a + $b', ({ a, b, expected }) => {
test(`returns ${expected}`, () => {
expect(a + b).toBe(expected);
});
test('does not mutate first arg', () => {
a + b;
expect(a).toBe(a);
});
test('does not mutate second arg', () => {
a + b;
expect(b).toBe(b);
});
});
each
takes a tagged template string with:|
${value}
syntax..describe
:String
the title of the test
, use $variable
in the name string to inject test values into the test title from the tagged template expressionsFunction
the suite of test
/it
s to be ran, this is the function that will receive the parameters in each row as function arguments.test(name, fn)
Alias: .it(name, fn)
each`
a | b | expected
${1} | ${1} | ${2}
${1} | ${2} | ${3}
${2} | ${1} | ${3}
`.test('returns $expected when adding $a to $b', ({ a, b, expected }) => {
expect(a + b).toBe(expected);
});
.test.only(name, fn)
Aliases: .it.only(name, fn)
or .fit(name, fn)
each`
a | b | expected
${1} | ${1} | ${2}
${1} | ${2} | ${3}
${2} | ${1} | ${3}
`.test.only('returns $expected when adding $a to $b', ({ a, b, expected }) => {
expect(a + b).toBe(expected);
});
.test.skip(name, fn)
Aliases: .it.skip(name, fn)
or .xit(name, fn)
or .xtest(name, fn)
each`
a | b | expected
${1} | ${1} | ${2}
${1} | ${2} | ${3}
${2} | ${1} | ${3}
`.test.skip('returns $expected when adding $a to $b', ({ a, b, expected }) => {
expect(a + b).toBe(expected);
});
.test(name, fn(done))
Alias: .it(name, fn(done))
each`
str
${'hello'}
${'mr'}
${'spy'}
`.test('gives 007 secret message: $str', ({ str }, done) => {
const asynchronousSpy = (message) => {
expect(message).toBe(str);
done();
};
callSomeAsynchronousFunction(asynchronousSpy)(str);
});
.describe(name, fn)
each`
a | b | expected
${1} | ${1} | ${2}
${1} | ${2} | ${3}
${2} | ${1} | ${3}
`.describe('$a + $b', ({ a, b, expected }) => {
test(`returns ${expected}`, () => {
expect(a + b).toBe(expected);
});
test('does not mutate first arg', () => {
a + b;
expect(a).toBe(a);
});
test('does not mutate second arg', () => {
a + b;
expect(b).toBe(b);
});
});
.describe.only(name, fn)
Aliases: .fdescribe(name, fn)
each`
a | b | expected
${1} | ${1} | ${2}
${1} | ${2} | ${3}
${2} | ${1} | ${3}
`.describe.only('$a + $b', ({ a, b, expected }) => {
test(`returns ${expected}`, () => {
expect(a + b).toBe(expected);
});
});
.describe.skip(name, fn)
Aliases: .xdescribe(name, fn)
each`
a | b | expected
${1} | ${1} | ${2}
${1} | ${2} | ${3}
${2} | ${1} | ${3}
`.describe.skip('$a + $b', ({ a, b, expected }) => {
test(`returns ${expected}`, () => {
expect(a + b).toBe(expected);
});
});
MIT
FAQs
Unknown package
We found that jest-each demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.