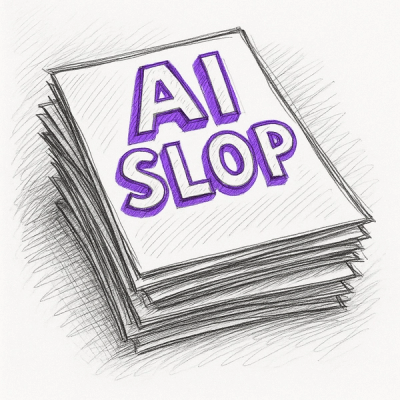
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
The js-sha1 npm package is a JavaScript implementation of the SHA-1 hash function. It allows you to generate SHA-1 hashes from strings, arrays, and array buffers. This package is useful for cryptographic operations, data integrity checks, and other scenarios where SHA-1 hashing is required.
Hashing a String
This feature allows you to generate a SHA-1 hash from a string. The code sample demonstrates how to hash the string 'Hello, World!' and print the resulting hash.
const sha1 = require('js-sha1');
const hash = sha1('Hello, World!');
console.log(hash); // '943a702d06f34599aee1f8da8ef9f7296031d699'
Hashing an Array
This feature allows you to generate a SHA-1 hash from an array of bytes. The code sample demonstrates how to hash the array [0x61, 0x62, 0x63] and print the resulting hash.
const sha1 = require('js-sha1');
const hash = sha1([0x61, 0x62, 0x63]);
console.log(hash); // 'a9993e364706816aba3e25717850c26c9cd0d89d'
Hashing an ArrayBuffer
This feature allows you to generate a SHA-1 hash from an ArrayBuffer. The code sample demonstrates how to hash an ArrayBuffer containing the bytes [0x61, 0x62, 0x63] and print the resulting hash.
const sha1 = require('js-sha1');
const buffer = new ArrayBuffer(3);
const view = new Uint8Array(buffer);
view[0] = 0x61;
view[1] = 0x62;
view[2] = 0x63;
const hash = sha1(buffer);
console.log(hash); // 'a9993e364706816aba3e25717850c26c9cd0d89d'
CryptoJS is a widely-used library that provides a variety of cryptographic algorithms, including SHA-1, SHA-256, MD5, and more. It offers a more comprehensive set of cryptographic functions compared to js-sha1, making it suitable for applications that require multiple types of hashing and encryption.
Hash.js is a library that provides a variety of hash functions, including SHA-1, SHA-256, and SHA-512. It is similar to js-sha1 in that it focuses on hashing, but it offers a broader range of hash functions, making it more versatile for different cryptographic needs.
Sha.js is a simple library that provides SHA-1, SHA-224, SHA-256, SHA-384, and SHA-512 hash functions. It is similar to js-sha1 but offers additional SHA variants, making it a good choice for applications that require different SHA algorithms.
A simple SHA1 hash function for JavaScript supports UTF-8 encoding.
For node.js, you can use this command to install:
npm install js-sha1
If you use node.js, you should require the module first:
sha1 = require('js-sha1');
And you could use like this:
sha1('Message to hash');
Code
sha1('');
sha1('The quick brown fox jumps over the lazy dog');
sha1('The quick brown fox jumps over the lazy dog.');
Output
da39a3ee5e6b4b0d3255bfef95601890afd80709
2fd4e1c67a2d28fced849ee1bb76e7391b93eb12
408d94384216f890ff7a0c3528e8bed1e0b01621
It also supports UTF-8 encoding:
Code
sha1('δΈζ');
Output
7be2d2d20c106eee0836c9bc2b939890a78e8fb3
You can open tests/index.html
in browser or use node.js to run test
node tests/node-test.js
or
npm test
If you prefer jQuery style, you can add following code to add a jQuery extension.
Code
jQuery.sha1 = sha1
And then you could use like this:
$.sha1('message');
If you prefer prototype style, you can add following code to add a prototype extension.
Code
String.prototype.sha1 = function() {
return sha1(this);
};
And then you could use like this:
'message'.sha1();
The project is released under the MIT license.
The project's website is located at https://github.com/emn178/js-sha1
Author: emn178@gmail.com
FAQs
A simple SHA1 hash function for JavaScript supports UTF-8 encoding.
The npm package js-sha1 receives a total of 242,350 weekly downloads. As such, js-sha1 popularity was classified as popular.
We found that js-sha1 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.