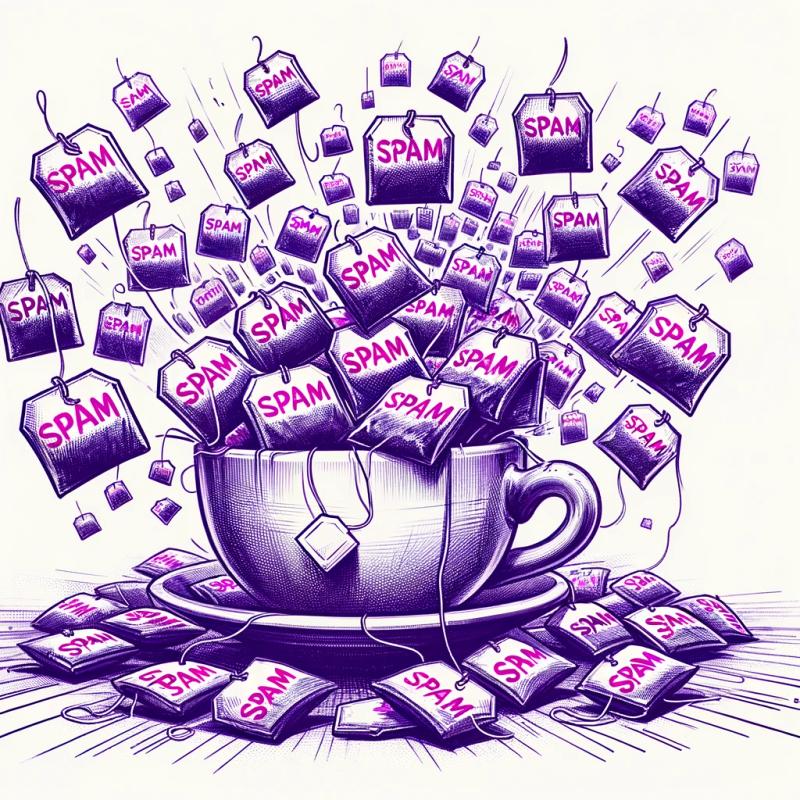
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
json-case-convertor
Advanced tools
Readme
JsonCaseConvertor can handle literraly any json object for case convertion.
Connect with author : [https://www.linkedin.com/in/mir-taha-a40bb270/]
npm install json-case-convertor
Import:
const jcc = require('json-case-convertor')
Convert only object KEYS Names:
const jsonData = {
"firstName": "John",
"lastName": "Wick",
"car": "Ford Mustang",
"car2": null,
"prize": 2000,
"other" : ['sample1', 'sample2'],
"other2" : {
"location": "America",
"longitude1": 23.4,
"latitude1" : 23.11
}
}
jcc.snakeCaseKeys(jsonData); //Convert all the keys of object to snake case
Output:
{
first_name: 'John',
last_name: 'Wick',
car: 'Ford Mustang',
car_2: null,
prize: 2000,
other: [ 'sample1', 'sample2' ],
other_2: { location: 'America', longitude_1: 23.4, latitude_1: 23.11 }
}
Convert only object values:
jcc.snakeCaseValues(jsonData) //Convert all the values of object to snake case
Output:
{
firstName: 'john',
lastName: 'wick',
car: 'ford_mustang',
car2: null,
prize: 2000,
other: [ 'sample_1', 'sample_2' ],
other2: { location: 'america', longitude1: 23.4, latitude1: 23.11 }
}
Convert only object keys and values:
jcc.snakeCaseAll(jsonData) //Convert all the values and keys of object to snake case
Output:
{
first_name: 'john',
last_name: 'wick',
car: 'ford_mustang',
car_2: null,
prize: 2000,
other: [ 'sample_1', 'sample_2' ],
other_2: { location: 'america', longitude_1: 23.4, latitude_1: 23.11 }
}
const jcc = require('json-case-convertor')
jcc.pascalCaseKeys(jsonData)
jcc.camelCaseKeys(jsonData)
jcc.snakeCaseKeys(jsonData)
jcc.kebabCaseKeys(jsonData)
jcc.upperCaseKeys(jsonData)
jcc.lowerCaseKeys(jsonData)
jcc.constantCaseKeys(jsonData)
jcc.dotCaseKeys(jsonData)
jcc.pathCaseKeys(jsonData)
jcc.sentenceCaseKeys(jsonData)
jcc.titleCaseKeys(jsonData)
//-------------------------
jcc.pascalCaseAll(jsonData)
jcc.camelCaseAll(jsonData)
jcc.snakeCaseAll(jsonData)
jcc.kebabCaseAll(jsonData)
jcc.upperCaseAll(jsonData)
jcc.lowerCaseAll(jsonData)
jcc.constantCaseAll(jsonData)
jcc.dotCaseAll(jsonData)
jcc.pathCaseAll(jsonData)
jcc.sentenceCaseAll(jsonData)
jcc.titleCaseAll(jsonData)
//----------------------------
jcc.pascalCaseValues(jsonData)
jcc.camelCaseValues(jsonData)
jcc.snakeCaseValues(jsonData)
jcc.kebabCaseValues(jsonData)
jcc.upperCaseValues(jsonData)
jcc.lowerCaseValues(jsonData)
jcc.constantCaseValues(jsonData)
jcc.dotCaseValues(jsonData)
jcc.pathCaseValues(jsonData)
jcc.sentenceCaseValues(jsonData)
jcc.titleCaseValues(jsonData)
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
Please make sure to update tests as appropriate.
ISC
FAQs
JsonCaseConvertor can handle literraly any json object for case convertion. ## Features
The npm package json-case-convertor receives a total of 477 weekly downloads. As such, json-case-convertor popularity was classified as not popular.
We found that json-case-convertor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.