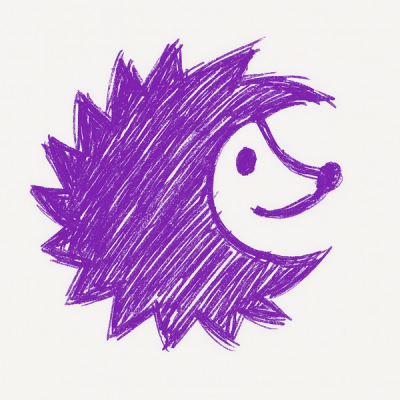
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
json-fetch
Advanced tools
A wrapper around ES6 fetch to simplify interacting with JSON APIs.
Content-Type
: application/json
headeryarn add json-fetch
# or...
npm install json-fetch
import jsonFetch from 'json-fetch';
jsonFetch('http://www.test.com/products/1234', {
body: {name: 'apple'}, // content to be JSON stringified
credentials: 'omit', // "include" by default
expectedStatuses: [201], // rejects "FetchUnexpectedStatusError" on unexpected status (optional)
// supports all normal json-fetch options:
method: 'POST',
})
.then((response) => {
// handle response with expected status:
console.log(response.body); // json response here
console.log(response.status);
console.log(response.statusText);
console.log(response.headers);
})
.catch((err) => {
// handle response with unexpected status:
console.log(err.name);
console.log(err.message);
console.log(err.response.status);
console.log(err.response.statusText);
console.log(err.response.body);
console.log(err.response.text);
console.log(err.response.headers);
});
This library comes with built-in TypeScript type declarations.
Due to complexities in dealing with isomorphic-fetch - which uses whatwg-fetch in browsers and node-fetch
in node.js, which are subtly different - these type declarations only work if you include the DOM
built-in
TypeScript lib in your tsconfig.json
. For example:
{
"lib": ["DOM", "ES2020"]
}
This happens implicitly if you don't set a lib
.
This may be fixed in the future.
By default, jsonFetch doesn't retry requests. However, you may opt in to jsonFetch's very flexible retry behavior, provided by the excellent promise-retry
library. Here's a quick example:
import jsonFetch, {retriers} from 'json-fetch'
jsonFetch('http://www.test.com/products/1234', {
method: 'POST',
body: {name: 'apple'},
shouldRetry: retriers.isNetworkError // after every request, retry if a network error is thrown
retry: {
// Retry 5 times, in addition to the original request
retries: 5,
}
}).then(response => {
// handle responses
});
Any option that promise-retry
accepts will be passed through from options.retry
. See the promise-retry documentation for all options.
We've provided two default "retrier" functions that decide to retry 503/504 status code responses and network errors (jsonFetch.retriers.is5xx
and jsonFetch.retriers.isNetworkError
respectively). You can easily provide your own custom retrier function to options.shouldRetry
.
The contract for a retrier function is:
shouldRetry([Error || FetchResponse]) returns bool
You can use any attribute of the FetchResponse or Error to determine whether to retry or not. Your function must handle both errors (such as network errors) and FetchResponse objects without blowing up. We recommend stateless, side-effect free functions. You do not need to worry about the maximum number of retries -- promise-retry will stop retrying after the maximum you specify. See the tests and src/retriers.js
file for examples.
Two callback functions can be passed as options to do something onRequestStart
and onRequestEnd
. This may be especially helpful to log request and response data on each request.
If you have retries enabled, these will trigger before and after each actual, individual request.
onRequestStart
If given, onRequestStart
is called with:
{
// ... all the original json-fetch options, plus:
url: string;
retryCount: number;
}
onRequestEnd
If given, onRequestEnd
is called with:
{
// ... all the original json-fetch options, plus:
url: string;
retryCount: number;
status?: Response['status'];
error?: Error;
}
For example, to log before and after each request:
const requestUrl = 'http://www.test.com/products/1234';
await jsonFetch(requestUrl, {
onRequestStart: ({url, timeout, retryCount}) =>
console.log(`Requesting ${url} with timeout ${timeout}, attempt ${retryCount}`),
onRequestEnd: ({url, retryCount, status}) =>
console.log(`Requested ${url}, attempt ${retryCount}, got status ${status}`),
});
Please follow our Code of Conduct when contributing to this project.
yarn install
yarn test
This module is automatically deployed when a version tag bump is detected by Travis. Remember to update the changelog!
yarn version
Module scaffold generated by generator-goodeggs-npm.
null
before setting the code property.FAQs
A wrapper around ES6 fetch to simplify interacting with JSON APIs.
The npm package json-fetch receives a total of 64 weekly downloads. As such, json-fetch popularity was classified as not popular.
We found that json-fetch demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.