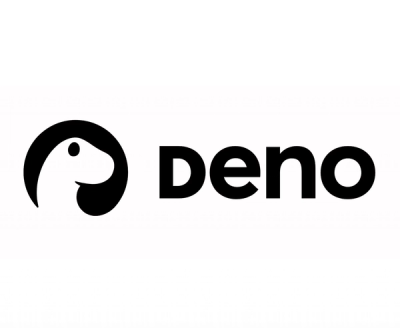
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
json-file-plus
Advanced tools
[![github actions][actions-image]][actions-url] [![coverage][codecov-image]][codecov-url] [![dependency status][deps-svg]][deps-url] [![dev dependency status][dev-deps-svg]][dev-deps-url] [![License][license-image]][license-url] [![Downloads][downloads-im
A module to read from and write to JSON files, without losing formatting, to minimize diffs.
const jsonFile = require('json-file-plus');
const path = require('path');
const filename = path.join(process.cwd(), 'package.json');
jsonFile(filename).then((file) => {
file.data; // Direct access to the data from the file
file.format; // extracted formatting data. change at will.
file.get('version'); // get top-level keys. returns a Promise
file.get(); // get entire data. returns a Promise
/* pass any plain object into "set" to merge in a deep copy */
/* please note: references will be broken. */
/* if a non-plain object is passed, will throw a TypeError. */
file.set({
foo: 'bar',
bar: {
baz: true,
},
});
file.remove('description'); // remove a specific key-value pair. returns a Promise
/* change the filename if desired */
file.filename = path.join(process.cwd(), 'new-package.json');
/* Save the file, preserving formatting. returns a Promise. */
file.save().then(function () {
console.log('success!');
}).catch(function (err) {
console.log('error!', err);
});
});
Simply run npm test
in the repo
FAQs
[![github actions][actions-image]][actions-url] [![coverage][codecov-image]][codecov-url] [![dependency status][deps-svg]][deps-url] [![dev dependency status][dev-deps-svg]][dev-deps-url] [![License][license-image]][license-url] [![Downloads][downloads-im
The npm package json-file-plus receives a total of 83,324 weekly downloads. As such, json-file-plus popularity was classified as popular.
We found that json-file-plus demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.