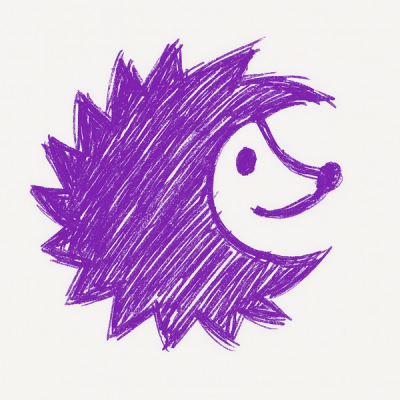
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
json-schema-ref-resolver
Advanced tools
The json-schema-ref-resolver npm package is a utility for resolving JSON Schema $ref references. It allows you to dereference, bundle, and resolve JSON Schema references, making it easier to work with complex schemas that have multiple references.
Dereferencing
Dereferencing resolves all $ref pointers in the schema, replacing them with the actual referenced values. This is useful for working with a fully expanded schema.
const $RefParser = require('json-schema-ref-resolver');
const schema = {
properties: {
name: { $ref: '#/definitions/name' }
},
definitions: {
name: { type: 'string' }
}
};
$RefParser.dereference(schema, (err, dereferencedSchema) => {
if (err) {
console.error(err);
} else {
console.log(dereferencedSchema);
}
});
Bundling
Bundling resolves all external $ref pointers and bundles them into a single schema. This is useful for creating a self-contained schema that doesn't rely on external files.
const $RefParser = require('json-schema-ref-resolver');
const schema = {
properties: {
name: { $ref: 'definitions.json#/name' }
}
};
$RefParser.bundle(schema, (err, bundledSchema) => {
if (err) {
console.error(err);
} else {
console.log(bundledSchema);
}
});
Resolving
Resolving retrieves and parses all referenced files, but does not replace the $ref pointers. This is useful for understanding the structure and dependencies of a schema without modifying it.
const $RefParser = require('json-schema-ref-resolver');
const schema = {
properties: {
name: { $ref: 'definitions.json#/name' }
}
};
$RefParser.resolve(schema, (err, resolvedSchema) => {
if (err) {
console.error(err);
} else {
console.log(resolvedSchema);
}
});
json-schema-ref-parser is a similar package that also resolves JSON Schema $ref pointers. It provides similar functionality for dereferencing, bundling, and resolving schemas. It is known for its ease of use and comprehensive documentation.
json-schema-deref-sync is another package that resolves JSON Schema $ref pointers, but it does so synchronously. This can be useful in environments where asynchronous operations are not desired or necessary.
json-schema-resolver is a lightweight package for resolving JSON Schema $ref pointers. It focuses on simplicity and minimalism, making it a good choice for smaller projects or those with simpler schemas.
json-schema-ref-resolver is a javascript library that resolves references in JSON schemas.
npm install json-schema-ref-resolver
const assert = require('node:assert')
const { RefResolver } = require('json-schema-ref-resolver')
const sourceSchema = {
$id: 'sourceSchema',
type: 'object',
properties: {
foo: {
$ref: 'targetSchema#/definitions/bar'
}
}
}
const targetSchema = {
$id: 'targetSchema',
definitions: {
bar: {
type: 'string'
}
}
}
const refResolver = new RefResolver()
refResolver.addSchema(sourceSchema)
refResolver.addSchema(targetSchema)
const derefSourceSchema = refResolver.getDerefSchema('sourceSchema')
assert.deepStrictEqual(derefSourceSchema, {
$id: 'sourceSchema',
type: 'object',
properties: {
foo: {
type: 'string'
}
}
})
allowEqualDuplicates - if set to false
, an error will be thrown if a schema with the same $id
is added to the resolver. If set to true
, the error will be thrown only if the schemas are not equal. Default: true
.
insertRefSymbol - if set to true
resolver inserts a Symbol.for('json-schema-ref')
instead of the $ref
property when dereferencing a schema. Default false
.
cloneSchemaWithoutRefs - if set to false
resolver would not clone a schema if it does not have references. That allows to significantly improve performance when dereferencing a schema. If you want to modify a schema after dereferencing, set this option to true
. Default: false
.
Adds a json schema to the resolver. If the schema has an $id
property, it will be used as the schema id. Otherwise, the schemaId
argument will be used as the schema id. During the addition of the schema, ref resolver will find and add all nested schemas and references.
schema
<object> - json schema to add to the resolver.schemaId
<string> - schema id to use. Will be used only if the schema does not have an $id
property.Returns a json schema by its id. If the jsonPointer
argument is provided, ref resolver will return the schema at the specified json pointer location.
If shema with the specified id is not found, an error will be thrown. If the jsonPointer
argument is provided and the schema at the specified location is not found, getSchema will return null
.
schemaId
<string> - schema id of the schema to return.jsonPointer
<string> - json pointer to the schema location.null
if the schema at the specified location is not found.Example:
const assert = require('node:assert')
const { RefResolver } = require('json-schema-ref-resolver')
const schema = {
$id: 'schema',
type: 'object',
properties: {
foo: { type: 'string' }
}
}
const refResolver = new RefResolver()
refResolver.addSchema(schema)
const rootSchema = refResolver.getSchema(schema.$id)
assert.deepStrictEqual(rootSchema, schema)
const subSchema = refResolver.getSchema(schema.$id, '#/properties/foo')
assert.deepStrictEqual(subSchema, { type: 'string' })
getSchema
can also be used to get a schema by its json schema anchor. To get schema by schema anchor, the schemaId
argument must be set to the $id
of the schema that contains the anchor, and the jsonPointer
argument must be set to the anchor.
Example:
const assert = require('node:assert')
const { RefResolver } = require('json-schema-ref-resolver')
const schema = {
$id: 'schema',
definitions: {
bar: {
$id: '#bar',
type: 'string'
}
}
}
const refResolver = new RefResolver()
refResolver.addSchema(schema)
const anchorSchema = refResolver.getSchema(schema.$id, '#bar')
assert.deepStrictEqual(subSchema, {
$id: '#bar',
type: 'string'
})
Returns all references found in the schema during the addition of the schema to the resolver. If schema with the specified id is not found, an error will be thrown.
schemaId
<string> - schema id of the schema to return references for.schemaId
<string> - schema id of the reference.jsonPointer
<string> - json pointer of the reference.If a reference does not have a schema id part, the schema id of the schema that contains the reference is used as the schema id of the reference.
Example:
const assert = require('node:assert')
const { RefResolver } = require('json-schema-ref-resolver')
const sourceSchema = {
$id: 'sourceSchema',
type: 'object',
properties: {
foo: {
$ref: 'targetSchema#/definitions/bar'
},
baz: {
$ref: 'targetSchema#/definitions/qux'
}
}
}
const refResolver = new RefResolver()
refResolver.addSchema(sourceSchema)
const refs = refResolver.getSchemaRefs('sourceSchema')
assert.deepStrictEqual(refs, [
{
schemaId: 'targetSchema',
jsonPointer: '#/definitions/bar'
},
{
schemaId: 'targetSchema',
jsonPointer: '#/definitions/qux'
}
])
Returns all dependencies including nested dependencies found in the schema during the addition of the schema to the resolver. If schema with the specified id is not found, an error will be thrown.
schemaId
<string> - schema id of the schema to return dependencies for.Example:
const assert = require('node:assert')
const { RefResolver } = require('json-schema-ref-resolver')
const targetSchema1 = {
$id: 'targetSchema1',
definitions: {
bar: { type: 'string' }
}
}
const targetSchema2 = {
$id: 'targetSchema2',
type: 'object',
properties: {
qux: { $ref: 'targetSchema1' }
}
}
const sourceSchema = {
$id: 'sourceSchema',
type: 'object',
properties: {
foo: { $ref: 'targetSchema2' }
}
}
const refResolver = new RefResolver()
refResolver.addSchema(sourceSchema)
refResolver.addSchema(targetSchema1)
refResolver.addSchema(targetSchema2)
const dependencies = refResolver.getSchemaDependencies('sourceSchema')
assert.deepStrictEqual(dependencies, { targetSchema1, targetSchema2 })
Dereferences all references in the schema. All dependencies will also be dereferenced. If schema with the specified id is not found, an error will be thrown.
schemaId
<string> - schema id of the schema to dereference.If a json schema has circular references or circular cross-references, dereferenced schema will have js circular references. Be careful when traversing the dereferenced schema.
Example
const assert = require('node:assert')
const { RefResolver } = require('json-schema-ref-resolver')
const sourceSchema = {
$id: 'sourceSchema',
type: 'object',
properties: {
foo: {
$ref: 'targetSchema#/definitions/bar'
}
}
}
const targetSchema = {
$id: 'targetSchema',
definitions: {
bar: {
type: 'string'
}
}
}
const refResolver = new RefResolver()
refResolver.addSchema(sourceSchema)
refResolver.addSchema(targetSchema)
refResolver.derefSchema('sourceSchema')
const derefSourceSchema = refResolver.getDerefSchema('sourceSchema')
assert.deepStrictEqual(derefSourceSchema, {
$id: 'sourceSchema',
type: 'object',
properties: {
foo: {
type: 'string'
}
}
})
Returns a dereferenced schema by schema id and json pointer. If schema with the specified id is not found, an error will be thrown. If the jsonPointer
argument is provided, ref resolver will return the schema at the specified json pointer location. If the jsonPointer
argument is provided and the schema at the specified location is not found, getDerefSchema will return null
.
If schema was not dereferenced before, it will be dereferenced during the call to getDerefSchema
.
schemaId
<string> - schema id of the schema to return.jsonPointer
<string> - json pointer to the schema location.null
if the schema at the specified location is not found.Example:
const assert = require('node:assert')
const { RefResolver } = require('json-schema-ref-resolver')
const sourceSchema = {
$id: 'sourceSchema',
type: 'object',
properties: {
foo: {
$ref: 'targetSchema#/definitions/bar'
}
}
}
const targetSchema = {
$id: 'targetSchema',
definitions: {
bar: {
type: 'string'
}
}
}
const refResolver = new RefResolver()
refResolver.addSchema(sourceSchema)
refResolver.addSchema(targetSchema)
const derefSourceSchema = refResolver.getDerefSchema('sourceSchema')
assert.deepStrictEqual(derefSourceSchema, {
$id: 'sourceSchema',
type: 'object',
properties: {
foo: {
type: 'string'
}
}
})
derefSchema
or getDerefSchema
.Example:
const assert = require('node:assert')
const { RefResolver } = require('json-schema-ref-resolver')
const targetSchema = {
$id: 'targetSchema',
definitions: {
bar: {
type: 'object',
properties: {
foo: { type: 'string' }
}
}
}
}
const sourceSchema = {
$id: 'sourceSchema',
type: 'object',
$ref: 'targetSchema#/definitions/bar'
properties: {
foo: { type: 'number' }
}
}
const refResolver = new RefResolver()
refResolver.addSchema(targetSchema)
refResolver.addSchema(sourceSchema)
refResolver.derefSchema('sourceSchema') // Throws an error
Licensed under MIT.
FAQs
JSON schema reference resolver
The npm package json-schema-ref-resolver receives a total of 1,165,358 weekly downloads. As such, json-schema-ref-resolver popularity was classified as popular.
We found that json-schema-ref-resolver demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.