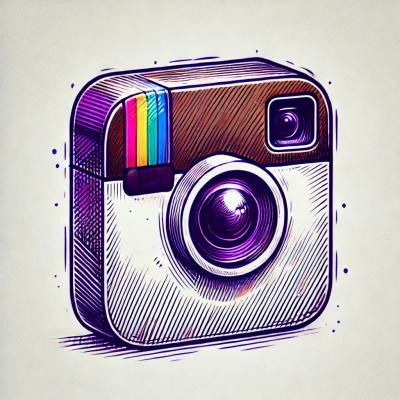
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
The jsonfile npm package is a simple JSON file reading and writing library for Node.js. It provides utility functions to read and write JSON files with ease, handling the asynchronous file system operations and JSON parsing/stringifying under the hood.
Reading JSON files
This feature allows you to read JSON files asynchronously. The readFile function takes a file path and a callback function that receives an error or the parsed JSON object.
const jsonfile = require('jsonfile');
jsonfile.readFile('/path/to/file.json', (err, obj) => {
if (err) console.error(err);
console.log(obj);
});
Writing JSON files
This feature allows you to write JSON objects to files asynchronously. The writeFile function takes a file path, the object to be written, and a callback function that is called upon completion or error.
const jsonfile = require('jsonfile');
const obj = {name: 'John', age: 30};
jsonfile.writeFile('/path/to/file.json', obj, (err) => {
if (err) console.error(err);
});
Reading JSON files synchronously
This feature allows you to read JSON files synchronously, blocking the event loop until the file is read. The readFileSync function takes a file path and returns the parsed JSON object or throws an error.
const jsonfile = require('jsonfile');
try {
const obj = jsonfile.readFileSync('/path/to/file.json');
console.log(obj);
} catch (err) {
console.error(err);
}
Writing JSON files synchronously
This feature allows you to write JSON objects to files synchronously, blocking the event loop until the file is written. The writeFileSync function takes a file path and the object to be written, and it will throw an error if the operation fails.
const jsonfile = require('jsonfile');
const obj = {name: 'Jane', age: 25};
try {
jsonfile.writeFileSync('/path/to/file.json', obj);
} catch (err) {
console.error(err);
}
Customizing JSON spacing
This feature allows you to customize the indentation of the JSON output. The writeFile and writeFileSync functions accept an options object where you can specify the number of spaces to use for indentation.
const jsonfile = require('jsonfile');
const obj = {name: 'Alice', age: 28};
jsonfile.writeFile('/path/to/file.json', obj, {spaces: 2}, (err) => {
if (err) console.error(err);
});
fs-extra is a package that builds on the native fs module, providing additional methods and functionality. It includes methods for JSON file handling similar to jsonfile, such as readJson, writeJson, readJsonSync, and writeJsonSync. fs-extra offers a broader set of file system operations, making it a more comprehensive choice for general file system tasks.
fs-jetpack is another file system library that offers a fluent API for file operations. It includes read and write functionalities for JSON files with methods like readAsync, writeAsync, read, and write. fs-jetpack provides a different API design and additional file manipulation methods, which might be preferred by some developers for its convenience and chaining capabilities.
Easily read/write JSON files in Node.js. Note: this module cannot be used in the browser.
Writing JSON.stringify()
and then fs.writeFile()
and JSON.parse()
with fs.readFile()
enclosed in try/catch
blocks became annoying.
npm install --save jsonfile
readFile(filename, [options], callback)
readFileSync(filename, [options])
writeFile(filename, obj, [options], callback)
writeFileSync(filename, obj, [options])
options
(object
, default undefined
): Pass in any fs.readFile
options or set reviver
for a JSON reviver.
throws
(boolean
, default: true
). If JSON.parse
throws an error, pass this error to the callback.
If false
, returns null
for the object.const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
jsonfile.readFile(file, function (err, obj) {
if (err) console.error(err)
console.dir(obj)
})
You can also use this method with promises. The readFile
method will return a promise if you do not pass a callback function.
const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
jsonfile.readFile(file)
.then(obj => console.dir(obj))
.catch(error => console.error(error))
options
(object
, default undefined
): Pass in any fs.readFileSync
options or set reviver
for a JSON reviver.
throws
(boolean
, default: true
). If an error is encountered reading or parsing the file, throw the error. If false
, returns null
for the object.const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
console.dir(jsonfile.readFileSync(file))
options
: Pass in any fs.writeFile
options or set replacer
for a JSON replacer. Can also pass in spaces
, or override EOL
string or set finalEOL
flag as false
to not save the file with EOL
at the end.
const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
const obj = { name: 'JP' }
jsonfile.writeFile(file, obj, function (err) {
if (err) console.error(err)
})
Or use with promises as follows:
const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
const obj = { name: 'JP' }
jsonfile.writeFile(file, obj)
.then(res => {
console.log('Write complete')
})
.catch(error => console.error(error))
formatting with spaces:
const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
const obj = { name: 'JP' }
jsonfile.writeFile(file, obj, { spaces: 2 }, function (err) {
if (err) console.error(err)
})
overriding EOL:
const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
const obj = { name: 'JP' }
jsonfile.writeFile(file, obj, { spaces: 2, EOL: '\r\n' }, function (err) {
if (err) console.error(err)
})
disabling the EOL at the end of file:
const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
const obj = { name: 'JP' }
jsonfile.writeFile(file, obj, { spaces: 2, finalEOL: false }, function (err) {
if (err) console.log(err)
})
appending to an existing JSON file:
You can use fs.writeFile
option { flag: 'a' }
to achieve this.
const jsonfile = require('jsonfile')
const file = '/tmp/mayAlreadyExistedData.json'
const obj = { name: 'JP' }
jsonfile.writeFile(file, obj, { flag: 'a' }, function (err) {
if (err) console.error(err)
})
options
: Pass in any fs.writeFileSync
options or set replacer
for a JSON replacer. Can also pass in spaces
, or override EOL
string or set finalEOL
flag as false
to not save the file with EOL
at the end.
const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
const obj = { name: 'JP' }
jsonfile.writeFileSync(file, obj)
formatting with spaces:
const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
const obj = { name: 'JP' }
jsonfile.writeFileSync(file, obj, { spaces: 2 })
overriding EOL:
const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
const obj = { name: 'JP' }
jsonfile.writeFileSync(file, obj, { spaces: 2, EOL: '\r\n' })
disabling the EOL at the end of file:
const jsonfile = require('jsonfile')
const file = '/tmp/data.json'
const obj = { name: 'JP' }
jsonfile.writeFileSync(file, obj, { spaces: 2, finalEOL: false })
appending to an existing JSON file:
You can use fs.writeFileSync
option { flag: 'a' }
to achieve this.
const jsonfile = require('jsonfile')
const file = '/tmp/mayAlreadyExistedData.json'
const obj = { name: 'JP' }
jsonfile.writeFileSync(file, obj, { flag: 'a' })
(MIT License)
Copyright 2012-2016, JP Richardson jprichardson@gmail.com
FAQs
Easily read/write JSON files.
The npm package jsonfile receives a total of 58,385,748 weekly downloads. As such, jsonfile popularity was classified as popular.
We found that jsonfile demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.