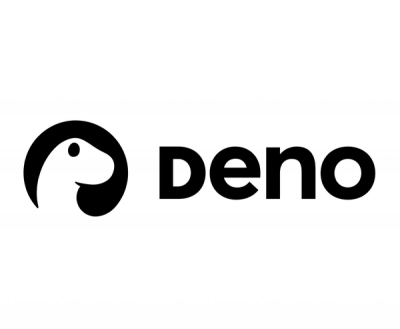
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
[](https://gitlab.com/michaelsoftware/jsonui/) [](https://www.npmjs.com/package/jso
The jsonUI is more a proof of concept than something you could or should use in a production environment. It is more for small private projects that wants to try something new.
Personally I have used the jsonUI (or jUI) since over a year within my private homeserver-project. Certainly not in the current form (it was a mess and written in ES5).
jsonUI is a framework to create a user interface mainly with json. It is planned, that you could send your jsonUI besides the normal data of your application. jsonUI could use something like minfied json to reduce the overhead.
To have all the freedom you need, jsonUI allows you to define custom elements and add them to the parser (the default elements also use these methods).
Currently only the parser is ready for NPM yet but a creator (mainly for the backend) will be added to this project too.
npm install -S jsonui@latest
jsonUI is written in ES6 and needs to be "compiled" by babel to work in all browsers. You maybe have to polyfill methods in some browsers. jsonUI has some basic polyfills that it needs to work correctly. When you need them, you have to import them from src/polyfills (or lib/polyfills) or use your own ones. The polyfills are only needed in some older browsers.
This is the list of all polyfills that you maybe need:
You have to import the parser first:
import {Parser} from 'jsonui';
Than you could create your first jsonUI-object. We will create a headline with the text Hello World.
const jui = {
body: [{
type: 'headline',
value: 'Hello World'
}]
}
The jsonUI-Parser can parse this object to a JuiDocument. Moreover the jsonUI-Parser could handle a json-string, that it parses automatically. The JuiDocument is only a wrapper to add listeners that handles actions (e.g. submit). You could append the views from it to the dom:
let juiParser = new Parser();
juiParser.parse(jui, (juiDocument) => {
const views = juiDocument.getViews();
document.body.appendChild(views);
});
Currently some elements needs to get some texts from the language-files (already build-in). You have to set it up once:
import {config} from 'jsonui';
config.init({
langCode: 'en_GB'
});
The jui-object from the last example could be rewritten using the jsonUI-constants. You need to import them too (or use the numbers directly, which is not recommended).
import {constants} from 'jsonui';
const jui = {
body: [{
[constants.keys.type]: constants.values.type.headline,
[constants.keys.value]: "Hello World",
}]
}
I can't describe everything that you could do with jsonUi here. This are only some basics. I would recommend developers that wants to develop own elements to have a closer look on the src/elements directory.
webpack is currently only used as a dev-server. It serves the example, with which you could develop new components easily.
Most parts of the project (the most important parts) are commented using the jsdoc-syntax.
If you have installed jsdoc globally on your computer you could run npm run jsdoc
to
generate a jsdoc in the directory jsdoc with the newest definitions.
There is an eslint config in this project. You could check the source code with npm run eslint
.
jsonUI uses karma, mocha and chai to test the project for errors. It can not guarantee that
everything work as expected, but it helps to prevent big mistakes.
The project could be tested using npm run test
for a single run test or
npm run test:dev
for a test that watches for changes.
FAQs
[](https://gitlab.com/michaelsoftware/jsonui/) [](https://www.npmjs.com/package/jso
We found that jsonui demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.