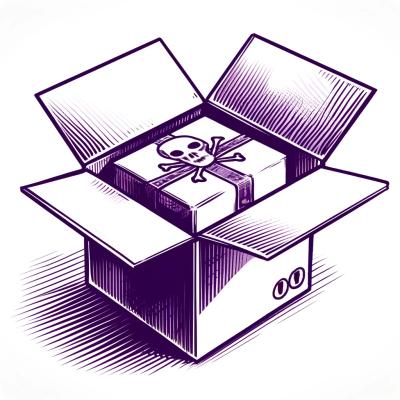
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
koa-helmet
Advanced tools
koa-helmet is a middleware for Koa.js that helps secure your Koa applications by setting various HTTP headers. It is a Koa wrapper for the popular Helmet.js library, which is widely used in Express.js applications.
Content Security Policy
This feature helps prevent cross-site scripting (XSS) attacks and other cross-site injections by setting the Content-Security-Policy header.
const Koa = require('koa');
const helmet = require('koa-helmet');
const app = new Koa();
app.use(helmet.contentSecurityPolicy({
directives: {
defaultSrc: ["'self'"],
styleSrc: ["'self'", 'https:']
}
}));
app.listen(3000);
DNS Prefetch Control
This feature controls browser DNS prefetching by setting the X-DNS-Prefetch-Control header.
const Koa = require('koa');
const helmet = require('koa-helmet');
const app = new Koa();
app.use(helmet.dnsPrefetchControl({ allow: false }));
app.listen(3000);
Frameguard
This feature helps prevent clickjacking attacks by setting the X-Frame-Options header.
const Koa = require('koa');
const helmet = require('koa-helmet');
const app = new Koa();
app.use(helmet.frameguard({ action: 'deny' }));
app.listen(3000);
Hide Powered-By
This feature removes the X-Powered-By header to make it harder for attackers to see what technology your site is using.
const Koa = require('koa');
const helmet = require('koa-helmet');
const app = new Koa();
app.use(helmet.hidePoweredBy());
app.listen(3000);
HSTS
This feature adds the Strict-Transport-Security header to enforce secure (HTTP over SSL/TLS) connections to the server.
const Koa = require('koa');
const helmet = require('koa-helmet');
const app = new Koa();
app.use(helmet.hsts({ maxAge: 31536000 }));
app.listen(3000);
Helmet is a middleware for Express.js that helps secure your Express applications by setting various HTTP headers. It is the original library that koa-helmet wraps around. While koa-helmet is specifically designed for Koa.js, Helmet is designed for Express.js.
Lusca is another security middleware for Express.js that provides various security features such as CSRF protection, XSS protection, and more. It is similar to Helmet but offers additional features like CSRF protection out of the box.
Express-rate-limit is a middleware for Express.js that helps to limit repeated requests to public APIs and/or endpoints. While it does not provide the same HTTP header protections as koa-helmet, it is useful for preventing abuse and brute-force attacks.
koa-helmet is a wrapper for helmet to work with koa. It provides important security headers to make your app more secure by default.
npm i koa-helmet helmet
# or:
yarn add koa-helmet helmet
Usage is the same as helmet
Helmet offers 11 security middleware functions:
// This...
app.use(helmet());
// ...is equivalent to this:
app.use(helmet.contentSecurityPolicy());
app.use(helmet.dnsPrefetchControl());
app.use(helmet.expectCt());
app.use(helmet.frameguard());
app.use(helmet.hidePoweredBy());
app.use(helmet.hsts());
app.use(helmet.ieNoOpen());
app.use(helmet.noSniff());
app.use(helmet.permittedCrossDomainPolicies());
app.use(helmet.referrerPolicy());
app.use(helmet.xssFilter());
You can see more in the documentation.
import Koa from "koa";
import helmet from "koa-helmet";
const app = new Koa();
app.use(helmet());
app.use((ctx) => {
ctx.body = "Hello World";
});
app.listen(4000);
To run the tests, simply run
npm test
FAQs
Security header middleware collection for koa
The npm package koa-helmet receives a total of 196,966 weekly downloads. As such, koa-helmet popularity was classified as popular.
We found that koa-helmet demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.