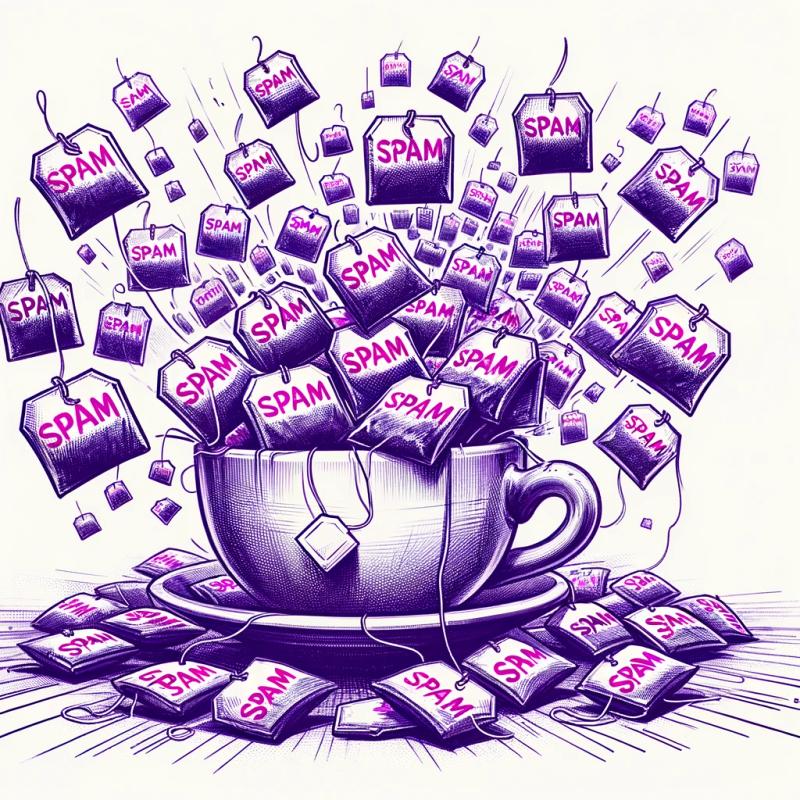
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
koa-send
Advanced tools
Readme
Static file serving middleware.
$ npm install koa-send
maxage
Browser cache max-age in milliseconds. (defaults to 0
).immutable
Tell the browser the resource is immutable and can be cached indefinitely. (defaults to false
).hidden
Allow transfer of hidden files. (defaults to false
).root
Root directory to restrict file access.index
Name of the index file to serve automatically when visiting the root location. (defaults to none).gzip
Try to serve the gzipped version of a file automatically when gzip
is supported by a client and if the requested file with .gz
extension exists. (defaults to true
).brotli
Try to serve the brotli version of a file automatically when brotli
is supported by a client and if the requested file with .br
extension exists. (defaults to true
).format
If not false
(defaults to true
), format the path to serve static file servers and not require a trailing slash for directories, so that you can do both /directory
and /directory/
.setHeaders
Function to set custom headers on response.extensions
Try to match extensions from passed array to search for file when no extension is sufficed in URL. First found is served. (defaults to false
)Note that root
is required, defaults to ''
and will be resolved,
removing the leading /
to make the path relative and this
path must not contain "..", protecting developers from
concatenating user input. If you plan on serving files based on
user input supply a root
directory from which to serve from.
For example to serve files from ./public
:
app.use(async (ctx) => {
await send(ctx, ctx.path, { root: __dirname + '/public' });
})
To serve developer specified files:
app.use(async (ctx) => {
await send(ctx, 'path/to/my.js');
})
The function is called as fn(res, path, stats)
, where the arguments are:
res
: the response object.path
: the resolved file path that is being sent.stats
: the stats object of the file that is being sent.You should only use the setHeaders
option when you wish to edit the Cache-Control
or Last-Modified
headers, because doing it before is useless (it's overwritten by send
), and doing it after is too late because the headers are already sent.
If you want to edit any other header, simply set them before calling send
.
const send = require('koa-send');
const Koa = require('koa');
const app = new Koa();
// $ GET /package.json
// $ GET /
app.use(async (ctx) => {
if ('/' == ctx.path) return ctx.body = 'Try GET /package.json';
await send(ctx, ctx.path);
})
app.listen(3000);
console.log('listening on port 3000');
FAQs
Transfer static files
The npm package koa-send receives a total of 691,956 weekly downloads. As such, koa-send popularity was classified as popular.
We found that koa-send demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.