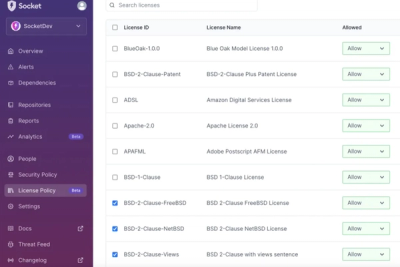
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
koios-tiny-client
Advanced tools
TypeScript client for XRAY | Graph | Koios API and original Koios API
Automatically generated Axios-based client for Koios Cardano RESTful API based on koiosapi.yaml schema. Works great with XRAY | Graph | Koios API (a dockered Koios-based & DB Sync Cardano API solution) and the original Koios.
To install the client with Yarn, run:
yarn install koios-tiny-client
To install the client with NPM, run:
npm i koios-tiny-client
import KoiosTinyClient from "koios-tiny-client"
const { client, methods: Koios } = new KoiosTinyClient("https://api.koios.rest/api/v0")
// async call
const app = async () => {
const Tip = await Koios.Tip()
if (Tip.ok) {
console.log(Tip.ok.data[0].block_no)
}
if (Tip.error) {
console.error(Tip.error)
}
}
app()
Read https://api.koios.rest/#overview--api-usage for more information
const { methods: Koios } = new KoiosTinyClient("https://api.koios.rest/api/v0")
const Blocks = await Koios.Blocks("&pool=eq.pool155efqn9xpcf73pphkk88cmlkdwx4ulkg606tne970qswczg3asc&order=block_height.asc")
console.log(Blocks)
const PoolsList = await Koios.PoolList("&ticker=like.*RAY*")
console.log(PoolsList)
const { methods: Koios } = new KoiosTinyClient("https://api.koios.rest/api/v0")
// simple offset limit
const Blocks = await Koios.Blocks("&offset=10&limit=5")
console.log(Blocks)
// advanced pagination
const headers = { Prefer: "count=estimated" } // get the exact "content-range" header in the request
const PoolListFirst5Items = await Koios.PoolList("&limit=5", headers)
if (PoolListFirst5Items.ok) {
const contentRange = PoolListFirst5Items.ok.headers?.["content-range"] || ""
const [currentPosition, totalItems] = contentRange.split('/')
const queryRange = `${Number(totalItems) - 5}-${Number(totalItems)}`
const PoolListLast5Items = await Koios.PoolList(undefined, { ...headers, Range: queryRange})
console.log(PoolListLast5Items)
}
const { client, methods: Koios } = new KoiosTinyClient("https://api.koios.rest/api/v0")
// clear interceptors
client.interceptors.response.clear()
// your response interceptor, used as default shown in the example below
client.interceptors.response.use(
(response: AxiosResponse): any => {
return {
ok: response,
}
},
(error: AxiosError): any => {
return {
error,
}
}
)
// your request interceptor
client.interceptors.request.use(
// ...
)
const { methods: Koios } = new KoiosTinyClient("https://api.koios.rest/api/v0")
const abortController = new AbortController()
setTimeout(() => {
abortController.abort() // cancel request
console.log('Aborted!')
}, 200)
const PoolList = await Koios.PoolList(undefined, undefined, abortController.signal)
import KoiosTinyClient, { KoiosTypes } from "koios-tiny-client"
// no error
const correctItem: KoiosTypes.IAssetTokenRegistry = {
policy_id: "somePolidyId",
asset_name: "someAssetNameOrNull",
asset_name_ascii: "someAsciiName",
ticker: "someTicker",
description: "someDescription",
url: "someUrl",
decimals: 6,
logo: "someBase64PngString"
}
// error
const wrongItem: KoiosTypes.IAssetTokenRegistry = {
policy_id: "somePolidyId",
}
Managed by Cardano Community (Koios Elastic Query Layer / HAproxy Balancer)
https://api.koios.rest/api/v1
https://preprod.koios.rest/api/v1
https://preview.koios.rest/api/v1
https://guild.koios.rest/api/v1
Managed by Ray Network (RayGraph-Output Cluster / Cloudflare WAF & Load Balancer)
https://graph.xray.app/output/mainnet/koios/api/v1
https://graph.xray.app/output/preprod/koios/api/v1
https://graph.xray.app/output/preview/koios/api/v1
Downloads the YAML schema and generates library methods and types
yarn codegen && yarn build
Use the https://api.koios.rest/ sandbox to explore and live query all available API methods
Differences with the original Koios:
/submittx
endpoint: not available, use XRAY | Graph | Tx Turbo Send API/ogmios
endpoint: not available, use XRAY | Graph | Ogmios APIName | Description |
---|---|
Tip() |
Query Chain Tip Get the tip info about the latest block seen by chain |
Genesis() |
Get Genesis info Get the Genesis parameters used to start specific era on chain |
Totals() |
Get historical tokenomic stats Get the circulating utxo, treasury, rewards, supply and reserves in lovelace for specified epoch, all epochs if empty |
ParamUpdates() |
Param Update Proposals Get all parameter update proposals submitted to the chain starting Shelley era |
ReserveWithdrawals() |
Reserve Withdrawals List of all withdrawals from reserves against stake accounts |
TreasuryWithdrawals() |
Treasury Withdrawals List of all withdrawals from treasury against stake accounts |
EpochInfo() |
Epoch Information Get the epoch information, all epochs if no epoch specified |
EpochParams() |
Epoch's Protocol Parameters Get the protocol parameters for specific epoch, returns information about all epochs if no epoch specified |
EpochBlockProtocols() |
Epoch's Block Protocols Get the information about block protocol distribution in epoch |
Blocks() |
Block List Get summarised details about all blocks (paginated - latest first) |
BlockInfo() |
Block Information Get detailed information about a specific block |
BlockTxs() |
Block Transactions Get a list of all transactions included in provided blocks |
UtxoInfo() |
UTxO Info Get UTxO set for requested UTxO references |
TxInfo() |
Transaction Information Get detailed information about transaction(s) |
TxMetadata() |
Transaction Metadata Get metadata information (if any) for given transaction(s) |
TxMetalabels() |
Transaction Metadata Labels Get a list of all transaction metalabels |
TxStatus() |
Transaction Status Get the number of block confirmations for a given transaction hash list |
TxUtxos() |
Transaction UTxOs Get UTxO set (inputs/outputs) of transactions [DEPRECATED - Use /utxo_info instead]. |
AddressInfo() |
Address Information Get address info - balance, associated stake address (if any) and UTxO set for given addresses |
AddressUtxos() |
Address UTXOs Get UTxO set for given addresses |
CredentialUtxos() |
UTxOs from payment credentials Get UTxO details for requested payment credentials |
AddressTxs() |
Address Transactions Get the transaction hash list of input address array, optionally filtering after specified block height (inclusive) |
CredentialTxs() |
Transactions from payment credentials Get the transaction hash list of input payment credential array, optionally filtering after specified block height (inclusive) |
AddressAssets() |
Address Assets Get the list of all the assets (policy, name and quantity) for given addresses |
AccountList() |
Account List Get a list of all stake addresses that have atleast 1 transaction |
AccountInfo() |
Account Information Get the account information for given stake addresses |
AccountInfoCached() |
Account Information (Cached) Get the cached account information for given stake addresses (effective for performance query against registered accounts) |
AccountUtxos() |
UTxOs for stake addresses (accounts) Get a list of all UTxOs for given stake addresses (account)s |
AccountTxs() |
Account Txs Get a list of all Txs for a given stake address (account) |
AccountRewards() |
Account Rewards Get the full rewards history (including MIR) for given stake addresses |
AccountUpdates() |
Account Updates Get the account updates (registration, deregistration, delegation and withdrawals) for given stake addresses |
AccountAddresses() |
Account Addresses Get all addresses associated with given staking accounts |
AccountAssets() |
Account Assets Get the native asset balance for a given stake address |
AccountHistory() |
Account History Get the staking history of given stake addresses (accounts) |
AssetList() |
Asset List Get the list of all native assets (paginated) |
PolicyAssetList() |
Policy Asset List Get the list of asset under the given policy (including balances) |
AssetTokenRegistry() |
Asset Token Registry Get a list of assets registered via token registry on github |
AssetInfoBulk() |
Asset Information (Bulk) Get the information of a list of assets including first minting & token registry metadata |
AssetUtxos() |
Asset UTXOs Get the UTXO information of a list of assets including |
AssetHistory() |
Asset History Get the mint/burn history of an asset |
AssetAddresses() |
Asset Addresses Get the list of all addresses holding a given asset `Note - Due to cardano's UTxO design and usage from projects, asset to addresses map can be infinite. Thus, for a small subset of active projects with millions of transactions, these might end up with timeouts (HTTP code 504) on free layer. Such large-scale projects are free to subscribe to query layers to have a dedicated cache table for themselves served via Koios.` |
AssetNftAddress() |
NFT Address Get the address where specified NFT currently reside on. |
PolicyAssetAddresses() |
Policy Asset Address List Get the list of addresses with quantity for each asset on the given policy `Note - Due to cardano's UTxO design and usage from projects, asset to addresses map can be infinite. Thus, for a small subset of active projects with millions of transactions, these might end up with timeouts (HTTP code 504) on free layer. Such large-scale projects are free to subscribe to query layers to have a dedicated cache table for themselves served via Koios.` |
PolicyAssetInfo() |
Policy Asset Information Get the information for all assets under the same policy |
AssetSummary() |
Asset Summary Get the summary of an asset (total transactions exclude minting/total wallets include only wallets with asset balance) |
AssetTxs() |
Asset Transactions Get the list of current or all asset transaction hashes (newest first) |
AssetAddressList() |
Asset Address List Get the list of all addresses holding a given asset [DEPRECATED - replaced by asset_addresses] |
AssetPolicyInfo() |
Asset Policy Information Get the information for all assets under the same policy (DEPRECATED - replaced by policy_asset_info) |
PoolList() |
Pool List List of brief info for all pools |
PoolInfo() |
Pool Information Current pool statuses and details for a specified list of pool ids |
PoolStakeSnapshot() |
Pool Stake Snapshot Returns Mark, Set and Go stake snapshots for the selected pool, useful for leaderlog calculation |
PoolDelegators() |
Pool Delegators List Return information about live delegators for a given pool. |
PoolDelegatorsHistory() |
Pool Delegators History Return information about active delegators (incl. history) for a given pool and epoch number (all epochs if not specified). |
PoolBlocks() |
Pool Blocks Return information about blocks minted by a given pool for all epochs (or _epoch_no if provided) |
PoolHistory() |
Pool Stake, Block and Reward History Return information about pool stake, block and reward history in a given epoch _epoch_no (or all epochs that pool existed for, in descending order if no _epoch_no was provided) |
PoolUpdates() |
Pool Updates (History) Return all pool updates for all pools or only updates for specific pool if specified |
PoolRegistrations() |
Pool Registrations Return all pool registrations initiated in the requested epoch |
PoolRetirements() |
Pool Retirements Return all pool retirements initiated in the requested epoch |
PoolRelays() |
Pool Relays A list of registered relays for all pools |
PoolMetadata() |
Pool Metadata Metadata (on & off-chain) for all pools |
ScriptInfo() |
Script Information List of script information for given script hashes |
NativeScriptList() |
Native Script List List of all existing native script hashes along with their creation transaction hashes |
PlutusScriptList() |
Plutus Script List List of all existing Plutus script hashes along with their creation transaction hashes |
ScriptRedeemers() |
Script Redeemers List of all redeemers for a given script hash |
ScriptUtxos() |
Script UTXOs List of all UTXOs for a given script hash |
DatumInfo() |
Datum Information List of datum information for given datum hashes |
FAQs
TypeScript client for XRAY | Graph | Koios API and original Koios API
The npm package koios-tiny-client receives a total of 0 weekly downloads. As such, koios-tiny-client popularity was classified as not popular.
We found that koios-tiny-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.