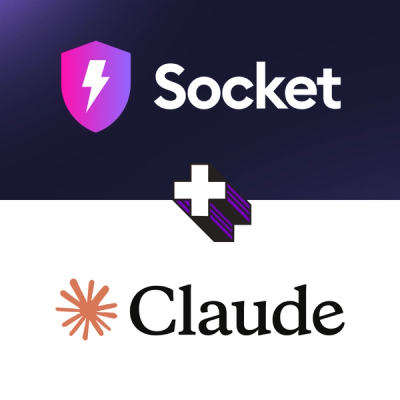
Product
Introducing Socket MCP for Claude Desktop
Add secure dependency scanning to Claude Desktop with Socket MCP, a one-click extension that keeps your coding conversations safe from malicious packages.
lastfm-njs
Advanced tools
A fully featured interface for Node and the Last.FM api
The full Last.FM API description can be found here
You'll need an API key from Create API Account
You can install using npm install --save lastfm-njs
Then you can set up like so, where username is a default username:
var lastfm = require("lastfm-njs");
var config = require("./config");
var lfm = new lastfm.default({
apiKey: config.key,
apiSecret: config.secret,
username: config.username,
});
If a method requires writing to an account, then you also need a password
var lfm = new lastfm.default({
apiKey: config.key,
apiSecret: config.secret,
username: config.username,
password: config.password,
});
This library supports both esm and cjs importing, commonjs will export as a default. You can use modules like
import LastFM from 'lastfm-njs';
const client = new LastFM(...)
or require with
const LastFM = require("lastfm-njs");
const client = new LastFM.default(...)
After this, you can use any of the methods
lastfm
.auth_getMobileSession()
.then(function (result) {
lastfm
.album_addTags({
artist: "Oh Pep!",
album: "Living",
tags: "peppy,folk,music",
})
.then(printRes)
.catch(printError);
})
.catch(printError);
See example files for other examples
A username and password is required
auth_getMobileSession();
which either returns
{
success: true,
key: 'XXX'
}
or
{
success: false,
error: [lastFMError]
}
album_addTags(opt)
, where
opt = {
artist: artist, //req
album: album, //req
tags: tags, //req, max: 10, comma separated list
};
*Requires Authentication
album_getInfo(opt)
, where
opt = {
artist: artist, //req unless mbid
album: album, //req unless mbid
mbid: mbid, //opt
lang: lang, //opt
username: username, //opt
};
album_getTags(opt)
, where
opt = {
artist: artist, //req unless mbid
album: album, //req unless mbid
username: username, //req
mbid: mbid, //opt
};
album_getTopTags(opt)
, where
opt = {
artist: artist, //req unless mbid
album: album, //req unless mbid
mbid: mbid, //opt
};
album_removeTag(opt)
, where
opt = {
artist: artist, //req
album: album, //req
tag: tag, //req, single tag to remove
};
*Requires Authentication
album_getTopTags(opt)
, where
opt = {
album: album, //req
limit: limit, //opt, defaults to 30
page: page, //opt, defaults to 1
};
artist_addTags(opt)
, where
opt = {
artist: artist //req
tags: tags, //req, max 10, comma separated
}
*Requires Authentication
artist_getCorrection(opt)
, where
opt = {
artist: artist, //req
};
artist_getInfo(opt)
, where
opt = {
artist: artist, //req unless mbid
mbid: mbid, //opt
username: username, //opt
};
artist_getSimilar(opt)
, where
opt = {
artist: artist, //req unless mbid
mbid: mbid, //opt
limit: limit, //opt
};
artist_getTags(opt)
, where
opt = {
artist: artist, //req unless mbid
user: username, //req
mbid: mbid, //opt
};
artist_getTopAlbums(opt)
, where
opt = {
artist: artist, //req unless mbid
mbid: mbid, //opt
page: page, //opt, default is 50
limit: limit, //opt, default is 1
};
artist_getTopTags(opt)
, where
opt = {
artist: artist, //req unless mbid
mbid: mbid, //opt
};
artist_getTopTracks(opt)
, where
opt = {
artist: artist, //req unless mbid
mbid: mbid, //opt
page: page, //opt, default is 1
limit: limit, //opt, default is 50
};
artist_removeTag(opt)
, where
opt = {
artist: artist //req
tag: tag, //req, 1 tag to be removed
}
*Requires Authentication
artist_search(opt)
, where
opt = {
artist: artist, //req unless mbid
page: page, //opt, default is 1
limit: limit, //opt, default is 50
};
chart_getTopArtists(opt)
, where
opt = {
page: page, //opt, default is 1
limit: limit, //opt, default is 50
};
chart_getTopTags(opt)
, where
opt = {
page: page, //opt, default is 1
limit: limit, //opt, default is 50
};
chart_getTopTracks(opt)
, where
opt = {
page: page, //opt, default is 1
limit: limit, //opt, default is 50
};
geo_getTopArtists(opt)
, where
opt = {
country: country, //req, ISO 3166-1 format
page: page, //opt, default is 1
limit: limit, //opt, default is 50
};
geo_getTopTracks(opt)
, where
opt = {
country: country, //req, ISO 3166-1 format
page: page, //opt, default is 1
limit: limit, //opt, default is 50
};
library_getArtists(opt)
, where
opt = {
user: username, //req
page: page, //opt, default is 1
limit: limit, //opt, default is 50
};
tag_getInfo(opt)
, where
opt = {
tag: tag, //req
lang: lang, //opt
};
tag_getSimilar(opt)
, where
opt = {
tag: tag, //req
};
tag_getTopAlbums(opt)
, where
opt = {
tag: tag, //req
limit: limit, //opt, default is 50
page: page, //opt, default is 1
};
tag_getTopArtists(opt)
, where
opt = {
tag: tag, //req
limit: limit, //opt, default is 50
page: page, //opt, default is 1
};
tag_getTopTags(opt)
, where
opt = {};
tag_getTopTracks(opt)
, where
opt = {
tag: tag, //req
limit: limit, //opt, defaults to 50
page: page, //opt, defaults to 1
};
tag_getWeeklyChartList(opt)
, where
opt = {
tag: tag, //req
};
track_addTags(opt)
, where
opt = {
artist: artist, //req
track: track, //req
tags: tags, //req, max: 10
};
*Requires Authentication
track_getCorrection(opt)
, where
opt = {
artist: artist, //req
track: track, //req
};
track_getInfo(opt)
, where
opt = {
artist: artist, //req unless mbid
track: track, //req unless mbid
mbid: mbid, //opt
username: username, //opt
};
track_getSimilar(opt)
, where
opt = {
artist: artist, //req unless mbid
track: track, //req unless mbid
mbid: mbid, //opt
limit: limit, //opt
};
track_getTags(opt)
, where
opt = {
artist: artist, //req unless mbid
track: track, //req unless mbid
username: username, //req
mbid: mbid, //opt
};
track_getTopTags(opt)
, where
opt = {
artist: artist, //req unless mbid
track: track, //req unless mbid
mbid: mbid, //opt
};
track_love(opt)
, where
opt = {
artist: artist, //req unless mbid
track: track, //req unless mbid
};
*Requires Authentication
track_removeTag(opt)
, where
opt = {
artist: artist, //req
track: track, //req
tag: tag, //req, single tag to remove
};
*Requires Authentication
track_scrobble(opt)
, where
opt = {
artist: artist[i], //req
track: track[i], //req
timestamp: timestamp[i], //req
album: album[i], //opt
context: context[i], //opt
streamId: streamId[i], //opt
chosenByUser: chosenByUser[i], //opt
trackNumber: trackNumber[i], //opt
mbid: mbid[i], //opt
albumArtist: albumArtist[i], //opt
duration: duration[i], //opt
};
*Requires Authentication
track_search(opt)
, where
opt = {
track: track, //req
artist: artist, //opt
limit: limit, //opt, defaults to 30
page: page, //opt, defaults to 1
};
track_unlove(opt)
, where
opt = {
track: track, //req
artist: artist, //req
};
*Requires Authentication
track_updateNowPlaying(opt)
, where
opt = {
artist: artist, //req
track: track, //req
album: album, //opt
context: context //opt
trackNumber: trackNumber, //opt
mbid: mbid, //opt
albumArtist: albumArtist, //opt
duration: duration, //opt
}
*Requires Authentication
user_getArtistTracks(opt)
, where
opt = {
user: username, //opt
artist: artist, //req
startTimestamp: startTimestamp, //opt defaults to all time
page: page, //opt, default is 1
endTimestamp: endTimestamp, //opt defaults to all time
};
user_getFriends(opt)
, where
opt = {
user: username, //opt
recentTracks: recentTracks, //opt, true|false
limit: limit, //opt defaults to 50
page: page, //opt, default is 1
};
user_getInfo(opt)
, where
opt = {
user: username, //opt, defaults to init user
};
user_getLovedTracks(opt)
, where
opt = {
user: username, //opt
limit: limit, //opt, default is 50
page: page, //opt, default is 1
};
user_getPersonalTags(opt)
, where
opt = {
user: username, //opt
tag: tag, //req
taggingtype: artist | album | track, //req
limit: limit, //opt, default is 50
page: page, //opt, default is 1
};
user_getRecentTracks(opt)
, where
opt = {
user: username, //opt
from: startTime, //opt
extended: 0 | 1, //opt
to: endTime, //opt
limit: limit, //opt, default is 50
page: page, //opt, default is 1
};
user_getTopAlbums(opt)
, where
opt = {
user: username, //opt
period: overall|7day|1month|3month|6month|12month, //opt, default is overall
limit: limit, //opt, default is 50
page: page, //opt, default is 1
}
user_getTopArtists(opt)
, where
opt = {
user: username, //opt
period: overall|7day|1month|3month|6month|12month, //opt, default is overall
limit: limit, //opt, default is 50
page: page, //opt, default is 1
}
user_getTopTags(opt)
, where
opt = {
user: username, //opt
limit: limit, //opt, default is 50
};
user_getTopTracks(opt)
, where
opt = {
user: username, //opt
period: overall|7day|1month|3month|6month|12month, //opt, default is overall
limit: limit, //opt, default is 50
page: page, //opt, default is 1
}
user_getWeeklyAlbumChart(opt)
, where
opt = {
user: username, //opt
from: startdate, //opt, default is overall
to: enddate, //opt, default is 50
};
user_getWeeklyArtistChart(opt)
, where
opt = {
user: username, //opt
from: startdate, //opt, default is overall
to: enddate, //opt, default is 50
};
user_getWeeklyChartList(opt)
, where
opt = {
user: username, //opt
};
user_getWeeklyTrackChart(opt)
, where
opt = {
user: username, //opt
from: startdate, //opt, default is overall
to: enddate, //opt, default is 50
};
FAQs
A complete last.fm API for Node.JS
The npm package lastfm-njs receives a total of 9 weekly downloads. As such, lastfm-njs popularity was classified as not popular.
We found that lastfm-njs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Add secure dependency scanning to Claude Desktop with Socket MCP, a one-click extension that keeps your coding conversations safe from malicious packages.
Product
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
Application Security
/Security News
Socket CEO Feross Aboukhadijeh and a16z partner Joel de la Garza discuss vibe coding, AI-driven software development, and how the rise of LLMs, despite their risks, still points toward a more secure and innovative future.