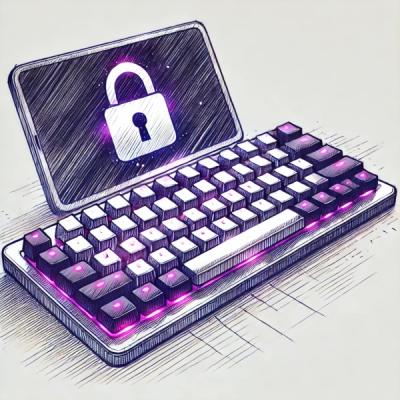
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
layer-api
Advanced tools
Readme
A Node.js library, which provides a wrapper for the Layer Platform API.
The Layer Platform API is designed to empower developers to automate, extend, and integrate functionality provided by the Layer platform with other services and applications. For more on this see our blog post.
This library supports requests from your servers only.
You can find full documentation on Platform API at developer.layer.com/docs/platform.
npm install layer-api
var LayerAPI = require('layer-api');
// Initialize by providing your Layer credentials
var layerAPI = new LayerAPI({
token: API_TOKEN,
appId: APP_ID
});
// Create a Conversation
layerAPI.conversations.create({participants: ['abcd']}, function(err, res) {
var cid = res.body.id;
// Send a Message
layerAPI.messages.sendTexFromUser(cid, 'abcd', 'Hello, World!', function(err, res) {
console.log(err || res.body);
});
});
To use this library you need to create a new instance of the layer-api
module by passing config
object to a constructor.
Layer API constructor is initialized with the following configuration values:
token
- Layer Platform API token which can be obtained from Developer DashboardappId
- Layer application IDOptional values:
version
- API version to use (default: 1.0
)timeout
- Request timeout in milliseconds (default: 10000
milliseconds)debug
- Enable debugging (default: false
)Conversations coordinate messaging within Layer and can contain up to 25 participants. All Messages sent are sent within the context of a conversation.
Create a new Conversation by providing paylod. Payload should contain at least participants
array. Optional properties are metadata
object and distinct
boolean.
payload
- Payload objectcallback(err, res)
- Optional Callback function returns an error and response objectslayerAPI.conversations.create({participants: ['abcd']}, function(err, res) {
if (err) return console.error(err);
// conversation ID
var cid = res.body.id;
});
Retrieve an existing Conversation by providing conversation ID. Response body
will result in conversation object representation.
cid
- Conversation IDcallback(err, res)
- Callback function returns an error and response objectslayerAPI.conversations.get(cid, function(err, res) {
if (err) return console.error(err);
// conversation data
var conversation = res.body;
});
Edit an existing Conversation by providing conversation ID and one or more operations
as described by the Layer Patch format.
cid
- Conversation IDoperations
- Conversation operations arraycallback(err, res)
- Optional Callback function returns an error and response objectsvar operations = [
{"operation": "add", "property": "participants", "value": "user1"}
];
layerAPI.conversations.edit(cid, operations, function(err, res) {
if (err) return console.error(err);
// conversation data
var conversation = res.body;
});
Messages can be made up of one or many individual pieces of content.
sender
can be specified by user_id
or name
, but not both.parts
are the atomic object in the Layer universe. They represent the individual pieces of content embedded within a message.notification
object represents push notification payload.Send a Message by providing conversation ID and payload.
cid
- Conversation IDpayload
- Message payload containing sender
and parts
datacallback(err, res)
- Optional Callback function returns an error and response objectsvar payload = {
sender: {
user_id: 'abcd'
},
parts: [
{
body: 'Hello, World!',
mime_type: 'text/plain'
}
]
};
layerAPI.messages.send(cid, payload, function(err, res) {
if (err) return console.error(err);
// message ID
var messageId = res.body.id;
});
Shorthand method for sending a plain text Message by providing userId
and text
.
cid
- Conversation IDuserId
- User ID of the participant that this message will appear to be fromtext
- Text or base64 encoded data for your messagecallback(err, res)
- Optional Callback function returns an error and response objectsShorthand method for sending a plain text Message by providing name
and text
.
cid
- Conversation IDname
- Arbitrary string naming the service that this message will appear to be fromtext
- Text or base64 encoded data for your messagecallback(err, res)
- Optional Callback function returns an error and response objectsAnnouncements are messages sent to all users of the application or to a list of users.
Payload property recipients
can contain one or more user IDs or the literal string "everyone" in order to message the entire userbase.
Send an Announcement by providing a payload.
payload
- Message payload containing recipients
, sender
and parts
datacallback(err, res)
- Optional Callback function returns an error and response objectsvar payload = {
recipients: ['abcd', '12345'],
sender: {
name: 'The System'
},
parts: [
{
body: 'Hello, World!',
mime_type: 'text/plain'
}
]
};
layerAPI.announcements.send(payload, function(err, res) {
if (err) return console.error(err);
// announcement data
var announcement = res.body;
});
The unit tests are based on the mocha module, which may be installed via npm. To run the tests make sure that the npm dependencies are installed by running npm install
from the project directory.
npm test
Layer API is an Open Source project maintained by Layer. Feedback and contributions are always welcome and the maintainers try to process patches as quickly as possible. Feel free to open up a Pull Request or Issue on Github.
FAQs
Unknown package
We found that layer-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.