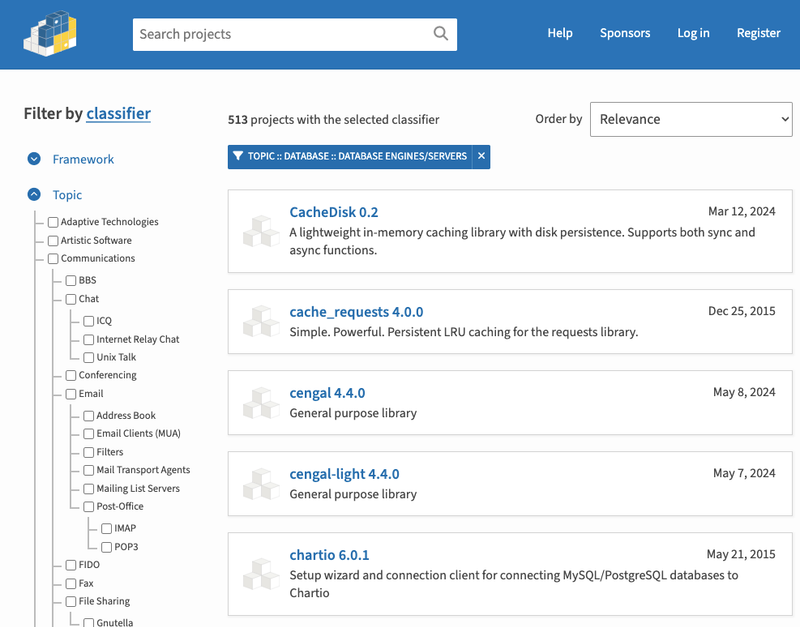
Security News
Python Software Foundation Announces 5-Year Sponsorship Commitment from Fastly
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).