Comparing version 1.2.1-alpha to 1.3.0-alpha
var Transformer = require('./../lib/transformer'); | ||
var _ = require('lodash'); | ||
module.exports = function (program, file) { | ||
var options = { | ||
transformers: {} | ||
// Enable all transformers by default | ||
var transformers = { | ||
classes: true, | ||
stringTemplates: true, | ||
arrowFunctions: true, | ||
let: true, | ||
defaultArguments: true, | ||
objectMethods: true, | ||
objectShorthands: true, | ||
noStrict: true, | ||
importCommonjs: false, | ||
exportCommonjs: false, | ||
}; | ||
// When --no-classes used, disable classes transformer | ||
if(! program.classes) { | ||
options.transformers.classes = false; | ||
transformers.classes = false; | ||
} | ||
var transformer = new Transformer(options); | ||
// When --transformers used turn off everything besides the specified tranformers | ||
if (program.transformers) { | ||
transformers = _.mapValues(transformers, _.constant(false)); | ||
program.transformers.forEach(function (name) { | ||
if (!transformers.hasOwnProperty(name)) { | ||
console.error("Unknown transformer '" + name + "'."); | ||
} | ||
transformers[name] = true; | ||
}); | ||
} | ||
// When --module=commonjs used, enable CommonJS Transformers | ||
if (program.module === 'commonjs') { | ||
transformers.importCommonjs = true; | ||
transformers.exportCommonjs = true; | ||
} | ||
else if (program.module) { | ||
console.error("Unsupported module system '" + program.module + "'."); | ||
} | ||
var transformer = new Transformer({transformers: transformers}); | ||
transformer.readFile(file[0]); | ||
@@ -14,0 +47,0 @@ transformer.applyTransformations(); |
@@ -9,4 +9,10 @@ #!/usr/bin/env node | ||
function list(val) { | ||
return val.split(','); | ||
} | ||
program.option("-o, --out-file [out]", "Compile into a single file"); | ||
program.option("--no-classes", "Don't convert function/prototypes into classes"); | ||
program.option("-t, --transformers [a,b,c]", "Perform only specified transforms", list); | ||
program.option("--module [commonjs]", "Transform CommonJS module syntax"); | ||
program.description(pkg.description); | ||
@@ -29,3 +35,3 @@ program.version(pkg.version); | ||
if (!program.outFile) { | ||
if (!program.outFile || program.outFile === true) { | ||
program.outFile = 'output.js'; | ||
@@ -32,0 +38,0 @@ } |
@@ -43,15 +43,10 @@ 'use strict'; | ||
_createClass(BlockStatement, [{ | ||
key: 'appendToBody', | ||
value: function appendToBody(statement) { | ||
this.body.push(statement); | ||
} | ||
/** | ||
* Check if an object is representing a BlockStatement | ||
* | ||
* @param object | ||
* @returns {boolean} | ||
*/ | ||
/** | ||
* Check if an object is representing a BlockStatement | ||
* | ||
* @param object | ||
* @returns {boolean} | ||
*/ | ||
}], [{ | ||
_createClass(BlockStatement, null, [{ | ||
key: 'is', | ||
@@ -58,0 +53,0 @@ value: function is(object) { |
@@ -7,4 +7,2 @@ 'use strict'; | ||
var _createClass = (function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ('value' in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; })(); | ||
var _get = function get(_x, _x2, _x3) { var _again = true; _function: while (_again) { var object = _x, property = _x2, receiver = _x3; _again = false; if (object === null) object = Function.prototype; var desc = Object.getOwnPropertyDescriptor(object, property); if (desc === undefined) { var parent = Object.getPrototypeOf(object); if (parent === null) { return undefined; } else { _x = parent; _x2 = property; _x3 = receiver; _again = true; desc = parent = undefined; continue _function; } } else if ('value' in desc) { return desc.value; } else { var getter = desc.get; if (getter === undefined) { return undefined; } return getter.call(receiver); } } }; | ||
@@ -54,9 +52,2 @@ | ||
_createClass(FunctionExpression, [{ | ||
key: 'appendToBody', | ||
value: function appendToBody(statement) { | ||
this.body.appendToBody(statement); | ||
} | ||
}]); | ||
return FunctionExpression; | ||
@@ -63,0 +54,0 @@ })(_baseJs2['default']); |
@@ -45,9 +45,5 @@ 'use strict'; | ||
// The raw version is as it looks in source, with escapes added | ||
_createClass(TemplateElement, [{ | ||
key: 'setValue', | ||
value: function setValue(value) { | ||
this.setRaw(value); | ||
this.setCooked(value); | ||
} | ||
}, { | ||
key: 'setRaw', | ||
@@ -57,2 +53,4 @@ value: function setRaw(raw) { | ||
} | ||
// The cooked varsion is the actual value | ||
}, { | ||
@@ -59,0 +57,0 @@ key: 'setCooked', |
@@ -62,6 +62,8 @@ 'use strict'; | ||
var element = new _templateElementJs2['default'](); | ||
curr = curr.value; | ||
var currVal = curr.value; | ||
var currRaw = this.escapeForTemplate(curr.raw); | ||
while (_utilsTypeCheckerJs2['default'].isString(parts[++i])) { | ||
curr += parts[i].value; | ||
currVal += parts[i].value; | ||
currRaw += this.escapeForTemplate(parts[i].raw); | ||
} | ||
@@ -71,3 +73,4 @@ | ||
element.setValue(curr); | ||
element.setCooked(currVal); | ||
element.setRaw(currRaw); | ||
this.quasis.push(element); | ||
@@ -93,2 +96,9 @@ } else { | ||
} | ||
// Strip surrounding quotes, escape backticks and unescape escaped quotes | ||
}, { | ||
key: 'escapeForTemplate', | ||
value: function escapeForTemplate(raw) { | ||
return raw.replace(/^['"]|['"]$/g, '').replace(/`/g, '\\`').replace(/\\(['"])/g, '$1'); | ||
} | ||
}]); | ||
@@ -95,0 +105,0 @@ |
@@ -13,10 +13,6 @@ 'use strict'; | ||
var _syntaxArrowExpressionJs = require('./../syntax/arrow-expression.js'); | ||
var _syntaxArrowFunctionExpressionJs = require('./../syntax/arrow-function-expression.js'); | ||
var _syntaxArrowExpressionJs2 = _interopRequireDefault(_syntaxArrowExpressionJs); | ||
var _syntaxArrowFunctionExpressionJs2 = _interopRequireDefault(_syntaxArrowFunctionExpressionJs); | ||
var _lodash = require('lodash'); | ||
var _lodash2 = _interopRequireDefault(_lodash); | ||
exports['default'] = function (ast) { | ||
@@ -28,86 +24,56 @@ _estraverse2['default'].replace(ast, { | ||
function callBackToArrow(node, parent) { | ||
if (node.type === 'FunctionExpression' && parent.type === 'CallExpression' && !hasThis(node)) { | ||
var arrow = new _syntaxArrowExpressionJs2['default'](); | ||
arrow.body = node.body; | ||
arrow.params = node.params; | ||
arrow.rest = node.rest; | ||
arrow.defaults = node.defaults; | ||
arrow.generator = node.generator; | ||
arrow.id = node.id; | ||
return arrow; | ||
function callBackToArrow(node) { | ||
if (isFunctionConvertableToArrow(node)) { | ||
return new _syntaxArrowFunctionExpressionJs2['default']({ | ||
body: extractArrowBody(node.body), | ||
params: node.params, | ||
defaults: node.defaults, | ||
rest: node.rest | ||
}); | ||
} | ||
} | ||
var objectProps = ['body', 'expression', 'left', 'right', 'object']; | ||
function isFunctionConvertableToArrow(node) { | ||
return node.type === 'FunctionExpression' && !node.id && !node.generator && !hasThis(node.body) && !hasArguments(node.body); | ||
} | ||
function hasThis(node) { | ||
function hasThis(ast) { | ||
return hasInFunctionBody(ast, function (node) { | ||
return node.type === 'ThisExpression'; | ||
}); | ||
} | ||
if (_lodash2['default'].isArray(node)) { | ||
var _iteratorNormalCompletion = true; | ||
var _didIteratorError = false; | ||
var _iteratorError = undefined; | ||
function hasArguments(ast) { | ||
return hasInFunctionBody(ast, function (node) { | ||
return node.type === 'Identifier' && node.name === 'arguments'; | ||
}); | ||
} | ||
try { | ||
for (var _iterator = node[Symbol.iterator](), _step; !(_iteratorNormalCompletion = (_step = _iterator.next()).done); _iteratorNormalCompletion = true) { | ||
var sub = _step.value; | ||
// Returns true when predicate matches any node in given function body, | ||
// excluding any nested functions | ||
function hasInFunctionBody(ast, predicate) { | ||
var found = false; | ||
var result = hasThis(sub); | ||
if (result) { | ||
return result; | ||
} | ||
_estraverse2['default'].traverse(ast, { | ||
enter: function enter(node) { | ||
if (predicate(node)) { | ||
found = true; | ||
this['break'](); | ||
} | ||
} catch (err) { | ||
_didIteratorError = true; | ||
_iteratorError = err; | ||
} finally { | ||
try { | ||
if (!_iteratorNormalCompletion && _iterator['return']) { | ||
_iterator['return'](); | ||
} | ||
} finally { | ||
if (_didIteratorError) { | ||
throw _iteratorError; | ||
} | ||
if (node.type === 'FunctionExpression' || node.type === 'FunctionDeclaration') { | ||
this.skip(); | ||
} | ||
} | ||
}); | ||
return false; | ||
} | ||
return found; | ||
} | ||
if (node.type === 'ThisExpression') { | ||
return true; | ||
function extractArrowBody(block) { | ||
if (block.body[0] && block.body[0].type === 'ReturnStatement') { | ||
return block.body[0].argument; | ||
} else { | ||
return block; | ||
} | ||
var _iteratorNormalCompletion2 = true; | ||
var _didIteratorError2 = false; | ||
var _iteratorError2 = undefined; | ||
try { | ||
for (var _iterator2 = objectProps[Symbol.iterator](), _step2; !(_iteratorNormalCompletion2 = (_step2 = _iterator2.next()).done); _iteratorNormalCompletion2 = true) { | ||
var prop = _step2.value; | ||
if (node[prop]) { | ||
return hasThis(node[prop]); | ||
} | ||
} | ||
} catch (err) { | ||
_didIteratorError2 = true; | ||
_iteratorError2 = err; | ||
} finally { | ||
try { | ||
if (!_iteratorNormalCompletion2 && _iterator2['return']) { | ||
_iterator2['return'](); | ||
} | ||
} finally { | ||
if (_didIteratorError2) { | ||
throw _iteratorError2; | ||
} | ||
} | ||
} | ||
return false; | ||
} | ||
module.exports = exports['default']; |
@@ -29,4 +29,4 @@ 'use strict'; | ||
if (node.type === 'AssignmentExpression') { | ||
var left = node.left.name || node.left.property.name; | ||
if (node.type === 'AssignmentExpression' && node.left.type === 'Identifier') { | ||
var left = node.left.name; | ||
@@ -36,3 +36,3 @@ if (declarations[left]) { | ||
} | ||
} else if (node.type === 'UpdateExpression') { | ||
} else if (node.type === 'UpdateExpression' && node.argument.type === 'Identifier') { | ||
var _name = node.argument.name; | ||
@@ -39,0 +39,0 @@ if (declarations[_name]) { |
@@ -17,5 +17,5 @@ 'use strict'; | ||
var _lodashObjectMergeJs = require('lodash/object/merge.js'); | ||
var _lodash = require('lodash'); | ||
var _lodashObjectMergeJs2 = _interopRequireDefault(_lodashObjectMergeJs); | ||
var _lodash2 = _interopRequireDefault(_lodash); | ||
@@ -60,2 +60,31 @@ var _recast = require('recast'); | ||
var _transformationObjectShorthandsJs = require('./transformation/object-shorthands.js'); | ||
var _transformationObjectShorthandsJs2 = _interopRequireDefault(_transformationObjectShorthandsJs); | ||
var _transformationNoStrictJs = require('./transformation/no-strict.js'); | ||
var _transformationNoStrictJs2 = _interopRequireDefault(_transformationNoStrictJs); | ||
var _transformationImportCommonjsJs = require('./transformation/import-commonjs.js'); | ||
var _transformationImportCommonjsJs2 = _interopRequireDefault(_transformationImportCommonjsJs); | ||
var _transformationExportCommonjsJs = require('./transformation/export-commonjs.js'); | ||
var _transformationExportCommonjsJs2 = _interopRequireDefault(_transformationExportCommonjsJs); | ||
var tranformersMap = { | ||
classes: _transformationClassesJs2['default'], | ||
stringTemplates: _transformationTemplateStringJs2['default'], | ||
arrowFunctions: _transformationArrowFunctionsJs2['default'], | ||
'let': _transformationLetJs2['default'], | ||
defaultArguments: _transformationDefaultArgumentsJs2['default'], | ||
objectMethods: _transformationObjectMethodsJs2['default'], | ||
objectShorthands: _transformationObjectShorthandsJs2['default'], | ||
noStrict: _transformationNoStrictJs2['default'], | ||
importCommonjs: _transformationImportCommonjsJs2['default'], | ||
exportCommonjs: _transformationExportCommonjsJs2['default'] | ||
}; | ||
var Transformer = (function () { | ||
@@ -73,41 +102,18 @@ | ||
this.ast = {}; | ||
this.options = (0, _lodashObjectMergeJs2['default'])(this.constructor.defaultOptions, options); | ||
this.transformations = []; | ||
this.options = options; | ||
this.prepareTransformations(); | ||
this.transformations = (0, _lodash2['default'])(options.transformers).pick(function (enabled) { | ||
return enabled; | ||
}).map(function (enabled, key) { | ||
return tranformersMap[key]; | ||
}).value(); | ||
} | ||
/** | ||
* Prepare transformations array by give options | ||
* Prepare the abstract syntax tree for given file | ||
* | ||
* @param filename | ||
*/ | ||
_createClass(Transformer, [{ | ||
key: 'prepareTransformations', | ||
value: function prepareTransformations() { | ||
var _this = this; | ||
var shouldTransform = function shouldTransform(key) { | ||
return typeof _this.options.transformers[key] !== 'undefined' && _this.options.transformers[key]; | ||
}; | ||
var doTransform = function doTransform(key, transformation) { | ||
if (shouldTransform(key)) { | ||
_this.transformations.push(transformation); | ||
} | ||
}; | ||
doTransform('classes', _transformationClassesJs2['default']); | ||
doTransform('stringTemplates', _transformationTemplateStringJs2['default']); | ||
doTransform('arrowFunctions', _transformationArrowFunctionsJs2['default']); | ||
doTransform('let', _transformationLetJs2['default']); | ||
doTransform('defaultArguments', _transformationDefaultArgumentsJs2['default']); | ||
doTransform('objectMethods', _transformationObjectMethodsJs2['default']); | ||
} | ||
/** | ||
* Prepare the abstract syntax tree for given file | ||
* | ||
* @param filename | ||
*/ | ||
}, { | ||
key: 'readFile', | ||
@@ -169,3 +175,3 @@ value: function readFile(filename) { | ||
if (this.options.formatter !== false) { | ||
if (this.options.formatter) { | ||
result = _esformatter2['default'].format(result, this.options.formatter); | ||
@@ -201,14 +207,2 @@ } | ||
exports['default'] = Transformer; | ||
Transformer.defaultOptions = { | ||
transformers: { | ||
classes: true, | ||
stringTemplates: true, | ||
arrowFunctions: true, | ||
'let': true, | ||
defaultArguments: true, | ||
objectMethods: true | ||
}, | ||
formatter: false | ||
}; | ||
module.exports = exports['default']; |
{ | ||
"name": "lebab", | ||
"version": "1.2.1-alpha", | ||
"version": "1.3.0-alpha", | ||
"description": "Turn your ES5 code into readable ES6", | ||
@@ -33,16 +33,16 @@ "main": "index.js", | ||
"dependencies": { | ||
"babel": "^5.0.12", | ||
"coffee-script": "^1.9.1", | ||
"commander": "^2.6.0", | ||
"babel": "^5.8.34", | ||
"coffee-script": "^1.10.0", | ||
"commander": "^2.9.0", | ||
"esformatter": "^0.6.1", | ||
"estraverse": "^1.9.1", | ||
"esutils": "^1.1.6", | ||
"lodash": "^3.3.1", | ||
"recast": "^0.10.39" | ||
"estraverse": "^4.1.1", | ||
"esutils": "^2.0.2", | ||
"lodash": "^3.10.1", | ||
"recast": "^0.11.0" | ||
}, | ||
"devDependencies": { | ||
"chai": "^2.2.0", | ||
"chai": "^3.4.1", | ||
"grunt": "^0.4.5", | ||
"grunt-babel": "^4.0.0", | ||
"grunt-contrib-clean": "^0.6.0", | ||
"grunt-babel": "^5.0.3", | ||
"grunt-contrib-clean": "^0.7.0", | ||
"grunt-contrib-jshint": "^0.11.1", | ||
@@ -52,4 +52,4 @@ "grunt-contrib-watch": "^0.6.1", | ||
"load-grunt-tasks": "^3.1.0", | ||
"mocha": "^2.2.1" | ||
"mocha": "^2.3.4" | ||
} | ||
} |
@@ -1,37 +0,9 @@ | ||
[](http://travis-ci.org/mohebifar/xto6) [](http://mohebifar.mit-license.org) [](http://js.org) | ||
[](http://travis-ci.org/mohebifar/lebab) [](http://mohebifar.mit-license.org) [](http://js.org) | ||
# Lebab | ||
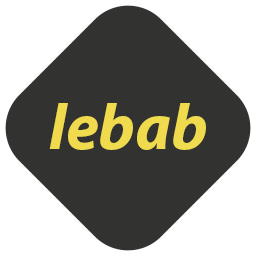 | ||
 | ||
**lebab** transpiles your ES5 code to ES6. It does exactly the opposite of what other transpilers do (like babel and traceur)! If you want to understand what lebab exactly does, [try the live demo](http://xto6.js.org/#try-live). | ||
**lebab** transpiles your ES5 code to ES2015. It does exactly the opposite of what babel does. If you want to understand what lebab exactly does, [try the live demo](http://lebab.io/try-it). | ||
## Why? | ||
Still coding this way? Think twice! | ||
```js | ||
var SomeClass = function () { | ||
console.log('This is the constructor.'); | ||
}; | ||
SomeClass.prototype.someOuterMethod = someFunction; | ||
SomeClass.prototype.someInnerMethod = function (birthYear) { | ||
var result = 'Your Age is : ' + (2015 - birthYear) + ' and you were born in ' + birthYear; | ||
return result; | ||
}; | ||
Object.defineProperty(SomeClass.prototype, 'someAccessor', { | ||
get: function () { | ||
return this._some; | ||
}, | ||
set: function (value) { | ||
this._some = value; | ||
} | ||
}); | ||
function someFunction(a, b) { | ||
return a + b; | ||
} | ||
``` | ||
## Usage | ||
@@ -49,33 +21,2 @@ Install it using npm : | ||
And the result for the code above is : | ||
```js | ||
class SomeClass { | ||
constructor() { | ||
console.log('This is the constructor.'); | ||
} | ||
someOuterMethod() { | ||
return someFunction.apply(this, arguments); | ||
} | ||
someInnerMethod(birthYear) { | ||
var result = `Your Age is : ${ 2015 - birthYear } and you were born in ${ birthYear }`; | ||
return result; | ||
} | ||
get someAccessor() { | ||
return this._some; | ||
} | ||
set someAccessor(value) { | ||
this._some = value; | ||
} | ||
} | ||
function someFunction(a, b) { | ||
return a + b; | ||
} | ||
``` | ||
## Supported Features | ||
@@ -89,1 +30,6 @@ | ||
* Function properties in objects to Object methods | ||
## Roadmap | ||
- [ ] For of loops | ||
- [ ] Convert arguments to rest parameters | ||
- [ ] Lexical this for arrow functions |
@@ -13,2 +13,6 @@ var expect = require('chai').expect; | ||
function expectNoChange(script) { | ||
expect(test(script)).to.equal(script); | ||
} | ||
describe('Callback to Arrow transformation', function () { | ||
@@ -19,3 +23,3 @@ | ||
expect(test(script)).to.equal('setTimeout(() => { return 2; });'); | ||
expect(test(script)).to.equal('setTimeout(() => 2);'); | ||
}); | ||
@@ -26,24 +30,85 @@ | ||
expect(test(script)).to.equal('a(b => { return b; });'); | ||
expect(test(script)).to.equal('a(b => b);'); | ||
}); | ||
it('should convert callbacks with a single argument', function () { | ||
it('should convert callbacks with multiple arguments', function () { | ||
var script = 'a(function(b, c) { return b; });'; | ||
expect(test(script)).to.equal('a((b, c) => { return b; });'); | ||
expect(test(script)).to.equal('a((b, c) => b);'); | ||
}); | ||
it('should convert function assignment', function () { | ||
var script = 'x = function () { foo(); };'; | ||
it('shouldn\'t convert other forms of functions', function () { | ||
var script = 'var x = function () {};'; | ||
expect(test(script)).to.equal('x = () => { foo(); };'); | ||
}); | ||
expect(test(script)).to.equal(script); | ||
it('should convert immediate function invocation', function () { | ||
var script = '(function () { foo(); }());'; | ||
expect(test(script)).to.equal('((() => { foo(); })());'); | ||
}); | ||
it('shouldn\'t convert functions using `this` keyword', function () { | ||
var script = 'a(function (b) { this.x = 2; });'; | ||
it('should convert returning of a function', function () { | ||
var script = 'function foo () { return function() { foo(); }; }'; | ||
expect(test(script)).to.equal(script); | ||
expect(test(script)).to.equal('function foo () { return () => { foo(); }; }'); | ||
}); | ||
}); | ||
it('should convert functions using `this` keyword inside a nested function', function () { | ||
var script = 'a(function () { return function() { this; }; });'; | ||
expect(test(script)).to.equal('a(() => function() { this; });'); | ||
}); | ||
it('should convert functions using `arguments` inside a nested function', function () { | ||
var script = 'a(function () { return function() { arguments; }; });'; | ||
expect(test(script)).to.equal('a(() => function() { arguments; });'); | ||
}); | ||
it('should preserve default parameters', function () { | ||
var script = 'foo(function (a=1, b=2, c) { });'; | ||
expect(test(script)).to.equal('foo((a=1, b=2, c) => { });'); | ||
}); | ||
it('should preserve rest parameters', function () { | ||
var script = 'foo(function (x, ...xs) { });'; | ||
expect(test(script)).to.equal('foo((x, ...xs) => { });'); | ||
}); | ||
it('should not convert function declarations', function () { | ||
expectNoChange('function foo() {};'); | ||
}); | ||
it('should not convert named function expressions', function () { | ||
expectNoChange('f = function fact(n) { return n * fact(n-1); };'); | ||
}); | ||
it('should not convert generators', function () { | ||
expectNoChange('f = function* (n) { };'); | ||
}); | ||
it('should not convert functions using `this` keyword', function () { | ||
expectNoChange('a(function () { this; });'); | ||
expectNoChange('a(function () { this.x = 2; });'); | ||
expectNoChange('a(function () { this.bar(); });'); | ||
expectNoChange('a(function () { foo(this); });'); | ||
expectNoChange('a(function () { foo(this.bar); });'); | ||
expectNoChange('a(function () { return this; });'); | ||
expectNoChange('a(function () { if (x) foo(this); });'); | ||
expectNoChange('a(function () { for (x of foo) { bar(this); } });'); | ||
}); | ||
it('should not convert functions using `arguments`', function () { | ||
expectNoChange('a(function () { arguments; });'); | ||
expectNoChange('a(function () { foo(arguments); });'); | ||
expectNoChange('a(function () { return arguments[0] + 1; });'); | ||
expectNoChange('a(function () { return Array.slice.apply(arguments); });'); | ||
expectNoChange('a(function () { if (x) foo(arguments); });'); | ||
}); | ||
}); |
var expect = require('chai').expect; | ||
var | ||
Transformer = require('./../../lib/transformer'), | ||
transformer = new Transformer({formatter: false}); | ||
transformer = new Transformer({formatter: false, transformers: {let: true}}); | ||
@@ -6,0 +6,0 @@ function test(script) { |
@@ -43,2 +43,13 @@ var expect = require('chai').expect; | ||
}); | ||
// Issue 53 | ||
it('should use const when similarly-named property is assigned to', function () { | ||
var script = 'var a = 0; b.a += 1;'; | ||
expect(test(script)).to.equal('const a = 0; b.a += 1;'); | ||
}); | ||
it('should use const when similarly-named property is updated', function () { | ||
var script = 'var a = 0; b.a++;'; | ||
expect(test(script)).to.equal('const a = 0; b.a++;'); | ||
}); | ||
}); |
@@ -51,2 +51,29 @@ var expect = require('chai').expect; | ||
it('should escape ` characters', function () { | ||
var script = "var result = 'Firstname: `' + firstname + '`';"; | ||
expect(test(script)).to.equal('var result = `Firstname: \\`${firstname}\\``;'); | ||
}); | ||
it('should leave \\t, \\r, \\n, \\v, \\f, \\b, \\0, \\\\ escaped as is', function () { | ||
expect(test("x = '\\t' + y;")).to.equal('x = `\\t${y}`;'); | ||
expect(test("x = '\\r' + y;")).to.equal('x = `\\r${y}`;'); | ||
expect(test("x = '\\n' + y;")).to.equal('x = `\\n${y}`;'); | ||
expect(test("x = '\\v' + y;")).to.equal('x = `\\v${y}`;'); | ||
expect(test("x = '\\f' + y;")).to.equal('x = `\\f${y}`;'); | ||
expect(test("x = '\\b' + y;")).to.equal('x = `\\b${y}`;'); | ||
expect(test("x = '\\0' + y;")).to.equal('x = `\\0${y}`;'); | ||
expect(test("x = '\\\\' + y;")).to.equal('x = `\\\\${y}`;'); | ||
}); | ||
it('should leave octal-, hex-, unicode-escapes as is', function () { | ||
expect(test("x = '\\251' + y;")).to.equal('x = `\\251${y}`;'); | ||
expect(test("x = '\\xA9' + y;")).to.equal('x = `\\xA9${y}`;'); | ||
expect(test("x = '\\u00A9' + y;")).to.equal('x = `\\u00A9${y}`;'); | ||
}); | ||
it('should eliminate escaping of quotes', function () { | ||
expect(test("x = '\\'' + y;")).to.equal("x = `'${y}`;"); | ||
expect(test('x = "\\"" + y;')).to.equal('x = `"${y}`;'); | ||
}); | ||
}); |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
111313
54
2160
1282
34
+ Addedestraverse@4.3.0(transitive)
- Removedestraverse@1.9.3(transitive)
- Removedesutils@1.1.6(transitive)
Updatedbabel@^5.8.34
Updatedcoffee-script@^1.10.0
Updatedcommander@^2.9.0
Updatedestraverse@^4.1.1
Updatedesutils@^2.0.2
Updatedlodash@^3.10.1
Updatedrecast@^0.11.0