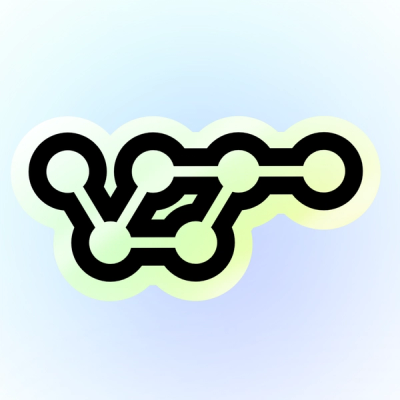
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
A light-weight JavaScript library for using LIFX HTTP API.
Read your lights, turn them on or off, change their colors and activate your favorite scene.
To use lifxjs
, you need to obtain a valid OAuth 2 access token first.
Install with npm:
npm install --save lifxjs
Install with yarn:
yarn add lifxjs
const Lifx = require('lifxjs');
const lifx = new Lifx();
lifx.init({ appToken: 'APP_TOKEN' });
(async function () {
// get all lights for the given access token
const lights = await lifx.get.all();
// find the light you are searching for
const officeBulb = lights.find(function (light) {
return light.label === 'Office Bulb';
});
// turn the light on
await lifx.power.light(officeBulb.id, 'on');
// set its color to a hue value...
await lifx.color.light(officeBulb.id, {
hue: 273,
saturation: 1,
brightness: 1
});
// ...or to a kelvin value
await lifx.color.light(officeBulb.id, {
kelvin: 3500,
brightness: 1
});
// turn it off when the job is done...
await lifx.power.light(officeBulb.id, 'off');
// ...or turn all the lights off instead
await lifx.power.all('off');
})();
(async function () {
// get all scenes for the given access token
const scenes = await lifx.get.scenes();
// find the scene you are searching for
const movieScene = scenes.find(function (scene) {
return scene.name === 'Sci-Fi Movie Scene';
});
// activate the scene
await lifx.scene.activate(movieScene.uuid);
})();
lifx.init(options)
To initialize the library and then be able to use the features, you first have to invoke .init()
and pass options
object as a parameter with the following properties:
Property | Details |
---|---|
appToken: string | How to obtain a LIFX Oauth2 Token |
lifx.get
Method | Parameters | Response |
---|---|---|
all() | None | List Lights |
light(id) | id: string | List Lights |
group(id) | id: string | List Lights |
location(id) | id: string | List Lights |
scenes() | None | List Scenes |
lifx.power
Method | Parameters | Response |
---|---|---|
all(status, duration?) | status: 'on' | 'off' duration: number (default: 1 ) | Set State |
light(id, status, duration?) | id: string status: 'on' | 'off' duration: number (default: 1 ) | Set State |
group(id, status, duration?) | id: string status: 'on' | 'off' duration: number (default: 1 ) | Set State |
location(id, status, duration?) | id: string status: 'on' | 'off' duration: number (default: 1 ) | Set State |
lifx.color
Method | Parameters | Response |
---|---|---|
all(color, wakeup?, duration?) | color: LifxColorConfig wakeup: boolean (default: true ) duration: number (default: 1 ) | Set State |
light(id, color, wakeup?, duration?) | id: string color: LifxColorConfig wakeup: boolean (default: true ) duration: number (default: 1 ) | Set State |
group(id, color, wakeup?, duration?) | id: string color: LifxColorConfig wakeup: boolean (default: true ) duration: number (default: 1 ) | Set State |
location(id, color, wakeup?, duration?) | id: string color: LifxColorConfig wakeup: boolean (default: true ) duration: number (default: 1 ) | Set State |
The LifxColorConfig
may have the following properties:
Property | Example |
---|---|
hex: string | hex: '#ff000' |
rgb: string | rgb: '255,255,0' |
hue: [0-360] | hue: 273 |
saturation: [0.0-1.0] | saturation: 1 |
kelvin: [1500-9000] | kelvin: 3500 |
brightness: [0.0-1.0] | brightness: 0.6 |
Please note when using LifxColorConfig
:
hex
nor rgb
can be combined with hue
, saturation
, kelvin
and brightness
.hue
, saturation
, kelvin
, and brightness
values can be combined to describe the desired color. Read more.lifx.scene
Method | Parameters | Response |
---|---|---|
activate(uuid) | uuid: string | Activate Scene |
Run tests once:
yarn test
Run tests with watch option
yarn test:watch
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
Please make sure to update tests as appropriate.
1.1.0 (2021-01-16)
Features:
duration
support when changing color and power state (#9)Housekeeping:
FAQs
A light-weight JavaScript library for using LIFX HTTP API
The npm package lifxjs receives a total of 1 weekly downloads. As such, lifxjs popularity was classified as not popular.
We found that lifxjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.