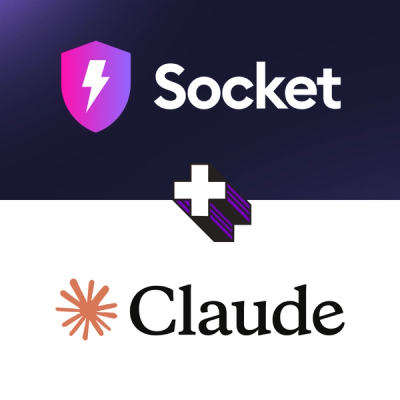
Product
Introducing Socket MCP for Claude Desktop
Add secure dependency scanning to Claude Desktop with Socket MCP, a one-click extension that keeps your coding conversations safe from malicious packages.
light-event-bus
Advanced tools
event-bus.js is a lightweight event bus for Node.js and the browser.
This library can be used both with Node.js and the browser.
You can download the library here. For the browser there are 2 choiches:
event-bus.min.js
and import it in your app using the <script>
tag.npm install event-bus
or
yarn add event-bus
Or download event-bus.module.min.js
and import it using ES6 imports.
These two files are also delivered through a CDN at these addresses:
event-bus.min.js
: addurlevent-bus.module.min.js
: addurl<script src='../build/event-bus.min.js'></script>
<script>
const eventBus = new EVENT_BUS.EventBus()
const subscription = eventBus.subscribe('event', arg => console.log(arg))
eventBus.publish('event', 'message')
subscription.unsubscribe()
</script>
<script type='module'>
// if you are using npm/yarn
import { EventBus } from 'event-bus'
// if you have downloaded the file manually
import { EventBus } from '../build/event-bus.module.min.js'
const eventBus = new EventBus()
const subscription = eventBus.subscribe('event', arg => console.log(arg))
eventBus.publish('event', 'message')
subscription.unsubscribe()
</script>
Run
npm install event-bus
or
yarn add event-bus
const { EventBus } = require('event-bus')
const eventBus = new EventBus()
const subscription = eventBus.subscribe('event', arg => console.log(arg))
eventBus.publish('event', 'message')
subscription.unsubscribe()
The EventBus
constructor can be accessed through the main object exposed by this library.
If you are using Node.js:
const { EventBus } = require('event-bus')
If you are using ES6 imports:
import { EventBus } from 'event-bus'
If you are using <script>
:
const eventBus = new EVENT_BUS.EventBus()
Instances of EventBus
expose a subscribe
method.
Call subscribe
when you want to start listening for an event.
subscribe
takes two arguments: eventType
and callback
.
Example:
eventBus.subscribe('event', arg => console.log(arg))
eventType
can be an object of any type. Strings are recomended.callback
must be a function. This function can have 0 or 1 argument.subscribe
returns an object that exposes an unsubscribe
method.
Call unsubscribe
to cancel the current subscription.
Example:
const subscription = eventBus.subscribe('event', arg => console.log(arg))
// To cancel the subscription
subscription.unsubscribe()
Instances of EventBus
expose a publish
method.
Call publish
when you want to publish an event on the event bus.
publish
takes two arguments: eventType
and argument
.
Example:
eventBus.publish('event', 'message')
eventType
can be an object of any type. Strings are recomended.argument
is optional. It will be passed to all the listeners for the event. It can be of any type.In the case of this examples, only subscribers of the event 'event'
will be called, with the string 'mesage'
as argument.
Often a single instance of EventBus
is needed across an entire application, a singleton. The main object of this library already exposes a singleton, so that you don't have to create your own.
You can access the EventBusSingleton
in the following ways:
If you are using Node.js:
const { EventBusSingleton } = require('event-bus')
If you are using ES6 imports:
import { EventBusSingleton } from 'event-bus'
If you are using <script>
:
const eventBus = new EVENT_BUS.EventBusSingleton()
This singleton is just an instance of EventBus
, so it can be used as any other user-defined EventBus
.
FAQs
Lightweight event bus for node and the browser.
The npm package light-event-bus receives a total of 373 weekly downloads. As such, light-event-bus popularity was classified as not popular.
We found that light-event-bus demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Add secure dependency scanning to Claude Desktop with Socket MCP, a one-click extension that keeps your coding conversations safe from malicious packages.
Product
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
Application Security
/Security News
Socket CEO Feross Aboukhadijeh and a16z partner Joel de la Garza discuss vibe coding, AI-driven software development, and how the rise of LLMs, despite their risks, still points toward a more secure and innovative future.