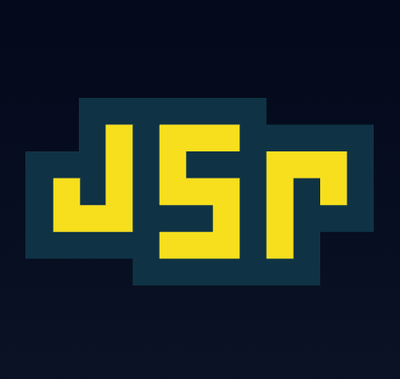
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The lilconfig npm package is a lightweight utility for loading configuration files for Node.js projects. It allows developers to search for and read configuration files in various formats from the file system, providing a simple API to work with project configurations.
Searching for configuration files
This feature allows you to search for configuration files related to 'myapp' in the project directory and its parent directories. The search method returns a promise that resolves with the result object containing the file path and its contents if found.
const lilconfig = require('lilconfig');
lilconfig('myapp').search().then(result => {
console.log(result);
});
Loading configuration from a specific file
This feature allows you to load configuration from a specific file. The load method returns a promise that resolves with the result object containing the file path and its parsed contents.
const lilconfig = require('lilconfig');
lilconfig('myapp').load('path/to/config.json').then(result => {
console.log(result);
});
Custom loaders for different file formats
This feature allows you to define custom loaders for different file formats. In this example, a custom loader for YAML files is provided using the 'yaml' npm package. The search method will use this loader when a '.yaml' file is encountered.
const lilconfig = require('lilconfig');
const yaml = require('yaml');
const loaders = {
'.yaml': async filepath => {
const content = await fs.promises.readFile(filepath, 'utf8');
return yaml.parse(content);
}
};
lilconfig('myapp', { loaders }).search().then(result => {
console.log(result);
});
Cosmiconfig is a similar package that searches for and loads configuration files. It supports various file formats and has a larger feature set, including caching and transforming configurations. It is more widely used than lilconfig but is also more complex and heavier.
The 'rc' package is another alternative that loads configuration from multiple sources, including command-line arguments, environment variables, and configuration files. It is less focused on file discovery and more on aggregating configuration from different sources.
The 'config' package is designed for managing configurations across different deployment environments. It is more opinionated and structured than lilconfig, with a predefined way of organizing configuration files based on the deployment environment.
A zero-dependency alternative to cosmiconfig with the same API.
npm install lilconfig
import {lilconfig, lilconfigSync} from 'lilconfig';
// all keys are optional
const options = {
stopDir: '/Users/you/some/dir',
searchPlaces: ['package.json', 'myapp.conf.js'],
ignoreEmptySearchPlaces: false
}
lilconfig(
'myapp',
options // optional
).search() // Promise<LilconfigResult>
lilconfigSync(
'myapp',
options // optional
).load(pathToConfig) // LilconfigResult
/**
* LilconfigResult
* {
* config: any; // your config
* filepath: string;
* }
*/
ESM configs can be loaded with async API only. Specifically js
files in projects with "type": "module"
in package.json
or mjs
files.
cosmiconfig
Lilconfig does not intend to be 100% compatible with cosmiconfig
but tries to mimic it where possible. The key difference is no support for yaml files out of the box(lilconfig
attempts to parse files with no extension as JSON instead of YAML). You can still add the support for YAML files by providing a loader, see an example below.
cosmiconfig option | lilconfig |
---|---|
cache | ✅ |
loaders | ✅ |
ignoreEmptySearchPlaces | ✅ |
packageProp | ✅ |
searchPlaces | ✅ |
stopDir | ✅ |
transform | ✅ |
If you need the YAML support you can provide your own loader
import {lilconfig} from 'lilconfig';
import yaml from 'yaml';
function loadYaml(filepath, content) {
return yaml.parse(content);
}
const options = {
loaders: {
'.yaml': loadYaml,
'.yml': loadYaml,
// loader for files with no extension
noExt: loadYaml
}
};
lilconfig('myapp', options)
.search()
.then(result => {
result // {config, filepath}
});
FAQs
A zero-dependency alternative to cosmiconfig
We found that lilconfig demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.