Comparing version 1.0.6 to 1.1.0
97
index.js
@@ -1,4 +0,4 @@ | ||
const chalk = require('chalk'); | ||
var chalk = require('chalk'); | ||
let m = { | ||
var m = { | ||
// default | ||
@@ -17,3 +17,3 @@ d: function(msg) { | ||
} else { | ||
console.log(chalk.bgRed(msg)); | ||
console.log(chalk.bgRed.white(msg)); | ||
} | ||
@@ -45,2 +45,93 @@ }, | ||
}, | ||
// lines | ||
line: function(char = '-', length = 30) { | ||
if (typeof(char) == 'object') { | ||
this.e("ERROR: the line() function can't accept objects!"); | ||
char = '-'; | ||
} else { | ||
if (length > 100) { | ||
// this.w("WARNING: Do you really need the line's length more than 100 ?"); | ||
} | ||
var theLine = ""; | ||
for (var i = 0; i < length; i++) { | ||
theLine = theLine + char; | ||
}; | ||
console.log(theLine); | ||
} | ||
}, | ||
dline: function(char = '-', length = 30) { | ||
if (typeof(char) == 'object') { | ||
this.e("ERROR: the line() functions can't accept objects!"); | ||
char = '-'; | ||
} else { | ||
if (length > 100) { | ||
// this.w("WARNING: Do you really need the line's length more than 100 ?"); | ||
} | ||
var theLine = ""; | ||
for (var i = 0; i < length; i++) { | ||
theLine = theLine + char; | ||
}; | ||
console.log(theLine); | ||
} | ||
}, | ||
eline: function(char = '-', length = 30) { | ||
if (typeof(char) == 'object') { | ||
this.e("ERROR: the line() functions can't accept objects!"); | ||
char = '-'; | ||
} else { | ||
if (length > 100) { | ||
// this.w("WARNING: Do you really need the line's length more than 100 ?"); | ||
} | ||
var theLine = ""; | ||
for (var i = 0; i < length; i++) { | ||
theLine = theLine + char; | ||
}; | ||
console.log(chalk.bgRed.white(theLine)); | ||
} | ||
}, | ||
wline: function(char = '-', length = 30) { | ||
if (typeof(char) == 'object') { | ||
this.e("ERROR: the line() functions can't accept objects!"); | ||
char = '-'; | ||
} else { | ||
if (length > 100) { | ||
// this.w("WARNING: Do you really need the line's length more than 100 ?"); | ||
} | ||
var theLine = ""; | ||
for (var i = 0; i < length; i++) { | ||
theLine = theLine + char; | ||
}; | ||
console.log(chalk.bgYellow.black(theLine)); | ||
} | ||
}, | ||
sline: function(char = '-', length = 30) { | ||
if (typeof(char) == 'object') { | ||
this.e("ERROR: the line() functions can't accept objects!"); | ||
char = '-'; | ||
} else { | ||
if (length > 100) { | ||
// this.w("WARNING: Do you really need the line's length more than 100 ?"); | ||
} | ||
var theLine = ""; | ||
for (var i = 0; i < length; i++) { | ||
theLine = theLine + char; | ||
}; | ||
console.log(chalk.bold.green(theLine)); | ||
} | ||
}, | ||
hline: function(char = '-', length = 30) { | ||
if (typeof(char) == 'object') { | ||
this.e("ERROR: the line() functions can't accept objects!"); | ||
char = '-'; | ||
} else { | ||
if (length > 100) { | ||
// this.w("WARNING: Do you really need the line's length more than 100 ?"); | ||
} | ||
var theLine = ""; | ||
for (var i = 0; i < length; i++) { | ||
theLine = theLine + char; | ||
}; | ||
console.log(chalk.bgCyan.black(theLine)); | ||
} | ||
} | ||
@@ -47,0 +138,0 @@ } |
{ | ||
"name": "lme", | ||
"version": "1.0.6", | ||
"version": "1.1.0", | ||
"description": "Simply and beautifully log to console.", | ||
@@ -5,0 +5,0 @@ "main": "index.js", |
@@ -14,2 +14,3 @@ 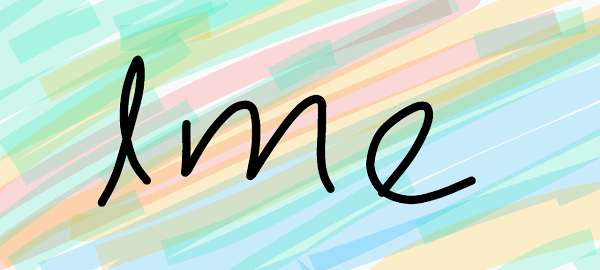 | ||
- Simpler to use than `console.log()` or even `console.log(chalk.red("hi"));` | ||
- Draw lines with just a single function, `lme.line()`. | ||
- Automatically expands `objects` and `arrays`. So that, you don't have to use `JSON.stringify()` anymore. | ||
@@ -22,3 +23,5 @@ - Clean and semantically focused. | ||
 | ||
 | ||
## Install | ||
@@ -44,2 +47,6 @@ | ||
lme.w("Attention! Thank you for your attention."); // used for logging warnings. | ||
lme.line() // used to draw lines | ||
lme.eline() // used to draw lines in error theme. | ||
lme.sline() // used to draw lines in success theme. | ||
``` | ||
@@ -56,3 +63,3 @@ | ||
| `d` | default | default output | `lme.d("hi");` | | ||
| `s` | successes | on success output | `lme.s("hi");` | | ||
| `s` | success | on success output | `lme.s("hi");` | | ||
| `e` | error | on error-ed output | `lme.e("hi");` | | ||
@@ -66,2 +73,38 @@ | `w` | warning | for warnings like output | `lme.w("hi");` | | ||
## Drawing lines with `lme.line()` | ||
**Syntax :** `lme.line(character, length)`. | ||
You can prefix `d`, `s`, `e`, `w`, `h` to the `line()` function to obtain the corresponding color scheme for your line. You can also simply use `lme.line()` which has some default values as described below. | ||
### Argument List | ||
| argument | type | purpose | default value | | ||
| --------------- | ---------- | ----------------------------------------------------------- | ---------------- | | ||
| `character` | `string` | determines which character should be used for drawing lines | `-` | | ||
| `length` | `integer` | length of the line | 30 | | ||
<br> | ||
### Examples | ||
```javascript | ||
lme.line(); | ||
lme.eline("^"); | ||
lme.sline("@", 12); | ||
lme.wline("#", 50); | ||
``` | ||
### APIs for drawing lines | ||
| status | name | when to use | example | | ||
| ----------------- | --------------- | -------------------------- | --------------------- | | ||
| `line` | default | default output | `lme.line();` | | ||
| `dline` | same as line | default output | `lme.d("hi");` | | ||
| `sline` | successe | on success output | `lme.s("hi");` | | ||
| `eline` | error | on error-ed output | `lme.e("hi");` | | ||
| `wline` | warning | for warnings like output | `lme.w("hi");` | | ||
| `hline` | highlight | for highlighting an output | `lme.h("hi");` | | ||
<br> | ||
<br> | ||
More configurations are on its way.<br> | ||
@@ -68,0 +111,0 @@ If you wish to file any feature/bugs, mention it on [issues](https://github.com/vajahath/lme/issues).<br> |
18
test.js
// sample tests | ||
/** | ||
run: | ||
node test.js | ||
*/ | ||
const lme = require('./index'); | ||
@@ -15,2 +22,11 @@ | ||
lme.d('hello'); | ||
lme.h('hello'); | ||
lme.h('hello'); | ||
lme.d('\n'); | ||
// lme.line('*', 150); | ||
lme.dline('*',30); | ||
lme.line('*',12); | ||
lme.eline("#"); | ||
lme.wline({yu:1}); | ||
lme.sline("/"); | ||
lme.hline({"o":9}); | ||
// lme.eline({ye:"ki"}); |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
123333
11
215
110