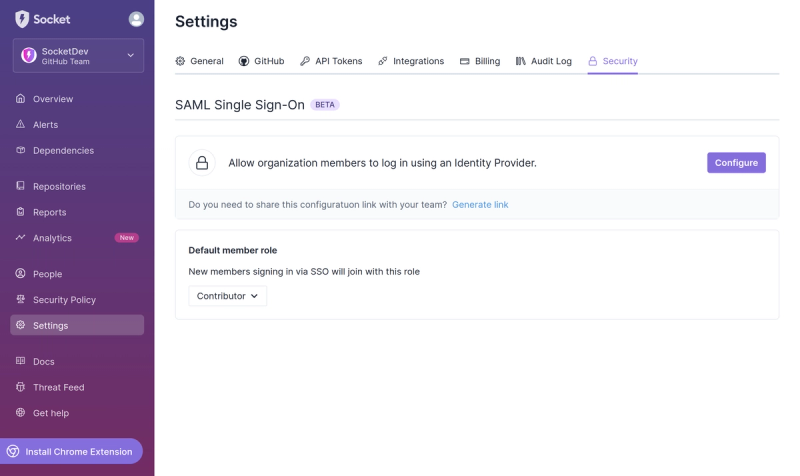
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
lnbits
Advanced tools
Readme
Easy way to add LNBits API to your JS application.
Help me to stack sats! :blush:
bc1qg2sm9tjqy35j50g0zf27s0e8fhflrame5d7q3s
Or donate via Lightning Network!
Using npm:
$ npm install lnbits
Using yarn:
$ yarn add lnbits
const LNBits = require('lnbits').default; // using require
import LNBits from 'lnbits'; // using import
const { wallet, userManager, paywall, withdraw, paylink, tpos } = LNBits({
adminKey: 'd00265e7de5f44f59b2408d9f0564181',
invoiceReadKey: '23e34be59d57408688a74500a3f24f03',
endpoint: 'https://lnbits.com', //default
});
const walletDetails = await wallet.walletDetails();
console.log(walletDetails);
Parameters:
const newInvoice = await wallet.createInvoice({
amount: 10,
memo: 'test',
out: false,
});
console.log(newInvoice);
Parameters:
const newPayInvoice = await wallet.payInvoice({
bolt11: '',
out: true,
});
console.log(newPayInvoice);
Parameters:
const checkinvoice = await wallet.checkInvoice({
payment_hash: '...',
});
console.log(checkinvoice);
const users = await userManager.getUsers();
console.log(users);
Parameters:
const wallets = await userManager.getWallets({
user_id: '355c5110bed24744bebb12aecf8fad14',
});
console.log(wallets);
Parameters:
const tx = await userManager.getTransactions({
wallet_id: '4a18ae4b204044069bd349a37ba0be1d',
});
console.log(tx);
Parameters:
const user = await userManager.createUser({
admin_id: '355c5110bed24744bebb12aecf8fad14',
user_name: 'user',
wallet_name: 'wallet',
});
console.log(user);
Parameters:
const userDeleted = await userManager.deleteUser({
user_id: 'b7cab6e3744347f2b6516510f5d40e9d',
});
console.log(userDeleted);
Parameters:
const walletDeleted = await userManager.deleteWallet({
wallet_id: '0d52c8a832f84f9b86bd993b985e6f10',
});
console.log(walletDeleted);
Parameters:
const extension = await userManager.activeExtension({
userid: '355c5110bed24744bebb12aecf8fad14',
extension: 'usermanager',
active: true,
});
console.log(extension);
const paywalls = await paywall.getPaywalls();
console.log(paywalls);
Parameters:
const paywallNew = await paywall.createPaywall({
amount: 10,
description: 'teste',
memo: 'teste memo',
remembers: false,
url: 'https://teste.com',
});
console.log(paywallNew);
Parameters:
const invoice = await paywall.createInvoice({
amount: 10,
paywall_id: '3UWoiHV7SYCytUjMfG8ySq',
});
console.log(invoice);
Parameters:
const invoiceCheck = await paywall.checkInvoice({
paywall_id: '3UWoiHV7SYCytUjMfG8ySq',
payment_hash:
'e73dc54e857823b7c0bdd3faf6c0f6e8af2b07556fdc304cc8fe7c692a2562e8',
});
console.log(invoiceCheck);
Parameters:
const paywallDeleted = await paywall.deletePaywall({
paywall_id: '3UWoiHV7SYCytUjMfG8ySq',
});
console.log(paywallDeleted);
const withdrawLinks = await withdraw.getLinks({
withdraw_id: '5o57EM9Qty5CLQB2QNjQ2p',
});
console.log(withdrawLinks);
Parameters:
const link = await withdraw.createLink({
title: 'title',
min_withdrawable: 10,
max_withdrawable: 20,
uses: 10,
wait_time: 3600,
is_unique: false,
});
console.log(link);
Parameters:
const linkUpdated = await withdraw.updateLink({
withdraw_id: 'aaWVY3cu655xHxJpYLJhcA',
title: 'title',
min_withdrawable: 10,
max_withdrawable: 20,
uses: 10,
wait_time: 3600,
is_unique: false,
});
console.log(linkUpdated);
Parameters:
const linkDeleted = await withdraw.deleteLink({
withdraw_id: 'aaWVY3cu655xHxJpYLJhcA',
});
console.log(linkDeleted);
const tposs = await tpos.getTPoS();
console.log(tposs);
Parameters:
const tposNew = await tpos.createTPoS({
currency: 'usd',
name: 'teste tpos',
});
console.log(tposNew);
Parameters:
const tposDeleted = await tpos.deleteTPoS({
tpos_id: 'PCXNcLsoLSaBhUybxHfoCN',
});
console.log(tposDeleted);
Pull requests are welcome! For major changes, please open an issue first to discuss what you would like to change.
FAQs
NPM Package for LNBits.com API.
The npm package lnbits receives a total of 33 weekly downloads. As such, lnbits popularity was classified as not popular.
We found that lnbits demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.