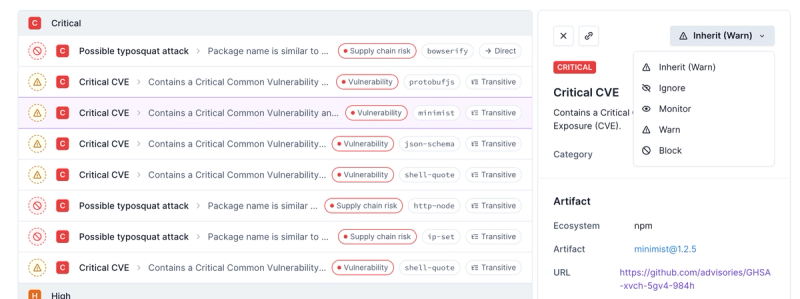
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
loader-cache
Advanced tools
Readme
Register loader functions that dynamically read, parse or otherwise transform file contents when the name of the loader matches a file extension. You can also compose loaders from other loaders.
npm i loader-cache --save
// register a loader for parsing YAML
loaders.register('yaml', function(fp) {
return YAML.safeLoad(fp);
});
// register a loader to be used in other loaders
loaders.register('read', function(fp) {
return fs.readFileSync(fp, 'utf8');
});
// create a new loader from the `yaml` and `read` loaders.
loaders.register('yml', ['read', 'yaml']);
// the `.load()` method calls any loaders registered
// to the `ext` on the given filepath
loaders.load('config.yml');
npm test
var loaders = require('loader-cache');
Create a new instance of Loaders
var Loaders = require('loader-cache');
var loaders = new Loaders();
Array of supported loader types as a convenience for creating utility functions to dynamically choose loaders. Currently supported types are:
sync
async
promise
stream
Register the given loader callback fn
as ext
. Any arbitrary name can be assigned to a loader, however, the loader will only be called when either: a. ext
matches the file extension of a path passed to the .load()
method, or b. ext
is an arbitrary name passed on the loader stack of another loader. Example below.
ext
{String|Array}: File extension or name of the loader.fn
{Function|Array}: A loader function, or create a loader from other others by passing an array of names.returns
{Object} Loaders
: to enable chainingExamples
// register a loader for parsing YAML
loaders.register('yaml', function(fp) {
return YAML.safeLoad(fp);
});
// register a loader to be used in other loaders
loaders.register('read', function(fp) {
return fs.readFileSync(fp, 'utf8');
});
// create a new loader from the `yaml` and `read` loaders.
loaders.register('yml', ['read', 'yaml']);
Register the given async loader callback fn
as ext
. Any arbitrary name can be assigned to a loader, however, the loader will only be called when either: a. ext
matches the file extension of a path passed to the .load()
method, or b. ext
is an arbitrary name passed on the loader stack of another loader. Example below.
ext
{String|Array}: File extension or name of the loader.fn
{Function|Array}: A loader function with a callback parameter, or create a loader from other others by passing an array of names.returns
{Object} Loaders
: to enable chainingExamples
// register an async loader for parsing YAML
loaders.registerAsync('yaml', function(fp, next) {
next(null, YAML.safeLoad(fp));
});
// register a loader to be used in other loaders
loaders.registerAsync('read', function(fp, next) {
fs.readFile(fp, 'utf8', next);
});
// create a new loader from the `yaml` and `read` loaders.
loaders.registerAsync('yml', ['read', 'yaml']);
Register the given promise loader callback fn
as ext
. Any arbitrary name can be assigned to a loader, however, the loader will only be called when either: a. ext
matches the file extension of a path passed to the .load()
method, or b. ext
is an arbitrary name passed on the loader stack of another loader. Example below.
ext
{String|Array}: File extension or name of the loader.fn
{Function|Array}: A loader function that returns a promise, or create a loader from other others by passing an array of names.returns
{Object} Loaders
: to enable chainingExamples
var Promise = require('bluebird');
// register an promise loader for parsing YAML
loaders.registerPromise('yaml', function(fp) {
var deferred = Promise.pending();
process.nextTick(function () {
deferred.fulfill(YAML.safeLoad(fp));
});
return deferred.promise;
});
// register a loader to be used in other loaders
loaders.registerPromise('read', function(fp) {
var Promise = require('bluebird');
var deferred = Promise.pending();
fs.readFile(fp, 'utf8', function (err, content) {
deferred.fulfill(content);
});
return deferred.promise;
});
// create a new loader from the `yaml` and `read` loaders.
loaders.registerPromise('yml', ['read', 'yaml']);
Register the given stream loader callback fn
as ext
. Any arbitrary name can be assigned to a loader, however, the loader will only be called when either: a. ext
matches the file extension of a path passed to the .load()
method, or b. ext
is an arbitrary name passed on the loader stack of another loader. Example below.
ext
{String|Array}: File extension or name of the loader.fn
{Stream|Array}: A stream loader, or create a loader from other others by passing an array of names.returns
{Object} Loaders
: to enable chainingExamples
// register an stream loader for parsing YAML
loaders.registerStream('yaml', es.through(function(fp) {
this.emit('data', YAML.safeLoad(fp));
});
// register a loader to be used in other loaders
loaders.registerStream('read', function(fp) {
fs.readFile(fp, 'utf8', function (err, content) {
this.emit('data', content);
});
});
// create a new loader from the `yaml` and `read` loaders.
loaders.registerStream('yml', ['read', 'yaml']);
loaders
{Array}: Names of stored loaders to add to the stack.type=sync
{String}returns
{Array}: Array of loadersCreate a loader stack of the given type
from an
array of loaders
.
Run loaders associated with ext
of the given filepath.
fp
{String}: File path to load.options
{String}: Options to pass to whatever loaders are defined.returns
: {String}Example
// this will run the `yml` loader from the `.compose()` example
loaders.load('config.yml');
Run async loaders associated with ext
of the given filepath.
fp
{String}: File path to load.options
{Object}: Options to pass to whatever loaders are defined.cb
{Function}: Callback to indicate loading has finishedreturns
: {String}Example
// this will run the `yml` async loader from the `.compose()` example
loaders.loadAsync('config.yml', function (err, obj) {
// do some async stuff
});
Run promise loaders associated with ext
of the given filepath.
fp
{String}: File path to load.options
{Object}: Options to pass to whatever loaders are defined.returns
{Promise}: a promise that will be fulfilled laterExample
// this will run the `yml` promise loader from the `.compose()` example
loaders.loadPromise('config.yml')
.then(function (results) {
// do some promise stuff
});
Run stream loaders associated with ext
of the given filepath.
fp
{String}: File path to load.options
{Object}: Options to pass to whatever loaders are defined.returns
{Stream}: a stream that will be fulfilled laterExample
// this will run the `yml` stream loader from the `.compose()` example
loaders.LoadStream('config.yml')
.pipe(foo())
.on('data', function (results) {
// do stuff
});
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue
Jon Schlinkert
Copyright (c) 2014 Jon Schlinkert
Released under the MIT license
This file was generated by verb on December 03, 2014.
FAQs
Register loader functions that dynamically read, parse or otherwise transform file contents when the name of the loader matches a file extension. You can also compose loaders from other loaders.
The npm package loader-cache receives a total of 190 weekly downloads. As such, loader-cache popularity was classified as not popular.
We found that loader-cache demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.