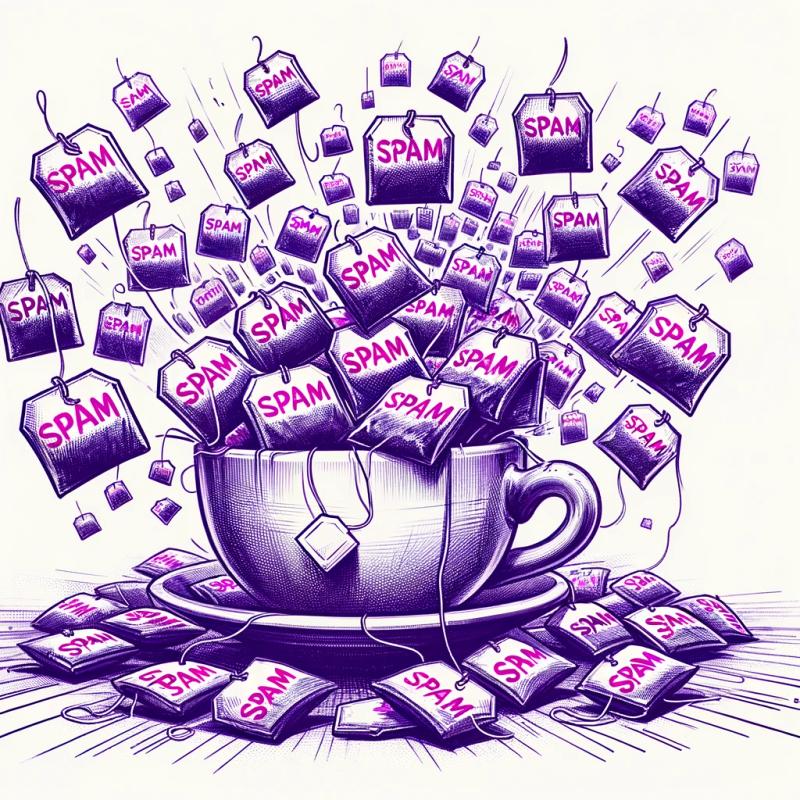
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
lodash.trim
Advanced tools
Readme
The lodash method _.trim
exported as a Node.js module.
Using npm:
$ {sudo -H} npm i -g npm
$ npm i --save lodash.trim
In Node.js:
var trim = require('lodash.trim');
See the documentation or package source for more details.
FAQs
The lodash method `_.trim` exported as a module.
The npm package lodash.trim receives a total of 123,813 weekly downloads. As such, lodash.trim popularity was classified as popular.
We found that lodash.trim demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.