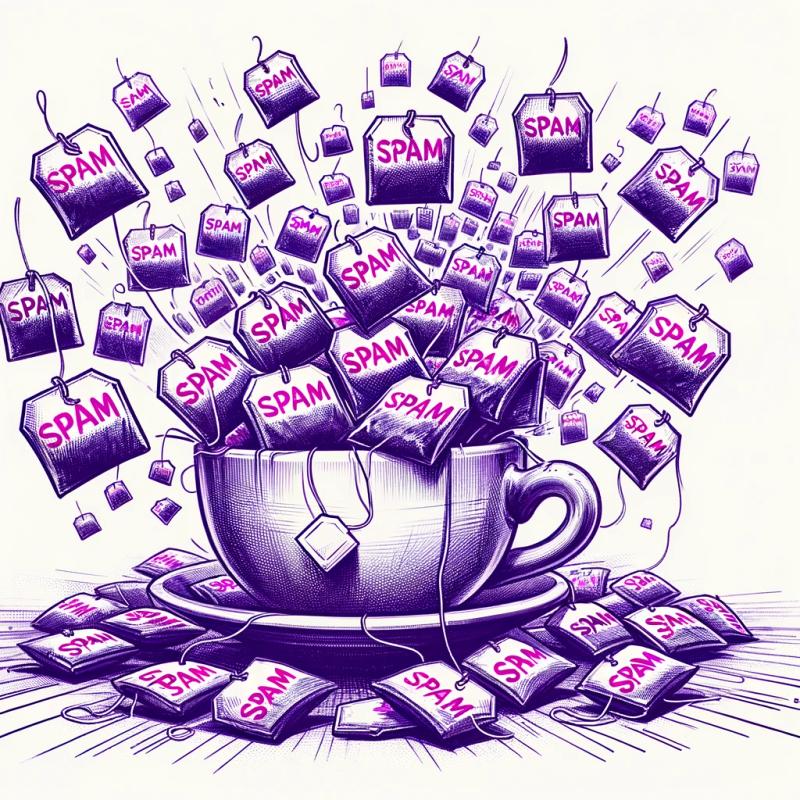
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
merge-options
Advanced tools
Readme
Merge Option Objects
merge-options
considers plain objects as Option Objects, everything else as Option Values.
$ npm install --save merge-options
const mergeOptions = require('merge-options');
mergeOptions({foo: 0}, {bar: 1}, {baz: 2}, {bar: 3})
//=> {foo: 0, bar: 3, baz: 2}
mergeOptions({nested: {unicorns: 'none'}}, {nested: {unicorns: 'many'}})
//=> {nested: {unicorns: 'many'}}
mergeOptions({[Symbol.for('key')]: 0}, {[Symbol.for('key')]: 42})
//=> {Symbol(key): 42}
const mergeOptions = require('merge-options').bind({ignoreUndefined: true});
mergeOptions({foo: 'bar'}, {foo: undefined})
//=> {foo: 'bar'}
mergeOptions
recursively merges one or more Option Objects into a new one and returns that. The options
are merged in order, thus Option Values of additional options
take precedence over previous ones.
The merging does not alter the passed option
arguments, taking roughly the following steps:
const defaultOpts = {
fn: () => false, // functions are Option Values
promise: Promise.reject(new Error()), // all non-plain objects are Option Values
array: ['foo'], // arrays are Option Values
nested: {unicorns: 'none'} // {…} is plain, therefore an Option Object
};
const opts = {
fn: () => true, // [1]
promise: Promise.resolve('bar'), // [2]
array: ['baz'], // [3]
nested: {unicorns: 'many'} // [4]
};
mergeOptions(defaultOpts, opts)
//=>
{
fn: [Function], // === [1]
promise: Promise { 'bar' }, // === [2]
array: ['baz'], // !== [3] (arrays are cloned)
nested: {unicorns: 'many'} // !== [4] (Option Objects are cloned)
}
Type: object
Type: boolean
Default: false
Concatenate arrays:
mergeOptions({src: ['src/**']}, {src: ['test/**']})
//=> {src: ['test/**']}
// Via call
mergeOptions.call({concatArrays: true}, {src: ['src/**']}, {src: ['test/**']})
//=> {src: ['src/**', 'test/**']}
// Via apply
mergeOptions.apply({concatArrays: true}, [{src: ['src/**']}, {src: ['test/**']}])
//=> {src: ['src/**', 'test/**']}
Type: boolean
Default: false
Ignore undefined values:
mergeOptions({foo: 'bar'}, {foo: undefined})
//=> {foo: undefined}
// Via call
mergeOptions.call({ignoreUndefined: true}, {foo: 'bar'}, {foo: undefined})
//=> {foo: 'bar'}
// Via apply
mergeOptions.apply({ignoreUndefined: true}, [{foo: 'bar'}, {foo: undefined}])
//=> {foo: 'bar'}
MIT © Michael Mayer
FAQs
Merge Option Objects
The npm package merge-options receives a total of 1,223,685 weekly downloads. As such, merge-options popularity was classified as popular.
We found that merge-options demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.